C# AOP监控特性MonitorAttribute
/*---------------------------------------------------------------- * 作 者 :姜 彦 * 项目名称 :EMRCPOE.Presentation.Common.Attributes * 类 名 称 :MonitorAttribute * 命名空间 :EMRCPOE.Presentation.Common.Attributes * CLR 版本 :4.0.30319.42000 * 创建时间 :2019/8/23 10:14:51 * 当前版本 :1.0.0.0 * WeChatQQ :771078740 * My Email :jiangyan2008.521@gmail.com * jiangyan2008.521@qq.com * 描述说明 :监视跟踪特性 * * 修改历史 : * ******************************************************************* * Copyright @ JiangYan 2019. All rights reserved. ******************************************************************* ------------------------------------------------------------------*/ using AspectCore.DynamicProxy; using AspectCore.DynamicProxy.Parameters; using EMRCPOE.Presentation.Common.Helper; using EMRCPOE.Presentation.Common.Message; using EMRCPOE.Presentation.Common.OperateMessage; using GalaSoft.MvvmLight.Messaging; using System; using System.Collections.Generic; using System.Diagnostics.CodeAnalysis; using System.Linq; using System.Text; using System.Threading.Tasks; using Util.Aspects.Base; namespace EMRCPOE.Presentation.Common.Attributes { /// <summary> /// 监视跟踪特性 /// </summary> public class MonitorAttribute : InterceptorBase { #region Construction public MonitorAttribute() { } public MonitorAttribute(string name) { _name.Value = name; } public MonitorAttribute(Type resourceType, string name) { _resourceType = resourceType; _name.Value = name; } #endregion #region Field /// <summary> /// 执行状态 /// </summary> private ExecuteState _executeState = null; #endregion #region Property private LocalizableString _name = new LocalizableString("Name"); /// <summary> /// 特性名称 /// </summary> public string Name { get { return this._name.Value; } set { if (this._name.Value != value) { this._name.Value = value; } } } private Type _resourceType; /// <summary> /// 特性资源文件 /// </summary> public Type ResourceType { get { return _resourceType; } set { if (this._resourceType != value) { this._resourceType = value; this._name.ResourceType = value; } } } #endregion #region Implementations /// <summary> /// 执行 /// </summary> /// <param name="context">切面上下文</param> /// <param name="next">切面委托</param> /// <returns></returns> public override async Task Invoke(AspectContext context, AspectDelegate next) { var methodName = GetMethodName(context); //var log = Log.GetLog(methodName); if (!Enabled()) return; ExecuteBefore(context, methodName); await next(context); ExecuteAfter(context, methodName); } #endregion #region Function #region protected /// <summary> /// 是否启用 /// </summary> protected virtual bool Enabled() { return true; } /// <summary> /// 写日志 /// </summary> protected void WriteLog() { } #endregion #region private /// <summary> /// 获取方法名 /// </summary> /// <param name="context">切面上下文</param> /// <returns></returns> private string GetMethodName(AspectContext context) { return $"{context.ServiceMethod.DeclaringType?.FullName}.{context.ServiceMethod?.Name}"; } /// <summary> /// 获取资源名称 /// </summary> /// <returns></returns> private string GetName() { return this._name.GetLocalizableValue(); } /// <summary> /// 执行前 /// </summary> /// <param name="context">切面上下文</param> /// <param name="methodName">方法名</param> private void ExecuteBefore(AspectContext context, string methodName) { _executeState = new ExecuteState(); _executeState.Id = Guid.NewGuid().ToString(); _executeState.Name = GetName() + MonitorName.ExecuteStart; _executeState.MethodName = methodName; _executeState.IsFinished = false; MonitorEventArgs args = new MonitorEventArgs(_executeState); MonitorObserver.Instance.Invoke(args); WriteLog(); } /// <summary> /// 执行后 /// </summary> /// <param name="context">切面上下文</param> /// <param name="methodName">方法名</param> private void ExecuteAfter(AspectContext context, string methodName) { _executeState.Name = GetName() + MonitorName.ExecuteEnd; _executeState.MethodName = methodName; _executeState.IsFinished = true; MonitorEventArgs args = new MonitorEventArgs(_executeState); MonitorObserver.Instance.Invoke(args); WriteLog(); } #endregion #endregion } } #region enabled code /* * //Messenger.Default.Send(new OperateMessage.OperateMessage { OperateType = OperateType.View, obj = Name+"开始执行..." }, MessengerToken.ExecuteState_OnMonitored); //MessageBox.Show(Name + "开始执行"); var name0 = context.ServiceMethod.Name; var name1 = context.ServiceMethod.DeclaringType?.FullName; var name2 = methodName; /// <summary> /// 执行后 /// </summary> /// <param name="context">切面上下文</param> /// <param name="methodName">方法名</param> private void ExecuteAfter(AspectContext context, string methodName) { _executeState.Name = Name + "执行完毕..."; _executeState.MethodName = methodName; _executeState.IsFinished = true; //Messenger.Default.Send(new OperateMessage.OperateMessage { OperateType = OperateType.View, obj = _executeState }, MessengerToken.ExecuteState_OnMonitored); //Messenger.Default.Send(new OperateMessage.OperateMessage { OperateType = OperateType.View, obj = Name + "执行完毕..." }, MessengerToken.ExecuteState_OnMonitored); //MessageBox.Show(Name + "执行完毕"); var name0 = context.ServiceMethod.Name; var name1 = context.ServiceMethod.DeclaringType?.FullName; var name2 = methodName; WriteLog(); } */ #endregion /*---------------------------------------------------------------- * 备 注 : * * * ******************************************************************* * Copyright @ JiangYan 2019. All rights reserved. ******************************************************************* ------------------------------------------------------------------*/
用法如下
/// <summary> /// 查询医院所有价格清单 /// </summary> /// <param name="query">查询条件</param> /// <returns></returns> [Monitor(ResourceType = typeof(MonitorName), Name = "IPriceService_QueryPriceList")] Task<List<PriceModel>> QueryPriceList(string hopitalCode, string languageCode); /// <summary> /// 查询医院所有药品价格清单 /// </summary> /// <param name="query">查询条件</param> /// <returns></returns> [Monitor(ResourceType = typeof(MonitorName), Name = "IPriceService_GetDrugPriceView")] Task<List<PriceModel>> GetDrugPriceView(string hopitalCode, string languageCode);
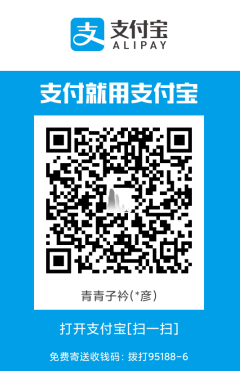
您的资助是我最大的动力!
金额随意,欢迎来赏!
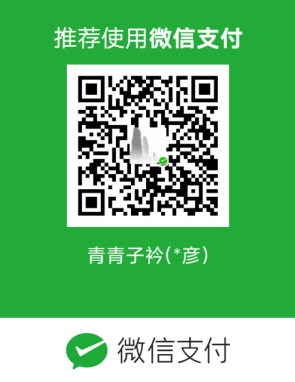
我写的东西能让你能懂,那是义务
毕竟占用了你生命中的宝贵的时间和注意力
要是你还能喜欢我的作品,那就是缘分了
如果,您希望更容易地发现我的新博客,不妨点击一下绿色通道的因为,我的写作热情也离不开您的肯定支持,感谢您的阅读,我是【青青子衿】!