使用DESCryptoServiceProvider 类进行加密
定义访问数据加密标准 (DES) 算法的加密服务提供程序 (CSP) 版本的包装对象
从理论上说,加密并不能防止对手检索到加密的数据,但它确实增加了这样做的成本
上面了两句后都是MSDN上的定义,DES加密属于对称加密,即同一密钥既用于加密又用于解密。
下面是DESCryptoServiceProvider的继承结构。
使用DESCryptoServiceProvider 类进行加密时需要用到加密流(CryptoStream),CryptoStream定义将数据流链接到加密转换的流,下面是他的唯一的构造函数:
public CryptoStream(Stream stream,ICryptoTransform transform,CryptoStreamMode mode)
第一个参数(stream)表示要执行加密的流;
第二个参数(transform)表示实现了ICryptoTransform接口的一个实例,比如SymmetricAlgorithm.CreateEncryptor ()的返回值;
第三个参数(mode)表示CryptoStreamMode 枚举的一只,Read或Write。
下面的代码是根据MSDN上的修改的,其实很简单,原来使用的没有带参数的CreateEncryptor方法,表示用当前的 Key 属性和初始化向量 (IV) 创建对称解密器对象。而我们使用带参数的 CreateEncryptor方法,他的参数是两个byte型的数组,第一个表示用于对称算法的密钥,第二个表示用于对称算法的初始化向量。

using System;
using System.IO;
using System.Text;
using System.Security.Cryptography;
class CryptoMemoryStream
{
// Main method.
public static void Main()
{
//创建用于加密的密钥和初始化向量
byte[] _key = new byte[8] {0x12,0x1,0xFD,0x3A,0xad,0x4D,0xD4,0x2F};
byte[] _iv = new byte[8] {0x6c,0xfd,0x32,0xDa,0x91,0xac,0x61,0x54};
// Create a new DES key.
DESCryptoServiceProvider DESkey = new DESCryptoServiceProvider();
// Encrypt a string to a byte array.
byte[] buffer = Encrypt("This is some plaintext!", DESkey,_key,_iv);
// Decrypt the byte array back to a string.
string plaintext = Decrypt(buffer, DESkey, _key, _iv);
// Display the plaintext value to the console.
Console.WriteLine(plaintext);
Console.ReadKey();
}
// Encrypt the string.
public static byte[] Encrypt(string PlainText, SymmetricAlgorithm DESkey,byte[] key,byte[] iv)
{
// Create a memory stream.
MemoryStream ms = new MemoryStream();
// Create a CryptoStream using the memory stream and the
// CSP DES key.
CryptoStream encStream = new CryptoStream(ms, DESkey.CreateEncryptor(key,iv), CryptoStreamMode.Write);
// Create a StreamWriter to write a string
// to the stream.
StreamWriter sw = new StreamWriter(encStream);
// Write the plaintext to the stream.
sw.WriteLine(PlainText);
// Close the StreamWriter and CryptoStream.
sw.Close();
encStream.Close();
// Get an array of bytes that represents
// the memory stream.
byte[] buffer = ms.ToArray();
// Close the memory stream.
ms.Close();
// Return the encrypted byte array.
return buffer;
}
// Decrypt the byte array.
public static string Decrypt(byte[] CypherText, SymmetricAlgorithm DESkey, byte[] key, byte[] iv)
{
// Create a memory stream to the passed buffer.
MemoryStream ms = new MemoryStream(CypherText);
// Create a CryptoStream using the memory stream and the
// CSP DES key.
CryptoStream encStream = new CryptoStream(ms, DESkey.CreateDecryptor(key, iv), CryptoStreamMode.Read);
// Create a StreamReader for reading the stream.
