python 21 days
python 21 days
钻石继承、多态、封装、几个装饰器函数
钻石继承:

class A: def func(self):print('in A') class B(A):pass # def func(self):print('in B') class C(A):pass # def func(self):print('in C') class D(B,C):pass # def func(self):print('in D') d = D() d.func()

in A
钻石继承 —— 4个类图形
D->B->C->A

class E: def func(self):print('in E') class F: def func(self):print('in F') class B(E):pass # def func(self):print('in B') class C(F): def func(self):print('in C') class D(B,C):pass # def func(self):print('in D') d = D() d.func()

in E

class A: def func(self): print('in A') class E(A): def func(self):print('in E') class F(A): def func(self):print('in F') class B(E):pass # def func(self):print('in B') class C(F): def func(self):print('in C') class D(B,C):pass # def func(self):print('in D') print(D.mro())

[<class '__main__.D'>, <class '__main__.B'>, <class '__main__.E'>, <class '__main__.C'>, <class '__main__.F'>, <class '__main__.A'>, <class 'object'>]
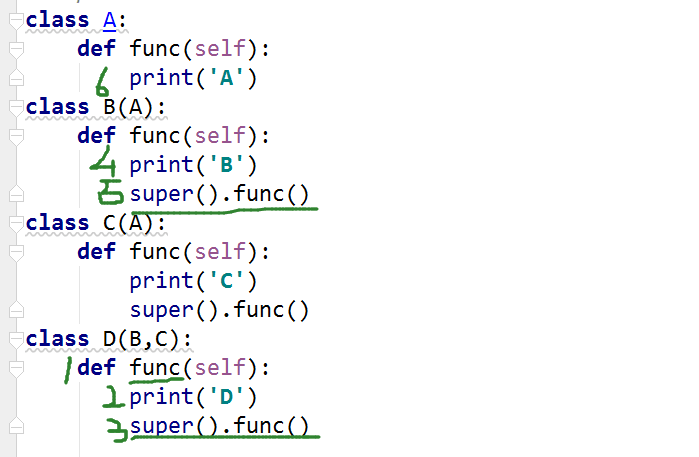
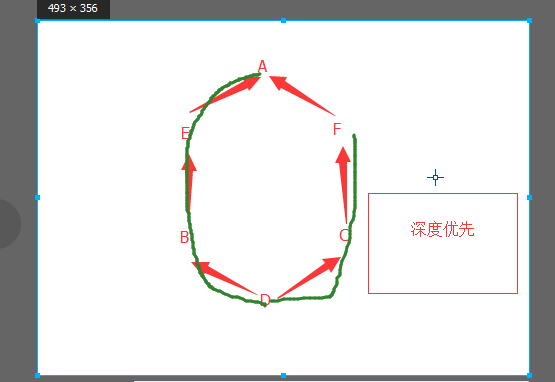
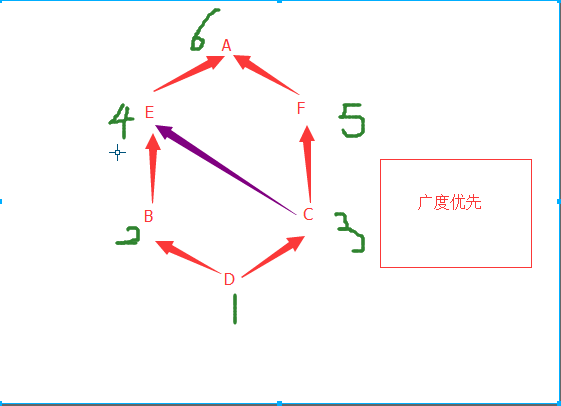
super:

class A: def func(self): print('A') class B(A): def func(self): print('B') super().func() class C(A): def func(self): print('C') super().func() class D(B,C): def func(self): print('D') super().func() D().func() B().func() print(B.mro())

