房产管理系统项目结构及后端代码
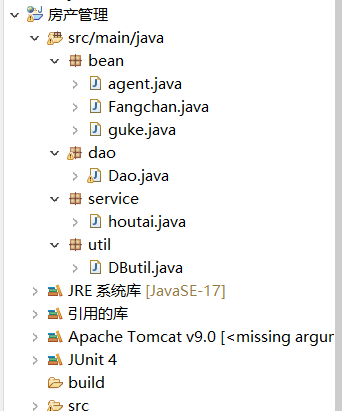
bean层
经纪人agent类
package bean;
public class agent {
private int id;
private String name;
private String address;
private String phone;
@Override
public String toString() {
return "agent [id=" + id + ", name=" + name + ", address=" + address + ", phone=" + phone + "]";
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public agent(int id, String name, String address, String phone) {
super();
this.id = id;
this.name = name;
this.address = address;
this.phone = phone;
}
}
房产信息Fangchan类
package bean;
public class Fangchan {
private int id;
private String huxing;
private String dizhi;
private String nianfen;
private String mianji;
private String baojia;
private String zhuangtai;
private int agent;
private int guke;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getHuxing() {
return huxing;
}
public void setHuxing(String huxing) {
this.huxing = huxing;
}
public String getDizhi() {
return dizhi;
}
public void setDizhi(String dizhi) {
this.dizhi = dizhi;
}
public String getNianfen() {
return nianfen;
}
public void setNianfen(String nianfen) {
this.nianfen = nianfen;
}
public String getMianji() {
return mianji;
}
public void setMianji(String mianji) {
this.mianji = mianji;
}
public String getBaojia() {
return baojia;
}
public void setBaojia(String baojia) {
this.baojia = baojia;
}
public String getZhuangtai() {
return zhuangtai;
}
public void setZhuangtai(String zhuangtai) {
this.zhuangtai = zhuangtai;
}
public int getAgent() {
return agent;
}
public void setAgent(int agent) {
this.agent = agent;
}
public int getGuke() {
return guke;
}
public void setGuke(int guke) {
this.guke = guke;
}
@Override
public String toString() {
return "Fangchan [id=" + id + ", huxing=" + huxing + ", dizhi=" + dizhi + ", nianfen=" + nianfen + ", mianji="
+ mianji + ", baojia=" + baojia + ", zhuangtai=" + zhuangtai + "]";
}
public Fangchan(String huxing, String dizhi, String nianfen, String mianji, String baojia, String zhuangtai,
int agent, int guke) {
super();
this.huxing = huxing;
this.dizhi = dizhi;
this.nianfen = nianfen;
this.mianji = mianji;
this.baojia = baojia;
this.zhuangtai = zhuangtai;
this.agent = agent;
this.guke = guke;
}
public Fangchan (String huxing, String dizhi, String nianfen, String mianji, String baojia, String zhuangtai) {
super();
this.huxing = huxing;
this.dizhi = dizhi;
this.nianfen = nianfen;
this.mianji = mianji;
this.baojia = baojia;
this.zhuangtai = zhuangtai;
}
public Fangchan(int id, String dizhi, String baojia, int guke) {
super();
this.id = id;
this.dizhi = dizhi;
this.baojia = baojia;
this.guke = guke;
}
public Fangchan(int id, int guke) {
super();
this.id = id;
this.guke = guke;
}
public Fangchan(int id, String dizhi, String baojia) {
super();
this.id = id;
this.dizhi = dizhi;
this.baojia = baojia;
}
public Fangchan(int id, String huxing, String dizhi, String nianfen, String mianji, String baojia, String zhuangtai) {
super();
this.id = id;
this.huxing = huxing;
this.dizhi = dizhi;
this.nianfen = nianfen;
this.mianji = mianji;
this.baojia = baojia;
this.zhuangtai = zhuangtai;
}
}
顾客guke类
package bean;
public class guke {
private int id;
private String name;
private String sex;
private String number;
private String phone;
private String address;
private String mima;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getMima() {
return mima;
}
public void setMima(String mima) {
this.mima = mima;
}
public guke(String name, String sex, String number, String phone, String address, String mima) {
super();
this.name = name;
this.sex = sex;
this.number = number;
this.phone = phone;
this.address = address;
this.mima = mima;
}
public guke(int id, String name, String sex, String number, String phone, String address, String mima) {
super();
this.id = id;
this.name = name;
this.sex = sex;
this.number = number;
this.phone = phone;
this.address = address;
this.mima = mima;
}
public guke(int id, String name, String sex) {
super();
this.id = id;
this.name = name;
this.sex = sex;
}
@Override
public String toString() {
return "guke [id=" + id + ", name=" + name + ", sex=" + sex + ", number=" + number + ", phone=" + phone
