BFS 遍历例子
以Leetcode题目 200. 岛屿数量 为例 展示BFS代码
题目如下 https://leetcode-cn.com/problems/number-of-islands/
给你一个由 '1'(陆地)和 '0'(水)组成的的二维网格,请你计算网格中岛屿的数量。
岛屿总是被水包围,并且每座岛屿只能由水平方向和/或竖直方向上相邻的陆地连接形成。
此外,你可以假设该网格的四条边均被水包围。
示例 1:
输入:grid = [
["1","1","1","1","0"],
["1","1","0","1","0"],
["1","1","0","0","0"],
["0","0","0","0","0"]
]
输出:1
示例 2:
输入:grid = [
["1","1","0","0","0"],
["1","1","0","0","0"],
["0","0","1","0","0"],
["0","0","0","1","1"]
]
输出:3
BFS遍历
class Solution {
public:
int addX[4] = {1,0,0,-1};
int addY[4] = {0,1,-1,0};
void bfs(vector<vector<char>>& grid,int x,int y){
grid[x][y] = '0';
queue<pair<int,int>> q;
q.push({x,y});
while(!q.empty()){
int x = q.front().first;
int y = q.front().second;
q.pop();
for(int i = 0; i < 4;i++){
int newx = x+addX[i];
int newy = y+addY[i];
if(newx>=0 && newx < grid.size() && newy>=0 && newy<grid[0].size() && grid[newx][newy] == '1'){
grid[newx][newy] = '0';
q.push({newx,newy});
}
}
}
}
int numIslands(vector<vector<char>>& grid) {
int ans =0;
for(int i = 0; i < grid.size();i++){
for(int j = 0; j < grid[0].size();j++){
if(grid[i][j] == '1'){
bfs(grid,i,j); ans++;
}
}
}
return ans;
}
};
作 者: itdef
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力

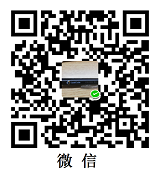