package com.example.myapplication;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class Assignment02_01 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity02_01);
Button button1 = findViewById(R.id.button1);
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(getApplicationContext(), "button1 被点击", Toast.LENGTH_SHORT).show();
}
});
Button button2 = findViewById(R.id.button2);
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(getApplicationContext(), "button2 被点击", Toast.LENGTH_SHORT).show();
}
});
}
public void button0Clicked(View v) {
Toast.makeText(getApplicationContext(), "button0 被点击", Toast.LENGTH_SHORT).show();
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:paddingTop="100dp"
tools:context=".Assignment02_01">
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="button0"
android:id="@+id/button0"
android:onClick="button0Clicked">
</Button>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="button1"
android:id="@+id/button1">
</Button>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="button2"
android:id="@+id/button2">
</Button>
</LinearLayout>
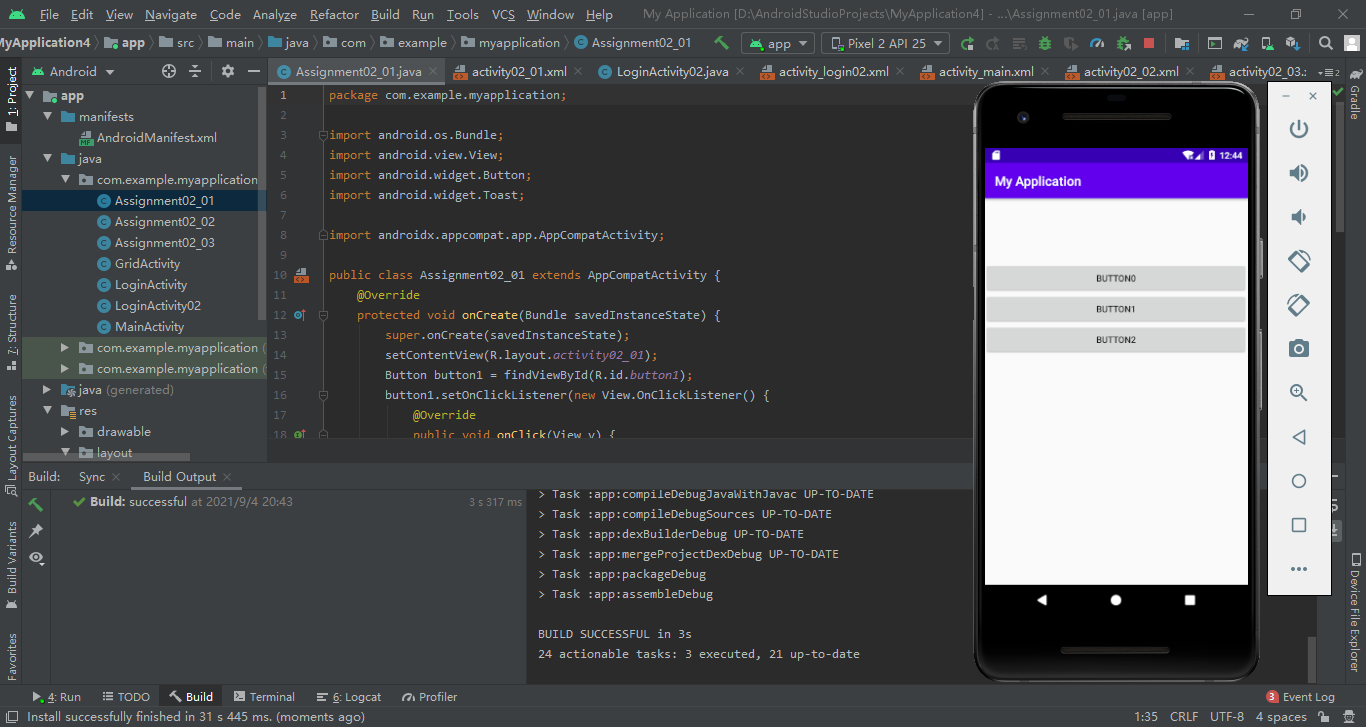
