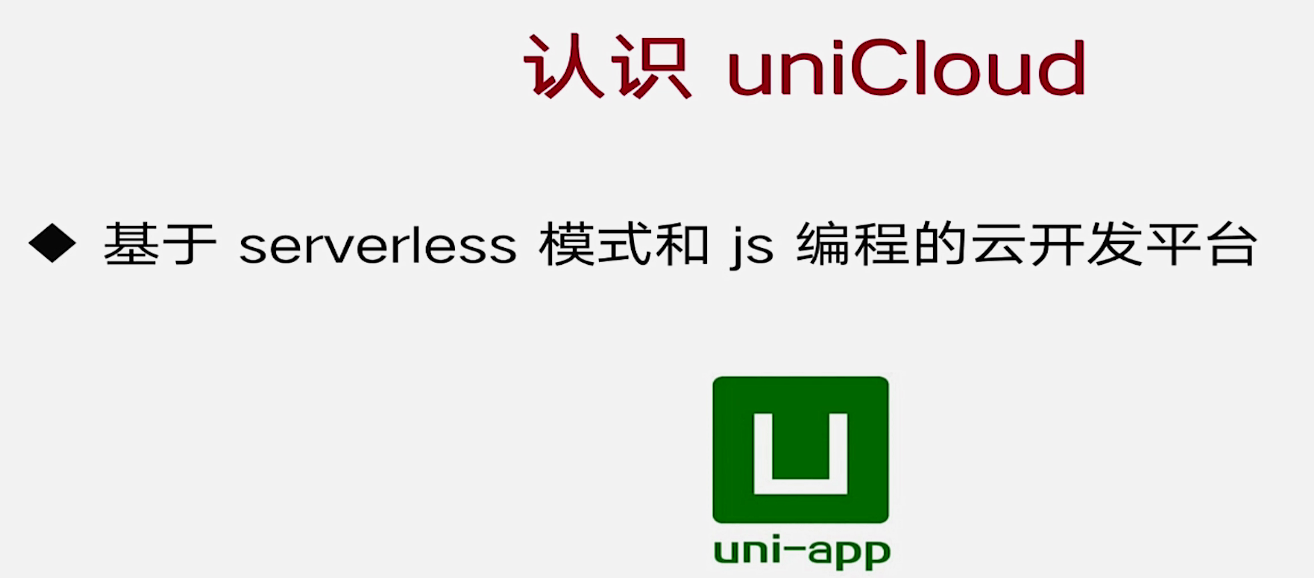
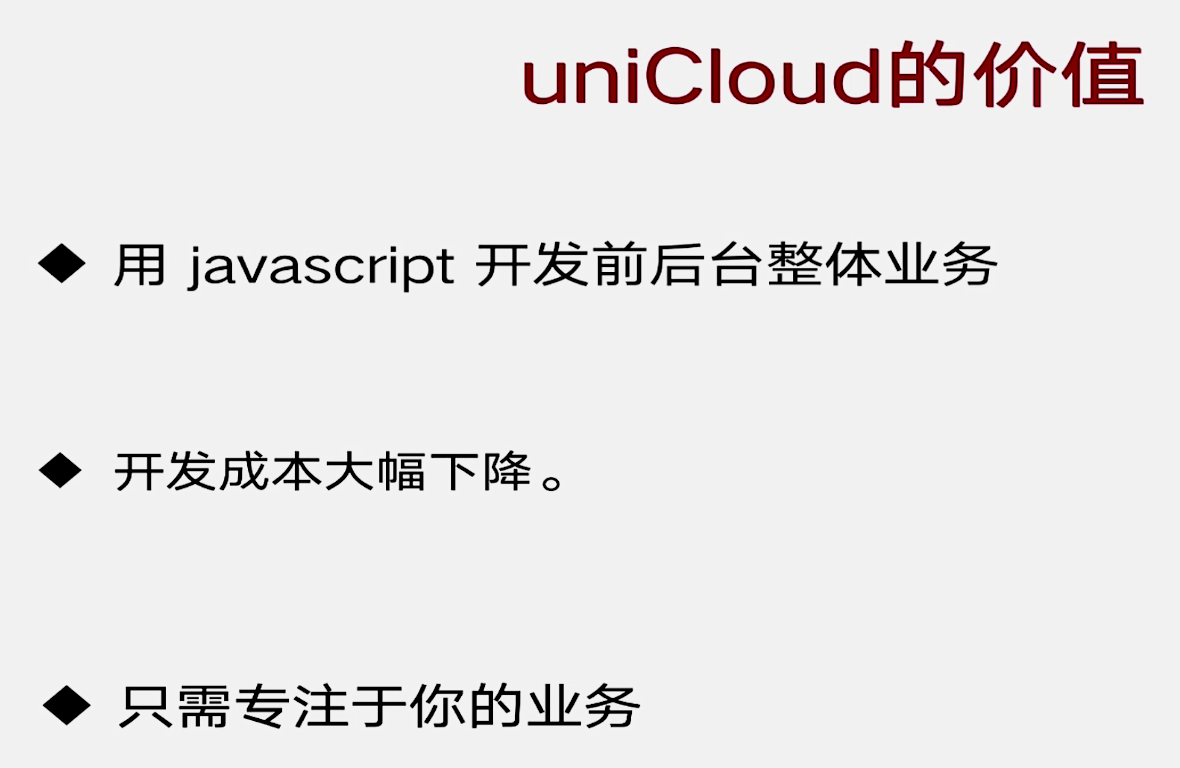
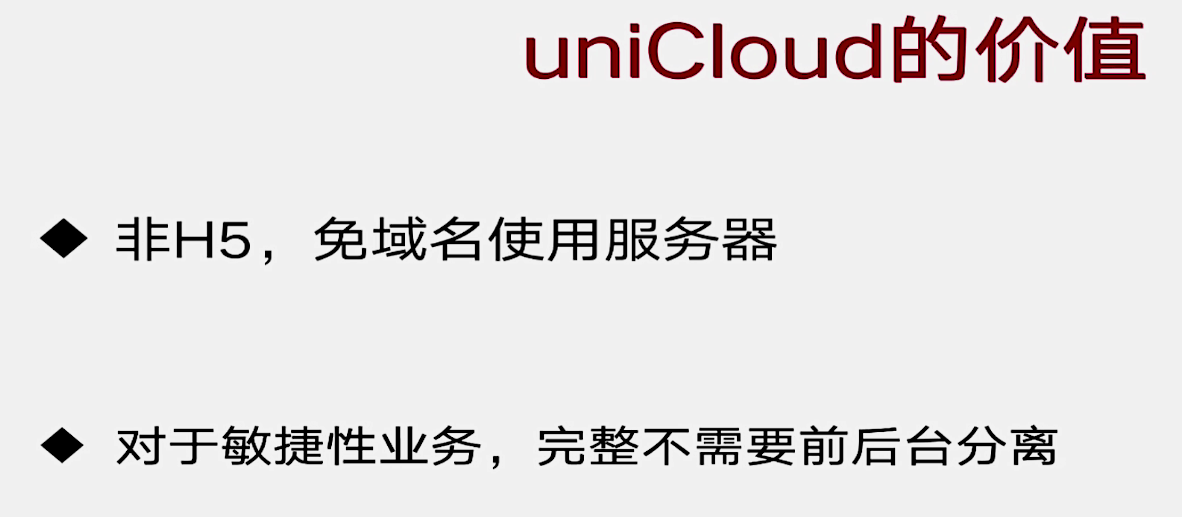
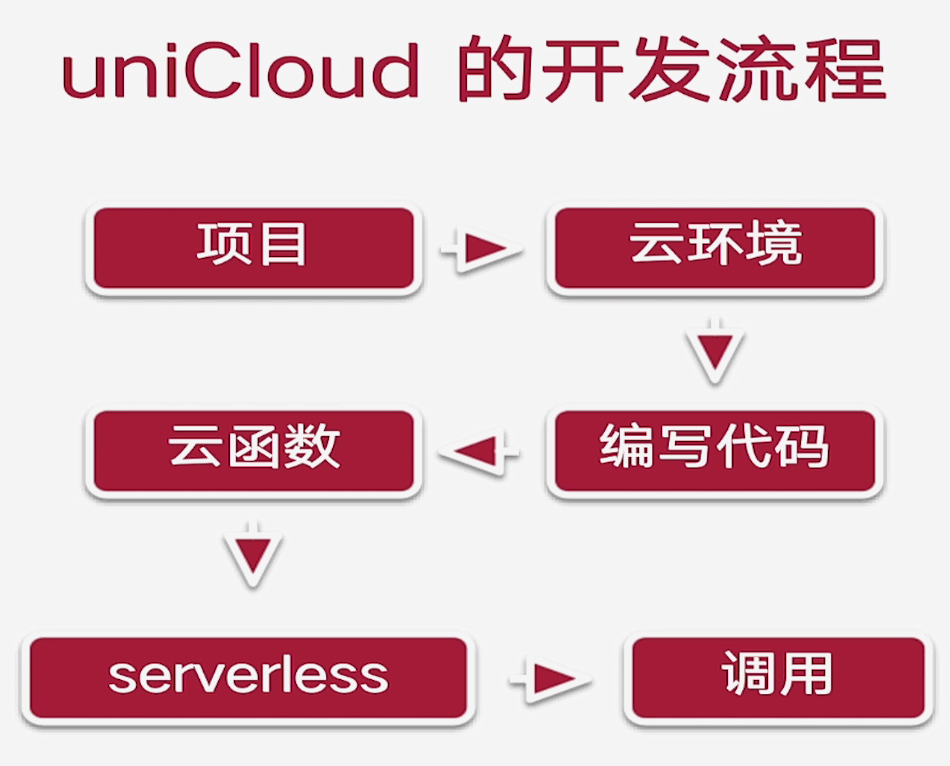
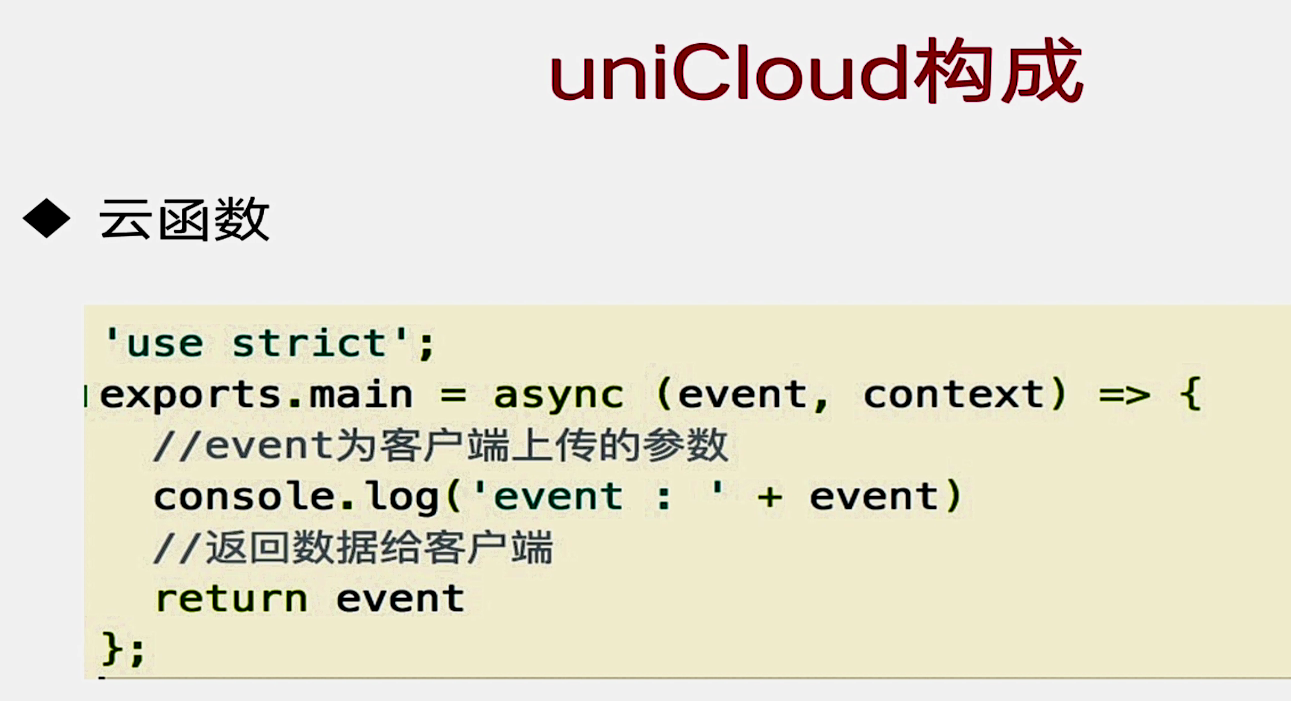
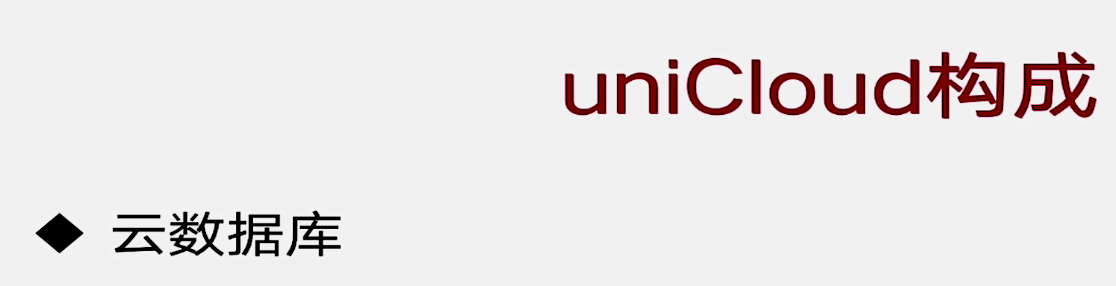
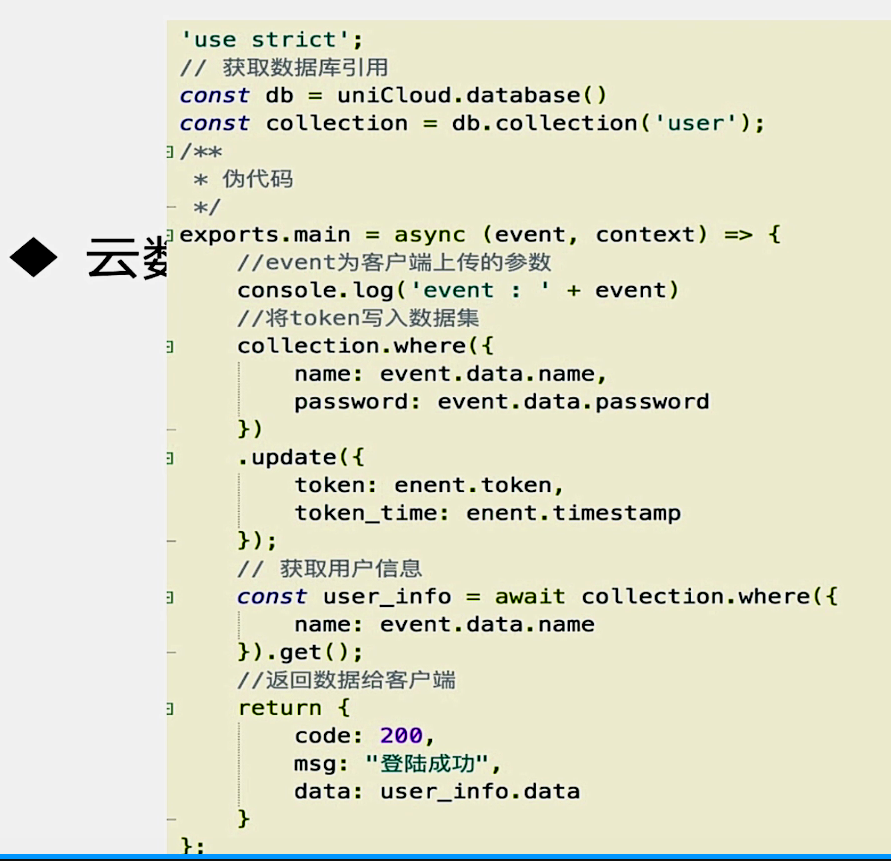
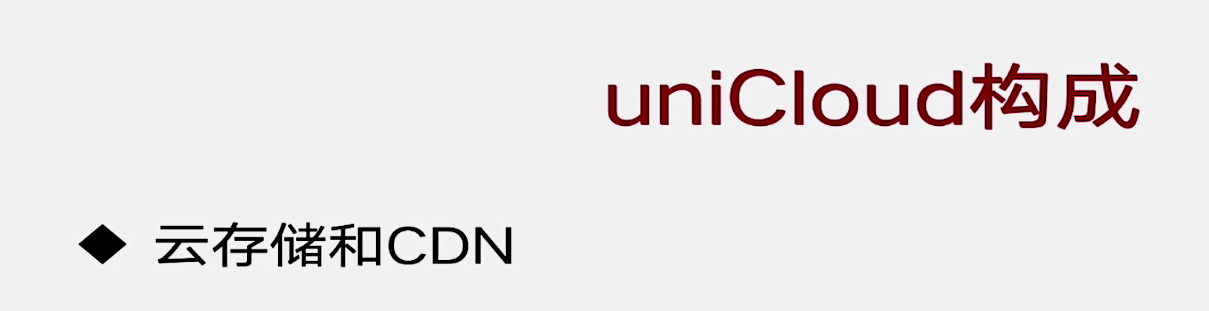

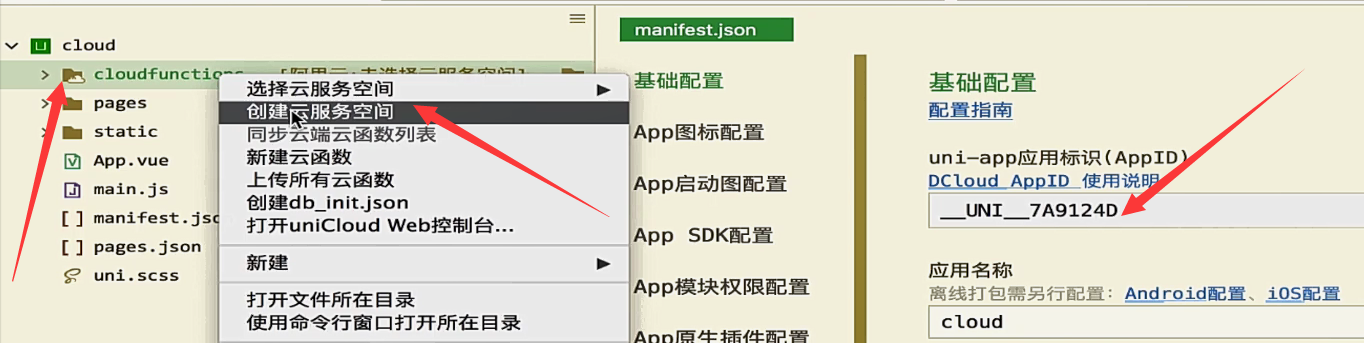
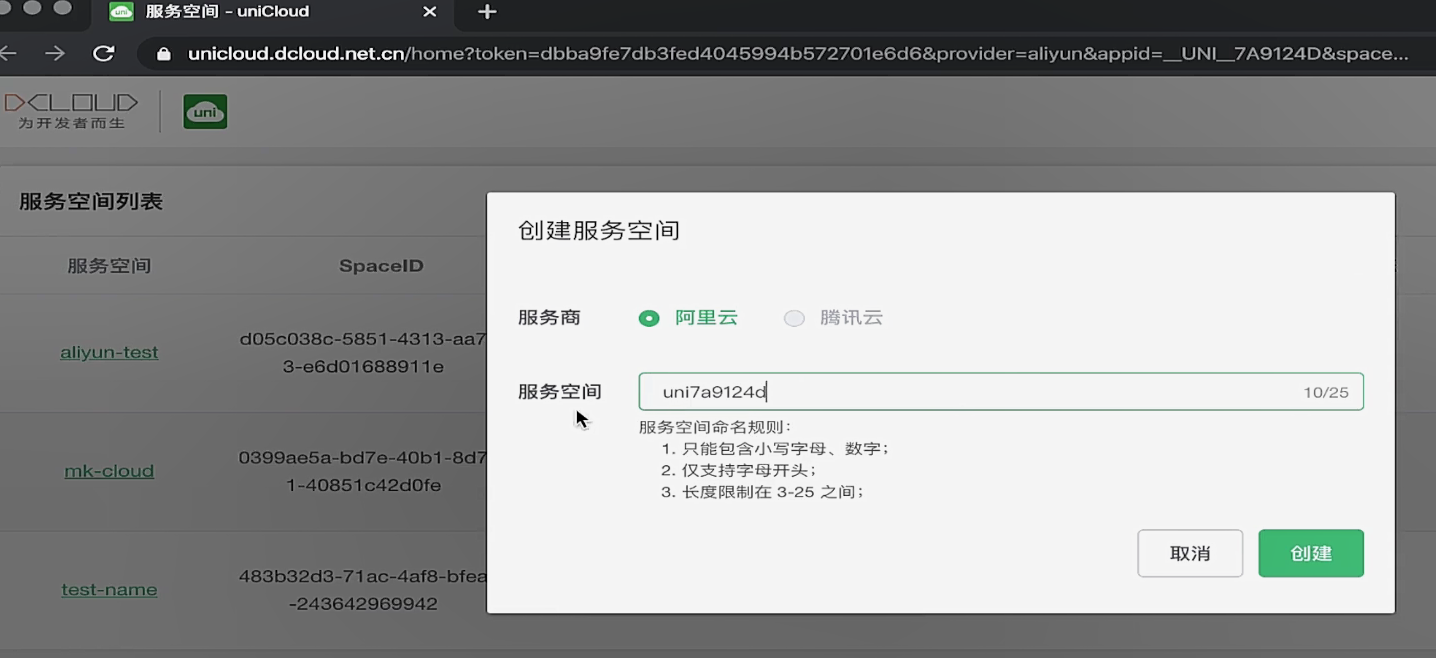
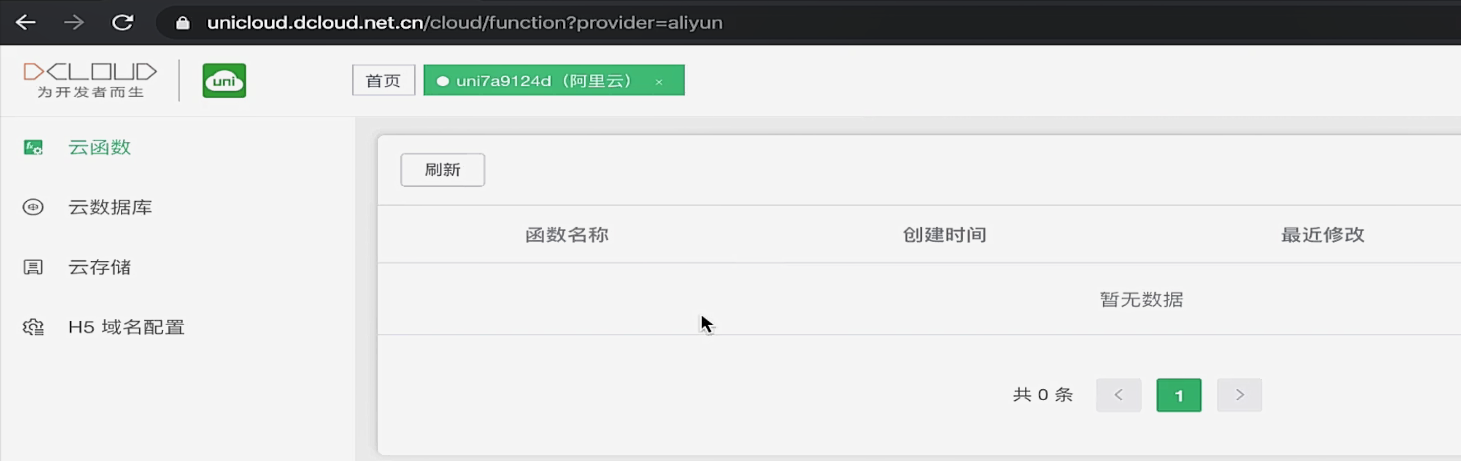
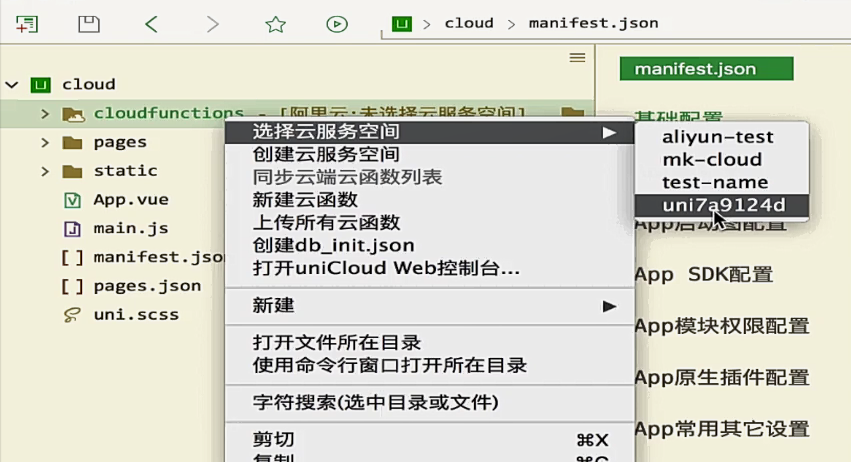
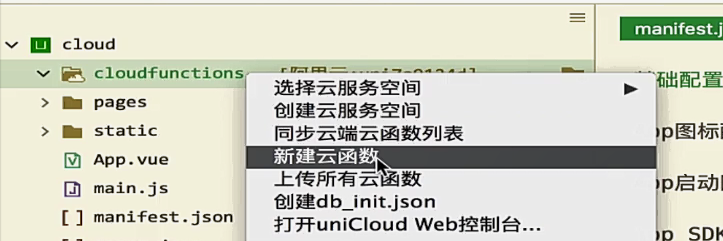
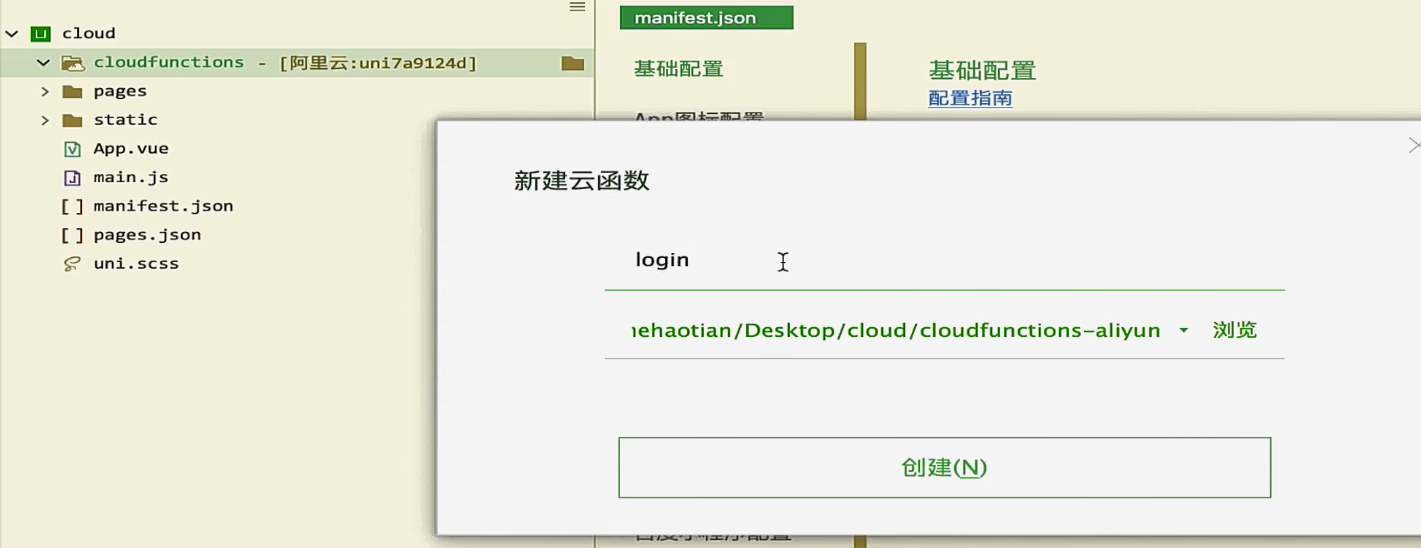
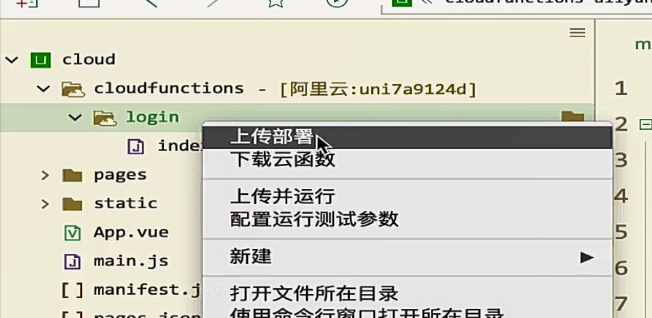
云函数的使用
index.vue
<template>
<view class="content">
