c++11 初始化列表 bind function 示例

1 // 111111111111.cpp: 定义控制台应用程序的入口点。 2 // 3 4 #include "stdafx.h" 5 #include <iostream> 6 #include <string> 7 #include <vector> 8 #include <map> 9 10 class FooVector { 11 std::vector<int> content_; 12 public: 13 FooVector(std::initializer_list<int> list) { 14 for (auto it = list.begin(); it != list.end(); it++) { 15 content_.push_back(*it); 16 } 17 } 18 }; 19 20 class FooMap { 21 std::map<int, int> content_; 22 using pair_t = std::map<int, int>::value_type; 23 public: 24 FooMap(std::initializer_list<pair_t> list) { 25 for (auto it = list.begin(); it != list.end(); ++it) { 26 content_.insert(*it); 27 } 28 } 29 }; 30 31 void test1() { 32 FooVector foo_1 = { 1,2,3,4,5 }; 33 FooMap foo_2 = { {1,2},{3,4},{5,6} }; 34 } 35 36 void func(std::initializer_list<int> l) { 37 for (auto it = l.begin(); it != l.end(); it++) { 38 std::cout << *it << std::endl; 39 } 40 } 41 42 void test2() 43 { 44 func({}); 45 func({ 1,2,3 }); 46 } 47 48 int main() 49 { 50 test1(); 51 test2(); 52 return 0; 53 }

1 // 111111111111.cpp: 定义控制台应用程序的入口点。 2 // 3 4 #include "stdafx.h" 5 #include <iostream> 6 #include <string> 7 #include <vector> 8 #include <map> 9 #include <functional> 10 11 void func(void) { 12 std::cout << __FUNCTION__ << std::endl; 13 } 14 15 class Foo { 16 public: 17 static int foo_func(int a) { 18 std::cout << __FUNCTION__ << "(" << a << ") ->:"; 19 return a; 20 } 21 }; 22 23 class Bar { 24 public: 25 int operator()(int a) { 26 std::cout << __FUNCTION__ << "(" << a << ") ->: "; 27 return a; 28 } 29 }; 30 31 void test1() 32 { 33 std::function<void(void)> fr1 = func; 34 fr1(); 35 36 std::function<int(int)> fr2 = Foo::foo_func; 37 std::cout << fr2(123) << std::endl; 38 39 Bar bar; 40 fr2 = bar; 41 std::cout << fr2(123) << std::endl; 42 } 43 //==================================================================== 44 class A { 45 std::function<void()> callback_; 46 public: 47 A(const std::function<void()>& f) :callback_(f) {} 48 void notify(void) { 49 callback_(); 50 } 51 }; 52 53 class Foo0 { 54 public: 55 void operator()(void) { 56 std::cout << __FUNCTION__ << std::endl; 57 } 58 }; 59 60 void test2() { 61 Foo0 foo; 62 A aa(foo); 63 aa.notify(); 64 } 65 //================================================================ 66 void call_when_even(int x, const std::function<void(int)>& f) { 67 if (!(x & 1)) { 68 f(x); 69 } 70 } 71 72 void output(int x) { 73 std::cout << x << " "; 74 } 75 76 void test3() { 77 for (int i = 0; i < 10; i++) { 78 call_when_even(i, output); 79 } 80 std::cout << std::endl; 81 } 82 //==================================================================== 83 void output_add_2(int x) { 84 std::cout << x + 2 << " "; 85 } 86 87 void test4() { 88 { 89 auto fr = std::bind(output, std::placeholders::_1); 90 for (int i = 0; i < 10; ++i) { 91 call_when_even(i, fr); 92 } 93 std::cout << std::endl; 94 } 95 { 96 auto fr = std::bind(output_add_2, std::placeholders::_1); 97 for (int i = 0; i < 10; ++i) { 98 call_when_even(i, fr); 99 } 100 std::cout << std::endl; 101 } 102 } 103 104 105 106 107 int main() 108 { 109 test1(); 110 test2(); 111 test3(); 112 test4(); 113 return 0; 114 }
作 者: itdef
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力

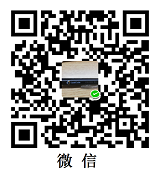