Build a 4-digit BCD (binary-coded decimal) counter. Each decimal digit is encoded using 4 bits: q[3:0] is the ones digit, q[7:4] is the tens digit, etc. For digits [3:1], also output an enable signal indicating when each of the upper three digits should be incremented.
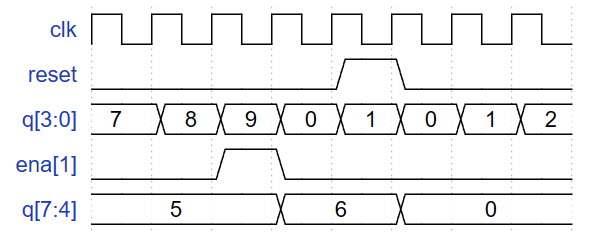
题目网站
module top_module (
input clk,
input reset, // Synchronous active-high reset
output [3:1] ena,
output [15:0] q);
reg [3:0]ones;
reg [3:0]tens;
reg [3:0]hundreds;
reg [3:0]thousands;
always@(posedge clk)begin
if(reset)begin
ones<=4'b0;
end
else begin
if(ones==4'd9)begin
ones<=4'b0;
end
else begin
ones<=ones+4'd1;
end
end
end
always@(posedge clk)begin
if(reset)begin
tens<=4'b0;
end
else begin
if(ones==4'd9&&tens==4'd9)begin
tens<=4'b0;
end
else if(ones==4'd9)begin
tens<=tens+4'd1;
end
end
end
always@(posedge clk)begin
if(reset)begin
hundreds<=4'b0;
end
else begin
if(ones==4'd9&&tens==4'd9&&hundreds==4'd9)begin
hundreds<=4'b0;
end
else if(ones==4'd9&&tens==4'd9)begin
hundreds<=hundreds+4'd1;
end
end
end
always@(posedge clk)begin
if(reset)begin
thousands<=4'b0;
end
else begin
if(ones==4'd9&&tens==4'd9&&hundreds==4'd9&&thousands==4'd9)begin
thousands<=4'b0;
end
else if(ones==4'd9&&tens==4'd9&&hundreds==4'd9)begin
thousands<=thousands+4'd1;
end
end
end //上面的四个模块分别是对个十百千位进行逐位递增判断
assign q={thousands,hundreds,tens,ones}; //对最终输出开始赋值
assign ena[1] = (ones == 4'd9) ? 1'b1 : 1'b0;
assign ena[2] = ((ones == 4'd9) && (tens == 4'd9)) ? 1'b1 : 1'b0;
assign ena[3] = ((ones == 4'd9) && (tens == 4'd9) && (hundreds == 4'd9)) ? 1'b1 : 1'b0; //与上一题一致
endmodule