<!-- <image class="logo" src="https://vkceyugu.cdn.bspapp.com/VKCEYUGU-62ae62c5-9a74-4911-a81a-e60651d1a985/bfcdf64a-3503-4c79-9d21-0fbac718664f.gif"></image> -->
<view class="text-area">
<text class="title">{{title}}</text>
</view>
<view class="">
<button type="default" @click="open">执行云函数</button>
</view>
</view>
</template>
<script>
export default {
data() {
return {
title: '杰帅666'
}
},
methods: {
open() {
// 前端代码通过uniCloud.callFunction()方法调用云函数
uniCloud.callFunction({
// name:云函数的名字
name: 'get_list',
// 传入参数
data: {
name: '呵呵',
age: 18
},
success(res) {
console.log(res);
},
fail(err) {
console.log(err);
}
})
}
}
}
</script>
<style>
.title {
font-size: 36rpx;
color: #8f8f94;
}
</style>
uniCloud-aliyun/cloudfunctions/get_list/index.js
'use strict';
// 运行在云端(服务器端)的函数,在node环境执行
exports.main = async (event, context) => {
// event为客户端上传的参数
console.log('event : ', event)
// context:包含了调用信息、运行状态,获取每次调用的上下文
// 返回数据给客户端
return {
code: 200,
status: '成功',
context,
event,
msg: `${event.name}的年龄是:${event.age}`
}
};
云数据库的添加和删除
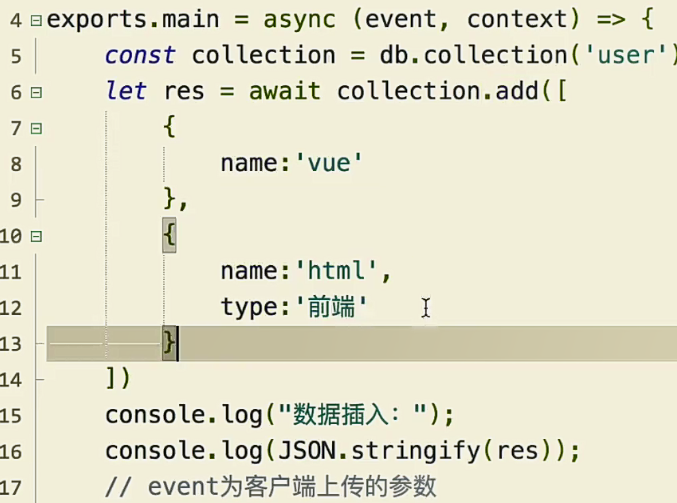
uniCloud-aliyun/cloudfunctions/get_list/index.js
'use strict';
const db = uniCloud.database()
const collection = db.collection('user') // 获取集合的引用
// 运行在云端(服务器端)的函数,在node环境执行
exports.main = async (event, context) => {
// event为客户端上传的参数
console.log('event : ', event)
// const collection = await db.collection('user') // 获取集合的引用
// 要写成数组的形式,只传单个对象,也要写成数组的形式,否则添加数据无效
