首先感谢@CFBDSIR2149的笔记整理,救我狗命(她的博客https://www.cnblogs.co/myqD-blogs/)
以下是我参考各种资料课件(主要是mooc)等,只针对我自己的注意点,并且可能有错误的地方,也欢迎指出(如果有人看的话)
mooc讲课老师的微博http://weibo.com/guoweiofpku,随笔大多都参考他的ppt
看到啥写啥,没有条理,甚至有我个人的日常生活记录
1.this指针的作用:非静态成员函数中指向成员函数所作用的对象,静态成员函数中不能用
class A
{
int i;
public:
void Hello() { cout << i << "hello" << endl; }
}; void Hello(A * this ) { cout << this->i << "hello"
<< endl; }
//this若为NULL,则出错!!
int main()
{
A * p = NULL;
p->Hello();
} // 输出:hello 19
2.类的非静态成员函数,真实的参数比所写的参数多1(多的是this指针)
3.静态成员变量为所有对象共享。
4.sizeof运算符不会计算静态成员变量,且静态成员变量不需要通过对象就能访问
5.关于如何访问静态成员
1) 类名::成员名
CRectangle::PrintTotal();
2) 对象名.成员名
CRectangle r; r.PrintTotal();
3) 指针->成员名
CRectangle * p = &r; p->PrintTotal();
4) 引用.成员名
CRectangle & ref = r; int n = ref.nTotalNumber;
6.静态成员函数本质上是全局函数。
7.在静态成员函数中,不能访问非静态成员变量,也不能调用非静态成员函数
8.补充插入!
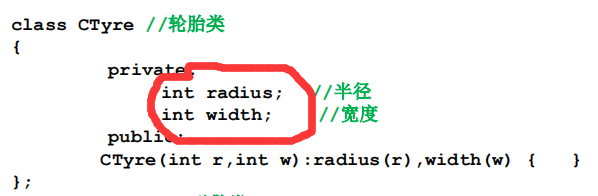
红圈里的是类中的成员变量,而CTyre a; a是CTyre的成员对象
9.有成员对象的类是封闭类
实例:
class CTyre {
public:
CTyre() { cout << "CTyre contructor" << endl; }
~CTyre() { cout << "CTyre destructor" << endl; }
};
class CEngine {
public:
CEngine() { cout << "CEngine contructor" << endl; }
~CEngine() { cout << "CEngine destructor" << endl; }
};
class CCar {
private:
CEngine engine;
CTyre tyre;
public:
CCar( ) { cout << “CCar contructor” << endl; }
~CCar() { cout << "CCar destructor" << endl; }
};
int main(){
CCar car;
return 0;
}
//输出结果:
CEngine contructor
CTyre contructor
CCar contructor
CCar destructor
CTyre destructor
CEngine destructor
10.b
11.友元函数: 一个类的友元函数可以访问该类的私有成员
class B {
public:
void function();
};
class A {
friend void B::function();
};
12.友元类: 如果A是B的友元类,那么A的成员函数可以访问B的私有成员。友元类之间的关系不能传递。不能继承
class CCar
{
private:
int price;
friend class CDriver; //声明CDriver为友元类
};
class CDriver
{
public:
CCar myCar;
void ModifyCar() {//改装汽车
myCar.price += 1000;//因CDriver是CCar的友元类,
//故此处可以访问其私有成员
}
};
int main(){ return 0; }
13. 常量成员函数如果不希望某个对象的值被改变,则定义该对象的时候可以在前面加 const关键字。在类的成员函数说明后面可以加const关键字,则该成员函数成为常量成员函数。常量对象只能使用常量成员函数(构造函数,析构函数,又const说明的函数)
class Sample {
private :
int value;
public:
void func() { };
Sample() { }
void SetValue() const {
value = 0; // wrong
func(); //wrong
}
};
const Sample Obj;
Obj.SetValue (); //常量对象上可以使用常量成员函数
14.两个函数,名字和参数表都一样,但是一个是const,一个不是,算重载。
15.mutable成员变量可以在const成员函数中修改的成员变量
class CTest
{
public:
bool GetData() const
{
m_n1++;
return m_b2;
}
private:
mutable int m_n1;
bool m_b2;
};