05类
函数题1-10 编程题 1-10
##1
public int sum(double...values)
//接受若干个,最后一个为valus
##2
//构造器条件判断
if(x > 0&& y > 0&&z > 0&&p > 0)
else
##3
/数字转化成字符串后返回
double area=this.width*this.height;
return String.format("%.2f", area);
System.out.println(r+" "+String.format("%.2f",h)+" "+mon);
##4最大公约数
//比较绝对值
public int gys(int a, int b)
{
int n = Math.min(Math.abs(a), Math.abs(b));
int m = Math.max(Math.abs(a), Math.abs(b));
int t;
while(n != 0)
{
t = m % n;
m = n;
n = t;
}
return m;
}
##5重写toString
@Override
public String toString() {
return "("+x+","+y+")";
}
##5
return new Point(x, y);
//多个值需要通过类或数组返回
Point p = new Point(cin.nextInt(), cin.nextInt());
//可以直接这样输入赋值合并
##6
double A=radius*radius*Math.PI;
return (float)((Math.round(A * 100)) / 100.0);
##7
//字符串转化为字符数组
String str=sc.next();
char[] ch=str.toCharArray();
06 继承多态接口
函数题1-10 编程题 1-8
##1类对象是否相等
public boolean equals(Object o) {
if (this == o) return true; // 同一对象
if (o == null || getClass() != o.getClass()) return false; // 空或不同类
Point point = (Point) o; // 类型转换
return this.xPos == point.xPos && this.yPos == point.yPos; // 属性比较
}
##2compare接口
class Octagon implements Comparable<Octagon>,Cloneable {
@Override
public int compareTo(Octagon o) {
if (this.side > o.getSide())
return 1;
else if (this.side < o.getSide())
return -1;
else
return 0;
}
}
@Override
protected Object clone() {
return this;
}
##3super
//子类重写的方法中调用父类方法
return 2 * super.area() + length() * z;
// 调用的是父类 Rect 中定义的 area() 方法
##4
USB[] usbs=new USB[2];
usbs[0]=new UPan();
usbs[1]=new Mouse();
for(int i=0;i<usbs.length;i++)
{
usbs[i].work();
usbs[i].stop();
}
##5答答租车系统
import java.util.Scanner;
class Car {
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public int id;
public int rent;
public String name;
public int getLoadr() {
return loadr;
}
public void setLoadr(int loadr) {
this.loadr = loadr;
}
public int getRent() {
return rent;
}
public void setRent(int rent) {
this.rent = rent;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getLoadh() {
return loadh;
}
public void setLoadh(double loadh) {
this.loadh = loadh;
}
public int loadr;
public double loadh;
public Car(int id, String name,int loadr, double loadh,int rent ) {
this.id=id;
this.name = name;
this.rent = rent;
this.loadr = loadr;
this.loadh = loadh;
}
}
public class Main
{
public static void main(String args[])
{
Car[] car = new Car[11];
car[1] = new Car(1, "A", 5, 0, 800);
car[2] = new Car(2, "B", 5, 0, 400);
car[3] = new Car(3, "C", 5, 0, 800);
car[4] = new Car(4, "D", 51, 0, 1300);
car[5] = new Car(5, "E", 55, 0, 1500);
car[6] = new Car(6, "F", 5, 0.45, 500);
car[7] = new Car(7, "G", 5, 2.0, 450);
car[8] = new Car(8, "H", 0, 3, 200);
car[9] = new Car(9, "I", 0, 25, 1500);
car[10] = new Car(10, "J", 0, 35, 2000);
Scanner sc=new Scanner(System.in);
int n=sc.nextInt();
if(n==1)
{
int num=sc.nextInt();
int r=0;
double h=0.00;
int mon=0;
for(int i=1;i<=num;i++)
{
int idd=sc.nextInt();
int day= sc.nextInt();
r+=car[idd].getLoadr()*day;
h+=car[idd].getLoadh()*day;
mon+=car[idd].getRent()*day;
}
System.out.println(r+" "+String.format("%.2f",h)+" "+mon);
}
else
