echarts-散点图-关系图-vue3-内阴影
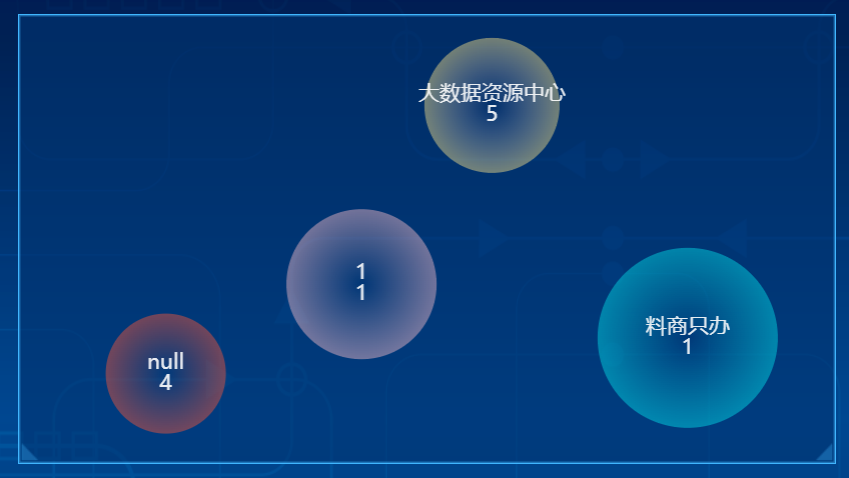
以上为效果图
但是有一个问题是,一开始需要定义位置和颜色数组,当数据量过多的时候会重叠而且要提前声明很多数据
后面我决定用关系图来做了,先放上散点图代码供参考
function initReport(sdata) { const myChart0 = echarts.init(main0.value); const offsetData = [ [10, 20], [40, 40], [60, 80], [90, 28], [30, 50], [20, 50], [30, 80], [40, 30], [50, 10], ]; const colorData = [ ["rgba(254,86,55,0.00)", "#FE5637"], ["rgba(254,192,203,0.00)", "#FEC0CB"], ["rgba(255,230,1800,0.00)", "#FFE612"], ["rgba(3,228,236,0.00)", "#03E4EC"], ["rgba(231,115,200,0.00)", "#E773C8"], ["rgba(0,217,138,0.00)", "#00D98A "], ["rgba(255,230,18,0.00)", "#B8860A"], ["rgba(184,134,10,0.00)", "#FFE612"], ["rgba(3,228,236,0.00)", "#03E4EC"], ]; const symbolSizeData = [80, 100, 90, 120, 120,70,60,60,80]; const optiondata = []; for (var i = 0; i < sdata.length; i++) { if (sdata.length > 9) { offsetData.push([ Math.floor(Math.random() * (100 - 40)) + 40, Math.floor(Math.random() * (100 - 30)) + 30, ]); colorData.push(["rgba(255,230,1800,0.00)", "#FFE612"]); symbolSizeData.push(Math.floor(Math.random() * (120 - 80)) + 80) } const item = sdata[i]; optiondata.push({ name: item.name, number: item.count, value: offsetData[i], symbolSize: symbolSizeData[i], color: colorData[i], itemStyle: { normal: { color: new echarts.graphic.RadialGradient(0.5, 0.5, 1, [ { offset: 0, color: colorData[i][0], }, { offset: 1, color: colorData[i][1], }, ]), opacity: 0.9, shadowColor: "transparent", }, }, }); } const option0 = { grid: { top: 0, bottom: 0, }, xAxis: [ { type: "value", show: false, min: 0, max: 100, }, ], yAxis: [ { show: false, min: 0, max: 100, }, ], series: [ { type: "scatter", symbol: "circle", label: { normal: { show: true, formatter: function (params) { var res = params.data.name + "\n" + params.data.number; return res; }, color: "#fff", textStyle: { fontSize: "14", }, }, }, animationDurationUpdate: 500, animationEasingUpdate: 500, animationDelay: function (idx) { return idx * 100; }, data: optiondata, }, ], }; myChart0.setOption(option0); window.addEventListener("resize", () => { myChart0.resize(); }); }
是在vue3框架里面写的,里面的数据格式为:
[{name: '可乐', count: 49}]
代码优化:转为关系图设置

function initTest(data = [], format = []) { // 入参说明: // 1. data 原始气泡数据,是一个对象数组,形如[{name: '可乐', count: 49}] // 2. format 数组依次指定气泡中展示的名称以及影响气泡大小的数据值, 形如['name', 'count'] let [maxValue, temp] = [0, []]; data.forEach((item) => { temp.push(item[format[1]]); }); maxValue = Math.max.apply(null, temp); // 气泡颜色数组 let colorData = [ ["rgba(254,86,55,0.00)", "#FE5637"], ["rgba(254,192,203,0.00)", "#FEC0CB"], ["rgba(255,230,1800,0.00)", "#FFE612"], ["rgba(3,228,236,0.00)", "#03E4EC"], ["rgba(231,115,200,0.00)", "#E773C8"], ["rgba(0,217,138,0.00)", "#00D98A "], ["rgba(255,230,18,0.00)", "#B8860A"], ["rgba(184,134,10,0.00)", "#FFE612"], ["rgba(3,228,236,0.00)", "#03E4EC"], ]; // 气泡颜色备份 let bakeColor = [...colorData]; // 气泡数据 let bubbleData = []; // 气泡基础大小 let basicSize = 70; // 节点之间的斥力因子,值越大,气泡间距越大 let repulsion = 380; // 根据气泡数量配置基础大小和斥力因子(以实际情况进行适当调整,使气泡合理分布) if (data.length >= 5 && data.length < 10) { basicSize = 50; repulsion = 230; } if (data.length >= 10 && data.length < 20) { basicSize = 40; repulsion = 150; } else if (data.length >= 20) { basicSize = 30; repulsion = 75; } // console.log(bakeColor) // 填充气泡数据数组bubbleData for (let item of data) { // 确保气泡数据条数少于或等于气泡颜色数组大小时,气泡颜色不重复 if (!bakeColor.length) bakeColor = [...colorData]; let colorSet = new Set(bakeColor); let curIndex = Math.round(Math.random() * (colorSet.size - 1)); let curColor = bakeColor[curIndex]; colorSet.delete(curColor); bakeColor = [...colorSet]; // 气泡大小设置 let size = (item[format[1]] * basicSize * 2) / maxValue; if (size < basicSize) size = basicSize; console.log(colorSet.size); bubbleData.push({ name: item[format[0]], value: item[format[1]], symbolSize: size, draggable: true, itemStyle: { normal: { color: new echarts.graphic.RadialGradient(0.5, 0.5, 1, [ { offset: 0, color: curColor[0], }, { offset: 1, color: curColor[1], }, ]), opacity: 0.9, shadowColor: "transparent", }, }, }); } let myChart0 = echarts.init(main0.value); let option0 = { animationEasingUpdate: "bounceIn", series: [ { type: "graph", layout: "force", force: { repulsion: repulsion, edgeLength: 10, }, label: { normal: { show: true, formatter: function (params) { var res = params.data.name + "\n" + params.data.value; return res; }, color: "#fff", textStyle: { fontSize: "14", }, }, }, // 是否开启鼠标缩放和平移漫游 roam: false, // label: { normal: { show: true } }, data: bubbleData, }, ], }; myChart0.setOption(option0); window.addEventListener("resize", () => { myChart0.resize(); }); }
本文仅提供参考,是本人闲时所写笔记,如有错误,还请赐教,作者:阿蒙不萌,大家可以随意转载