var ajax = function(o){
/*
* @o.url Request url
* @o.method Set to post or get
* @o.async Set async or not
* @o.data Parameters to be sending
* @o.success Callback function for success
* @o.fail Callback function for fail
* @o.header Set request http herder
* @o.user Set username
* @o.pwd Set password
*/
var xhr = window.XMLHttpRequest ? new XMLHttpRequest() : new ActiveXObject("Microsoft.XMLHTTP");
var data = null;
var url = o.url;
if( !o.data ) o.data = {};
o.data['NoCache'] = Math.random();
var temp= [];
for(var i in o.data) temp.push(i+"="+o.data[i]);
data = temp.join("&");
if( !o.method ) o.method = 'get';
if( o.method=="get" && data!=null ){
url = url+( /\?/.test(url) ? '&' : '?' )+data;
data = null
}
xhr.open( o.method, url, o.async||true, o.user||'', o.pwd||'' );
if(o.header){
for(var type in o.header){
xhr.setRequestHeader( type, o.header[type] );
}
}
if(!o.header || !o.header['Content-Type']) xhr.setRequestHeader('Content-Type','application/x-www-form-urlencoded');
xhr.onreadystatechange = function(){
if(xhr.readyState==4){
if(xhr.status==200||xhr.status==206){
if(o.success){ o.success.apply(xhr,[xhr.responseText]); }
}else{
if(o.fail){ o.fail.apply(xhr); }
}
}
};
xhr.send(data);
};
ajax({
url:"draw.html",
method:"get",
async:"true",
data:{ "url":"test", "sgg":"kktest" },
header:{
'Content-Type':'application/x-www-form-urlencoded; charset=UTF-8',
'Range':'bytes=0-2'
},
success:function(ret){
alert(ret);
alert(this.getAllResponseHeaders());
},
fail:function(){
alert(this.status);
}
});
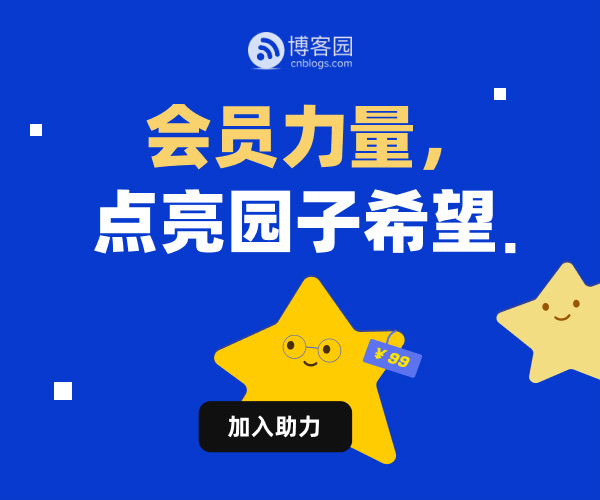