实验7. 8 .9
实验九:异常的抛出,捕获并处理
package jiangcai; public class Throwerror { public static void main(String[] args) throws IllegalArgumentException { Point point1=new Point (3,1); Point point2=new Point (6,2); Point point3=new Point (9,3); new Rectangle(point1, -12,3); new Triangle(point1,point2,point3); Point[] point= {point1,point2}; new Polygon(point); } } class Point { public Point() {}; public int x; public int y; public Point(int x,int y) { this.x=x; this.y=y; if(x<0||y<0) throw new IllegalArgumentException("Point中无效参数异常"); } } class Rectangle extends Point { public int length,width; public Rectangle(Point point1,int length,int width) { this.length=length; this.width=width; if(length<0||width<0) throw new IllegalArgumentException("Rectangle无效参数异常"); } } class Triangle extends Point { public Triangle(Point point1,Point point2,Point point3) { if(point1.x/point1.y==point2.x/point2.y&&point2.x/point2.y==point3.x/point3.y) throw new IllegalArgumentException("Triangle无效参数异常"); } } class Polygon extends Point { int i=0; public Polygon(Point[] points) { i=points.length; if(i<=2) throw new IllegalArgumentException("Polygon无效参数异常"); } }
实验八:接口与实现接口的类
package 项目;
interface Area
{
public abstract double area();
}
interface Volume
{
public abstract double volume();
}
public class yuanzhui extends Object implements Area,Volume
{
int height,raduis,length;
public yuanzhui(int height,int raduis,int length)
{
this.height=height;
this.raduis=raduis;
this.length=length;
}
public double area()
{
return (Math.PI*raduis*length+Math.PI*raduis*2);
}
public double volume()
{
return height*Math.PI*raduis*2/3;
}
public static double max(yuanzhui X,yuanzhui Y)
{
System.out.print("体积较大圆锥体体积为:");
if(X.volume()>Y.volume())
return X.volume();
else
return Y.volume();
}
public static void main(String[] args) {
yuanzhui YZ=new yuanzhui(3,4,5);
yuanzhui yz=new yuanzhui(4,5,6);
System.out.println("圆锥1表面积为:"+YZ.area());
System.out.println("圆锥2表面积为:"+yz.area());
System.out.println("圆锥1体积为:"+YZ.volume());
System.out.println("圆锥2体积为:"+yz.volume());
System.out.println("圆锥体积较大的为:"+Math.max(yz.volume(),YZ.volume()));
}
}
结果:
圆锥1表面积为:87.96459430051421
圆锥2表面积为:125.66370614359172
圆锥1体积为:25.132741228718345
圆锥2体积为:41.88790204786391
圆锥体积较大的为:41.88790204786391
实验七:类的多态
public String name;
public String birthday;
public String grade;
public String gender;
public String province;
public String city;
public static int count=0;
this.name=name;
this.birthday=birthday;
this.grade=grade;
this.gender=gender;
this.province=province;
this.city=city;
count++;
// TODO 自动生成的构造函数存根
}
public static void main(String[] args) {
Student stud1=new Student("李明","1998-1-1","2017","男","青海省","西宁市","计算机","网络工程",88,89);
stud1.show1();
stud1.Setnumber();
Student stud2=new Student("张三","1999-11-1","2018","男","四川省","成都市","计算机","物联网工程",98,96);
stud2.show1();
stud2.Setnumber();
Student stud3=new Student("王二","2000-10-1","2017","女","辽宁省","大连市","计算机","计算机科学与技术",78,84);
stud3.show1();
stud3.Setnumber();
