# include <iostream> # include <cstdio> # include <vector> # include <sstream> using namespace std; struct node { int key; node* parent; node* right; node* left; }; //中序遍历,从小到大遍历,即遍历完左子树再访问根节点,再遍历右子树。 void in_order_walk(node* p) { if(p == NULL) return; if(p->left != NULL) in_order_walk(p->left); printf("%d ",p->key); if(p->right != NULL) in_order_walk(p->right); } //插入 void insert(node** root, int key) { node *p = new node; p->key = key; p->left = p->right = p->parent = NULL; if((*root) == NULL) { *root = p; return; } if((*root)->left == NULL && key < (*root)->key) { p->parent = *root; (*root)->left = p; return; } if((*root)->right == NULL && key > (*root)->key) { p->parent = *root; (*root)->right = p; return; } if(key < (*root)->key) insert(&(*root)->left, key); else if(key > (*root)->key) insert(&(*root)->right, key); else return; } //初始化 void create(node** root, int* a, int length) { for(int i=0; i<length; ++i) insert(root, a[i]); } //查找key node* search(node* root, int key) { if(root == NULL) return NULL; if(key > root->key) return search(root->right, key); else if(key < root->key) return search(root->left, key); else return root; } //查询最小值 node* searchMin(node* root) { if(root == NULL) return NULL; if(root->left == NULL) return root; else return searchMin(root->left); } //查询最大值 node* searchMax(node* root) { if(root == NULL) return NULL; if(root->right == NULL) return root; else return searchMax(root->right); } //查找前驱节点 node* searchPredecessor(node* p) { if(p == NULL) return NULL; else if(p->left) return searchMax(p->left); else { while(p) { if(p->parent->right == p) break; p = p->parent; } return p->parent; } } //查找后继节点 node* searchSuccessor(node* p) { if(p == NULL) return NULL; else if(p->right)//有右孩子,返回右子树的最小值 return searchMin(p->right); else//没有右孩子,找到最近的祖先,使祖先的左孩子也为p的祖先,找前驱节点同理。 { while(p) { if(p->parent->left == p) break; p = p->parent; } return p->parent; } } //删除节点 bool delete_node(node** root, int key) { int temp; node* q = NULL; node* p = search(*root, key); if(!p) return false; if(p->left==NULL && p->right==NULL)//没有孩子,直接删除。 { if(p->parent == NULL) { delete(p); *root = NULL; } else { if(p->parent->left == p) p->parent->left = NULL; else p->parent->right = NULL; delete(p); } } else if(p->left==NULL && p->right)//没有左孩子,右孩子接上去 { p->right->parent = p->parent; if(p->parent == NULL) *root = p->right; else if(p->parent->left == p) p->parent->left = p->right; else p->parent->right = p->right; delete(p); } else if(p->left && p->right==NULL)//没有右孩子,左孩子接上去 { p->left->parent = p->parent; if(p->parent == NULL) *root = p->left; else if(p->parent->left == p) p->parent->left = p->left; else p->parent->right = p->left; delete(p); } else//有两个孩子,用右子树的最小值代替 { q = searchSuccessor(p); temp = q->key; delete_node(root, q->key); p->key = temp; } return true; } int main() { int a[10] = {9,1,2,5,4,3,10,8,7,6}; node* root = NULL; create(&root, a, 10); in_order_walk(root); return 0; }
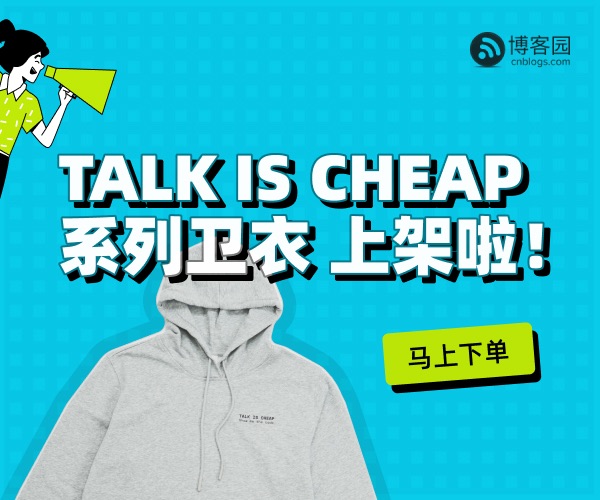