1、原型链继承 : 得到方法
function Parent(){}
Parent.prototype.test = function(){};
function Child(){}
Child.prototype = new Parent(); // 子类型的原型指向父类型实例
Child.prototype.constructor = Child
var child = new Child(); //有test()
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>01_原型链继承</title>
</head>
<body>
<!--
方式1: 原型链继承
1. 套路
1. 定义父类型构造函数
2. 给父类型的原型添加方法
3. 定义子类型的构造函数
4. 创建父类型的对象赋值给子类型的原型
5. 将子类型原型的构造属性设置为子类型
6. 给子类型原型添加方法
7. 创建子类型的对象: 可以调用父类型的方法
2. 关键
1. 子类型的原型为父类型的一个实例对象 【实例可以访问自己构造函数的原型对象的方法】
-->
<script type="text/javascript">
// Supper、Sub没有父子关系,只是我们自己把它们理解成父子类型。其实在js里,不是通过函数类型的继承来实现继承的
//父类型
// 按理,这里应该传形参。但是让子类的prototype指向父类的实例对象时,仅仅是为了访问父对象prototype上的方法,如果这里传形参就多余了,所以需要别的方式实现继承。
function Supper() {
this.supProp = 'Supper property'
}
Supper.prototype.showSupperProp = function() {
console.log(this.supProp)
}
//子类型
function Sub() {
this.subProp = 'Sub property'
}
// 子类型的原型为父类型的一个实例对象 【实例可以访问自己构造函数的原型对象的方法】
Sub.prototype = new Supper();
// 让子类型的原型的constructor指向子类型
Sub.prototype.constructor = Sub;
Sub.prototype.showSubProp = function() {
console.log(this.subProp)
}
var sub = new Sub()
sub.showSupperProp(); // Supper property
// sub.toString()
sub.showSubProp() // Sub property
console.log(sub) // Sub {subProp: "Sub property"}
console.log(Sub.prototype.constructor); // Sub自身
</script>
</body>
</html>
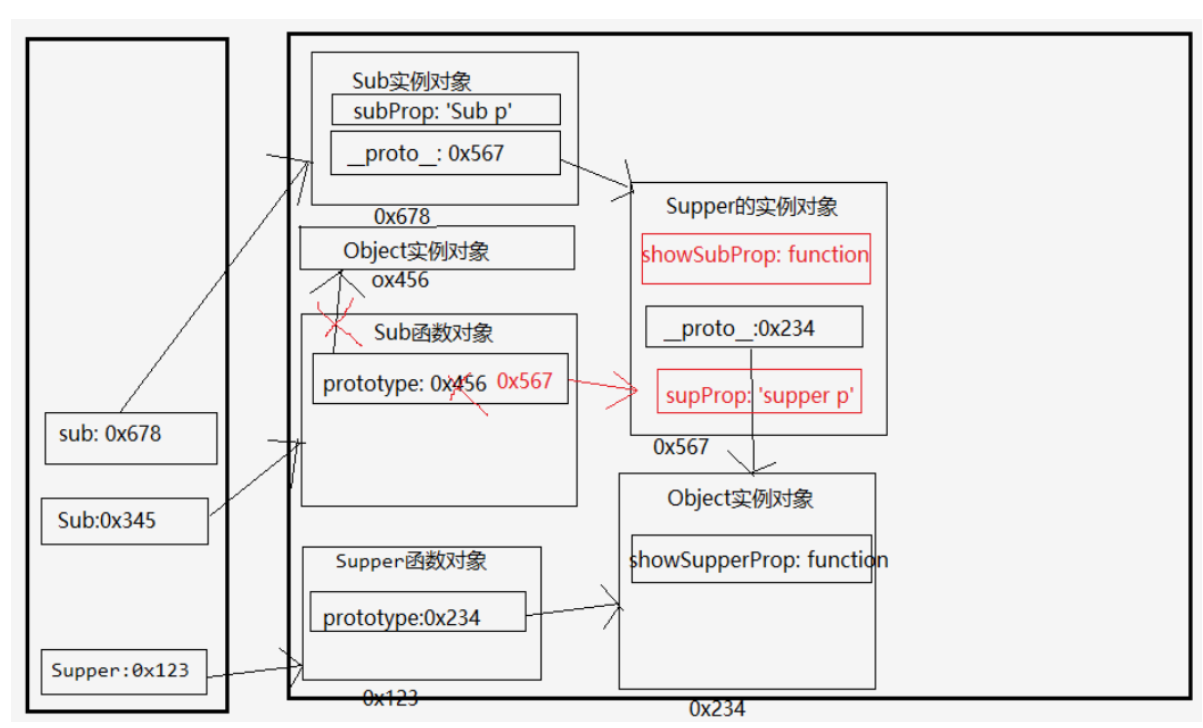
2、借用构造函数 : 得到属性
function Parent(xxx){this.xxx = xxx}
Parent.prototype.test = function(){};
function Child(xxx,yyy){
Parent.call(this, xxx);//借用构造函数 this.Parent(xxx)
}
var child = new Child('a', 'b'); //child.xxx为'a', 但child没有test()
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>02_借用构造函数继承</title>
</head>
<body>
<!--
方式2: 借用构造函数继承(假的)
1. 套路:
(1) 定义父类型构造函数
(2) 定义子类型构造函数
(3) 在子类型构造函数中调用父类型构造
2. 关键:
(1) 在子类型构造函数中通用call()调用父类型构造函数
-->
<script type="text/javascript">
function Person(name, age) {
this.name = name
this.age = age
}
function Student(name, age, price) {
Person.call(this, name, age); // 相当于: this.Person(name, age)
/*this.name = name
this.age = age*/
this.price = price
}
var s = new Student('Tom', 20, 14000)
console.log(s.name, s.age, s.price)
</script>
</body>
</html>
3、组合
function Parent(xxx){this.xxx = xxx}
Parent.prototype.test = function(){};
function Child(xxx,yyy){
Parent.call(this, xxx);//借用构造函数 this.Parent(xxx)
}
Child.prototype = new Parent(); //得到test()
var child = new Child(); //child.xxx为'a', 也有test()
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>03_组合继承</title>
</head>
<body>
<!--
方式3: 原型链+借用构造函数的组合继承
1. 利用原型链实现对父类型对象的方法继承
2. 利用super()借用父类型构建函数初始化相同属性
-->
<script type="text/javascript">
function Person(name, age) {
this.name = name
this.age = age
}
Person.prototype.setName = function(name) {
this.name = name
}
function Student(name, age, price) {
Person.call(this, name, age) // call是为了得到属性
this.price = price
}
Student.prototype = new Person() // 这里是为了能访问父类型的方法,不需要传任何参数
Student.prototype.constructor = Student //修正constructor属性
Student.prototype.setPrice = function(price) {
this.price = price
}
var s = new Student('Tom', 24, 15000)
s.setName('Bob')
s.setPrice(16000)
console.log(s.name, s.age, s.price) // Bob 24 16000
</script>
</body>
</html>
4、new一个对象背后做了些什么?
- 创建一个空对象
- 给对象设置__proto__, 值为构造函数对象的prototype属性值 【this.proto = Fn.prototype】
- 执行构造函数体(给对象添加属性/方法)