打印zigzag矩阵 威盛笔试题
打印zigzag矩阵
在网上找到的大部分解法都是通过统计前面已经有多少个表格被访问,然后进行遍历。大部分都是基于这个矩阵的一个特征分布,但是如果只是按照这个 zigzag方式去遍历,而不存在那个数列按照递增方式的填充就不能通过每个元素的特征分布直接进行访问了。
我觉得可以使用状态机,不停的转移状态,因为总共最多也就四个状态。直到访问完所有的元素.
使用状态机,每次遍历一个元素都修改状态机,用来记录下一个需要访问的元素的访问方式,也就是说下一步要访问元素的一个定位方式,在每次访问完以后都更新下一个要访问的元素的值。
源代码如下:
// ZigZag.cpp : Defines the entry point for the console application. // #include "stdafx.h" #include "iostream" using namespace std; int N=0; enum Status { down_straight, up, right, down_tilt }; int _tmain(int argc, _TCHAR* argv[]) { cout<<"please input N:"<<endl; cin>>N; if (N<=0) { cout<<"wrong number"<<endl; system("pause"); return 0; } int **arr=(int **)malloc(N*sizeof(int *)); // 申请一个指向指针的指针,一个指针数组 if (arr==NULL) { return 0; } // 分配每一行的存储 for (int i=0;i<N;i++) { arr[i]=(int *)malloc(N*sizeof(int)); // 分配每一个数组的存储空间 if (arr[i]==NULL) { return 0; } } // 数组初始化 for (int i=0;i<N;i++) { for(int j=0;j<N;j++) { arr[i][j]=0; } } // 打印测试 for (int i=0;i<N;i++) { for(int j=0;j<N;j++) { printf("%3d",arr[i][j]); } printf("\n"); } // 开发ZigZag遍历 Status stat=down_straight; int index_x=0; int index_y=0; int value=0; while(index_x!=(N-1)||index_y!=(N-1)) { arr[index_x][index_y]=value; value++; // 更新下一个value值 // 更新一下步遍历状态 if (stat==down_straight) { index_x=index_x+1; index_y=index_y; if (index_y==0) { stat=up; } else if (index_y==(N-1)) { stat=down_tilt; } continue; } if (stat==up) { index_x=index_x-1; index_y=index_y+1; if (index_x==0&&index_y!=(N-1)) { // 向上走到边界 stat=Status::right; } else if (index_y==(N-1)) { stat=down_straight; } else { stat=up; } continue; } if (stat==Status::right) { index_x=index_x; index_y=index_y+1; if (index_x==0) { stat=down_tilt; } else if (index_x==(N-1)) { stat=up; } continue; } if (stat==Status::down_tilt) { index_x=index_x+1; index_y=index_y-1; if (index_y==0&&index_x!=(N-1)) { stat=down_straight; } else if (index_x==(N-1)) { stat=Status::right; } else { stat=down_tilt; } continue; } } arr[index_x][index_y]=value; printf("***********************************************"); printf("\n"); // 打印测试 for (int i=0;i<N;i++) { for(int j=0;j<N;j++) { printf("%3d",arr[i][j]); } printf("\n"); } system("pause"); return 0; }
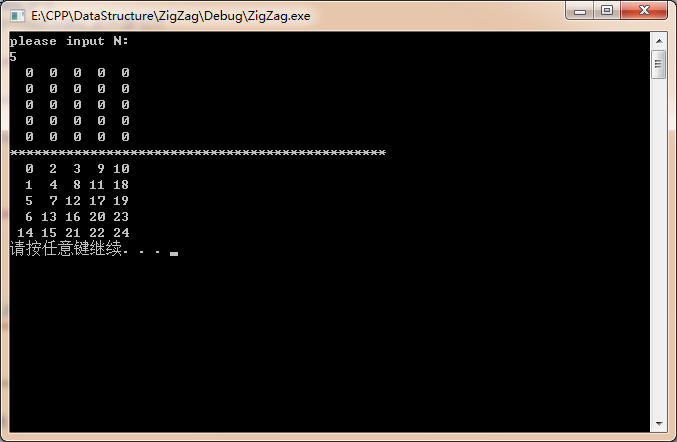