+ ", address=" + address + ", mima=" + mima + "]";
}
}
dao层Dao类
package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
import bean.Fangchan;
import bean.agent;
import bean.guke;
import util.DButil;
public class Dao {
public boolean addguke(guke e) throws SQLException {
try {
Connection conn=DButil.getconn();
String sql="insert into guke(name,sex,number,phone,address,mima,flag) value(?,?,?,?,?,?,?)";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setString(1,e.getName());
ps.setString(2,e.getSex());
ps.setString(3,e.getNumber());
ps.setString(4,e.getPhone());
ps.setString(5,e.getAddress());
ps.setString(6,e.getMima());
ps.setInt(7,0);
int count=ps.executeUpdate();
if(count>0)
return true;
}catch(Exception k) {
k.printStackTrace();
}
return false;
}
public boolean addfang(Fangchan f) throws SQLException {
try {
Connection conn=DButil.getconn();
String sql="insert into fangchan(huxing,dizhi,nianfen,mianji,baojia,zhuangtai) value(?,?,?,?,?,?)";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setString(1,f.getHuxing());
ps.setString(2,f.getDizhi());
ps.setString(3,f.getNianfen());
ps.setString(4,f.getMianji());
ps.setString(5,f.getBaojia());
ps.setString(6,f.getZhuangtai());
int count=ps.executeUpdate();
if(count>0)
return true;
}catch(Exception k) {
k.printStackTrace();
}
return false;
}
public ArrayList<Fangchan> test(){
ArrayList<Fangchan> fang=new ArrayList<Fangchan>();
Fangchan f=new Fangchan(1,"河北","100");
fang.add(f);
f=new Fangchan(2,"河北","100");
fang.add(f);
return fang;
}
public List<Fangchan> shouquan(){
ArrayList<Fangchan> home=new ArrayList<Fangchan>();
try {
Connection conn=DButil.getconn();
String sql="select * from fangchan where zhuangtai=?";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setString(1,"在售");
ResultSet rs=ps.executeQuery();
while(rs.next()) {
int id=rs.getInt("id");
String dizhi=rs.getString("dizhi");
String baojia=rs.getString("baojia");
Fangchan f=new Fangchan(id,dizhi,baojia);
home.add(f);
}
}catch(Exception k) {
k.printStackTrace();
}
return home;
}
public List<Fangchan> jiaoyi(int id){
ArrayList<Fangchan> home=new ArrayList<Fangchan>();
try {
Connection conn=DButil.getconn();
String sql="select * from fangchan where zhuangtai='意向' and agent=?";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setInt(1, id);
ResultSet rs=ps.executeQuery();
while(rs.next()) {
int id0=rs.getInt("id");
String dizhi=rs.getString("dizhi");
String baojia=rs.getString("baojia");
int guke=rs.getInt("guke");
Fangchan f=new Fangchan(id0,dizhi,baojia,guke);
home.add(f);
}
}catch(Exception k) {
k.printStackTrace();
}
return home;
}
public boolean jingji(String s) {
try {
Connection conn=DButil.getconn();
String sql="select * from agent where name=?";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setString(1, s);
ResultSet rs=ps.executeQuery();
while(rs.next()) {
return true;
}
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
public int rjingji(String s) {
try {
Connection conn=DButil.getconn();
String sql="select * from agent where name=?";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setString(1, s);
ResultSet rs=ps.executeQuery();
while(rs.next()) {
return rs.getInt(1);
}
}catch(Exception e) {
e.printStackTrace();
}
return 0;
}
public boolean jingji1(int s,int k) {
try {
Connection conn=DButil.getconn();
String sql="update fangchan set zhuangtai='待售',agent="+s+" where id="+k;
Statement st=conn.createStatement();
int count=st.executeUpdate(sql);
if(count>0)return true;
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
public List<Fangchan> chaxun(String s){
ArrayList<Fangchan> home=new ArrayList<>();
try {
Connection conn=DButil.getconn();
String sql="select * from fangchan where huxing like ? or dizhi like ? or nianfen like ? or mianji like ? or baojia like ?";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setString(1, "%"+s+"%");
ps.setString(2, "%"+s+"%");
ps.setString(3, "%"+s+"%");
ps.setString(4, "%"+s+"%");
ps.setString(5, "%"+s+"%");
ResultSet rs=ps.executeQuery();
while(rs.next()) {
int id=rs.getInt(1);