let res = await collection.add([{
name: 'html',
age: 333
},
{
name: '前端',
age: 555
}
])
console.log('数据插入:');
console.log(JSON.stringify(res));
// doc:获取对该集合中指定 id 的记录的引用
// remove:删除记录(触发请求)
const res2 = await collection.doc('602a55affac28b0001ab7a80').remove()
console.log(res2);
// 返回数据给客户端
return {}
};
查询
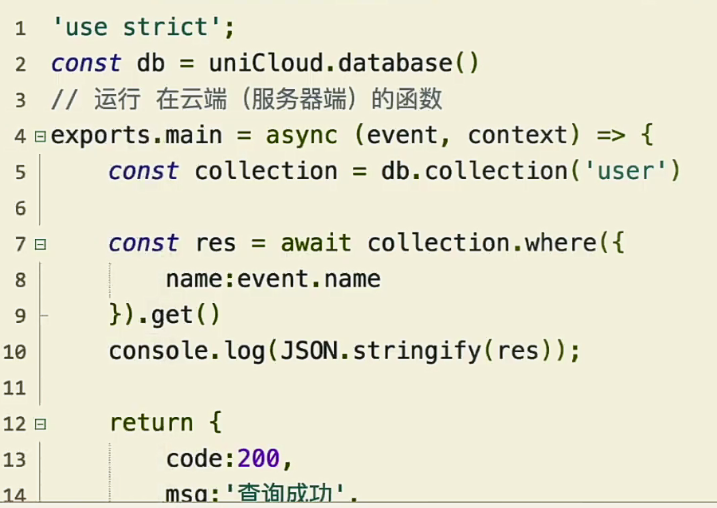
uniCloud-aliyun/cloudfunctions/get_list/index.js
'use strict';
const db = uniCloud.database()
// const collection = db.collection('user') // 获取集合的引用
// 运行在云端(服务器端)的函数,在node环境执行
exports.main = async (event, context) => {
const collection = db.collection('user') // 获取集合的引用
// event为客户端上传的参数
console.log('event--- ', event)
// ----------------添加数据------------------------
