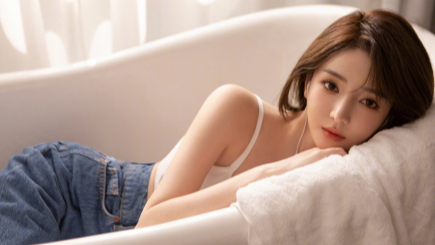
知识点:多边形向量叉乘;matplotlib绘制多边形。
判断polygon方向
根据polygon点坐标,判断该多边形绘制逻辑,顺时针 or 逆时针。
多边形坐标
# eg:
points = [(2.7388851109150023, 3.3239332759385984), (-17.760919951693104, 2.222307409516218), (-17.314910496242728, -2.516984005337407), (-12.852000304853743, -2.181040678562682), (-12.593791095515666, -5.8155342680533355), (-4.857700723763328, -5.360086611284489), (-4.72572589218098, -7.919382774996063), (-17.0160820270518, -8.620830962890897), (-17.867133295518986, -0.9456760895428342), (-22.258963842225317, -1.357221390151473), (-22.515649371106914, 1.792758532824255), (-50.111561102018456, 1.020935065299705), (-49.91522402991826, -6.579202348469052), (-39.25591973076352, -6.777074602691823), (-39.3470211782855, -14.14753079960294), (-4.451428730046921, -13.176437404289821), (19.38029991918383, -11.258640459629042), (18.678629198011325, -3.52091868564249), (40.24382063819184, -1.591592108027843), (40.610108155470044, -5.677529622149496), (53.63278351803943, -4.419178715222456), (54.019049536995844, -8.87311208850915), (87.09318512464871, -5.0339697036894435), (86.048475587818, 1.3394651586721347), (70.41625034816182, -1.3933549733832722), (70.05627257055237, 0.32943075873207184), (77.90519487909148, 1.835562744869648), (77.8005383315741, 2.6041726269511902), (83.7250982659767, 3.4304970071188574), (87.12772536434038, 4.021284170346685), (85.85772371362678, 11.705521972785498), (69.0134084370372, 9.44435529400685), (69.37946910976412, 6.777191877663952), (64.65690016784653, 6.192228087150736), (64.38067230336098, 8.457858764430892), (3.679804302962524, 3.3268451469218845)]
1. 通过计算叉乘判断
def calculate_polygon_area(points_list):
n = len(points_list)
# 点的个数<3 为line,非polygon
if n < 3:
return 0.0
area = 0
for i in range(n):
x = points_list[i][0]
y = points_list[i][1]
# (x1*y2 - y1*x2)
area += x * points_list[(i + 1) % n][1] - y * points_list[(i + 1) % n][0]
return area * -0.5
2. matplotlib通过画点判断顺逆时针
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
result = [(i[0], i[1]) for i in points]
xx = []
yy = []
fig, ax = plt.subplots()
line, = plt.plot([], [], '.-')
def init():
# 初始化函数用于绘制一块干净的画布,为后续绘图做准备
# 初始函数,设置绘图范围
ax.set_xlim(-100, 100)
ax.set_ylim(-100, 100)
return line
def update(step):
# 通过帧数来不断更新新的数值
ri = result[step]
# print(ri)
xx.append(ri[0])
yy.append(ri[1])
line.set_data(xx, yy)
return line
ani = FuncAnimation(fig, update, frames=[i for i in range(len(result))], init_func=init, interval=200, repeat=False)
plt.show()