String dizhi=rs.getString(3);
String baojia=rs.getString(6);
Fangchan f=new Fangchan(id,dizhi,baojia);
home.add(f);
}
}catch(Exception e) {
e.printStackTrace();
}
return home;
}
public List<Fangchan> chaxun(String s,String k){
ArrayList<Fangchan> home=new ArrayList<>();
try {
Connection conn=DButil.getconn();
String sql="select * from fangchan where ( huxing like ? or dizhi like ? or nianfen like ? or mianji like ? or baojia like ? ) and zhuangtai='在售'";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setString(1, "%"+s+"%");
ps.setString(2, "%"+s+"%");
ps.setString(3, "%"+s+"%");
ps.setString(4, "%"+s+"%");
ps.setString(5, "%"+s+"%");
ResultSet rs=ps.executeQuery();
while(rs.next()) {
int id=rs.getInt(1);
String dizhi=rs.getString(3);
String baojia=rs.getString(6);
Fangchan f=new Fangchan(id,dizhi,baojia);
home.add(f);
}
}catch(Exception e) {
e.printStackTrace();
}
return home;
}
public List<Fangchan> chaxun(String s,int k){
ArrayList<Fangchan> home=new ArrayList<>();
try {
Connection conn=DButil.getconn();
String sql="select * from fangchan where ( huxing like ? or dizhi like ? or nianfen like ? or mianji like ? or baojia like ? ) and agent="+k;
PreparedStatement ps=conn.prepareStatement(sql);
ps.setString(1, "%"+s+"%");
ps.setString(2, "%"+s+"%");
ps.setString(3, "%"+s+"%");
ps.setString(4, "%"+s+"%");
ps.setString(5, "%"+s+"%");
ResultSet rs=ps.executeQuery();
while(rs.next()) {
int id=rs.getInt(1);
String dizhi=rs.getString(3);
String baojia=rs.getString(6);
Fangchan f=new Fangchan(id,dizhi,baojia);
home.add(f);
}
}catch(Exception e) {
e.printStackTrace();
}
return home;
}
public boolean mai(int id,int guke) {
try {
Connection conn=DButil.getconn();
String sql="update fangchan set zhuangtai='意向', guke=? where id=? ";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setInt(1, guke);
ps.setInt(2, id);
int count =ps.executeUpdate();
if(count>0)
return true;
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
public List<Fangchan> liu(){
ArrayList<Fangchan> home=new ArrayList<>();
try {
Connection conn=DButil.getconn();
String sql="select * from fangchan where zhuangtai='在售' ;" ;
PreparedStatement ps=conn.prepareStatement(sql);
ResultSet rs=ps.executeQuery();
while(rs.next()) {
int id=rs.getInt(1);
String dizhi=rs.getString(3);
String baojia=rs.getString(6);
Fangchan f=new Fangchan(id,dizhi,baojia);
home.add(f);
}
}catch(Exception e) {
e.printStackTrace();
}
return home;
}
public List<guke>shenhe(){
ArrayList<guke> people=new ArrayList<>();
try {
Connection conn=DButil.getconn();
String sql="select * from guke where flag=0";
Statement st=conn.createStatement();
ResultSet rs=st.executeQuery(sql);
while(rs.next()) {
int id=rs.getInt(1);
String name=rs.getString(2);
String sex=rs.getString(3);
guke ren=new guke(id,name,sex);
people.add(ren);
}
}catch(Exception e) {
e.printStackTrace();
}
return people;
}
public boolean tingshou(int id) {
try {
Connection conn=DButil.getconn();
String sql="update fangchan set zhuangtai='停售' where id="+id;
Statement st=conn.createStatement();
int count=st.executeUpdate(sql);
if(count>0)return true;
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
public boolean addren(int id,String name,String address,String phone) {
try {
Connection conn=DButil.getconn();
String sql="insert into agent(id,name,address,phone) value(?,?,?,?)";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setInt(1, id);
ps.setString(2, name);
ps.setString(3, address);
ps.setString(4, phone);
int count=ps.executeUpdate();
if(count>0)
return true;
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
public boolean shenhe2(int id) {
try {
Connection conn=DButil.getconn();
String sql="update guke set flag=1 where id="+id;
Statement st=conn.createStatement();
int count=st.executeUpdate(sql);
if(count>0)return true;
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
public boolean judge1(int id,String password) {
try {
Connection conn=DButil.getconn();
String sql="select * from guke where id=? and mima=? and flag=1";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setInt(1, id);
ps.setString(2, password);
