package com.example.assignment;
import android.app.AlertDialog;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import android.os.Bundle;
import android.app.Activity;
import android.content.DialogInterface;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button buttonSave = (Button)findViewById(R.id.buttonSave);
buttonSave.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String filename = "data.txt";
String content = "helloworld";
FileOutputStream fos = null;
try {
fos = openFileOutput(filename, MODE_PRIVATE);
fos.write(content.getBytes());
}
catch(Exception e) {
e.printStackTrace();
}
finally {
try {
if (fos != null) {
fos.close();
}
}
catch (IOException e) {
e.printStackTrace();
}
}
}
});
Button buttonRead = (Button)findViewById(R.id.buttonRead);
buttonRead.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String content = "";
FileInputStream fis = null;
try {
fis = openFileInput("data.txt");
byte[] buffer = new byte[fis.available()];
fis.read(buffer);
content = new String(buffer);
}
catch (Exception e) {
e.printStackTrace();
}
finally {
try {
if (fis != null) {
fis.close();
}
}
catch (IOException e) {
e.printStackTrace();
}
}
AlertDialog dialog;
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this)
.setTitle("data content")
.setIcon(R.drawable.ic_launcher)
.setMessage(content)
.setPositiveButton("存储数据", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.dismiss();
}
});
dialog = builder.create();
dialog.show();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<Button