package com.example.myapplication;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
public class Assignment02_02 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity02_02);
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:layout_marginTop="70dp"
android:layout_marginLeft="34dp"
tools:context=".Assignment02_02">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="学历"
android:textSize="50dp">
</TextView>
<RadioGroup
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="初中"
android:textSize="49dp"
></RadioButton>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="高中"
android:textSize="49dp"
></RadioButton>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="专科"
android:textSize="49dp"
></RadioButton>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="本科"
android:textSize="49dp"
></RadioButton>
</RadioGroup>
</LinearLayout>
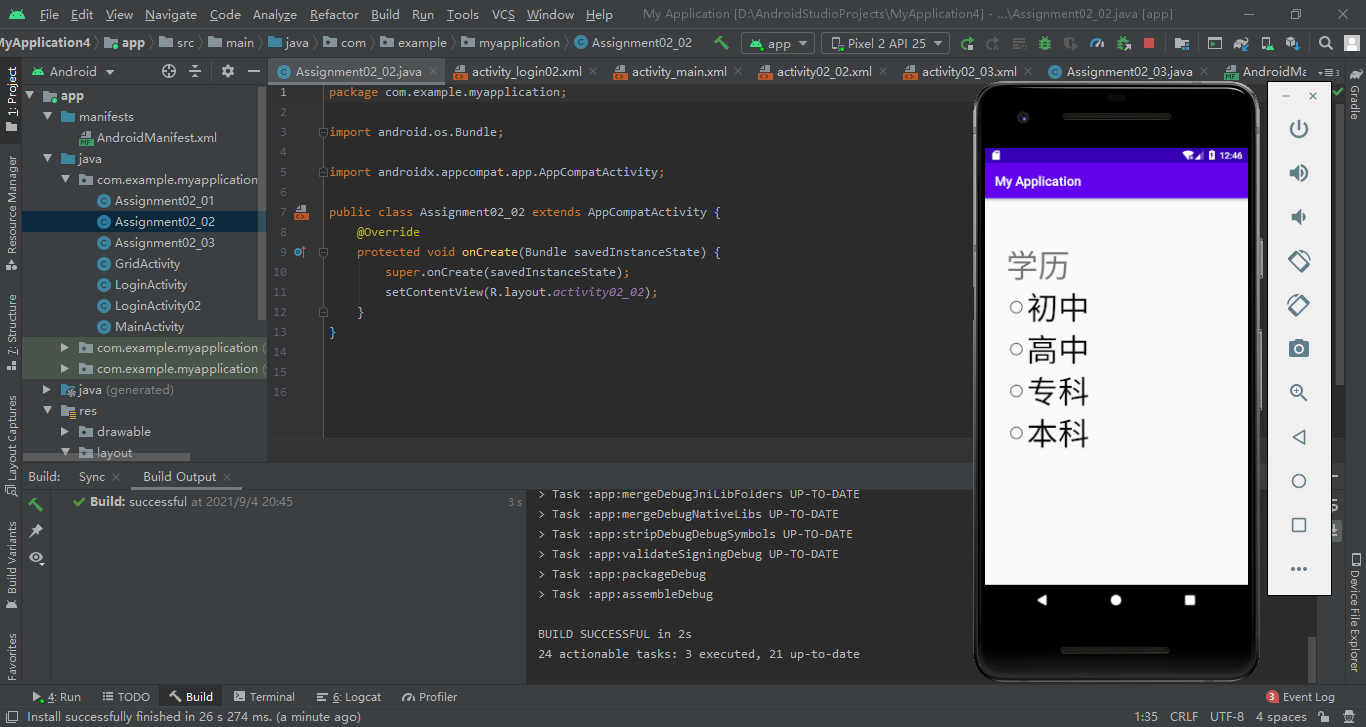
package com.example.myapplication;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
public class Assignment02_03 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity02_03);