ResultSet rs=ps.executeQuery();
while(rs.next()) {
return true;
}
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
public boolean judge2(int id,String password) {
try {
Connection conn=DButil.getconn();
String sql="select * from agent where id=? and password=? ;";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setInt(1, id);
ps.setString(2, password);
ResultSet rs=ps.executeQuery();
while(rs.next()) {
return true;
}
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
public Fangchan searchByid(int id) {
Fangchan f=null;
try {
Connection conn=DButil.getconn();
String sql="select * from fangchan where id=?";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setInt(1, id);
ResultSet rs=ps.executeQuery();
while(rs.next()) {
int id0=rs.getInt("id");
String huxing=rs.getString("huxing");
String dizhi=rs.getString("dizhi");
String nianfen=rs.getString("nianfen");
String mianji=rs.getString("mianji");
String baojia=rs.getString("baojia");
String zhuangtai=rs.getString("zhuangtai");
f=new Fangchan(id0,huxing,dizhi,nianfen,mianji,baojia,zhuangtai);
return f;
}
}catch(Exception e) {
e.printStackTrace();
}
return f;
}
public List<Fangchan> liulan(int id) {
ArrayList<Fangchan> home=new ArrayList<Fangchan>();
Fangchan f=null;
try {
Connection conn=DButil.getconn();
String sql="select * from fangchan where agent=?";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setInt(1, id);
ResultSet rs=ps.executeQuery();
while(rs.next()) {
int id0=rs.getInt("id");
String huxing=rs.getString("huxing");
String dizhi=rs.getString("dizhi");
String nianfen=rs.getString("nianfen");
String mianji=rs.getString("mianji");
String baojia=rs.getString("baojia");
String zhuangtai=rs.getString("zhuangtai");
f=new Fangchan(id0,huxing,dizhi,nianfen,mianji,baojia,zhuangtai);
home.add(f);
}
}catch(Exception e) {
e.printStackTrace();
}
return home;
}
public List<Fangchan> cha3(int id,String s) {
ArrayList<Fangchan> home=new ArrayList<>();
try {
Connection conn=DButil.getconn();
String sql="select * from fangchan where ( huxing like ? or dizhi like ? or nianfen like ? or mianji like ? or baojia like ? ) and ( agent=? )";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setString(1, "%"+s+"%");
ps.setString(2, "%"+s+"%");
ps.setString(3, "%"+s+"%");
ps.setString(4, "%"+s+"%");
ps.setString(5, "%"+s+"%");
ps.setInt(6, id);
ResultSet rs=ps.executeQuery();
while(rs.next()) {
int id0=rs.getInt(1);
String dizhi=rs.getString(3);
String baojia=rs.getString(6);
Fangchan f=new Fangchan(id,dizhi,baojia);
home.add(f);
}
}catch(Exception e) {
e.printStackTrace();
}
return home;
}
public guke searchByid1(int id) {
guke ren=null;
try {
Connection conn=DButil.getconn();
String sql="select * from guke where id=?";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setInt(1, id);
ResultSet rs=ps.executeQuery();
while(rs.next()) {
int id0=rs.getInt("id");
String name=rs.getString("name");
String sex=rs.getString("sex");
String number=rs.getString("number");
String phone=rs.getString("phone");
String address=rs.getString("address");
String mima=rs.getString("mima");
ren=new guke(id,name,sex,number,phone,address,mima);
return ren;
}
}catch(Exception e) {
e.printStackTrace();
}
return ren;
}
public agent searchByid3(int id) {
agent a=null;
try {
Connection conn=DButil.getconn();
String sql="select * from agent where id=?";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setInt(1, id);
ResultSet rs=ps.executeQuery();
while(rs.next()) {
int id0=rs.getInt("id");
String name=rs.getString("name");
String address=rs.getString("address");
String phone=rs.getString("phone");
a=new agent(id0,name,address,phone);
return a;
}
}catch(Exception e) {
e.printStackTrace();
}
return a;
}
public boolean change(int id) {
try {
Connection conn=DButil.getconn();
String sql="update agent set password='123456' where id=?";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setInt(1, id);
int count=ps.executeUpdate();
if(count>0)return true;
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
public boolean jiaoyi2(int id) {
try {
Connection conn=DButil.getconn();
