Python基础(二)-基本数据类型(列表,元组,字典,集合及格式化输出)
一、列表
1.1、列表的格式
列表中可以嵌套任何类型,中括号括起来,”,”分割每个元素, 列表中的元素可以是 数字,字符串,列表,布尔值..所有的都能放进去
li = [1, 12, 9, "age", ["aaa", ["19", 10], "bbb"], "ccc", False]
li = [11, 22, 33, 22, 44]
1.2、列表的方法
源码:
def append(self, p_object): # real signature unknown; restored from __doc__ """ L.append(object) -> None -- append object to end """ pass def clear(self): # real signature unknown; restored from __doc__ """ L.clear() -> None -- remove all items from L """ pass def copy(self): # real signature unknown; restored from __doc__ """ L.copy() -> list -- a shallow copy of L """ return [] def count(self, value): # real signature unknown; restored from __doc__ """ L.count(value) -> integer -- return number of occurrences of value """ return 0 def extend(self, iterable): # real signature unknown; restored from __doc__ """ L.extend(iterable) -> None -- extend list by appending elements from the iterable """ pass def index(self, value, start=None, stop=None): # real signature unknown; restored from __doc__ """ L.index(value, [start, [stop]]) -> integer -- return first index of value. Raises ValueError if the value is not present. """ return 0 def insert(self, index, p_object): # real signature unknown; restored from __doc__ """ L.insert(index, object) -- insert object before index """ pass def pop(self, index=None): # real signature unknown; restored from __doc__ """ L.pop([index]) -> item -- remove and return item at index (default last). Raises IndexError if list is empty or index is out of range. """ pass def remove(self, value): # real signature unknown; restored from __doc__ """ L.remove(value) -> None -- remove first occurrence of value. Raises ValueError if the value is not present. """ pass def reverse(self): # real signature unknown; restored from __doc__ """ L.reverse() -- reverse *IN PLACE* """ pass def sort(self, key=None, reverse=False): # real signature unknown; restored from __doc__ """ L.sort(key=None, reverse=False) -> None -- stable sort *IN PLACE* """ pass
1)append() ==>原来值最后追加,参数必须是可迭代对象
li = [1, 2, 3, 4] li.append(5) li.append("abc") li.append([1234, 2323]) print(li) #[1, 2, 3, 4, 5, 'abc', [1234, 2323]]
2)clear() ==>清空列表
li = [1, 2, 3, 4] li.clear() print(li) #[]
3)copy() ==>浅拷贝
li = [1, 2, 3, 4] v = li.copy() print(v) #[1, 2, 3, 4]
4)count() ==>计算元素出现的次数
li = [1, 2, 3, 4, 1] v = li.count(1) print(v) #2
5)extend() ==>扩展原列表,参数:可迭代对象
#使用append li = [11, 22, 33, 22, 44] li.append(["aa","bb"]) print(li) #[11, 22, 33, 22, 44, ['aa', 'bb']] #嵌套列表 #使用extend li = [11, 22, 33, 22, 44] li.extend([55,"aa"]) #[11, 22, 33, 22, 44, 55, 'aa'] #一个列表 print(li)
6)index() ==>根据值获取当前值索引位置(左边优先)
li = [11, 22, 33, 22, 44] v= li.index(22) print(v) #1
7)insert() ==>在指定索引位置插入元素
li = [11, 22, 33, 22, 44] li.insert(0,99) print(li) #[99, 11, 22, 33, 22, 44]
8)pop() ==>删除某个值(1.指定索引;2. 默认最后一个),并获取删除的值
li = [11, 22, 33, 22, 44] v = li.pop() print(li) #[11, 22, 33, 22] print(v) #44 li = [11, 22, 33, 22, 44] v = li.pop(1) print(li) #[11, 33, 22, 44] print(v) #22
9)remove() ==>删除列表中的指定值,左边优先
li = [11, 22, 33, 22, 44] li.remove(22) print(li) #[11, 33, 22, 44] #列表删除方法: #pop remove del li[0] del li[7:9] clear
10)sort() ==>列表的排序
li = [11,44, 22, 33, 22] li.sort() #[11, 22, 22, 33, 44] li.sort(reverse=True) #[44, 33, 22, 22, 11] print(li)
1.3、列表的取值
1)索引取值
li = [11,44, 22, 33, 22] print(li[3]) #33
2)切片,切片结果也是列表
li = [11,44, 22, 33, 22] print(li[2:-1]) #[22, 33]
3)while或for循环
for item in li: print(item)
4)列表值修改
li[1] = 120 li[1] = [11,22,33,44] li[1:3] = [120,90] #删除值 del li[1] del li[2:6]
