实验 6
实验任务 4
// Vector.hpp
#include <iostream>
#include <stdexcept>
#include <memory>
template <typename T>
class Vector {
public:
// 构造函数,动态指定大小
explicit Vector(int size) {
if (size < 0) {
throw std::length_error("Size cannot be negative");
}
_size = size;
_data = std::make_unique<T[]>(size);
}
// 构造函数,指定大小并初始化值
Vector(int size, T value) {
if (size < 0) {
throw std::length_error("Size cannot be negative");
}
_size = size;
_data = std::make_unique<T[]>(size);
for (int i = 0; i < size; ++i) {
_data[i] = value;
}
}
// 用已有 Vector 对象构造新的对象(深复制)
Vector(const Vector<T>& other) {
_size = other._size;
_data = std::make_unique<T[]>(_size);
for (int i = 0; i < _size; ++i) {
_data[i] = other._data[i];
}
}
// 析构函数,释放内存资源
~Vector() = default;
// 获取大小
int get_size() const {
return _size;
}
// 通过索引访问元素
T& at(int index) {
if (index < 0 || index >= _size) {
throw std::out_of_range("Index out of range");
}
return _data[index];
}
// 重载[]运算符
T& operator[](int index) {
return at(index);
}
// 友元函数,实现输出 Vector 类对象中的数据项
friend void output(const Vector<T>& vec) {
for (int i = 0; i < vec._size; ++i) {
std::cout << vec._data[i] << " ";
}
}
private:
int _size;
std::unique_ptr<T[]> _data;
};
#include <iostream>
#include "Vector.hpp"
void test1() {
using namespace std;
int n;
cout << "Enter n: ";
cin >> n;
Vector<double> x1(n);
for(auto i = 0; i < n; ++i)
x1.at(i) = i * 0.7;