String sql="update fangchan set zhuangtai='售出' where id=?";
PreparedStatement ps=conn.prepareStatement(sql);
ps.setInt(1, id);
int count=ps.executeUpdate();
if(count>0)return true;
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
public boolean xiugai(int id,String mima) {
try {
Connection conn=DButil.getconn();
String sql="update guke set mima=? where id=?";
PreparedStatement
ps=conn.prepareStatement(sql);
ps.setString(1, mima);
ps.setInt(2, id);
int count=ps.executeUpdate();
if(count>0)return true;
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
public boolean xiugai2(int id,String mima) {
try {
Connection conn=DButil.getconn();
String sql="update agent set password=? where id=?";
PreparedStatement
ps=conn.prepareStatement(sql);
ps.setString(1, mima);
ps.setInt(2, id);
int count=ps.executeUpdate();
if(count>0)return true;
}catch(Exception e) {
e.printStackTrace();
}
return false;
}
}
service层houtai的Servlet
package service;
import java.io.IOException;
import java.sql.SQLException;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import bean.Fangchan;
import bean.agent;
import bean.guke;
import dao.Dao;
/**
* Servlet implementation class houtai
*/
public class houtai extends HttpServlet {
Dao dao=new Dao();
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public houtai() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#service(HttpServletRequest request, HttpServletResponse response)
*/
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
request.setCharacterEncoding("utf-8");
String message=request.getParameter("method");
if("login".equals(message)) {
login(request,response);
}
else if("zhuce".equals(message)) {
zhuce(request,response);
}
else if("jiaoyi".equals(message)) {
jiaoyi(request,response);
}
else if("xiugai2".equals(message)) {
xiugai2(request,response);
}
else if("liulan1".equals(message)) {
liulan1(request,response);
}
else if("cha3".equals(message)) {
cha3(request,response);
}
else if("cha5".equals(message)) {
cha5(request,response);
}
else if("self1".equals(message)) {
self1(request,response);
}
else if("addfang".equals(message)) {
addfang(request,response);
}
else if("shouquan".equals(message)) {
shouquan(request,response);
}else if("shouquan1".equals(message)) {
shouquan1(request,response);
}
else if("chakan".equals(message)) {
chakan(request,response);
}
else if("test".equals(message)) {
test(request,response);
}
else if("tingshou".equals(message)) {
tingshou(request,response);
}
else if("tingshou1".equals(message)) {
tingshou1(request,response);
}
else if("tingshou2".equals(message)) {
tingshou2(request,response);
}
else if("chaxun".equals(message)) {
chaxun(request,response);
}
else if("gchaxun".equals(message)) {
gchaxun(request,response);
}
else if("jchaxun".equals(message)) {
jchaxun(request,response);
}
else if("chaxun1".equals(message)) {
chaxun1(request,response);
}
else if("shenhe".equals(message)) {
shenhe(request,response);
}
else if("shenhe1".equals(message)) {
shenhe1(request,response);
}
else if("shenhe2".equals(message)) {
shenhe2(request,response);
}
else if("addren".equals(message)) {
addren(request,response);
}
else if("chongzhi".equals(message)) {
chongzhi(request,response);
}
else if("self".equals(message)) {
self(request,response);
}
else if("xiugai1".equals(message)) {
xiugai1(request,response);
}
else if("liu".equals(message)) {
liu(request,response);
}
else if("mai".equals(message)) {
mai(request,response);
}
else if("mai1".equals(message)) {
mai1(request,response);
}
else if("mai2".equals(message)) {
mai2(request,response);
}
else if("jiaoyi1".equals(message)) {
jiaoyi1(request,response);
}
else if("jiaoyi2".equals(message)) {
jiaoyi2(request,response);
}
}
private void login(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String name=request.getParameter("name");
String password=request.getParameter("password");
String shenfen=request.getParameter("shenfen");
if(name.equals("root")&&password.equals("123456")&&shenfen.equals("管理员")) {
response.setHeader("refresh", "0;URL=1.jsp");
}
else if(shenfen.equals("顾客")) {
int id=Integer.parseInt(name);
if(dao.judge1(id, password)) {