5)列表in操作
li = [1, 12, 9, "age", ["aaa", ["19", 10], "bbb"], "ccc", True] v1 = "aaa" in li print(v1) #false v2 = "age" in li print(v2) #true
6)列表转换
#字符串转换为列表,内部使用for循环 s = "abc" new_li = list(s) print(new_li) #['a', 'b', 'c'] #列表转换成字符串 # 需要自己写for循环一个一个处理: 既有数字又有字符串 li = [11,22,33,44] r = str(li) print(r) #[11, 22, 33, 44] li = [11,22,33,44] s = "" for i in li: s = s + str(i) print(s) #11223344 #使用join li = ["123","abc"] v = "".join(li) print(v) #123abc
二、元组tuple
2.1、元组的格式
一级元素不可被修改,不能被增加或者删除,tu = (111,"a",(11,22),[(33,44)],True,33,44,)中列表可以修改
tu = (11,22,33,44)
tu = (111,"a",(11,22),[(33,44)],True,33,44,) #一般写元组的时候,推荐在最后加入 ,
2.2、元组的方法
源码:
def count(self, value): # real signature unknown; restored from __doc__ """ T.count(value) -> integer -- return number of occurrences of value """ return 0 def index(self, value, start=None, stop=None): # real signature unknown; restored from __doc__ """ T.index(value, [start, [stop]]) -> integer -- return first index of value. Raises ValueError if the value is not present. """ return 0
2.3、元组的取值
1)索引
v = tu[0] v = tu[0:2] #切片 #可以被for循环,可迭代对象 for item in tu: print(item)
2)元组转换
s = "asdfasdf0" li = ["asdf","asdfasdf"] tu = ("asdf","asdf") v = tuple(s) #字符串转换为元组 print(v) v = tuple(li) #列表转换为元组 print(v) v = list(tu) #元组转换为元组 print(v) v = "_".join(tu) #通过join方式转换为字符串 print(v) li = ["asdf","asdfasdf"] li.extend((11,22,33,)) print(li) #['asdf', 'asdfasdf', 11, 22, 33]
三、字典
3.1、字典的格式
字典的value可以是任何值,字典是无须的
dic = { "k1": 'v1', "k2": 'v2' }
3.2、字典的方法
源码:
def clear(self): # real signature unknown; restored from __doc__ """ D.clear() -> None. Remove all items from D. """ pass def copy(self): # real signature unknown; restored from __doc__ """ D.copy() -> a shallow copy of D """ pass @staticmethod # known case def fromkeys(*args, **kwargs): # real signature unknown """ Returns a new dict with keys from iterable and values equal to value. """ pass def get(self, k, d=None): # real signature unknown; restored from __doc__ """ D.get(k[,d]) -> D[k] if k in D, else d. d defaults to None. """ pass def items(self): # real signature unknown; restored from __doc__ """ D.items() -> a set-like object providing a view on D's items """ pass def keys(self): # real signature unknown; restored from __doc__ """ D.keys() -> a set-like object providing a view on D's keys """ pass def pop(self, k, d=None): # real signature unknown; restored from __doc__ """ D.pop(k[,d]) -> v, remove specified key and return the corresponding value. If key is not found, d is returned if given, otherwise KeyError is raised """ pass def popitem(self): # real signature unknown; restored from __doc__ """ D.popitem() -> (k, v), remove and return some (key, value) pair as a 2-tuple; but raise KeyError if D is empty. """ pass def setdefault(self, k, d=None): # real signature unknown; restored from __doc__ """ D.setdefault(k[,d]) -> D.get(k,d), also set D[k]=d if k not in D """ pass def update(self, E=None, **F): # known special case of dict.update """ D.update([E, ]**F) -> None. Update D from dict/iterable E and F. If E is present and has a .keys() method, then does: for k in E: D[k] = E[k] If E is present and lacks a .keys() method, then does: for k, v in E: D[k] = v In either case, this is followed by: for k in F: D[k] = F[k] """ pass def values(self): # real signature unknown; restored from __doc__ """ D.values() -> an object providing a view on D's values """ pass
1)fromkeys() ==>根据序列,创建字典,并指定统一的值
