Python基础(一)-变量,运算符,流程语句,基本类型(数字,字符串)
一、python变量定义
1.1、变量
1)变量字符中只能存在有字母,数字,下划线
2)不能以数字开头
3)不能是关键字:
'and', 'as', 'assert', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'exec', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'not', 'or', 'pass', 'print', 'raise', 'return', 'try', 'while', 'with', 'yield'
4)不要和python内置的东西重复
5)最好见名知意,单词一直可以下划线分割,如user_id
二、运算符
2.1、算数运算
2.2、比较运算
2.3、赋值运算
2.4、逻辑运算
2.5、成员运算
三、基本语句
3.1、条件语句
#if基本语句 if 条件: 内部代码块1 else: 内部代码块2 print('....') #if支持嵌套 if 条件1: if 条件2: 代码块1 else: 代码块2 else: 代码块3 #if elif语句 if 条件1: 代码块1 elif 条件2: 代码块2 elif 条件3: 代码块3 else: 代码块4 #可以在代码块中使用pass,表示不执行操作没直接跳过
3.2、while条件语句
while 条件: 代码块
continue :终止当前循环,开始下一次循环
break:终止所有循环
""" 给用户三次猜测机会,如果三次之内猜测对了, 则显示猜测正确,退出循环,如果三次之内没有猜测正确, 则自动退出循环,并显示‘太笨了你....’。 """ count = 0 age = 66 while count < 3: num = int(input("请输入年龄:")) if num > age: print("大了") elif num < age: print("小了") else: print("对了") break count += 1 else: print("太笨了你....")
3.3、for循环
#基本格式,支持break,continue for 变量名 in 字符串: 变量名 #使用while循环打印字符串元素 test = "abcdef" index = 0 while index < len(test): v = test[index] print(v) index += 1 print('=======') #使用for循环打印字符串元素 test = "abcdef" for item in test: print(item)
3.4、基本语句练习
1)使用while循环输入 1 2 3 4 5 6 8 9 10
n = 1 while n < 11: if n == 7: pass else: print(n) n = n + 1 print('----end----')
2)求1-100的所有数的和
n = 1 s = 0 while n < 101: s = s + n n = n + 1 print(s)
3)输出 1-100 内的所有奇数
n = 1 while n < 101: temp = n % 2 if temp == 0: pass else: print(n) n = n + 1 print('----end----')
4)求1-2+3-4+5 ... 99的所有数的和
n = 1 s = 0 while n < 100: temp = n % 2 if temp == 0: s = s - n else: s = s + n n = n + 1 print(s)
5)用户登录(三次机会)
count = 0 while count < 3: user = input('>>>') pwd = input('>>>') if user == 'root' and pwd == '123': print('欢迎登陆') print('..........') break else: print('用户名或者密码错误') count = count + 1
四、python基本数据类型
4.1、数字类型
4.1.1、数字运算
a1 = 10 a2 = 20 a3 = a1 + a2 a3 = a1 - a2 a3 = a1 * a2 a3 = 100 / 10 a3 = 4**4 a3 = 39 % 8 #获取39除以8得到的余数 a3 = 39 // 8 #获取商
4.1.2、数字类型方法
1)int ==>将字符串转换为数字
a = "123" print(type(a),a) b = int(a) print(type(b),b) num = "0011" v = int(num, base=16) #将num转换为16进制 print(v) #17
1)bit_lenght ==>当前数字的二进制,至少用多少位表示
age = 3 r = age.bit_length() print(r) #11 ===>2
4.2、字符串类型
4.2.1、字符串运算
#加法运算:字符串的连接 n1 = "aaa" n2 = "bbb" n4 = "ccc" n3 = n1 + n2 + n4 #"aaabbbccc" #乘法运算:重复字符串次数 n1 = "aaa" n3 = n1 * 2 #aaaaaa
4.2.2、字符串类型方法
1)capitalize() ==>首字母大写
test = "aBc" v = test.capitalize() print(v) #Abc
2)casefold() ==>所有变小写,casefold更牛,很多未知相应变小写
test = "aBc" v = test.casefold() print(v) #abc
3)lower() ==>所有变小写
test = "aBc" v = test.lower() print(v) #abc
4)center(self, width, fillchar=None) ==>设置宽度,两边用空白或一个字符填充
test = "aBc" v = test.center(20,"*") print(v) #********aBc*********
5) count(self, sub, start=None, end=None) ==>去字符串中寻找,寻找子序列的出现次数
test = "aLexalexr" v = test.count('ex') print(v) #2 test = "aLexalexr" v = test.count('ex',5,8) print(v) #1
6)endswith('str'),startswith('str') ==>以什么结尾,以什么开始
test = "abc" v = test.endswith('c') v = test.startswith('a') print(v)
7)expandtabs() ==>扩充tab,表格化输出
test = "username\temail\tpassword\nlaiying\tying@q.com\t123\nlaiying\tying@q.com\t123\nlaiying\tying@q.com\t123" v = test.expandtabs(20) print(v) username email password laiying ying@q.com 123 laiying ying@q.com 123 laiying ying@q.com 123
8)find() ==>从开始往后找,找到第一个之后,获取其位置,未找到返回-1
test = "abcdefg" v = test.find('c') print(v) #2
9)index() ==>从开始往后找,找不到,报错
test = "abcabc" v = test.index('b') print(v) #1,找不到报错:ValueError: substring not found
10)format(),format_map() ==>格式化,将一个字符串中的占位符替换为指定的值
