复杂data的处理方式
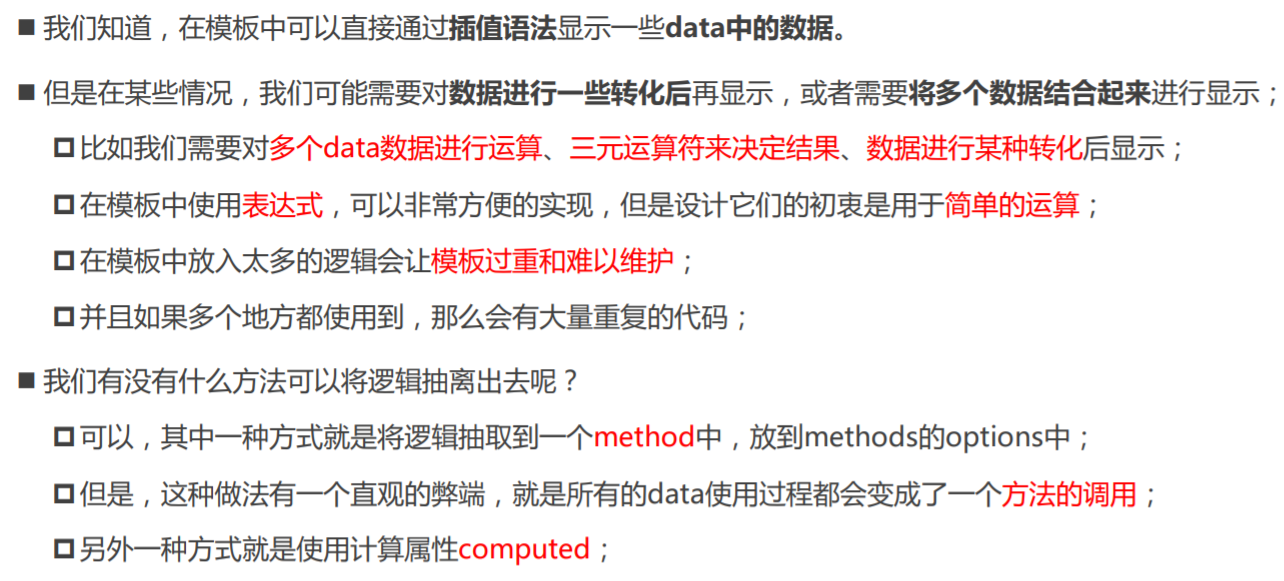
认识计算属性computed
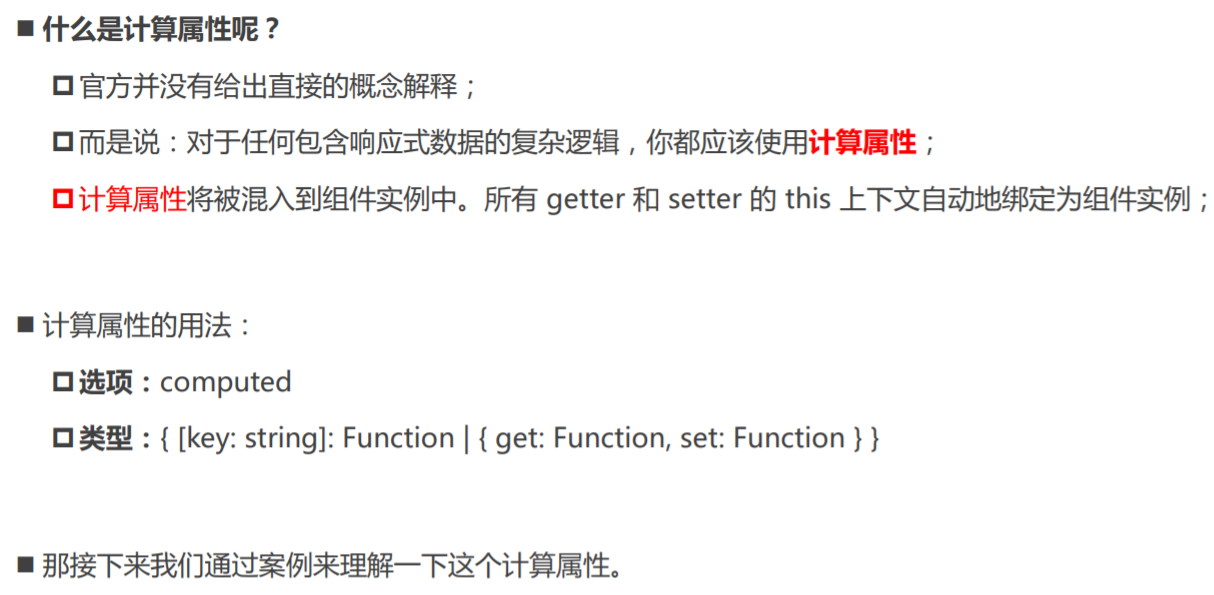
案例实现思路
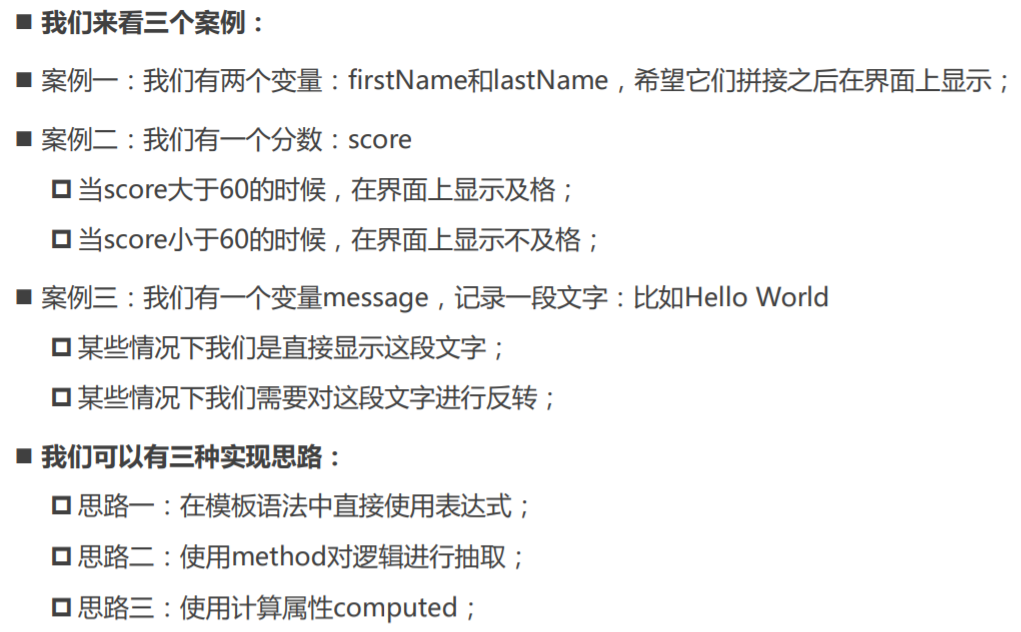
实现思路一:模板语法
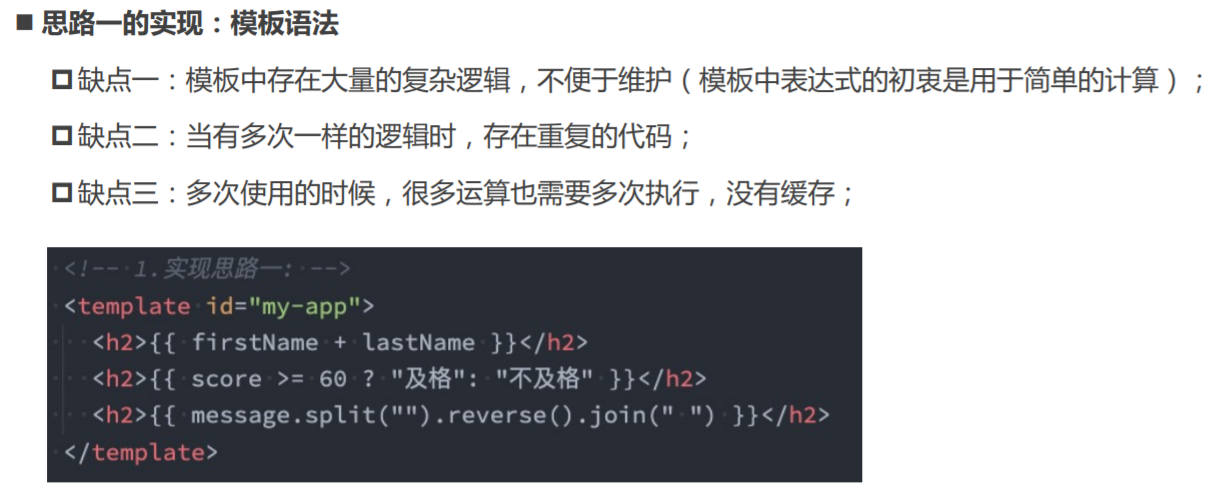
实现思路二:method实现
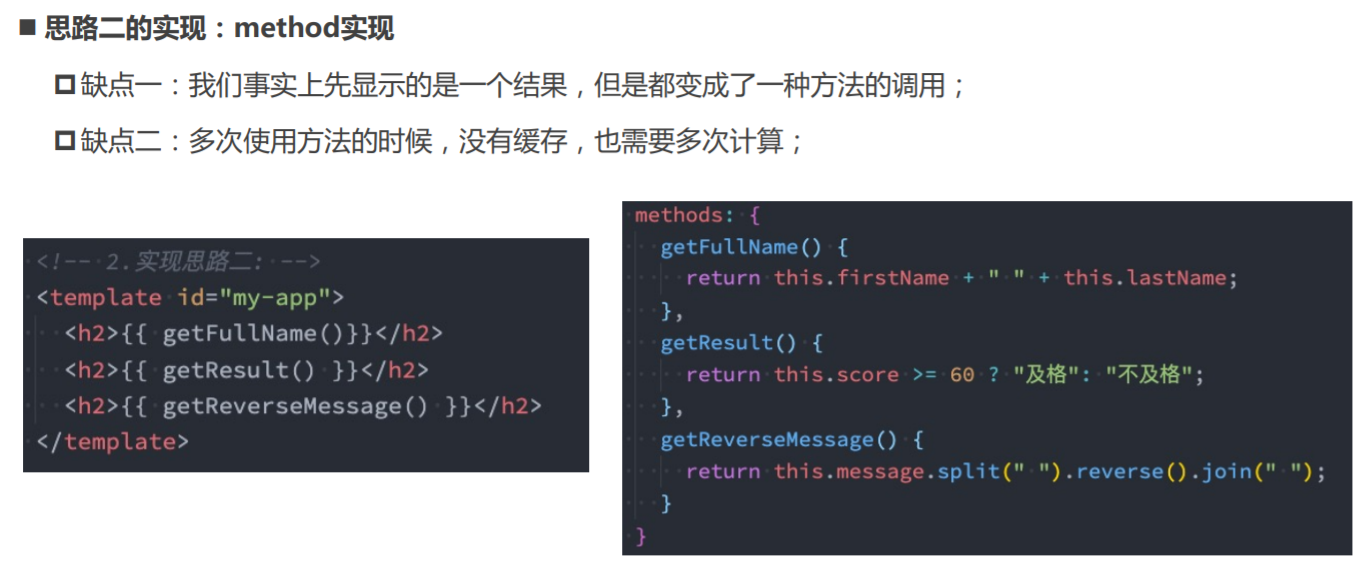
思路三的实现:computed实现
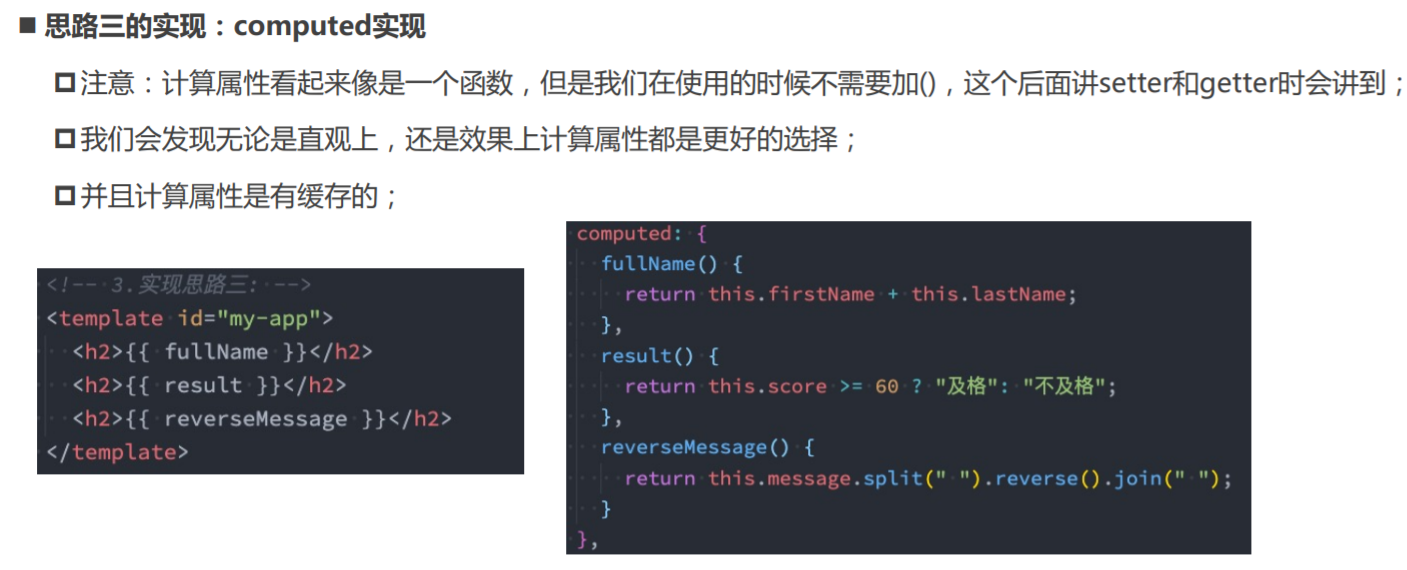
计算属性 vs methods
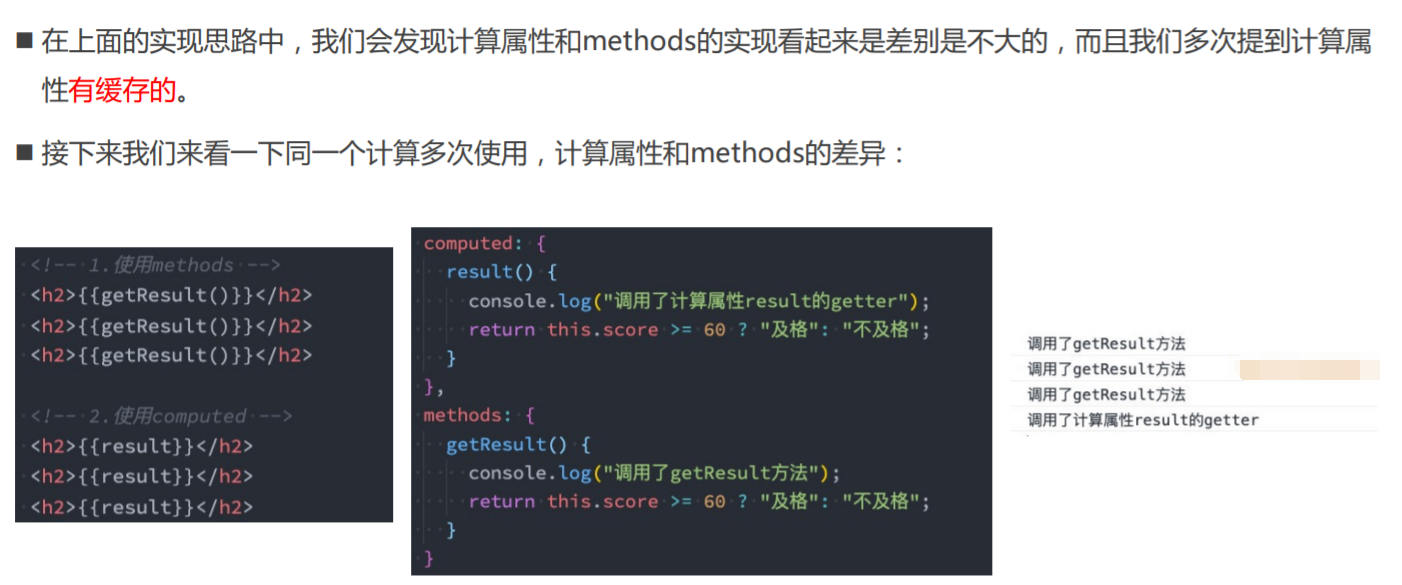
计算属性的缓存
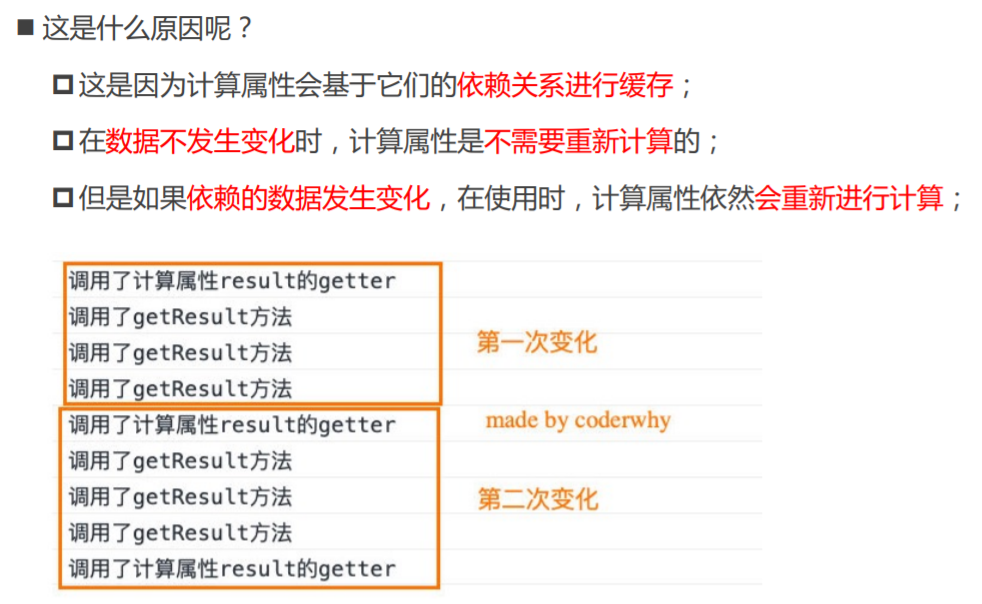
计算属性的setter和getter
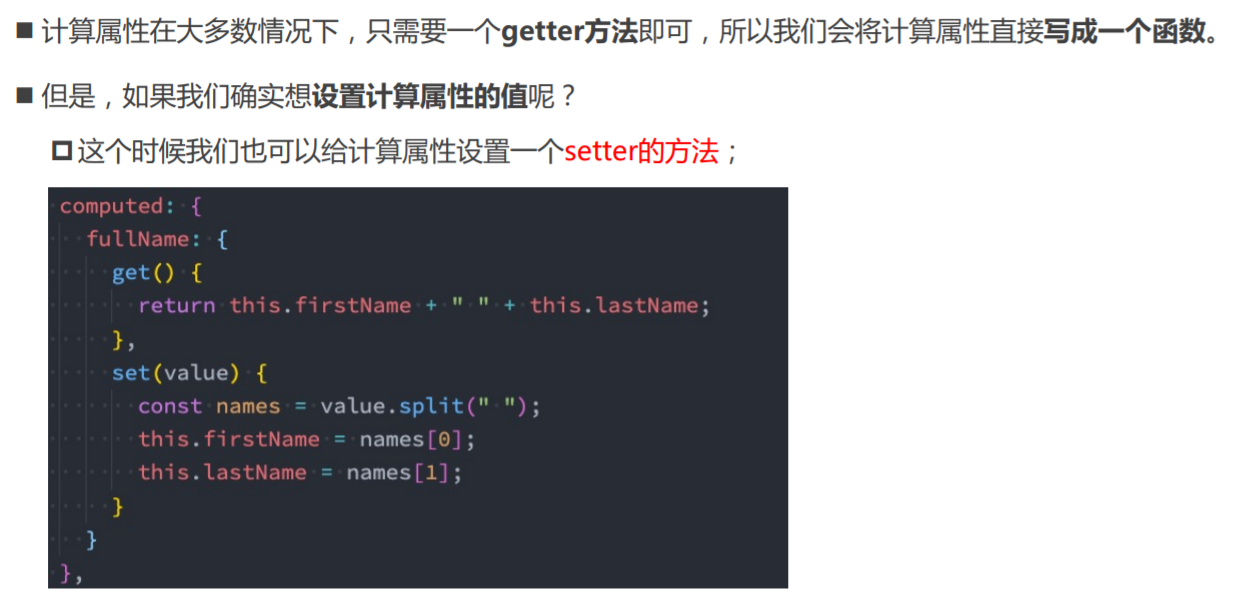
源码如何对setter和getter处理
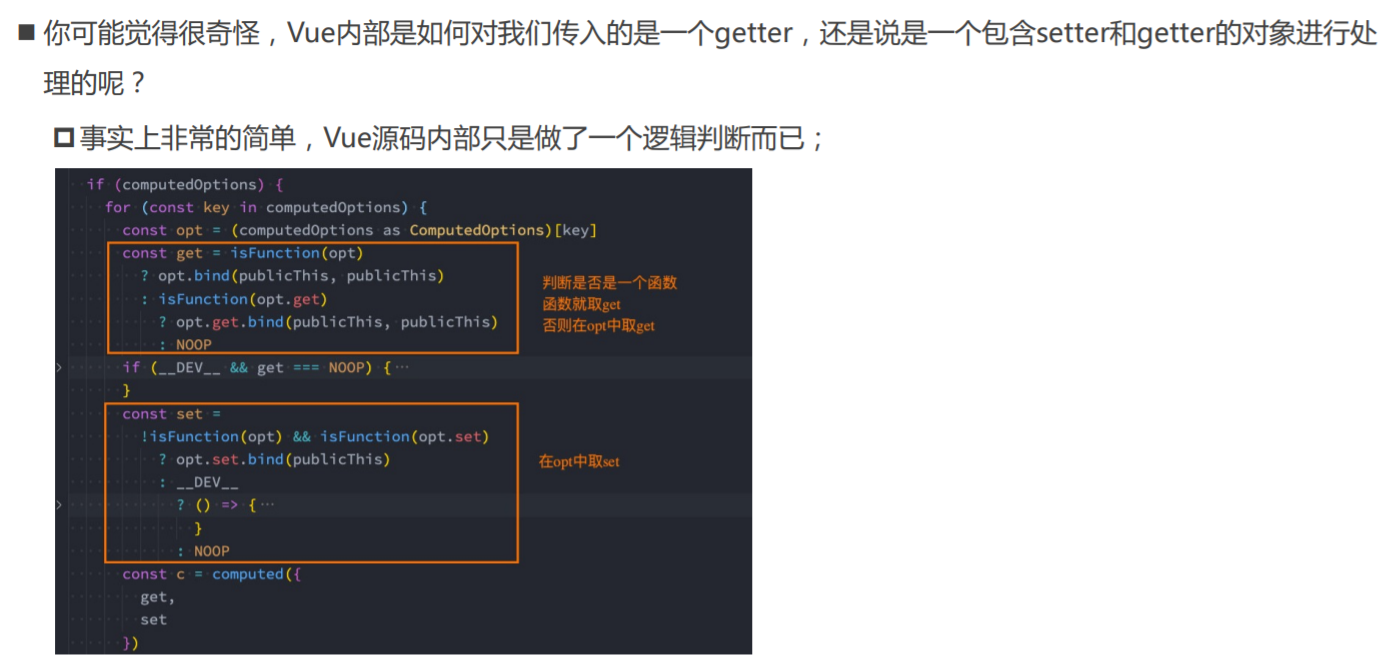
01_三个案例的实现-插值语法.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="my-app">