# v = dict.fromkeys(["k1",123,"999"],123) # print(v) #{123: 123, 'k1': 123, '999': 123} v = dict.fromkeys(["k1",123,"999"]) print(v) #{123: None, '999': None, 'k1': None}
2)get() ==>根据Key获取值,key不存在时,可以指定默认值(None)
dic = { "k1": 'v1', "k2": 'v2' } # v = dic['k11111'] #报错KeyError: 'k11111' # print(v) v = dic.get('k3',111111) print(v) #111111
3)pop(),popitem() ==>删除并获取值
dic = { "k1": 'v1', "k2": 'v2' } # v = dic.pop('k1',90) # print(dic,v) #{'k2': 'v2'} v1 k,v = dic.popitem() print(dic,k,v) #{'k1': 'v1'} k2 v2
4)setdefault() ==>设置值,已存在,不设置,获取当前key对应的值.不存在,设置,获取当前key对应的值
dic = { "k1": 'v1', "k2": 'v2' } v = dic.setdefault('k1111','123') print(dic,v) #{'k1111': '123', 'k2': 'v2', 'k1': 'v1'} 123
5)update() ==>更新
dic = { "k1": 'v1', "k2": 'v2' } # dic.update({'k1': '111111','k3': 123}) #{'k1': '111111', 'k2': 'v2', 'k3': 123} # print(dic) dic.update(k1=123, k3=345, k5="asdf") #{'k3': 345, 'k2': 'v2', 'k1': 123, 'k5': 'asdf'} print(dic)
3.3、字典的取值
1)根据索引取值
info = { "k1": 18, 2: True, "k3": [ 11, [], (), 22, 33, { 'kk1': 'vv1', 'kk2': 'vv2', 'kk3': (11,22), } ], "k4": (11,22,33,44) } # v = info['k1'] # print(v) # v = info[2] # print(v) v = info['k3'][5]['kk3'][0] print(v)
2)字典删除
del info['k1'] del info['k3'][5]['kk1']
3)字典for循环
for item in info: print(item) for item in info.keys(): print(item) for item in info.values(): print(item) for item in info.keys(): print(item,info[item]) for k,v in info.items(): print(k,v)
四、集合
4.1、集合的格式
s={1,2,3,4,5,6}
集合:可以包含多个元素,用逗号分割,
集合的元素遵循三个原则:
- 每个元素必须是不可变类型(可hash,可作为字典的key)
- 没有重复的元素
- 无序
集合的定义:
# s=set('hello') # print(s) #{'l', 'e', 'o', 'h'} s=set(['aa','bb','sb']) print(s) #{'bb', 'aa', 'sb'} # s={1,2,3,4,5,6}
4.2、集合的方法
源码:
def add(self, *args, **kwargs): # real signature unknown """ Add an element to a set. This has no effect if the element is already present. """ pass def clear(self, *args, **kwargs): # real signature unknown """ Remove all elements from this set. """ pass def copy(self, *args, **kwargs): # real signature unknown """ Return a shallow copy of a set. """ pass def difference(self, *args, **kwargs): # real signature unknown """ Return the difference of two or more sets as a new set. (i.e. all elements that are in this set but not the others.) """ pass def difference_update(self, *args, **kwargs): # real signature unknown """ Remove all elements of another set from this set. """ pass def discard(self, *args, **kwargs): # real signature unknown """ Remove an element from a set if it is a member. If the element is not a member, do nothing. """ pass def intersection(self, *args, **kwargs): # real signature unknown """ Return the intersection of two sets as a new set. (i.e. all elements that are in both sets.) """ pass def intersection_update(self, *args, **kwargs): # real signature unknown """ Update a set with the intersection of itself and another. """ pass def isdisjoint(self, *args, **kwargs): # real signature unknown """ Return True if two sets have a null intersection. """ pass def issubset(self, *args, **kwargs): # real signature unknown """ Report whether another set contains this set. """ pass def issuperset(self, *args, **kwargs): # real signature unknown """ Report whether this set contains another set. """ pass def pop(self, *args, **kwargs): # real signature unknown """ Remove and return an arbitrary set element. Raises KeyError if the set is empty. """ pass def remove(self, *args, **kwargs): # real signature unknown """ Remove an element from a set; it must be a member. If the element is not a member, raise a KeyError. """ pass def symmetric_difference(self, *args, **kwargs): # real signature