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:layout_marginTop="70dp"
android:layout_marginLeft="34dp"
tools:context=".Assignment02_03">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="学过哪些课程"
android:textSize="50dp">
</TextView>
<RadioGroup
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Java"
android:textSize="49dp"
></RadioButton>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Ios"
android:textSize="49dp"
></RadioButton>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Android"
android:textSize="49dp"
></RadioButton>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Html"
android:textSize="49dp"
></RadioButton>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Jsp"
android:textSize="49dp"
></RadioButton>
</RadioGroup>
</LinearLayout>
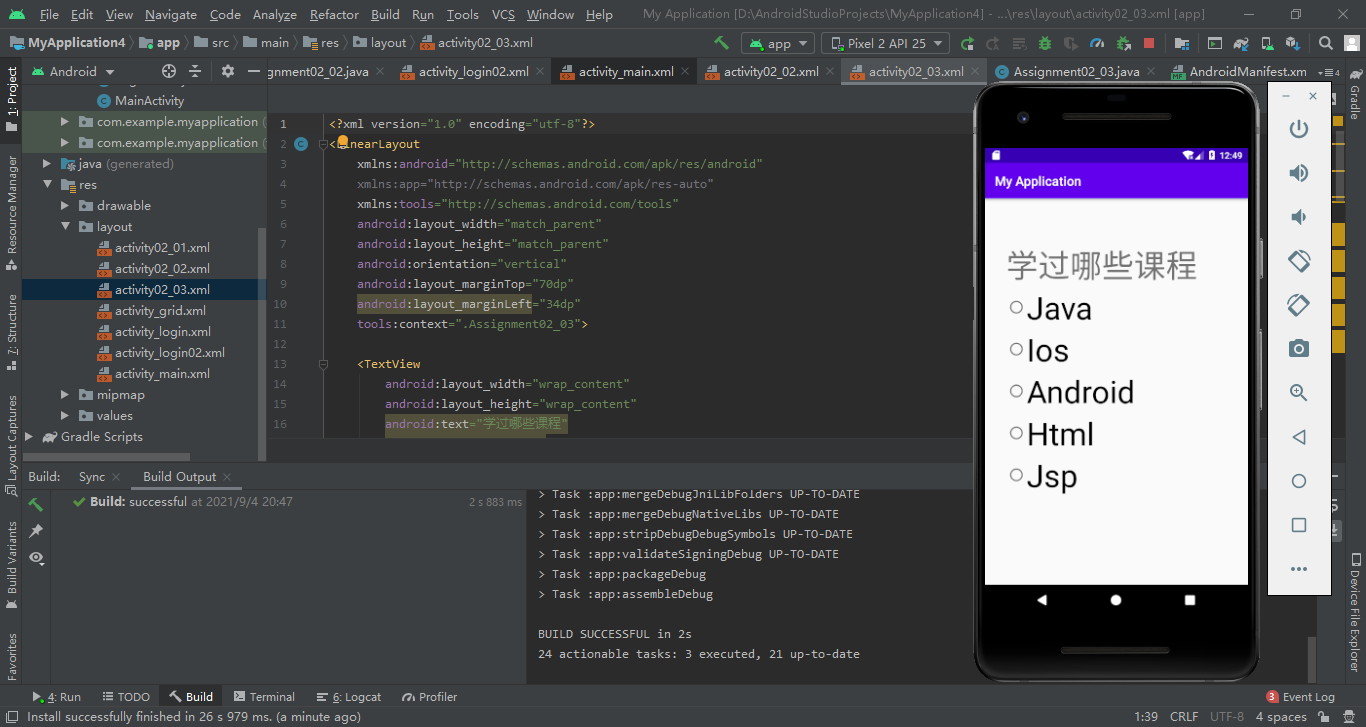
package com.example.myapplication;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class Assignment02_01 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity02_01);
Button button1 = findViewById(R.id.button1);
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(getApplicationContext(), "button1 被点击", Toast.LENGTH_SHORT).show();
