sql注入环境搭建
pip install tornado
import tornado.ioloop
import tornado.web
import pymysql
class LoginHandler(tornado.web.RequestHandler):
def get(self, *args, **kwargs):
self.render("login.html")
def post(self, *args, **kwargs):
username = self.get_argument('username', None)
password = self.get_argument('password', None)
conn = pymysql.connect(host='192.168.2.11', port=3306, user='root', password='123456', db='bbs')
cur = conn.cursor()
tmp = "select username from userinfo where username = '%s' and password='%s'" % (username, password)
print(tmp)
effect_row = cur.execute(tmp)
res = cur.fetchone()
if res:
self.write("登录成功")
else:
self.write("登录失败")
conn.commit()
cur.close()
conn.close()
settings = {
}
application = tornado.web.Application([
(r'/login', LoginHandler)
], **settings)
if __name__ == '__main__':
print("http://127.0.0.1:8888/login")
application.listen(8888)
tornado.ioloop.IOLoop.instance().start()
create table userinfo(
username varchar(40),
password varchar(40)
);
insert into userinfo(username,password)values(
'maotai','123456'
);
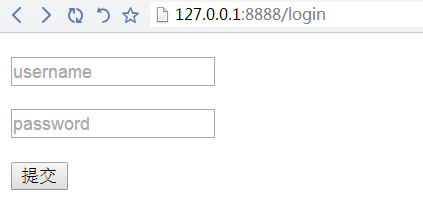
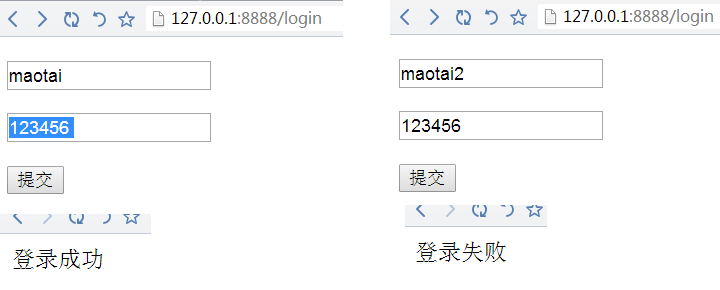
模拟sql注入
使用注释
maotai' -- f
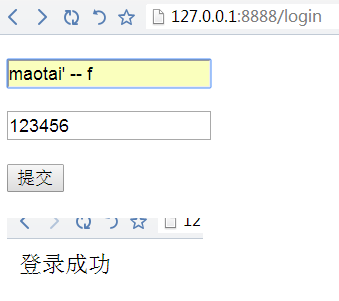
select username from userinfo where username = 'maotai' -- f' and password='123456'
使用or
aaron ' or 1=1 -- c
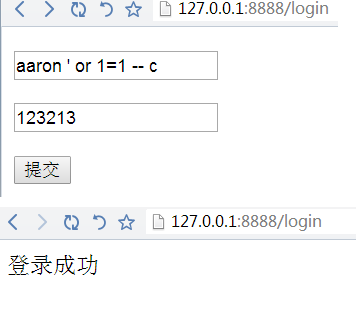
select username from userinfo where username = 'aaron ' or 1=1 -- c' and password='123213'
改进sql注入漏洞
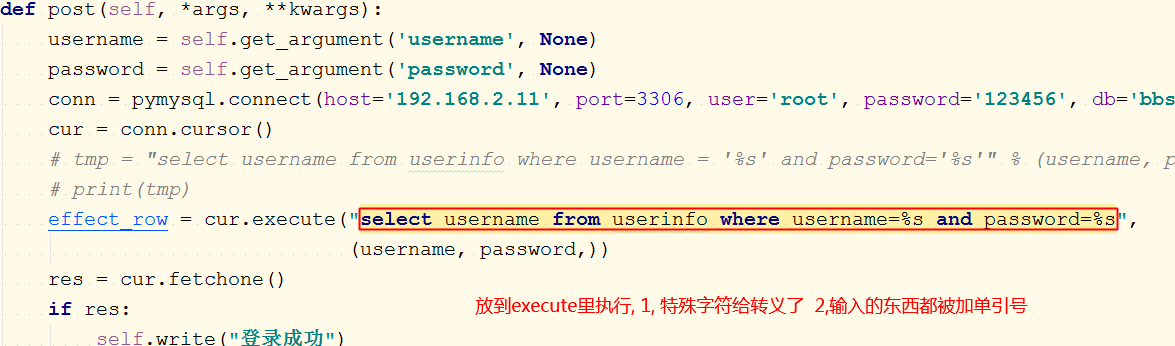
import tornado.ioloop
import tornado.web
import pymysql
class LoginHandler(tornado.web.RequestHandler):
def get(self, *args, **kwargs):
self.render("login.html")
def post(self, *args, **kwargs):
username = self.get_argument('username', None)
password = self.get_argument('password', None)
conn = pymysql.connect(host='192.168.2.11', port=3306, user='root', password='123456', db='bbs')
cur = conn.cursor()
# tmp = "select username from userinfo where username = '%s' and password='%s'" % (username, password)
# print(tmp)
effect_row = cur.execute("select username from userinfo where username=%s and password=%s",
(username, password,))
res = cur.fetchone()
if res:
self.write("登录成功")
else:
self.write("登录失败")
conn.commit()
cur.close()
conn.close()
settings = {
}
application = tornado.web.Application([
(r'/login', LoginHandler)
], **settings)
if __name__ == '__main__':
print("http://127.0.0.1:8888/login")
application.listen(8888)
tornado.ioloop.IOLoop.instance().start()