// 要写成数组的形式,只传单个对象,也要写成数组的形式,否则添加数据无效
const res = await collection.add([{
name: 'html',
age: 333
},
{
name: '前端',
age: 555
}
])
console.log('数据插入:');
console.log(JSON.stringify(res));
// ----------------删除数据------------------------
// doc:获取对该集合中指定 id 的记录的引用
// remove:删除记录(触发请求)
const res2 = await collection.doc('602a55affac28b0001ab7a80').remove()
console.log(res2);
// ---------------更新、添加数据-------------------------
// update:只能更新存在的记录
// set: 记录存在就更新,不存在就增加
const res3 = await collection.doc('602a560db6ce210001ac6af6').update({
name: 'vue',
age: 666,
job: 'coder'
})
console.log(res3);
const res5 = await collection.doc('123456').set({
name: 'css',
age: 777,
job: 'HR'
})
console.log(res5);
// ----------------查询数据------------------------
const res6 = await collection.doc('602a5accd6547d00017f34e4').get()
console.log(res6); // { affectedDocs: 1, data: [ { _id: '602a5accd6547d00017f34e4', name: '前端', age: 555 } ] }
const res7 = await collection.where({ name: 'uniapp' }).get()
console.log(res7); // { affectedDocs: 1, data: [ { _id: '602a3f2fd6547d00017ee0b8', name: 'uniapp' } ] }
// 查询字段通过客户端传入,使用event
const res8 = await collection.where({
"name": 'html'
}).get()
console.log(JSON.stringify(res8)); // {"affectedDocs":1,"data":[{"_id":"602a43e75dc5370001d7cf78","name":"html","age":333}]}
// 返回数据给客户端
return {
code: 200,
msg: '成功',
data: res8.data
}
};
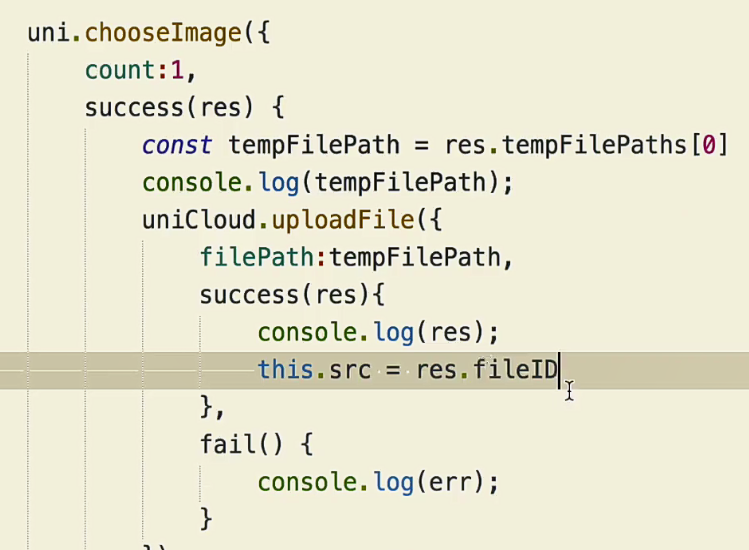
