POJ 1681· Painter's Problem (位压缩 或 高斯消元)
Painter's Problem
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 3880 | Accepted: 1889 |
Description
There is a square wall which is made of n*n small square bricks. Some bricks are white while some bricks are yellow. Bob is a painter and he wants to paint all the bricks yellow. But there is something wrong with Bob's brush. Once he uses this brush to paint brick (i, j), the bricks at (i, j), (i-1, j), (i+1, j), (i, j-1) and (i, j+1) all change their color. Your task is to find the minimum number of bricks Bob should paint in order to make all the bricks yellow.
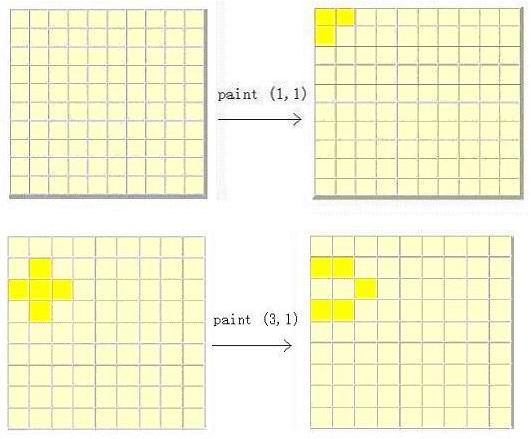
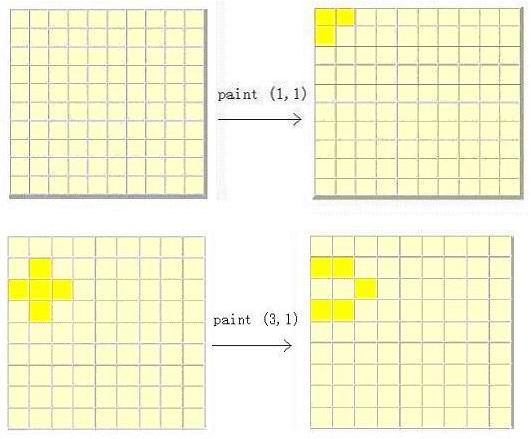
Input
The first line contains a single integer t (1 <= t <= 20) that indicates the number of test cases. Then follow the t cases. Each test case begins with a line contains an integer n (1 <= n <= 15), representing the size of wall. The next n lines represent the original wall. Each line contains n characters. The j-th character of the i-th line figures out the color of brick at position (i, j). We use a 'w' to express a white brick while a 'y' to express a yellow brick.
Output
For each case, output a line contains the minimum number of bricks Bob should paint. If Bob can't paint all the bricks yellow, print 'inf'.
Sample Input
2 3 yyy yyy yyy 5 wwwww wwwww wwwww wwwww wwwww
Sample Output
0 15
Source
题意:
刷墙每次刷一格会将上下左右中五个格子变色,求最少的刷方法使得所有的格子都变成yellow。
分析:
与POJ1185 炮兵阵地 有相似之处,状态枚举和位运算。同样从第一行开始枚举所有粉刷状态,然后后面每行每格paint[k][p]的粉刷情况要根据wall[k-1][p]经过paint[k-1][p]、paint[k-1][p-1]、paint[k-1][p+1]、paint[k-2][p]后的情况而定。若为1(即白色)则paint[k][p]=1。所有行处理完后,判断墙壁是否已经全为黄色,并记录下paint[i][j]为1的个数。循环至第一行枚举了0000 - 1111所有状态。
#include<iostream> #include<cstdio> #include<cstring> using namespace std; bool wall[20][20],paint[20][20]; int n,count; bool allYellow(){ count=0; for(int i=1;i<=n;i++) for(int j=1;j<=n;j++){ if(wall[i][j]^paint[i][j]^paint[i-1][j]^paint[i][j-1]^paint[i+1][j]^paint[i][j+1]) return 0; if(paint[i][j]) count++; } return 1; } int main(){ //freopen("input.txt","r",stdin); int t; scanf("%d",&t); char str[20]; while(t--){ scanf("%d",&n); memset(wall,0,sizeof(wall)); for(int i=1;i<=n;i++){ scanf("%s",str+1); for(int j=1;j<=n;j++) wall[i][j]=(str[j]=='w'); } int maxn=1<<n; int flag=0,ans=300; for(int i=0;i<maxn;i++){ memset(paint,0,sizeof(paint)); int tmp=i; for(int k=1;k<=n;k++){ paint[1][k]=tmp&1; tmp>>=1; } for(int j=2;j<=n;j++) for(int k=1;k<=n;k++) //根据wall[k-1][p]的paint后状态 决定paint[k][p]值 if(wall[j-1][k]^paint[j-1][k]^paint[j-1][k+1]^paint[j-1][k-1]^paint[j-2][k]) paint[j][k]=1; if(allYellow()){ flag=1; ans=min(ans,count); } } if(flag) printf("%d\n",ans); else printf("inf\n"); } return 0; }
/* POJ 1681 */ #include<stdio.h> #include<algorithm> #include<math.h> #include<string.h> #include<iostream> using namespace std; const int MAXN=300; //有equ个方程,var个变元。增广矩阵行数为equ,分别为0到equ-1,列数为var+1,分别为0到var. int equ,var; int a[MAXN][MAXN];//增广矩阵 int x[MAXN];//解集 bool free_x[MAXN];//标记是否是不确定的变元 int free_num;//不确定变元个数 void Debug(void) { int i, j; for (i = 0; i < equ; i++) { for (j = 0; j < var + 1; j++) { cout << a[i][j] << " "; } cout << endl; } cout << endl; } int Gauss() { int i,j,k; int max_r; int col; int temp; int free_x_num; int free_index; col=0; for(k=0;k<equ&&col<var;k++,col++) { max_r=k; for(i=k+1;i<equ;i++) { if(abs(a[i][col])>abs(a[max_r][col]))max_r=i; } if(max_r!=k) { for(j=col;j<var+1;j++)swap(a[k][j],a[max_r][j]); } if(a[k][col]==0) { k--; continue; } for(i=k+1;i<equ;i++) { if(a[i][col]!=0) { for(j=col;j<var+1;j++) a[i][j]^=a[k][j]; } } } for(i=k;i<equ;i++) { if(a[i][col]!=0)return -1;//无解 } for(i=var-1;i>=0;i--) { x[i]=a[i][var]; for(j=i+1;j<var;j++) x[i]^=(a[i][j]&&x[j]); } return 0; } int n; void init() { memset(a,0,sizeof(a)); memset(x,0,sizeof(x)); memset(free_x, 1, sizeof(free_x)); // 一开始全是不确定的变元. equ=n*n; var=n*n; for(int i=0;i<n;i++) for(int j=0;j<n;j++) { int t=i*n+j; a[t][t]=1; if(i>0)a[(i-1)*n+j][t]=1; if(i<n-1)a[(i+1)*n+j][t]=1; if(j>0)a[i*n+j-1][t]=1; if(j<n-1)a[i*n+j+1][t]=1; } } char str[20]; int main() { // freopen("in.txt","r",stdin); // freopen("out.txt","w",stdout); int T; scanf("%d",&T); while(T--) { scanf("%d",&n); init(); for(int i=0;i<n;i++) { scanf("%s",&str); for(int j=0;j<n;j++) { if(str[j]=='y')a[i*n+j][n*n]=0; else a[i*n+j][n*n]=1; } } int t=Gauss(); if(t==-1) { printf("inf\n"); continue; } int ans=0; for(int i=0;i<n*n;i++) if(x[i]==1)ans++; printf("%d\n",ans); } return 0; }