POJ 2549
Sumsets
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 8235 | Accepted: 2260 |
Description
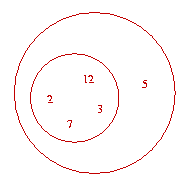
Input
Several S, each consisting of a line containing an integer 1 <= n <= 1000 indicating the number of elements in S, followed by the elements of S, one per line. Each element of S is a distinct integer between -536870912 and +536870911 inclusive. The last line of input contains 0.
Output
For each S, a single line containing d, or a single line containing "no solution".
Sample Input
5 2 3 5 7 12 5 2 16 64 256 1024 0
Sample Output
12 no solution
Source
分成a + b 和 d - c两个集合
x = d - c 保存a + b 的所有值,二分查找x并判断合理性

1 #include <iostream> 2 #include <cstdio> 3 #include <cstring> 4 #include <algorithm> 5 6 using namespace std; 7 8 struct node { int v,a,b; }; 9 const int MAX = 1005; 10 int N,len = 0; 11 int ele[MAX]; 12 node y[MAX * MAX]; 13 14 bool cmp(node a,node b) { return a.v < b.v; } 15 16 bool solve() { 17 for(int i = N - 1; i >= 0; --i) { 18 for(int j = N - 1; j >= 0; --j) { 19 if(i == j) continue; 20 int d = ele[i] - ele[j]; 21 // printf("ele = %d d = %d\n",ele[i],d); 22 int l = 0,r = len - 1; 23 while(l < r) { 24 int mid = (l + r + 1) >> 1; 25 if(y[mid].v > d) r = mid - 1; 26 else l = mid; 27 } 28 if(y[l].v == d) { 29 for(int k = l; k < len; ++k) { 30 if(y[k].v == d && y[k].a != ele[j] && y[k].b != ele[j] 31 && y[k].a != ele[i] && y[k].b != ele[i]) { 32 printf("%d\n",ele[i]); 33 return true; 34 } 35 } 36 for(int k = l; k >= 0; --k) { 37 if(y[k].v == d && y[k].a != ele[j] && y[k].b != ele[j] 38 && y[k].a != ele[i] && y[k].b != ele[i]) { 39 printf("%d\n",ele[i]); 40 return true; 41 } 42 43 } 44 45 } 46 47 } 48 } 49 50 return false; 51 52 } 53 54 int main() 55 { 56 // freopen("sw.in","r",stdin); 57 58 while(~scanf("%d",&N) && N ) { 59 for(int i = 0; i < N; ++i) scanf("%d",&ele[i]); 60 61 sort(ele,ele + N); 62 N = unique(ele,ele + N) - ele; 63 64 65 len = 0; 66 for(int i = 0; i < N; ++i) { 67 for(int j = i + 1; j < N; ++j) { 68 y[len].v = ele[i] + ele[j]; 69 y[len].a = ele[i]; 70 y[len++].b = ele[j]; 71 } 72 } 73 74 sort(y,y + len,cmp); 75 76 if(!solve()) printf("no solution\n"); 77 } 78 return 0; 79 }