request.getSession().setAttribute("id", id);
response.setHeader("refresh", "0;URL=2.jsp");
}
}
else if(shenfen.equals("经纪")) {
int id=Integer.parseInt(name);
if(dao.judge2(id, password)) {
request.getSession().setAttribute("id", id);
response.setHeader("refresh", "0;URL=3.jsp");
}
}
else {
request.setAttribute("message", "登录失败!");
response.setHeader("refresh", "0;URL=login.jsp");
}
}
private void zhuce(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String name=request.getParameter("name");
String mima=request.getParameter("mima");
String number=request.getParameter("number");
String sex=request.getParameter("sex");
String phone=request.getParameter("phone");
String address=request.getParameter("address");
guke g=new guke(name,sex,number,phone,address,mima);
try {
if(dao.addguke(g)) {
request.setAttribute("message", "注册成功,请等待审核");
}
else request.setAttribute("message", "注册失败");
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
request.getRequestDispatcher("zhuce.jsp").forward(request, response);
}
private void addfang(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String huxing=request.getParameter("huxing");
String dizhi=request.getParameter("dizhi");
String nianfen=request.getParameter("nianfen");
String mianji=request.getParameter("mianji");
String baojia=request.getParameter("baojia");
String zhuangtai=request.getParameter("zhuangtai");
Fangchan f=new Fangchan(huxing,dizhi,nianfen,mianji,baojia,zhuangtai);
try {
if(dao.addfang(f)) {
request.setAttribute("message", "添加成功");
}
else request.setAttribute("message", "添加失败");
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
request.getRequestDispatcher("addfang.jsp").forward(request, response);
}
private void chongzhi(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
int id=Integer.parseInt(request.getParameter("gonghao"));
try {
agent a=dao.searchByid3(id);
if(a!=null&&dao.change(id)) {
request.setAttribute("message", a);
}
else request.setAttribute("message", "重置失败");
} catch (Exception e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
request.getRequestDispatcher("mimachongzhi.jsp").forward(request, response);
}
private void addren(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
int id=Integer.parseInt(request.getParameter("gonghao"));
String name=request.getParameter("name");
String address=request.getParameter("address");
String phone =request.getParameter("phone");
try {
if(dao.addren(id,name,address,phone)) {
request.setAttribute("message", "添加成功");
}
else request.setAttribute("message", "添加失败");
} catch (Exception e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
request.getRequestDispatcher("addren.jsp").forward(request, response);
}
private void self(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
int id=(int) request.getSession().getAttribute("id");
guke a=dao.searchByid1(id);
request.setAttribute("a", a);
request.getRequestDispatcher("new.jsp").forward(request, response);
}
private void self1(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
int id=(int) request.getSession().getAttribute("id");
agent a=dao.searchByid3(id);
request.setAttribute("message", a);
request.getRequestDispatcher("3.jsp").forward(request, response);
}
private void liu(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
List<Fangchan> home=dao.liu();
request.setAttribute("message", home);
request.getRequestDispatcher("2.jsp").forward(request, response);
}
private void liulan1(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
int id=(int)request.getSession().getAttribute("id");
List<Fangchan> home=dao.liulan(id);
request.setAttribute("home", home);
request.getSession().setAttribute("id", id);
request.getRequestDispatcher("liulan1.jsp").forward(request, response);
}
private void mai(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
List<Fangchan> home=dao.liu();
int id=(int)request.getSession().getAttribute("id");
request.getSession().setAttribute("id", id);
request.setAttribute("home", home);
request.getRequestDispatcher("mai.jsp").forward(request, response);
}
private void shouquan(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
