Vue3笔记
本节代码可以从
git clone https://gitee.com/fullstacker1024/vue3-component-demo.git
下载
其中项目实例https://gitee.com/fullstacker1024/vue3-big-event-admin.git
1、前言
1)Vue3的优势
(1)更容易维护
①组合式API
②更好的TypeScript支持
(2)更小的体积
①良好的TreeShaking
②按需引入
(3)更快的速度
①重写diff算法
②模板编译优化
③更高效的组件初始化
(4)更优的数据响应式
proxy
2)create-vue搭建Vue3项目
create-vue是Vue官方新的脚手架工具,底层切换到了
vite(下一代构建工具)
,为开发提供极速响应。
(1)前提环境条件
已安装16.0或更高版本的Node.js
(2)创建一个Vue应用
npm init vue@latest
这一指令将会安装并执行create-vue
运行项目:
npm install
npm run dev
3)项目目录和关键文件
vue2插件是Vetur,vue3插件是volar
2、组合式API
1)setup选项
(1)setup选项的的写法和执行时机
(2)setp选项中写代码的特点
(3)<script setup/>
语法糖
2)reactive和ref函数
(1)reactive()
作用:接受对象类型数据的参数传入
并返回一个响应式的对象
核心步骤:
从vue包中导入reactive函数
在<script setup/>
中执行reactive函数并传入类型为对象的初始值,并使用变量接收返回值
<script setup>
import {reactive} from 'vue'
const state =reactive(对象类型数据)
</script>
(2)ref()
作用:接收简单类型或对象类型的数据
传入并返回一个响应式对象
核心步骤:
从vue包中导入ref函数
在<script setup/>
中执行ref函数并传入初始值,使用变量接收ref函数的返回值
<script setup>
import {ref} from 'vue'
const count =ref(对象类型数据)
</script>
注意点:
脚本中访问数据,需要通过
.value
在template中,.value
不需要加
3)computed
计算属性的基本思想和Vue2的完全一致,组合式API下的计算属性只是修改了写法。
核心步骤:
导入
computed
函数
执行函数在回调参数中return基于响应式数据做计算的值,用变量接收
示例:
点击查看代码
<script setup>
import { ref, computed } from 'vue'
const list = ref([1, 2, 3, 4, 5, 6, 7, 8, 9])
const computedList = computed(() => {
return list.value.filter(item=>item>5)
})
const addFn=()=>{
var randomNumber = Math.floor(Math.random() * 10) + 1;
list.value.push(randomNumber)
}
</script>
<template>
<div>{{ list }}</div>
<div>>5:{{ computedList }}</div>
<div><button @click="addFn">增加随机数</button></div>
</template>
<style scoped></style>
计算属性中不应该有“副作用”:比如异步请求/修改dom
避免直接修改计算属性的值:计算属性应该是只读的,特殊情况可以配置get/set
4)watch
作用:侦听一个或者多个数据的变化,数据变化时执行回调函数。
两个额外参数:1.immediate(立即执行)2.deep(深度侦听)
(1)侦听单个数据
①导入watch函数
②执行watch函数传入要侦听的响应式数据(ref对象)
和回调函数
点击查看代码
<script setup>
import { watch, ref } from 'vue'
const count = ref(0)
watch(count, (newValue, oldValue) => {
console.log(`count发生了变化,旧值为${oldValue},新值为${newValue}`);
})
</script>
(2)侦听多个数据
同时侦听多个响应式数据的变化,不管哪个数据变化都需要侦听
点击查看代码
<script setup>
import { watch, ref } from 'vue'
const count = ref(0)
const name = ref('cp')
watch([count, name], ([newCount, newName], [oldCount, oldName]) => {
console.log('count或者name发生了变化', [newCount, newName], [oldCount, oldName]);
})
const changeCount=()=>{
count.value++
}
</script>
<template>
<div>{{count}}-{{ name }}</div>
<div><button @click="changeCount">watch</button></div>