Student stud4=new Student("张四","1997-12-1","2017","女","青海省","海东市","计算机","网络工程",74,84);
stud4.show1();
stud4.Setnumber();
stud1.searchname("张");
stud2.searchname("张");
stud3.searchname("张");
stud1.searchplace("青海省");
stud2.searchplace("青海省");
stud3.searchplace("青海省");
stud1.Sum();
stud2.Sum();
stud3.Sum();
stud4.Sum();
}
}
class Student extends person1
{
public String department,speciality;
public int math;
public int computer;
public static int sum=0;
public static int total=0;
public static int sum1=0;
public static int total1=0;
public static int sum2=0;
public static int total2=0;
public Student(String name,String birthday,String grade,String gender,String province,String city,String department,String speciality,int math,int computer)
{
super(name,birthday,grade,gender,province,city);
this.department=department;
this.speciality=speciality;
this.math=math;
this.computer=computer;
}
public void show1()
{
System.out.println("\n");
System.out.println("基本信息为:");
System.out.println("姓名为:"+this.name);
System.out.println("生日为:"+this.birthday);
System.out.println("性别为:"+this.gender);
System.out.println("省份为:"+this.province);
System.out.println("城市为:"+this.city);
System.out.println("系为:"+this.department);
System.out.println("专业为:"+this.speciality);
System.out.println("数学成绩为:"+this.math);
System.out.println("计算机成绩为:"+this.computer);
}
public void Setnumber()
{
System.out.print("学号为:");
if(this.speciality=="网络工程")
System.out.println(this.grade+"33111"+this.count);
else if(this.speciality=="物联网工程")
System.out.println(this.grade+"33112"+this.count);
else if(this.speciality=="计算机科学与技术")
System.out.println(this.grade+"33113"+this.count);
}
public void searchname(String nam)
{
int i=1;
i=name.indexOf(nam);
if(i!=-1) {
System.out.println("\n");
System.out.println("输入查找姓名信息的查找结果为:");
System.out.println(nam);
System.out.println(name+" "+birthday+" "+grade+" "+gender+" "+province+" "+city+" "+department+" "+speciality+" "+math+" "+computer);
}
}
public void searchplace(String place)
{
int i=1;
i=province.indexOf(place);
if(i!=-1) {
System.out.println("\n");
System.out.println("输入查找地名信息的查找结果为:");
System.out.println(place);
System.out.println(name+" "+birthday+" "+grade+" "+gender+" "+province+" "+city+" "+department+" "+speciality+" "+math+" "+computer);
}
}
public void Sum()
{
System.out.println("\n");
if(this.speciality=="网络工程") {
System.out.println("经汇总后的网络工程班的数学成绩和计算机成绩分别为:");
this.sum=this.sum+this.math;
this.total=this.total+this.computer;
System.out.println(this.sum);
System.out.println(this.total);
}
else if(this.speciality=="物联网工程"){
System.out.println("经汇总后的物联网工程班的数学成绩和计算机成绩分别为:");
this.sum1=this.sum1+this.math;
this.total1=this.total1+this.computer;
System.out.println(this.sum1);
System.out.println(this.total1);
}
else if(this.speciality=="计算机科学与技术"){
System.out.println("经汇总后的计算机科学班的数学成绩和计算机成绩分别为:");
this.sum2=this.sum2+this.math;
this.total2=this.total2+this.computer;
System.out.println(this.sum2);
System.out.println(this.total2);
}
}
}
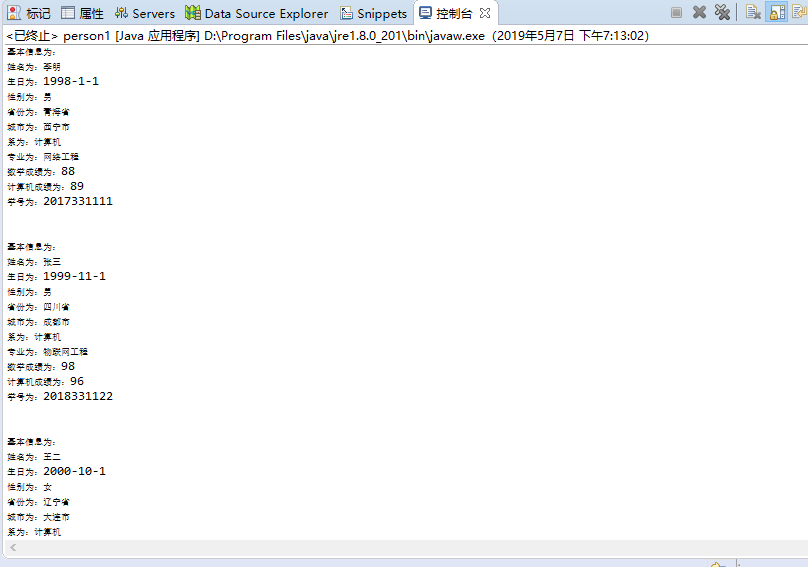