}
});
Button button2 = findViewById(R.id.button2);
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(getApplicationContext(), "button2 被点击", Toast.LENGTH_SHORT).show();
}
});
}
public void button0Clicked(View v) {
Toast.makeText(getApplicationContext(), "button0 被点击", Toast.LENGTH_SHORT).show();
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:paddingTop="100dp"
tools:context=".Assignment02_01">
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="button0"
android:id="@+id/button0"
android:onClick="button0Clicked">
</Button>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="button1"
android:id="@+id/button1">
</Button>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="button2"
android:id="@+id/button2">
</Button>
</LinearLayout>
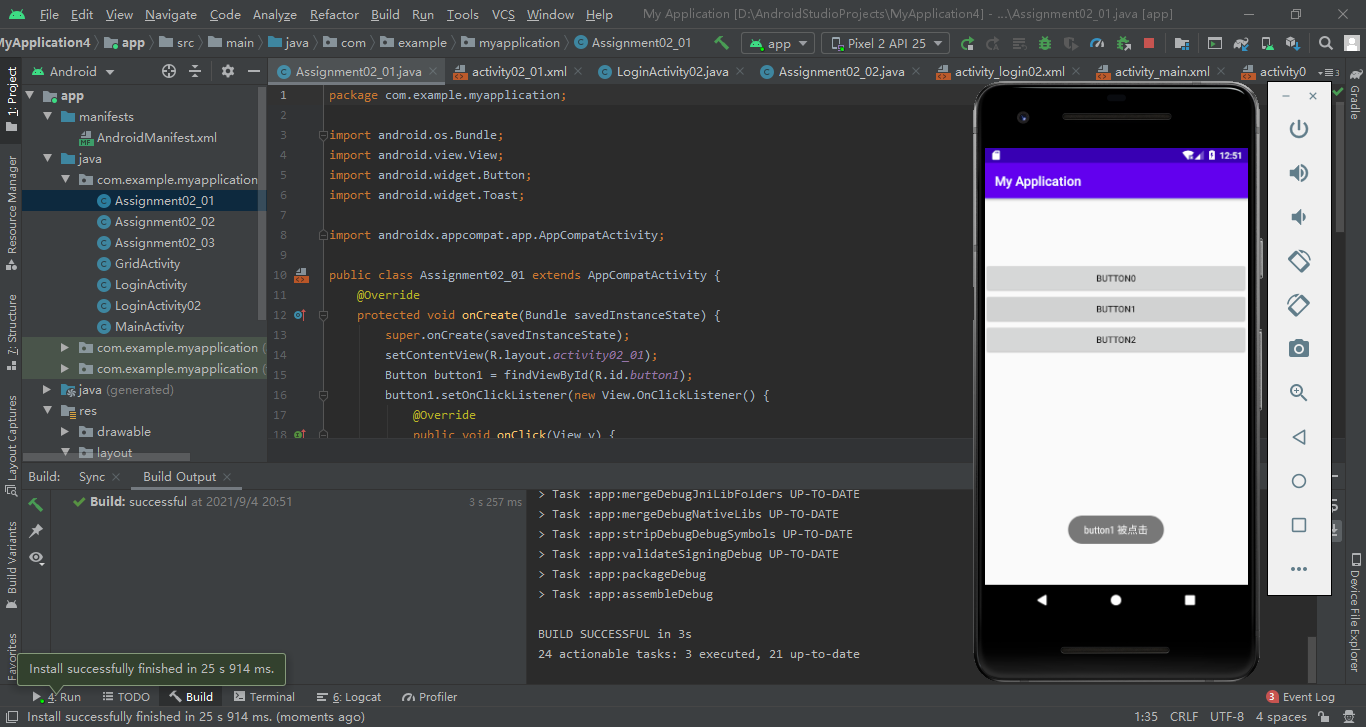
package com.example.myapplication;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
public class LoginActivity02 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login02);
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".LoginActivity02">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:layout_marginTop="25dp">