使用云存储上传文件
<template>
<view class="content">
<image class="logo" :src="src"></image>
<view class="text-area">
<text class="title">{{title}}</text>
</view>
<view class="">
<button type="default" @click="open">上传图片</button>
</view>
<view class="">
<button type="default" @click="deleteImg">删除图片</button>
</view>
</view>
</template>
<script>
export default {
data() {
return {
title: '杰帅666',
src: ''
}
},
methods: {
open() {
// 上传图片
uni.chooseImage({
count: 1,
success: res => {
// console.log('chooseImage---', res);
const tempFilePath = res.tempFilePaths[0]
// console.log('chooseImage---', tempFilePath);
// 注意,要使用uniCloud.uploadFile,而不是uni.uploadFile
uniCloud.uploadFile({
filePath: tempFilePath,
cloudPath: 'a.jpg',
success: res => {
// console.log('uploadFile---', res);
this.src = res.fileID
},
fail: rej => {
console.log('uploadFile---', rej);
}
})
},
fail: rej => {
console.log('chooseImage---', rej);
}
})
},
// 删除图片 【1、浏览器控制台输出err:没有权限删除;2、阿里云不支持then调用。】
deleteImg() {
uniCloud.deleteFile({
// fileList: ['20433d2a-4e11-43fb-823a-66d1b83b679d'],
// 文件的下载地址
fileList: ['https://vkceyugu.cdn.bspapp.com/VKCEYUGU-62ae62c5-9a74-4911-a81a-e60651d1a985/25ef0b4c-e4a7-4172-b889-9a74d1bd4a62.jpg'],
success: res => {
console.log('deleteImg---', res);
},
fail: rej => {
console.log('deleteImg---', rej);
}
})
}
}
}
</script>
<style>
.title {
font-size: 36rpx;
color: #8f8f94;
}
</style>