unknown """ Return the symmetric difference of two sets as a new set. (i.e. all elements that are in exactly one of the sets.) """ pass def symmetric_difference_update(self, *args, **kwargs): # real signature unknown """ Update a set with the symmetric difference of itself and another. """ pass def union(self, *args, **kwargs): # real signature unknown """ Return the union of sets as a new set. (i.e. all elements that are in either set.) """ pass def update(self, *args, **kwargs): # real signature unknown """ Update a set with the union of itself and others. """ pass
1)add() ==>添加
s={1,2,3,4,5,6} s.add('s') s.add('3') s.add(3) print(s) #{1, 2, 3, 4, 5, 6, '3', 's'}
2)clear() ==>清空集合
3)copy() ==>拷贝集合
4)pop() ==>随即删除
s={'sb',1,2,3,4,5,6} #随机删 s.pop() print(s) #结果可能是{2, 3, 4, 5, 6, 'sb'}
5)remove(),discard() ==>指定删除
s={'abc',1,2,3,4,5,6} # s.remove('abc') #{1, 2, 3, 4, 5, 6} # s.remove('hell') #删除元素不存在会报错 s.discard('abc')#{2, 3, 4, 5, 1, 6},删除元素不存在不会报错 print(s)
6)交集,并集,差集
python_l=['lcg','szw','zjw','lcg']
python_l=['lcg','szw','zjw','lcg'] linux_l=['lcg','szw','sb'] p_s=set(python_l) l_s=set(linux_l) #求交集 print(p_s.intersection(l_s)) #{'lcg', 'szw'} print(p_s&l_s) #{'lcg', 'szw'} # #求并集 print(p_s.union(l_s)) #{'lcg', 'szw', 'zjw', 'sb'} print(p_s|l_s) #{'lcg', 'szw', 'zjw', 'sb'} # #差集 print('差集',p_s-l_s) #差集 {'zjw'} print(p_s.difference(l_s)) #{'zjw'} print('差集',l_s-p_s) #差集 {'sb'} print(l_s.difference(p_s)) #{'sb'} #交叉补集 print('交叉补集',p_s.symmetric_difference(l_s)) #交叉补集 {'zjw', 'sb'} print('交叉补集',p_s^l_s) #交叉补集 {'zjw', 'sb'}
7)子集,父级
s1={1,2} s2={1,2,3} print(s1.issubset(s2))#s1 是s2 的子集 print(s2.issubset(s1))#False print(s2.issuperset(s1))#s1 是s2 的父集
8)update
s1={1,2} s2={1,2,3} s1.update(s2) print(s1) #{1, 2, 3}
9)不可变集合
s=frozenset('hello') print(s) #frozenset({'o', 'l', 'e', 'h'})
五、字符串格式化输出
5.1、百分号方式
tpl = "i am %s" % "abc" tp1 = "i am %s age %d" % ("abc", 18) tp1 = "i am %(name)s age %(age)d" % {"name": "abc", "age": 18} tp1 = "percent %.2f" % 99.97623 #percent 99.98 tp1 = "i am %(pp).2f" % {"pp": 123.425556, } #i am 123.43 tp1 = "i am %.2f %%" % {"pp": 123.425556, } tpl = "i am %(name)s age %(age)d" % {"name": "abc", "age": 18} print('root','x','0','0',sep=':') #root:x:0:0
5.2、format方式
tpl = "i am {}, age {}, {}".format("seven", 18, 'alex') tpl = "i am {}, age {}, {}".format(*["seven", 18, 'alex']) #集合需要* tpl = "i am {0}, age {1}, really {0}".format("seven", 18) tpl = "i am {0}, age {1}, really {0}".format(*["seven", 18]) tpl = "i am {name}, age {age}, really {name}".format(name="seven", age=18) tpl = "i am {name}, age {age}, really {name}".format(**{"name": "seven", "age": 18}) #字典需要** tpl = "i am {0[0]}, age {0[1]}, really {0[2]}".format([1, 2, 3], [11, 22, 33]) tpl = "i am {:s}, age {:d}, money {:f}".format("seven", 18, 88888.1) tpl = "i am {:s}, age {:d}".format(*["seven", 18]) tpl = "i am {name:s}, age {age:d}".format(name="seven", age=18) tpl = "i am {name:s}, age {age:d}".format(**{"name": "seven", "age": 18}) tpl = "numbers: {:b},{:o},{:d},{:x},{:X}, {:%}".format(15, 15, 15, 15, 15, 15.87623, 2) tpl = "numbers: {:b},{:o},{:d},{:x},{:X}, {:%}".format(15, 15, 15, 15, 15, 15.87623, 2) tpl = "numbers: {0:b},{0:o},{0:d},{0:x},{0:X}, {0:%}".format(15) tpl = "numbers: {num:b},{num:o},{num:d},{num:x},{num:X}, {num:%}".format(num=15)
5.3、f方式
s = f"今天下雨了{input('>>>')}" print(s) s11 = "学习进度为:%s" print(s11 % ("不错")) #学习进度为:不错 s = f"{1}{2}{3}" print(s) #123
-------------------------------------------
个性签名:独学而无友,则孤陋而寡闻。做一个灵魂有趣的人!
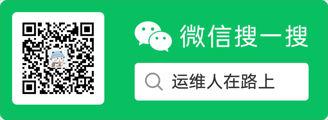