test = 'i am {name}, age {a}' v = test.format(name='lawrence',a=19) print(v) test = 'i am {0}, age {1}' v = test.format('Lawrence',19) print(v) test = 'i am {name}, age {a}' v2 = test.format_map({"name": 'Lawrence', "a": 19}) print(v2)
11)isalnum() ==>字符串中是否只包含 字母和数字
# test = "123abd" #true test = "123abd@" #false v = test.isalnum() print(v)
12)isalpha() ==> 是否是字母,汉字
# test = "as2df" #false test = "中" #true v = test.isalpha() print(v)
13)isdecimal(),isdigit(),isnumeric() ==>当前输入是否是数字
test = "1" v1 = test.isdecimal() #true v2 = test.isdigit() #true v3 = test.isnumeric() #true print(v1,v2,v3) test = "二" v1 = test.isdecimal() #false v2 = test.isdigit() #false v3 = test.isnumeric() #faulse print(v1,v2,v3) test = "②" v1 = test.isdecimal() #false v2 = test.isdigit() #true v3 = test.isnumeric() #false print(v1,v2,v3)
14)isprintable() ==>是否存在不可显示的字符\t,\n
test = "oiuas\tdfkj" v = test.isprintable() print(v) #false
15)isspace() ==>判断是否全部是空格
test = " " v = test.isspace() print(v) #true
16)title(),istitle() ==>判断是否是标题
test = "Return True if all cased characters in S are uppercase and there is" v1 = test.istitle() print(v1) #false v2 = test.title() print(v2) #Return True If All Cased Characters In S Are Uppercase And There Is v3 = v2.istitle() print(v3) #true
17)join() ==>将字符串中的每一个元素按照指定分隔符进行拼接
test = "abcdef" v = "*".join(test) print(v) #a*b*c*d*e*f
18)lower(),islower() ==>判断是否全部是大小写和转换为大小写
test = "Abc" v1 = test.islower() v2 = test.lower() print(v1, v2) #false abc v1 = test.isupper() v2 = test.upper() print(v1,v2) #false ABC
19) strip(),lstrip(),rstrip() ==>移除指定的字符串或空白
#移除指定的字符 test = "xa" v = test.lstrip('x') #a # v = test.rstrip('a') #x # v = test.strip('x') #x print(v) #去除左右两边的空白,去除\t \n test = " abc " v = test.lstrip() v = test.rstrip() v = test.strip() print(v)
20)translate() ==>对应关系替换
test = "aeiou" test1 = "12345" v = "asidufkasd;fiuadkf;adfkjalsdjf" m = str.maketrans("aeiou", "12345") new_v = v.translate(m) print(new_v) #1s3d5fk1sd;f351dkf;1dfkj1lsdjf
21) partition() ==>分割字符串,分割成三份,包含分割字符串的元组
test = "testasdsddfg" # v = test.partition('s') #('te', 's', 'tasdsddfg'),从左边第一个分割,包含分割字符串 # print(v) v = test.rpartition('s') #('testasd', 's', 'ddfg'),从右边第一个分割,包含分割字符串 print(v)
22)split(),rsplit() ==>分割为指定个数
test = "testasdsddfg" v = test.split('s') #['te', 'ta', 'd', 'ddfg'] # v = test.split('s',2) #['te', 'ta', 'dsddfg'] print(v)
23)splitlines() ==>分割,根据true,false是否保留换行
test = "asdfadfasdf\nasdfasdf\nadfasdf" v = test.splitlines(True) #['asdfadfasdf\n', 'asdfasdf\n', 'adfasdf'] #v = test.splitlines(False) #['asdfadfasdf', 'asdfasdf', 'adfasdf'] print(v)
24)swapcase() ==>大小写转换
test = "aBc" v = test.swapcase() print(v) #AbC
25)isidentifier() ==>判断是否是字母,数字,下划线,标识符,如def,class
# a = "def" #true a = "=" #false v = a.isidentifier() print(v)
26)replace() ==>将指定字符串替换为指定字符串
test = "abcabcabc" v = test.replace("c", 'XXX') #abXXXabXXXabXXX #v = test.replace("c", 'XXX', 2) #abXXXabXXXabc 从左到右替换两个 print(v)
4.2.3、字符串索引及切片
1)索引
test = "abcdef" v = test[3] print(v)
2)切片 ==>顾头不顾尾
test = "abcdef" v1 = test[1:3] #bc v2 = test[0:-1] #abcde print(v1,v2)
3)获取字符串的长度,有几个字符组成
test = "abcdef" print(len(test)) #6
五、其他操作
5.1、range()函数
获取连续或不连续的数字
注意:Python2中直接创建在内容中,python3中只有for循环时,才一个一个创建
# v = range(10) #0-10 # v = range(1,10) #1-10 v = range(1,10,2) #指定步长 for item in v: print(item)
根据用户输入的值,输出每一个字符以及当前字符所在的索引位置:
test = input(">>>") for item in range(0, len(test)): print(item, test[item])
-------------------------------------------
个性签名:独学而无友,则孤陋而寡闻。做一个灵魂有趣的人!
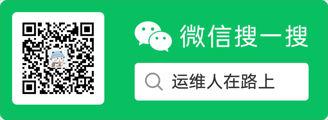