<ImageView
android:layout_width="232dp"
android:layout_height="232dp"
android:src="@mipmap/card"
android:layout_gravity="center"></ImageView>
<GridLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="用户"
android:textSize="61dp">
</TextView>
<EditText
android:layout_width="241dp"
android:layout_height="wrap_content"
android:textSize="52dp">
</EditText>
</GridLayout>
<GridLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="密码"
android:textSize="61dp">
</TextView>
<EditText
android:layout_width="241dp"
android:layout_height="wrap_content"
android:password="true"
android:textSize="52dp">
</EditText>
</GridLayout>
<Button
android:layout_width="259dp"
android:layout_height="wrap_content"
android:text="登陆"
android:textSize="25dp"
android:layout_gravity="center"
android:layout_marginTop="25dp"></Button>
<Button
android:layout_width="259dp"
android:layout_height="wrap_content"
android:text="不注册, 跳过登陆"
android:textSize="25dp"
android:layout_gravity="center"
android:layout_marginTop="25dp"></Button>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
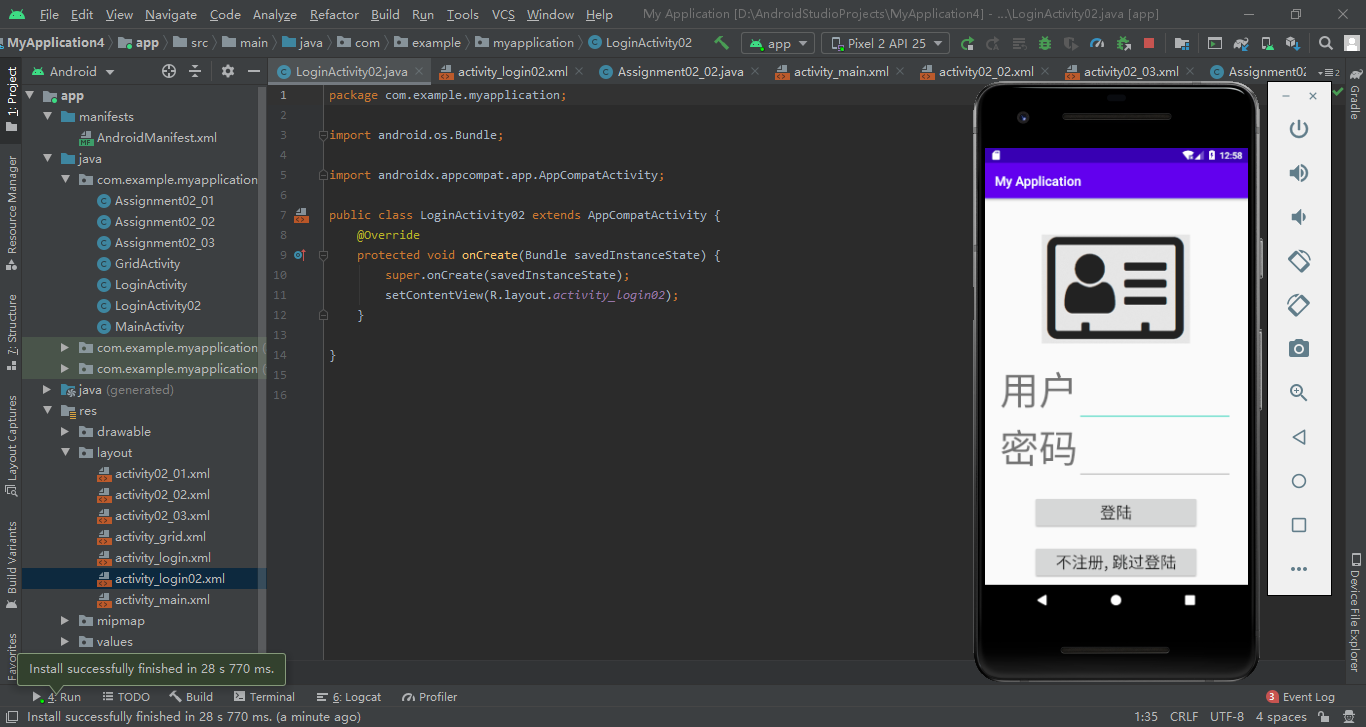