D B C A B A [<class '__main__.B'>, <class '__main__.A'>, <class 'object'>]
super并不是单纯的找父类,和mro顺序是完全对应的。
类 : python3里全部都是新式类
新式类默认继承object
py2里面
新式类 主动继承object
经典类 不主动继承object —— 遵循深度优先遍历算法,没有mro方法,没有super
新式类默认继承object
py2里面
新式类 主动继承object
经典类 不主动继承object —— 遵循深度优先遍历算法,没有mro方法,没有super
Python 弱、强?类型语言 动态强类型语言
弱类型 1+‘2’ 参数的数据类型也不需要指定
强类型 同类型之间可以做运算 参数的数据类型也需要指定
弱类型 1+‘2’ 参数的数据类型也不需要指定
强类型 同类型之间可以做运算 参数的数据类型也需要指定
多态:
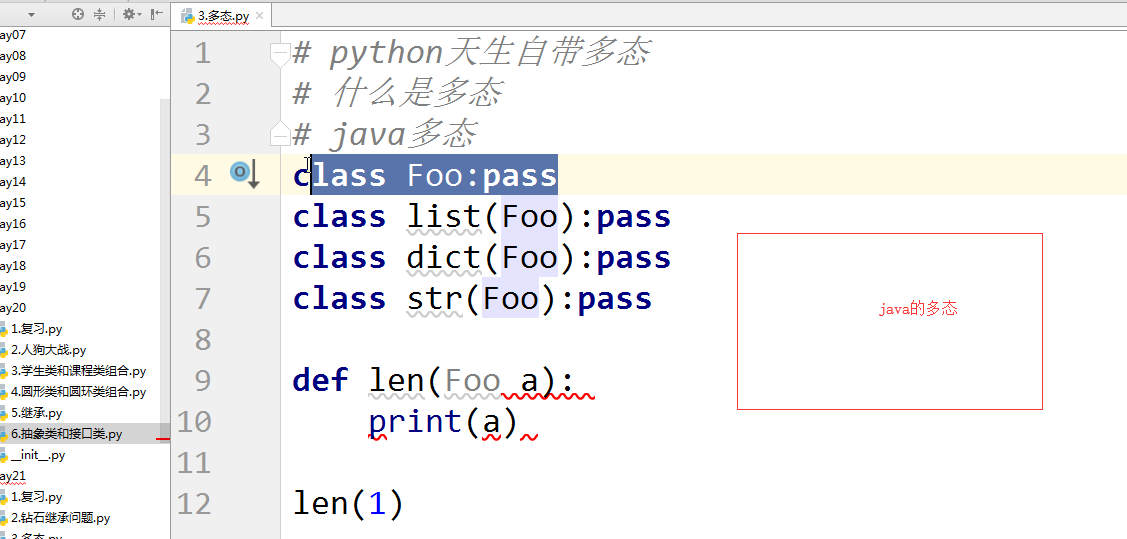
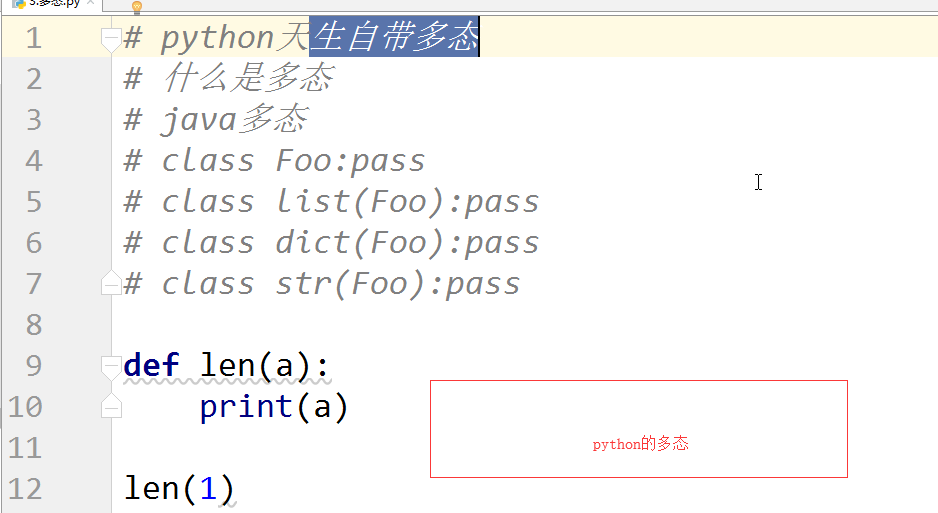
python天生自带多态
什么是多态
java多态
什么是多态
java多态

