leetcode74
【题目】
二维矩阵里找目标值target
Write an efficient algorithm that searches for a value in an m x n
matrix. This matrix has the following properties:
- Integers in each row are sorted from left to right.
- The first integer of each row is greater than the last integer of the previous row.
Example 1:
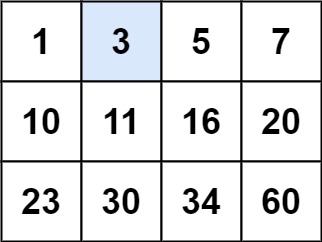
Input: matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 3 Output: true
Example 2:
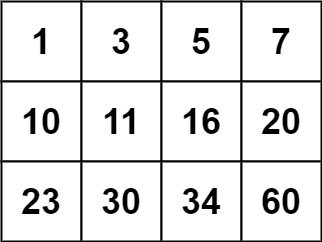
Input: matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 13 Output: false
【思路】
二分
每行单独二分查找,找到直接返回true,没找到最后返回false
【代码】
class Solution { public boolean searchMatrix(int[][] matrix, int target) { for(int i=0;i<matrix.length;i++){ int left=0;int right=matrix[0].length; while(left<right){ int mid=left+(right-left)/2; if(matrix[i][mid]==target){ return true; } else if(matrix[i][mid]<target){ left=mid+1; } else if(matrix[i][mid]>target){ right=mid; } } } return false; } }
leetcode240
【题目】
变式,同样是二维矩阵里找目标值target,但满足上到下,左到右均递增
Write an efficient algorithm that searches for a target
value in an m x n
integer matrix
. The matrix
has the following properties:
- Integers in each row are sorted in ascending from left to right.
- Integers in each column are sorted in ascending from top to bottom.
Example 1:
Input: matrix = [[1,4,7,11,15],[2,5,8,12,19],[3,6,9,16,22],[10,13,14,17,24],[18,21,23,26,30]], target = 5 Output: true
Example 2:
Input: matrix = [[1,4,7,11,15],[2,5,8,12,19],[3,6,9,16,22],[10,13,14,17,24],[18,21,23,26,30]], target = 20 Output: false
【思路】
可以直接用74的方法解,但时间较久
这里考虑到上下、左右递增,从左下/右上角出发遍历即可
每个点都有两种大/小了的选择
左上同大,右下同小,不行
B站https://www.bilibili.com/video/BV1Dy4y1u7RH 讲的很清楚
【代码】
public static boolean searchMatrix(int[][] matrix, int target) { if (matrix == null || matrix.length < 1 || matrix[0].length < 1) return false; int j = 0; int i= matrix.length - 1; while (j <= matrix[0].length - 1 && i>= 0) { if (target == matrix[i][j]) return true; else if (target < matrix[i][j]) i--; else if (target > matrix[i][j]) j++; } return false; }