hdu A Famous Grid
A Famous Grid
Problem Description
Mr. B has recently discovered the grid named "spiral grid".
Construct the grid like the following figure. (The grid is actually infinite. The figure is only a small part of it.)
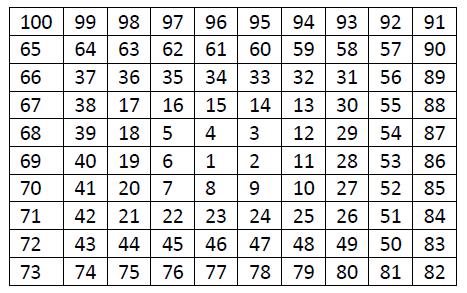
Considering traveling in it, you are free to any cell containing a composite number or 1, but traveling to any cell containing a prime number is disallowed. You can travel up, down, left or right, but not diagonally. Write a program to find the length of the shortest path between pairs of nonprime numbers, or report it's impossible.
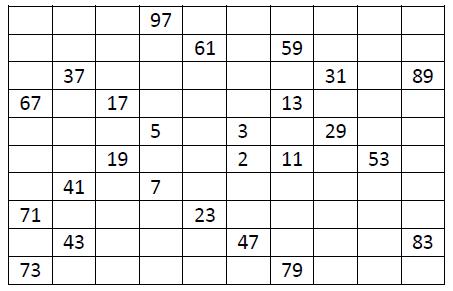
Construct the grid like the following figure. (The grid is actually infinite. The figure is only a small part of it.)
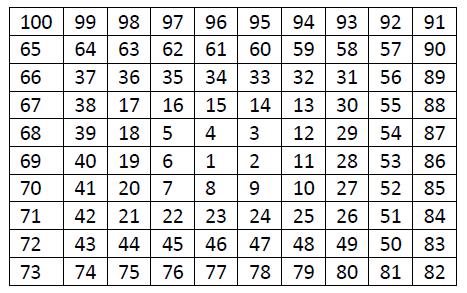
Considering traveling in it, you are free to any cell containing a composite number or 1, but traveling to any cell containing a prime number is disallowed. You can travel up, down, left or right, but not diagonally. Write a program to find the length of the shortest path between pairs of nonprime numbers, or report it's impossible.
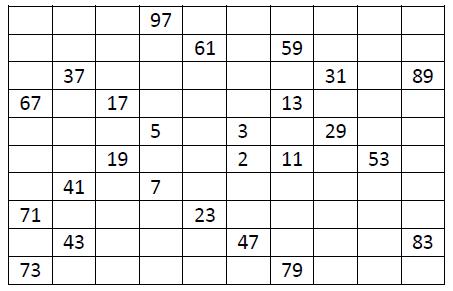
Input
Each test case is described by a line of input containing two nonprime integer 1 <=x, y<=10,000.
Output
For each test case, display its case number followed by the length of the shortest path or "impossible" (without quotes) in one line.
Sample Input
1 4
9 32
10 12
Sample Output
Case 1: 1
Case 2: 7
Case 3: impossible
AC代码:
#include <stdio.h> #include <string.h> #include <queue> using namespace std; int x[40000],y[40000]; int valid[200+5][200+5]; int dx[4]={0,0,1,-1}; int dy[4]={1,-1,0,0}; int xa,ya,xb,yb; struct node { int x,y; int step; }; int isprime( int n) { int i; if(n<2) return 0; for( i=2;i*i<=n;i++) { if(n%i==0) return 0; } return 1; } int in( int x,int y) { return x>=0&&y>=0&&x<200&&y<200; } void prepare( ) { int px,py,i,now,j; for( now=1,i=1,px=100,py=100;;i+=2) { for( j=0;j<i;j++) { x[now]=px; y[now]=py; valid[px][py]=!isprime(now); px++; now++; if(!in(px,py)) return ; } for( j=0;j<i;j++) { x[now]=px; y[now]=py; valid[px][py]=!isprime(now); py++; now++; if(!in(px,py)) return ; } for( j=0;j<=i;j++) { x[now]=px; y[now]=py; valid[px][py]=!isprime(now); px--; now++; if(!in(px,py)) return ; } for( j=0;j<=i;j++) { x[now]=px; y[now]=py; valid[px][py]=!isprime(now); py--; now++; if(!in(px,py)) return ; } } } int bfs( ) { int visit[400][400]; memset(visit,0,sizeof(visit)); int i; queue<node>q; node p; p.x=xa; p.y=ya; p.step=0; q.push(p); while(!q.empty( )) { node px=q.front( ); q.pop( ); xa=px.x; ya=px.y; visit[xa][ya]=1; int dis=px.step; if(xa==xb&&ya==yb) { printf(" %d\n",dis); return 1; } for( i=0;i<4;i++) { int xx=xa+dx[i],yy=ya+dy[i]; if(in(xx,yy)&&valid[xx][yy]&&visit[xx][yy]==0) { node py; py.x=xx,py.y=yy,py.step=dis+1; visit[xx][yy]=1; q.push(py); } } } return 0; } int main( ) { int a,b; int t=1; prepare( );
while(scanf("%d%d",&a,&b)!=EOF) { xa=x[a]; ya=y[a]; xb=x[b]; yb=y[b]; printf("Case %d:",t++); if(!bfs( )) puts(" impossible"); } return 0; }