class Foo:pass class list(Foo):pass class dict(Foo):pass class str(Foo):pass def len(a): print(a) len(1)

1
封装:
封装 成一个函数
封装 成一个类
封装 面向对象的特性
class A:
__静态属性 = 'aaa' # 私有的静态属性
print(__静态属性) # __静态属性,_类名__名字
在一个变量之前 加上两个双下划线是有特殊意义的
加上了这个双下划线,这个变量就变成了私有的
class A:
__静态属性 = 'aaa' # 私有的静态属性
print(__静态属性) # __静态属性,_类名__名字
在一个变量之前 加上两个双下划线是有特殊意义的
加上了这个双下划线,这个变量就变成了私有的
print(A.__静态属性) # 报错 私有的名字不能在类的外部使用
print(A.__dict__)
# print(A._A__静态属性) # 从语法的角度上不允许你直接调用的
A.__wahaha = 'hahaha' # 在一个类的外部是不可能定义一个私有的名字的
print(A.__dict__)
print(A.__dict__)
# print(A._A__静态属性) # 从语法的角度上不允许你直接调用的
A.__wahaha = 'hahaha' # 在一个类的外部是不可能定义一个私有的名字的
print(A.__dict__)
私有的对象属性
练习题:房间的面积

class Room: def __init__(self,owner,id,length,width,height): self.owner = owner self.id = id self.__length = length self.__width = width self.__height = height def area(self): return self.__length * self.__width r = Room('鞠哥哥',302,2,1.5,0.5) print(r.area())

3.0
私有的方法:
不希望从外部去调用这个方法,不独立完成一个功能,而是类整体完成某个功能的一部分
不希望从外部去调用这个方法,不独立完成一个功能,而是类整体完成某个功能的一部分

class Student: # 对密码进行加密 def __init__(self,name,pwd): self.name = name self.__pwd = pwd def __getpwd(self): return self.__pwd[::-1] def login(self): self.__getpwd()

class A: def __init__(self): self.func() def func(self): print('A') class B(A): def func(self): print('B') B()

B

class A: def __init__(self): self.__func() def __func(self): # _A__func print('A') class B(A): def __func(self): print('B') # def __init__(self): # self.__func() # _B__func B()

A
名字
公有的 在类的外部用 内部用 子类用
__私有的 在类的内部用
公有的 在类的外部用 内部用 子类用
__私有的 在类的内部用
私有的概念和面试题 ****
私有的属性和静态属性 ***
私有的方法 **
私有的属性和静态属性 ***
私有的方法 **
几个装饰器函数:
property
classmethod
staticmethod
装饰器
classmethod
staticmethod
装饰器
圆形
半径 r
面积 area
周长 perimeter
半径 r
面积 area
周长 perimeter
求圆的面积:

from math import pi class Circle: def __init__(self,r): self.r = r @property def area(self): return self.r**2*pi c = Circle(5) print(c.area) # ==> c.area()

78.53981633974483
求圆的周长:

from math import pi class Circle: def __init__(self,r): self.r = r @property def perimeter(self): return 2*pi*self.r c = Circle(5) print(c.perimeter)

