zoj1649 营救(Rescue) BFS
地址 https://vjudge.net/problem/ZOJ-1649
BFS 搜索 另开一个二维数组记录能达到该点的最小步数
代码

1 // 111111.cpp : 此文件包含 "main" 函数。程序执行将在此处开始并结束。 2 // 3 4 #include <iostream> 5 #include <vector> 6 #include <string> 7 #include <memory.h> 8 #include <queue> 9 #include <algorithm> 10 11 using namespace std; 12 13 const int MAXN = 200; 14 15 char g[MAXN][MAXN]; 16 int vis[MAXN][MAXN]; 17 queue<vector<int>> q; 18 19 int n, m; 20 21 int startx = -1; 22 int starty = -1; 23 24 int endx = -1; 25 int endy = -1; 26 27 int minWalk = 999999; 28 29 int addx[] = { 1,-1,0,0 }; 30 int addy[] = { 0,0,1,-1 }; 31 32 33 void bfs() 34 { 35 vector<int> start{ startx, starty ,0 }; 36 vis[startx][starty] = 0; 37 q.push(start); 38 39 while (!q.empty()) { 40 vector<int> ele = q.front(); 41 q.pop(); 42 43 for (int i = 0; i < 4; i++) { 44 int newx = ele[0] + addx[i]; 45 int newy = ele[1] + addy[i]; 46 int walk = ele[2]+1; 47 48 if (newx >= 0 && newx < n && newy >= 0 && newy < m && 49 (g[newx][newy] != '#')) { 50 if (g[newx][newy] == 'x') walk++; 51 if (walk < vis[newx][newy]) { 52 vis[newx][newy] = walk; 53 vector<int> input{newx,newy,walk}; 54 q.push(input); 55 } 56 if (g[newx][newy] == 'r') { 57 minWalk = min(minWalk, walk); 58 } 59 }//if (newx >= 0 && newx < n && newy >= 0 && newy < m) 60 } 61 } 62 63 } 64 65 66 67 68 69 int main() 70 { 71 while (~scanf("%d%d", &n, &m)) { 72 minWalk = 999999; 73 74 memset(g, 0, sizeof g); 75 memset(vis, 0x3f3f3f3f,sizeof vis); 76 startx = -1; endx = -1; 77 for (int i = 0; i < n; i++) { 78 scanf("%s", g[i]); 79 if (startx == -1) { 80 //寻找 起点 81 for (int j = 0; j < m; j++) { 82 if (g[i][j] == 'a') { 83 startx = i; starty = j; 84 } 85 } 86 } 87 88 if (endx == -1) { 89 //寻找 终点 90 for (int j = 0; j < m; j++) { 91 if (g[i][j] == 'r') { 92 endx = i; endy = j; 93 } 94 } 95 } 96 } 97 98 bfs(); 99 if (minWalk != 999999) 100 printf("%d\n", minWalk); 101 else 102 printf("Poor ANGEL has to stay in the prison all his life.\n"); 103 } 104 return 0; 105 }
作 者: itdef
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力

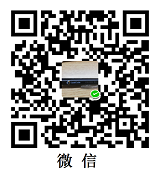