System.out.println("0 0.00 0");
}
}
##6 赋值一定记得判断0
public Rectangle(double l, double h) {
if (l > 0 && h > 0) {
// 二者均大于0,才能构成图形
this.l = l;
this.h = h;
}
}
## 7 判断类类型
if (A[i] instanceof Rectangle) {
System.out.print("Rectangle ");
System.out.print(A[i].toString());
}
##8
ArrayList<PersonOverride> ap=new ArrayList<PersonOverride>();
for (int i=0;i<n1;i++){
ap.add(new PersonOverride());
}
int n2= sc.nextInt();
// sc.nextLine();
for (int i=0;i<n2;i++){
f=true;
PersonOverride a=new PersonOverride(sc.next(),sc.nextInt(), sc.nextBoolean());
// sc.nextLine();
for (PersonOverride aa:ap) {
if (aa.equals(a)){
f=false;
break;
}
}
if (f)ap.add(a);
}
for(PersonOverride a:ap){
System.out.println(a.toString());
}
System.out.println(ap.size()-n1);
System.out.println(Arrays.toString(PersonOverride.class.getConstructors()));
//输出构造器,它返回的是一个 Constructor 类型的数组,所以用Arrays.toString()
## 8输出
System.out.println("The area of " + c.toString() + " is " + String.format("%.2f",c.getArea()));
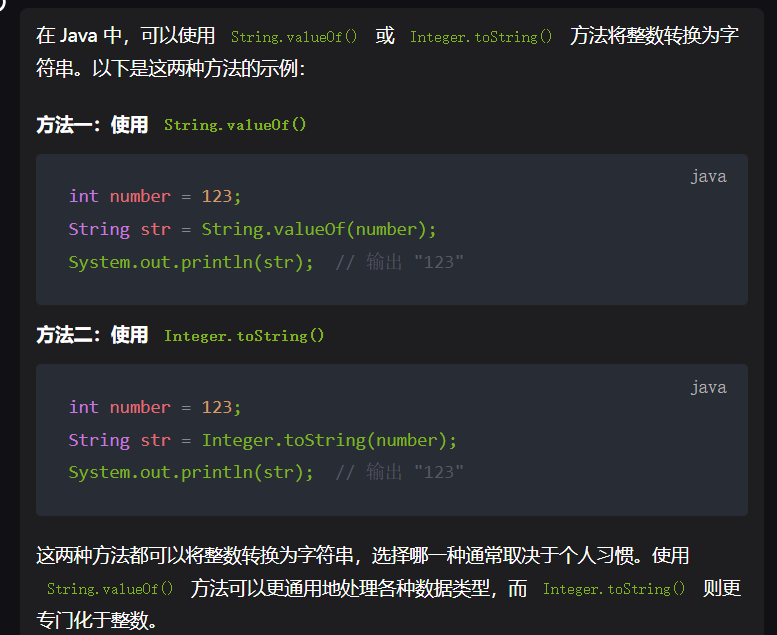
07 异常
函数题 1-6 编程题1-6
## 1
public Integer push(Integer item) throws FullStackException {
if(this.size() >= this.arrStack.length )throw new FullStackException();
if (item == null)
return null;
this.arrStack[this.top] = item;
this.top++;
return item;
}//如果item为null,则不入栈直接返回null。如果栈满,抛出`FullStackException`。
public Integer pop() throws EmptyStackException {
if(this.empty()) throw new EmptyStackException();
if (this.arrStack[top-1] == null)
return null;
this.top--;
return this.arrStack[top];
//pop返回的是弹出的元素,指向这个元素,但是因为peek不这么取所以相当于弹出
}//出栈。如果栈空,抛出EmptyStackException。否则返回
public Integer peek() throws EmptyStackException {
if(top == 0) throw new EmptyStackException();
if (arrStack[top-1] == null) return null;
return arrStack[top-1];
}//获得栈顶元素。如果栈空,抛出EmptyStackException。
##2 自定义异常类
import java.util.*;
public class Main {
public static void main(String[] args) {
double s=0;
Scanner sc=new Scanner(System.in);
double r1,r2;
r1=sc.nextDouble();
r2=sc.nextDouble();
Circle c1=new Circle(r1);
try{
s = c1.area();
System.out.println(s);
}
catch (CircleException e){
e.print();
}
}
}
class Circle{
double r;
public Circle(double r)
{
this.r=r;
}
double area()throws CircleException{
if(this.r<0)
{
throw new CircleException(this.r);
//throw实例化时不加s
}
return 3.14*r*r;
}
}
//自定义异常类
class CircleException extends Exception{
double r;
public CircleException(double r)
{
this.r=r;
}
void print(){
System.out.println("圆半径为"+this.r+"不合理");
}
}
## 3
System.out.println(e.toString()); 和 System.out.println(e); 在 Java 中是等效的,都会自动调用对象的 toString() 方法。
## 3强制转化注意
else if (a.equals("cast")){
// String b = input.next();
try {
Object str = new String("");
//必须用Object
System.out.println((Integer)(str));
}catch (Exception e){
System.out.println(e);
}
## 4 退出程序,数组输出
System.exit(0);
System.out.println(Arrays.toString(a));
## 5 自定义异常(综合)
import java.util.*;
public class Main{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
while (sc.hasNext()) {
//这里有一个while循环
if (!sc.next().equals("new")) {
System.out.println("scanner closed");
sc.close();
break;
}
else {
sc.nextLine();
//要读空格