cout << "x1: "; output(x1);
Vector<int> x2(n, 42);
const Vector<int> x3(x2);
cout << "x2: "; output(x2);
cout << "x3: "; output(x3);
x2.at(0) = 77;
x2.at(1) = 777;
cout << "x2: "; output(x2);
cout << "x3: "; output(x3);
}
void test2() {
using namespace std;
int n, index;
while(cout << "Enter n and index: ", cin >> n >> index) {
try {
Vector<int> v(n, n);
v.at(index) = -999;
cout << "v: "; output(v);
}
catch (const exception &e) {
cout << e.what() << endl;
}
}
}
int main() {
std::cout << "²âÊÔ1: Ä£°åÀà½Ó¿Ú²âÊÔ\n";
test1();
std::cout << "\n²âÊÔ2: Ä£°åÀàÒì³£´¦Àí²âÊÔ\n";
test2();
}
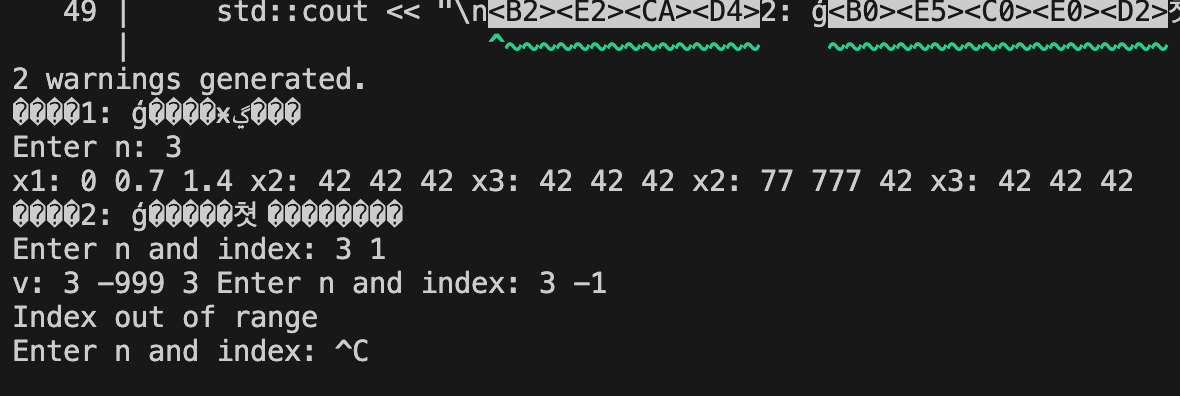
实验任务 5
#include <fstream>
#include <iostream>
#include <string>
using namespace std;
struct Student {
int id;
string name;
string major;
int score;
};
int main() {
auto fin = ifstream("data5.txt");
if (!fin) {
cout << "Failed to open file." << endl;
return 1;
}
string line;
getline(fin, line);
auto stus = vector<Student>();
while (!fin.eof()) {
int id, score;
string name, major;
fin >> id >> name >> major >> score;
stus.push_back({id, name, major, score});
}
auto fout = ofstream("ans5.txt");
for (auto &stu : stus) {
cout << stu.id << " " << stu.name << " " << stu.major << " " << stu.score
<< endl;
fout << stu.id << " " << stu.name << " " << stu.major << " " << stu.score
<< endl;
}
return 0;
}
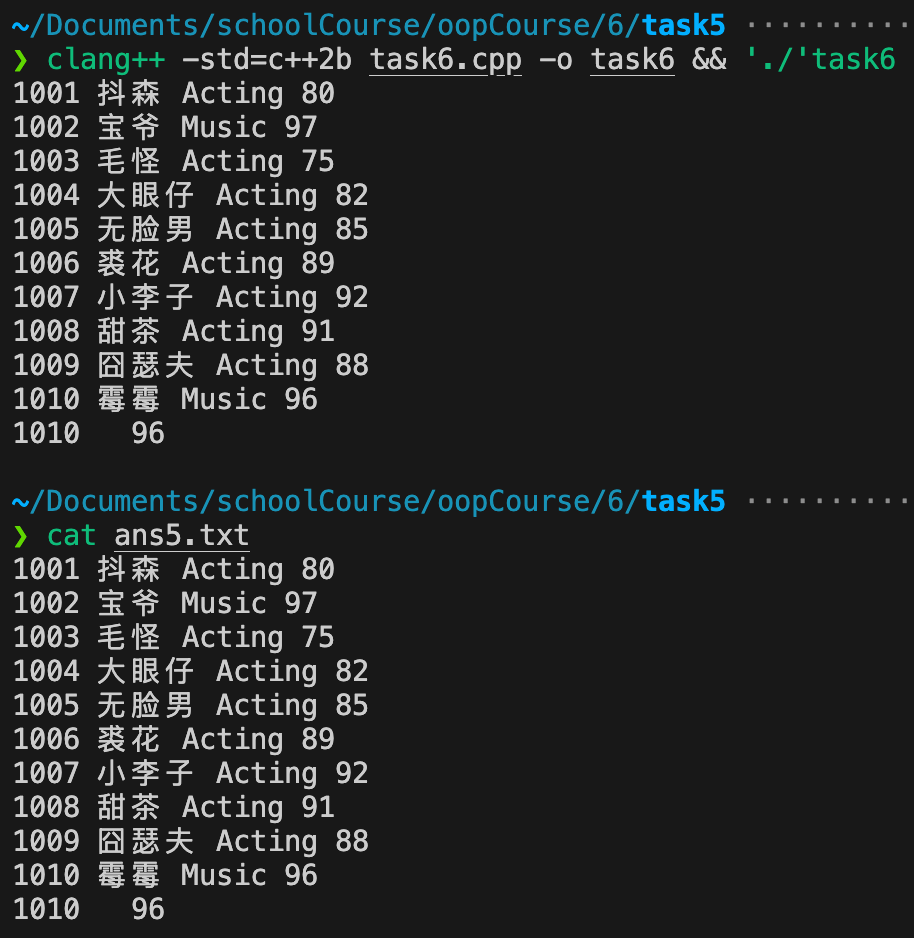