StreamReader sr = new StreamReader(encStream);
// Read the stream as a string.
string val = sr.ReadLine();
// Close the streams.
sr.Close();
encStream.Close();
ms.Close();
return val;
}
}
从理论上说,加密并不能防止对手检索到加密的数据,但它确实增加了这样做的成本
上面了两句后都是MSDN上的定义,DES加密属于对称加密,即同一密钥既用于加密又用于解密。
下面是DESCryptoServiceProvider的继承结构。
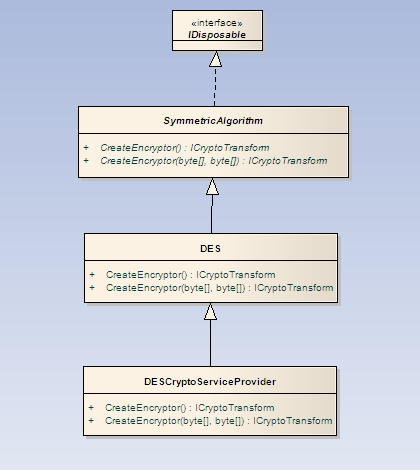
使用DESCryptoServiceProvider 类进行加密时需要用到加密流(CryptoStream),CryptoStream定义将数据流链接到加密转换的流,下面是他的唯一的构造函数:
public CryptoStream(Stream stream,ICryptoTransform transform,CryptoStreamMode mode)
第一个参数(stream)表示要执行加密的流;
第二个参数(transform)表示实现了ICryptoTransform接口的一个实例,比如SymmetricAlgorithm.CreateEncryptor ()的返回值;
第三个参数(mode)表示CryptoStreamMode 枚举的一只,Read或Write。
下面的代码是根据MSDN上的修改的,其实很简单,原来使用的没有带参数的CreateEncryptor方法,表示用当前的 Key 属性和初始化向量 (IV) 创建对称解密器对象。而我们使用带参数的 CreateEncryptor方法,他的参数是两个byte型的数组,第一个表示用于对称算法的密钥,第二个表示用于对称算法的初始化向量。

using System;
using System.IO;
using System.Text;
using System.Security.Cryptography;
class CryptoMemoryStream
{
// Main method.
public static void Main()
{
//创建用于加密的密钥和初始化向量
byte[] _key = new byte[8] {0x12,0x1,0xFD,0x3A,0xad,0x4D,0xD4,0x2F};
byte[] _iv = new byte[8] {0x6c,0xfd,0x32,0xDa,0x91,0xac,0x61,0x54};
// Create a new DES key.
DESCryptoServiceProvider DESkey = new DESCryptoServiceProvider();
// Encrypt a string to a byte array.
byte[] buffer = Encrypt("This is some plaintext!", DESkey,_key,_iv);
// Decrypt the byte array back to a string.
string plaintext = Decrypt(buffer, DESkey, _key, _iv);
// Display the plaintext value to the console.
Console.WriteLine(plaintext);
Console.ReadKey();
}
// Encrypt the string.
public static byte[] Encrypt(string PlainText, SymmetricAlgorithm DESkey,byte[] key,byte[] iv)
{
// Create a memory stream.
MemoryStream ms = new MemoryStream();
// Create a CryptoStream using the memory stream and the
// CSP DES key.
CryptoStream encStream = new CryptoStream(ms, DESkey.CreateEncryptor(key,iv), CryptoStreamMode.Write);
// Create a StreamWriter to write a string
// to the stream.
StreamWriter sw = new StreamWriter(encStream);
// Write the plaintext to the stream.
sw.WriteLine(PlainText);
// Close the StreamWriter and CryptoStream.
sw.Close();
encStream.Close();
// Get an array of bytes that represents
// the memory stream.
byte[] buffer = ms.ToArray();
// Close the memory stream.
ms.Close();
// Return the encrypted byte array.
return buffer;
}
// Decrypt the byte array.
public static string Decrypt(byte[] CypherText, SymmetricAlgorithm DESkey, byte[] key, byte[] iv)
{
// Create a memory stream to the passed buffer.
MemoryStream ms = new MemoryStream(CypherText);
// Create a CryptoStream using the memory stream and the
// CSP DES key.
CryptoStream encStream = new CryptoStream(ms, DESkey.CreateDecryptor(key, iv), CryptoStreamMode.Read);
// Create a StreamReader for reading the stream.
StreamReader sr = new StreamReader(encStream);
// Read the stream as a string.
string val = sr.ReadLine();
// Close the streams.
sr.Close();
encStream.Close();
ms.Close();
return val;
}
}