Imports System Imports System.Data Imports System.Data.SqlClient namespace HowTo.Samples.ADONET public class updatingdata public shared sub Main() Dim myupdatingdata as updatingdata myupdatingdata = new updatingdata() myupdatingdata.Run() end sub public sub Run() Dim myConnection as SqlConnection Dim mySqlDataAdapter as SqlDataAdapter Dim mySqlDataAdapter1 as SqlDataAdapter myConnection = new SqlConnection("server=(local)\NetSDK;Trusted_Connection=yes;database=northwind") mySqlDataAdapter = new SqlDataAdapter("select * from customers", myConnection) mySqlDataAdapter1 = new SqlDataAdapter("select * from orders", myConnection) ' Restore database to it's original condition so sample will work correctly. Cleanup() try Dim myDataSet as DataSet = new DataSet() Dim myDataRow as DataRow ' Create command builder. This line automatically generates the update commands for you, so you don't ' have to provide or create your own. Dim myDataRowsCommandBuilder as SqlCommandBuilder = new SqlCommandBuilder(mySqlDataAdapter) ' Set the MissingSchemaAction property to AddWithKey because Fill will not cause primary ' key & unique key information to be retrieved unless AddWithKey is specified. mySqlDataAdapter.MissingSchemaAction = MissingSchemaAction.AddWithKey mySqlDataAdapter1.MissingSchemaAction = MissingSchemaAction.AddWithKey mySqlDataAdapter.Fill(myDataSet,"Customers") Console.WriteLine("已将数据从 Customers 表加载到数据集中。") mySqlDataAdapter1.Fill(myDataSet,"Orders") Console.WriteLine("已将数据从 Orders 表加载到数据集中。") ' ADD RELATION myDataSet.Relations.Add("CustOrders",myDataSet.Tables("Customers").Columns("CustomerId"),myDataSet.Tables("Orders").Columns("CustomerId")) ' EDIT myDataSet.Tables("Customers").Rows(0)("ContactName")="Peach" ' ADD myDataRow = myDataSet.Tables("Customers").NewRow() myDataRow("CustomerId") ="新 ID" myDataRow("ContactName") = "新姓名" myDataRow("CompanyName") = "新公司名称" myDataSet.Tables("Customers").Rows.Add(myDataRow) Console.WriteLine("已将新行插入 Customers。") ' Update Database with SqlDataAdapter mySqlDataAdapter.Update(myDataSet, "Customers") Console.WriteLine("已将更新发送到数据库。") Console.WriteLine("数据集处理已成功完成!") catch e as Exception Console.WriteLine(e.ToString()) end try end sub public sub Cleanup() Dim myConnection as SqlConnection Dim CleanupCommand as SqlCommand myConnection = new SqlConnection("server=(local)\NetSDK;Trusted_Connection=yes;database=northwind") try ' Restore database to it's original condition so sample will work correctly. myConnection.Open() CleanupCommand = new SqlCommand("DELETE FROM Customers WHERE CustomerId = 'NewID'", myConnection) CleanupCommand.ExecuteNonQuery() catch e as Exception Console.WriteLine(e.ToString()) finally myConnection.Close() end try end sub end class end namespace
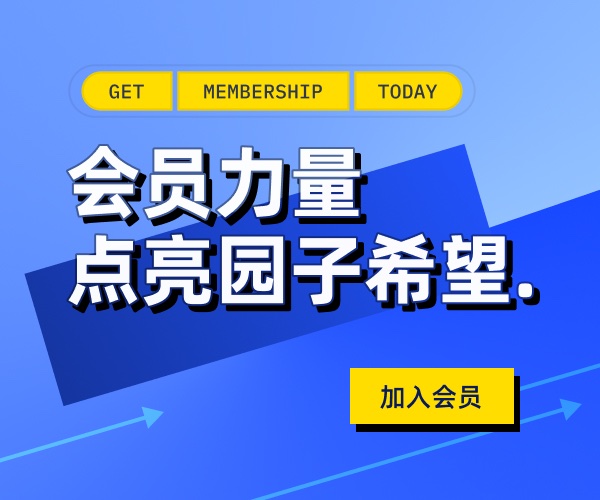