List<Fangchan> home=dao.shouquan();
request.setAttribute("home",home);
request.getRequestDispatcher("shouquan.jsp").forward(request, response);
}
private void chaxun(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
List<Fangchan> home=null;
String data=request.getParameter("data");
home=dao.chaxun(data);
request.setAttribute("home",home);
request.getRequestDispatcher("chaxun1.jsp").forward(request, response);
}
private void cha3(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
List<Fangchan> home=null;
int id=(int)request.getSession().getAttribute("id");
String data=request.getParameter("data");
home=dao.cha3(id, data);
request.setAttribute("home",home);
request.getRequestDispatcher("cha4.jsp").forward(request, response);
}
private void jiaoyi(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
List<Fangchan> home=null;
int id=(int) request.getSession().getAttribute("id");
home=dao.jiaoyi(id);
request.getSession().setAttribute("id", id);
request.setAttribute("home",home);
request.getRequestDispatcher("jiaoyi.jsp").forward(request, response);
}
private void gchaxun(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
List<Fangchan> home=null;
String data=request.getParameter("data");
home=dao.chaxun(data," ");
request.setAttribute("home",home);
request.getRequestDispatcher("chaxun1.jsp").forward(request, response);
}
private void jchaxun(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
List<Fangchan> home=null;
String data=request.getParameter("data");
int id=(int) request.getSession().getAttribute("id");
home=dao.chaxun(data,id);
request.setAttribute("home",home);
request.getRequestDispatcher("chaxun1.jsp").forward(request, response);
}
private void chaxun1(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
int id = Integer.parseInt(request.getParameter("id"));
Fangchan f=dao.searchByid(id);
request.getSession().setAttribute("id", id);
request.setAttribute("f", f);
request.getRequestDispatcher("chaxun2.jsp").forward(request, response);
}
private void shenhe1(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
int id = Integer.parseInt(request.getParameter("id"));
guke f=dao.searchByid1(id);
request.getSession().setAttribute("id", id);
request.setAttribute("f", f);
request.getRequestDispatcher("shenhe1.jsp").forward(request, response);
}
private void jiaoyi1(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
int id=(int) request.getSession().getAttribute("id");
int id0 = Integer.parseInt(request.getParameter("id0"));
Fangchan f=dao.searchByid(id0);
request.getSession().setAttribute("id", id);
request.getSession().setAttribute("id0", id0);
request.setAttribute("f", f);
request.getRequestDispatcher("jiao1.jsp").forward(request, response);
}
private void shenhe2(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
int id =(int) request.getSession().getAttribute("id");
if(dao.shenhe2(id)) {
request.setAttribute("message", "审核成功");
}
else {
request.setAttribute("message", "审核失败");
}
request.getRequestDispatcher("shenhe.jsp").forward(request, response);
}
private void tingshou(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
List<Fangchan> home=dao.shouquan();
request.setAttribute("home",home);
request.getRequestDispatcher("tingshou.jsp").forward(request, response);
}
private void shenhe(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
List<guke> home=dao.shenhe();
request.setAttribute("home",home);
request.getRequestDispatcher("shenhe.jsp").forward(request, response);
}
private void shouquan1(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String name=request.getParameter("name");
int id =(int) request.getSession().getAttribute("id");
if(dao.jingji(name)&&dao.jingji1(dao.rjingji(name),id)) {
request.setAttribute("message", "授权成功");
request.getRequestDispatcher("shouquan.jsp").forward(request, response);
}
else
{
request.setAttribute("message", "授权失败");
request.getRequestDispatcher("shouquan.jsp").forward(request, response);
}
}
private void cha5(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
int id0 =Integer.parseInt(request.getParameter("id0"));
int id=(int) request.getSession().getAttribute("id");