31.41592653589793
将一个方法伪装成一个属性

class Person: def __init__(self,name): self.__name = name # self.name = name @property def name(self): return self.__name @name.setter def name(self,new): if type(new) is str: self.__name = new alex = Person('alex') print(alex.name) alex.name = 'sb' # 能不能改? —— 不能直接改 print(alex.name)

alex
sb
查看装饰器:

def __init__(self,wahaha): self.__wahaha = wahaha @property # wahaha = property(wahaha) def wahaha(self): print('in wahaha') return self.__wahaha @wahaha.setter # wahaha = wahaha.setter(wahaha) # 也是一个装饰器方法 def wahaha(self,new): self.__wahaha = new d = Demo('wahaa') print(d.wahaha) # 可以被查看

in wahaha wahaa
修改装饰器:

class Demo: def __init__(self,wahaha): self.__wahaha = wahaha @property # wahaha = property(wahaha) def wahaha(self): # print('in wahaha') return self.__wahaha @wahaha.setter # wahaha = wahaha.setter(wahaha) # 也是一个装饰器方法 def wahaha(self,new): self.__wahaha = new d = Demo('wahaa') print(d.wahaha) # 可以被查看 d.wahaha = 123 # 可以被修改 print(d.wahaha)

wahaa
123
苹果降价后:

class Goods: def __init__(self,discount,origin_price): self.__price = origin_price self.__discount = discount @property def price(self): return round(self.__price * self.__discount,2) @price.setter def price(self,new_price): self.__price = new_price # apple = Goods(0.8,10) print(apple.price)

8.0
苹果降价前:

class Goods: def __init__(self,discount,origin_price): self.__price = origin_price self.__discount = discount @property def price(self): return round(self.__price * self.__discount,2) @price.setter def price(self,new_price): self.__price = new_price apple = Goods(0.8,10) apple.price = 12 print(apple.price)

9.6
一个属性可以被查看:

class A:pass a = A() a.name = 'egon' print(a.__dict__)

{'name': 'egon'}
一个属性可以被删除:

class A:pass a = A() a.name = 'egon' print(a.__dict__) del a.name print(a.__dict__)

{'name': 'egon'} {}
用装饰器写入文件句柄:

class A: def __init__(self,path): self.__f = open(path,'w') @property def f(self):return self.__f @f.deleter def f(self): # 所有的借用操作系统的资源,在删除一个变量之前都必须先归还资源 self.close() del self.__f # def write(self,content): self.__f.write(content) def close(self): self.__f.close() obj = A('wahaha') obj.write('wahahayawahaha') obj.close()
property ***
setter **
deleter *
苹果装饰器的价格:

lass Goods: __discount = 0.8 def __init__(self,origin_price): self.__price = origin_price @property def price(self): return round(self.__price * Goods.__discount,2) @price.setter def price(self,new_price): self.__price = new_price @classmethod def change_discount(cls,new_discount): # print(cls,Goods) #Goods.__discount = new_discount cls.__discount = new_discount # # apple = Goods(10) # apple.change_discount(1) Goods.change_discount(0.7) apple = Goods(10) print(apple.price)

7.0
装饰器学生登陆的行为:

lass Student: def __init__(self,name,sex): self.name = name self.sex = sex @staticmethod # 相当于函数 def login(): name = input('鞠哥哥') if name == 'alex':print('登录成功') # 学生的登陆行为 print(Student.login())

鞠哥哥
method 方法 —— 函数 由实例化对象去调用
property 伪装成属性的方法 —— 特性 由实例化对象去调用
classmethod 类方法 由类调用,只使用类中的静态变量
staticmethod 静态方法 由类调用,一个方法既不会用到对象的属性,也不会用到类的属性。
property 伪装成属性的方法 —— 特性 由实例化对象去调用
classmethod 类方法 由类调用,只使用类中的静态变量
staticmethod 静态方法 由类调用,一个方法既不会用到对象的属性,也不会用到类的属性。