android:id="@+id/buttonSave"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="保存数据" />
<Button
android:id="@+id/buttonRead"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="读取数据" />
</LinearLayout>
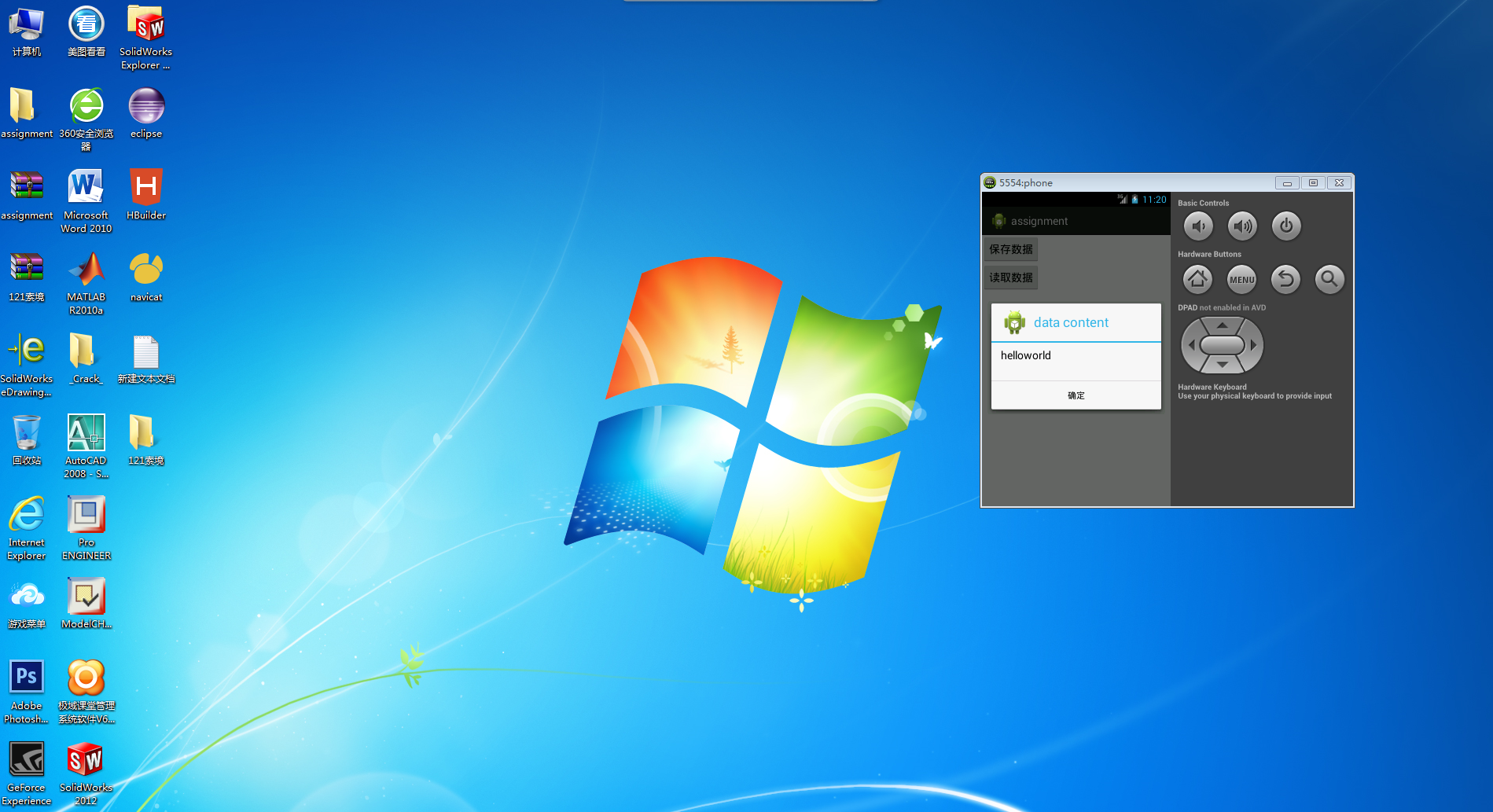
package com.example.assignment;
import android.os.Bundle;
import android.app.Activity;
import android.app.AlertDialog;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.content.DialogInterface;
import android.content.SharedPreferences;
public class LoginActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
SharedPreferences sharedPreferences = getSharedPreferences("data", MODE_PRIVATE);
String username = sharedPreferences.getString("username", "");
String password = sharedPreferences.getString("password", "");
EditText editTextUserName = (EditText)findViewById(R.id.editTextUserName);
editTextUserName.setText(username);
EditText editTextPassword = (EditText)findViewById(R.id.editTextPassword);
editTextPassword.setText(password);
Button buttonSave = (Button)findViewById(R.id.buttonSave);
buttonSave.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
SharedPreferences sharedPreferences = getSharedPreferences("data", MODE_PRIVATE);
SharedPreferences.Editor editor = sharedPreferences.edit();
EditText editTextUserName = (EditText)findViewById(R.id.editTextUserName);
editor.putString("username", editTextUserName.getText().toString());
EditText editTextPassword = (EditText)findViewById(R.id.editTextPassword);
editor.putString("password", editTextPassword.getText().toString());
editor.commit();
}
});
Button buttonLogin = (Button)findViewById(R.id.buttonLogin);
