c++11 并发 条件变量 超时等待的代码练习
资料地址
http://en.cppreference.com/w/cpp/thread/condition_variable/wait_until
http://www.cnblogs.com/haippy/p/3252041.html

1 // asdasdwa.cpp: 定义控制台应用程序的入口点。 2 // 3 4 #include "stdafx.h" 5 6 #include <mutex> // std::mutex, std::unique_lock 7 #include <iostream> 8 #include <string> 9 #include <atomic> 10 #include <condition_variable> 11 #include <thread> 12 #include <chrono> 13 #include <vector> 14 15 #include <windows.h> 16 using namespace std::chrono_literals; 17 18 19 /* 20 作 者: itdef 欢迎转帖 请保持文本完整并注明出处 21 技术博客 http://www.cnblogs.com/itdef/ 22 技术交流群 群号码:324164944 23 欢迎c c++ windows驱动爱好者 服务器程序员沟通交流 24 部分老代码存放地点 http://www.oschina.net/code/list_by_user?id=614253 25 */ 26 27 std::condition_variable cv; 28 std::mutex mtx; 29 std::vector<std::string> messageVec; 30 31 std::atomic<int> i; 32 33 void SingalFunc() { 34 std::lock_guard<std::mutex> lock(mtx); 35 messageVec.push_back("1234567890test.hello world!"); 36 std::cout << "push" << std::endl; 37 cv.notify_one(); 38 } 39 40 41 void WaitTimeOutFunc1() 42 { 43 while(1) 44 { 45 std::unique_lock<std::mutex> lck(mtx); 46 auto now = std::chrono::system_clock::now(); 47 if (cv.wait_until(lck, now + 2s, []() {return !messageVec.empty(); })) { 48 std::cerr << "Thread " << " finished waiting. " << std::endl; 49 messageVec.pop_back(); 50 } 51 else { 52 std::cerr << "Thread " << " timed out." << std::endl; 53 return; 54 } 55 } 56 } 57 58 59 void WaitTimeOutFunc() 60 { 61 while(1) 62 { 63 std::unique_lock<std::mutex> lck(mtx); 64 std::cv_status status = std::cv_status::timeout; 65 auto now = std::chrono::system_clock::now(); 66 while (messageVec.empty()) { 67 status = cv.wait_until(lck, now + 1s); 68 if (status == std::cv_status::timeout) { 69 std::cout << i << " timeout" << std::endl; 70 return; 71 } 72 } 73 74 i.fetch_add(1); 75 std::cout << i << " no_timeout" << std::endl; 76 // std::cout << messageVec.back() << std::endl; 77 messageVec.pop_back(); 78 } 79 return; 80 } 81 82 83 int main() 84 { 85 std::thread t[2000]; 86 for (int i = 0; i < 5000; i++) { 87 SingalFunc(); 88 } 89 for (int i = 0; i <2000; i++) { 90 t[i] = std::thread(WaitTimeOutFunc); 91 } 92 93 //for (int i = 0; i < 5000; i++) { 94 // SingalFunc(); 95 //} 96 97 for (int i = 0; i < 2000; i++) { 98 t[i].join(); 99 } 100 101 return 1; 102 }
作 者: itdef
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力

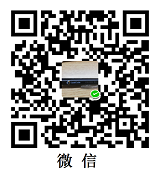