Fangchan f=dao.searchByid(id0);
request.getSession().setAttribute("id", id);
request.setAttribute("f", f);
request.getRequestDispatcher("chaxun2.jsp").forward(request, response);
}
private void test(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
List<Fangchan> home=dao.test();
request.setAttribute("home",home);
request.getRequestDispatcher("test1.jsp").forward(request, response);
}
private void chakan(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
int id = Integer.parseInt(request.getParameter("id"));
Fangchan f=dao.searchByid(id);
request.getSession().setAttribute("id", id);
request.setAttribute("f", f);
request.getRequestDispatcher("chakan.jsp").forward(request, response);
}
private void tingshou1(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
int id = Integer.parseInt(request.getParameter("id"));
Fangchan f=dao.searchByid(id);
request.getSession().setAttribute("id", id);
request.setAttribute("f", f);
request.getRequestDispatcher("tingshou1.jsp").forward(request, response);
}
private void mai1(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
int id0 = Integer.parseInt(request.getParameter("id0"));
Fangchan f=dao.searchByid(id0);
int id=(int)request.getSession().getAttribute("id");
request.getSession().setAttribute("id0", id0);
request.getSession().setAttribute("id", id);
request.setAttribute("f", f);
request.getRequestDispatcher("mai1.jsp").forward(request, response);
}
private void mai2(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
int id0 =(int) request.getSession().getAttribute("id0");
int guke =(int) request.getSession().getAttribute("id");
if(dao.mai(id0, guke)) {
request.setAttribute("message", "更新成功");
request.getRequestDispatcher("2.jsp").forward(request, response);
}
else
{
request.setAttribute("message", "停售失败");
request.getRequestDispatcher("shouquan.jsp").forward(request, response);
}
}
private void jiaoyi2(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
int id0 =(int) request.getSession().getAttribute("id0");
int id =(int) request.getSession().getAttribute("id");
request.getSession().setAttribute("id",id);
if(dao.jiaoyi2(id0)) {
request.setAttribute("message", "售出成功");
request.getRequestDispatcher("3.jsp").forward(request, response);
}
else
{
request.setAttribute("message", "售出失败");
request.getRequestDispatcher("3.jsp").forward(request, response);
}
}
private void xiugai1(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
int id = (int) request.getSession().getAttribute("id");
String mima=request.getParameter("mima");
if(dao.xiugai(id, mima)) {
request.setAttribute("message", "修改成功");
}
request.getRequestDispatcher("2.jsp").forward(request, response);
}
private void xiugai2(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
int id = (int) request.getSession().getAttribute("id");
String mima=request.getParameter("mima");
if(dao.xiugai2(id, mima)) {
request.setAttribute("message", "修改成功");
}
request.getRequestDispatcher("3.jsp").forward(request, response);
}
private void tingshou2(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
int id =(int) request.getSession().getAttribute("id");
if(dao.tingshou(id)) {
request.setAttribute("message", "停售成功");
request.getRequestDispatcher("shouquan.jsp").forward(request, response);
}
else
{
request.setAttribute("message", "停售失败");
request.getRequestDispatcher("shouquan.jsp").forward(request, response);
}
}
}
util层DButil类
package util;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class DButil {
public static Connection getconn() {
Connection conn=null;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
conn=DriverManager.getConnection("jdbc:mysql://localhost:3306/fangchan","root","byc666");
}catch(Exception e) {
e.printStackTrace();
}
return conn;
}
public static void close(Connection conn,PreparedStatement ps,ResultSet rs) {
if(rs!=null) {
try {
rs.close();
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
}if(ps!=null) {
try {
ps.close();
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
}
if(conn!=null) {
try {
conn.close();
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
}
}
}