buttonLogin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditText editTextUserName = (EditText)findViewById(R.id.editTextUserName);
String username = editTextUserName.getText().toString();
EditText editTextPassword = (EditText)findViewById(R.id.editTextPassword);
String password = editTextPassword.getText().toString();
AlertDialog dialog;
AlertDialog.Builder builder = new AlertDialog.Builder(LoginActivity.this)
.setTitle("data content")
.setIcon(R.drawable.ic_launcher)
.setMessage("data" + username + "\n" + password + "\n")
.setPositiveButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.dismiss();
}
});
dialog = builder.create();
dialog.show();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.login, menu);
return true;
}
}
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".LoginActivity" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="52px"
android:layout_height="wrap_content"
android:text="账号" />
<EditText
android:id="@+id/editTextUserName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:hint="请输入用户名" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="52px"
android:layout_height="wrap_content"
android:text="密码" />
<EditText
android:id="@+id/editTextPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:hint="请输入密码" />
</LinearLayout>
<Button
android:id="@+id/buttonSave"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="保存" />
<Button
android:id="@+id/buttonLogin"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="登录" />
</LinearLayout>
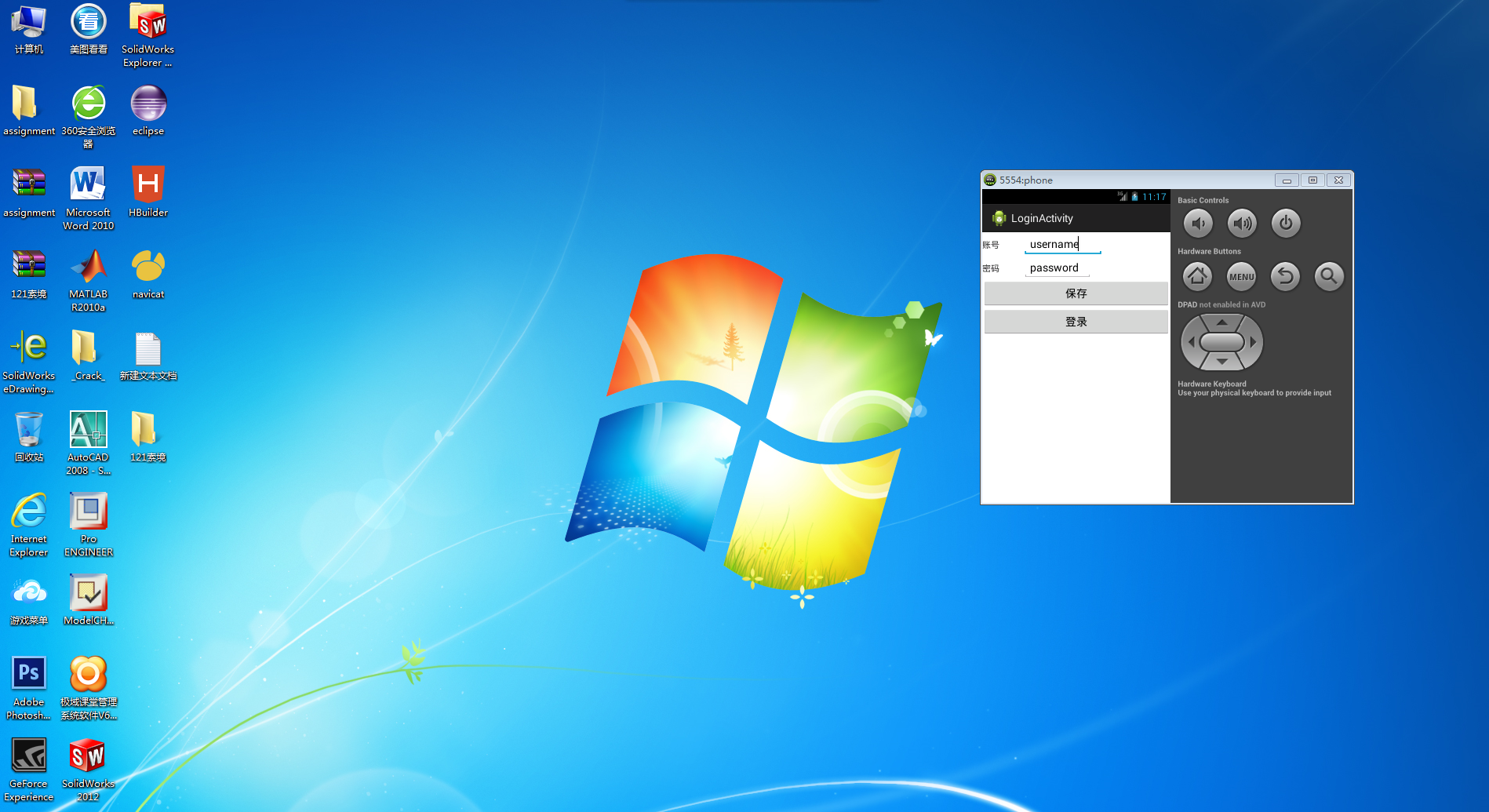