<h2>{{firstName + " " + lastName}}</h2>
<h2>{{score >= 60 ? '及格': '不及格'}}</h2>
<h2>{{message.split(" ").reverse().join(" ")}}</h2>
</template>
<script src="../js/vue.js"></script>
<script>
const App = {
template: '#my-app',
data() {
return {
firstName: "Kobe",
lastName: "Bryant",
score: 80,
message: "Hello World"
}
}
}
Vue.createApp(App).mount('#app');
</script>
</body>
</html>
02_三个案例的实现-methods.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="my-app">
<h2>{{getFullName()}}</h2>
<h2>{{getResult()}}</h2>
<h2>{{getReverseMessage()}}</h2>
</template>
<script src="../js/vue.js"></script>
<script>
const App = {
template: '#my-app',
data() {
return {
firstName: "Kobe",
lastName: "Bryant",
score: 80,
message: "Hello World"
}
},
methods: {
getFullName() {
return this.firstName + " " + this.lastName;
},
getResult() {
return this.score >= 60 ? "及格": "不及格";
},
getReverseMessage() {
return this.message.split(" ").reverse().join(" ");
}
}
}
Vue.createApp(App).mount('#app');
</script>
</body>
</html>
03_三个案例的实现-computed.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="my-app">
<h2>{{fullName}}</h2>
<h2>{{result}}</h2>
<h2>{{reverseMessage}}</h2>