String s = sc.nextLine();
String[] s2 = s.split("\\s+");
try {
Student stu = new Student();
stu.setName(s2[0]);
stu.addScore(Integer.parseInt(s2[1]));
System.out.println(stu);
}
catch (IllegalNameException|IllegalScoreException e)
//只在最后一个加e
{
System.out.println(e);
} catch (Exception e) {
System.out.println("java.util.NoSuchElementException");
}
}
}
}
}
class Student{
Student(){
this.score = 0;
};
private String name;
public int getScore() {
return score;
}
public void setScore() {
this.score=score;
}
public void addScore(int score) throws IllegalScoreException {
if (this.score + score < 0||this.score + score > 100)
throw new IllegalScoreException(score);
this.score = score;
}
public String getName() {
return name;
}
public void setName(String name) throws IllegalNameException {
if(name.charAt(0)>='0'&&name.charAt(0)<='9')
throw new IllegalNameException(name);
this.name = name;
}
private int score;
@Override
public String toString() {
return "Student [name="+name+", score="+score+"]";
}
}
class IllegalNameException extends Exception{
String name;
IllegalNameException(String name)
{
this.name=name;
}
public String toString()
{
return "IllegalNameException: the first char of name must not be digit, name="+name;
}
}
class IllegalScoreException extends Exception{
int score;
IllegalScoreException(int score)
{
this.score=score;
}
public String toString()
//继承的是父类,不用传参
{
return "IllegalScoreException: score out of range, score="+score;
}
}
//是addscore
08 字符串
编程题 1-8
## 1 StringBuffer
StringBuffer buffer = new StringBuffer(str);
System.out.println(buffer.reverse());
## 2 去除数字
String newstr = str.replaceAll("\\d+", "");
## 3
String[] split = line.split(";");
book.indexOf(":")
book.substring(0, index)
book.trim();
Integer.parseInt(bookPrice);
## 4 map
map.put();
map.get();
map.getOrDefault(name,0);
map.keySet();
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String name=null;
Map<String,Integer> map=new LinkedHashMap<String,Integer>();
//是integer不是int
while(true)
{
name=sc.next();
if(name.equals("end"))
break;
map.put(name, map.getOrDefault(name, 0)+1);
//map.getordefault
}
for(String name1:map.keySet())
{
System.out.println(name1+" "+map.get(name1));
}
}
}
## 5合法字符
Character.isJavaIdentifierStart(s.charAt(0))
Character.isJavaIdentifierPart(s.charAt(i))
s.endsWith(".java")
09 其他
编程题 1-7
## 1 大数
num1 = new BigInteger(reader.next());
add,substract,multiply,divede,mod;
## 2进制
public static String bin(int num1){//递归实现,将十进制转为二进制
if(num1/2==0){//商为0时
return num1%2 + "";//返回转换为String类型的余数
}else{
return bin(num1/2) + num1%2 + " ";//下一层递归的结果放左侧
}
}
## 3 随机数
Random r = new Random(seed);
double x =r.nextDouble()*2-1;
## 4 日历
import java.util.Calendar;
import java.util.Scanner;
public class Main{
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
Calendar cal=Calendar.getInstance();
int a=sc.nextInt();
int count=0;
if (a>=1998) {
for (int i = 0; i <11; i++) {
cal.set(Calendar.YEAR, a);
cal.set(Calendar.MONTH, i);//月份少一
cal.set(Calendar.DATE, 13);
int t=cal.get(Calendar.DAY_OF_WEEK);
if (t==6) {//天数+1
count++;
}
}
}
System.out.println(count);
}
}