</template>
<script src="../js/vue.js"></script>
<script>
const App = {
template: '#my-app',
data() {
return {
firstName: "Kobe",
lastName: "Bryant",
score: 80,
message: "Hello World"
}
},
computed: {
// 定义了一个计算属性叫fullname
fullName() {
return this.firstName + " " + this.lastName;
},
result() {
return this.score >= 60 ? "及格": "不及格";
},
reverseMessage() {
return this.message.split(" ").reverse().join(" ");
}
}
}
Vue.createApp(App).mount('#app');
</script>
</body>
</html>
04_methods-computed区别.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="my-app">
<button @click="changeFirstName">修改firstName</button>
<h2>{{fullName}}</h2>
<h2>{{fullName}}</h2>
<h2>{{fullName}}</h2>
<h2>{{fullName}}</h2>
<h2>{{fullName}}</h2>
<h2>{{fullName}}</h2>
<h2>{{fullName}}</h2>
<h2>{{fullName}}</h2>
<h2>{{getFullName()}}</h2>
<h2>{{getFullName()}}</h2>
<h2>{{getFullName()}}</h2>
<h2>{{getFullName()}}</h2>
<h2>{{getFullName()}}</h2>
<h2>{{getFullName()}}</h2>
</template>
<script src="../js/vue.js"></script>
<script>
const App = {
template: '#my-app',
data() {
return {
firstName: "Kobe",
lastName: "Bryant"
}
},
computed: {
// 计算属性是有缓存的, 当我们多次使用计算属性时, 计算属性中的运算只会执行一次.
// 计算属性会随着依赖的数据(firstName)的改变, 而进行重新计算.
fullName() {
console.log("computed的fullName中的计算");
return this.firstName + " " + this.lastName;
}
},
methods: {
getFullName() {
console.log("methods的getFullName中的计算");
return this.firstName + " " + this.lastName;
},
changeFirstName() {
this.firstName = "Coder"
}
}
}
Vue.createApp(App).mount('#app');
</script>
</body>
</html>
05_computed的setter和getter.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="my-app">
<button @click="changeFullName">修改fullName</button>
<h2>{{fullName}}</h2>
</template>
<script src="../js/vue.js"></script>
<script>
const App = {
template: '#my-app',
data() {
return {
firstName: "Kobe",
lastName: "Bryant"
}
},
computed: {
// fullName 的 getter方法,语法糖
// fullName() {
// return this.firstName + " " + this.lastName;
// },
// fullName的getter和setter方法
fullName: {
get: function() {
return this.firstName + " " + this.lastName;
},
set: function(newValue) {
console.log(newValue);
const names = newValue.split(" ");
[this.firstName, this.lastName] = names
// this.firstName = names[0];
// this.lastName = names[1];
}
}
},
methods: {
changeFullName() {
this.fullName = "Coder Why";
}
}
}
Vue.createApp(App).mount('#app');
</script>
</body>
</html>