</template>
<style scoped></style>
(3)immediate
立刻执行
点击查看代码
<script setup>
import { watch, ref } from 'vue'
const count = ref(0)
watch(count, (newValue, oldValue) => {
console.log(`count发生了变化,旧值为${oldValue},新值为${newValue}`);
},{
immediate:true
})
</script>
(4)deep
深度监视
,默认watch进行的是浅层监视
const ref1=ref(简单类型) //可以直接监视
const ref2=ref(复杂类型)//监视不到复杂类型内部数据的变化
点击查看代码
<script setup>
import { watch, ref } from 'vue'
const userInfo = ref({
name: 'zs',
age: 18
})
watch(userInfo, (newValue) => {
console.log(newValue)
},{
deep:true
})
const changeUserInfo = () => {
userInfo.value.name = 'lisi'
}
</script>
<template>
<div>{{ userInfo }}</div>
<div><button @click="changeUserInfo">watch</button></div>
</template>
<style scoped></style>
(5)精确侦听对象的某个属性
在不开启deep的前提下,侦听age的变化,只有age变化时才知晓回调。
5)生命周期函数
6)父子通信-defineProps、defineEmits
(1)父传子
基本思想:
父组件中给子组件绑定属性
子组件内部通过props选项接收defineProps编译器宏
defineProps原理:就是编译阶段的一个标识,实际编译器解析时,遇到后悔进行编译转换
父组件传过来的数据在script中需要使用props.message形式调用
父组件传过来的数据在template中直接调用:message
动态值使用:
绑定
(2)子传父
父组件中给子组件标签通过@绑定事件
子组件内部通过emit方法触发事件defineEmits
7)模板引用-defineExpose
通过ref标识
获取真实的dom对象或者组件实例对象
调用ref函数生成一个ref对象
通过ref标识绑定ref对象到标签
(1)defineExpose()
默认情况下<scritp setup>语法糖下组件内部的属性和方法是不开放
给父组件访问的,可以通过defineExpose
编译宏指定哪些属性和方法容许访问
8)provide和inject
作用和场景:顶层组件向任意的底层组件传递数据和方法,实现跨层组件通信。
顶层组件通过provide函数提供数据
底层组件通过inject函数获取数据
(1)跨层传递普通数据
provide第二个参数是普通数据
//top.vue
import { provide } from 'vue';
//普通数据
provide('theme-color', 'pink')
//bottom.vue
import { inject } from 'vue';
const themeColor =inject('theme-color')
(2)跨层传递响应式数据
provide第二个参数是ref对象
//top.vue
import { provide, ref } from 'vue';
//响应式数据
const count = ref(100)
provide('count', count)
//bottom.vue
import { inject } from 'vue';
const count=inject('count')
(3)跨层传递方法
顶层组件可以向底层组件传递方法,底层组件调用方法修改顶层组件中的数据。
provide第二个参数是函数
//top.vue
//传递方法
import { provide, ref } from 'vue';
const count = ref(100)
provide('changeCount', (newCount) => {
count.value = newCount
})
//bottom.vue
import { inject } from 'vue';
const changeCount=inject('changeCount')
const ChangeFn=()=>{
changeCount(5)
}
3、Vue3.3新特性
1)defineOptions
背景说明:
有< script setup/>之前,如果要定义props,emits可以轻而易举地天剑一个与setup平级的属性。但是用了< script setup/>后,就没法这么干了,setup属性已经没有了,自然无法添加与其平级的属性。
为了解决这一问题,引入了
defineProps
与defineEmits
这两个宏。但这只解决了props与emits这两个属性。如果我们要定义组件的name或其他自定义的属性,还是得回到最原始的用法:再添加一个普通的< script>标签。这样就会存在两个< script>标签。让人无法接受。
所以在Vue3.3中新引入了
defineOptions
宏。顾名思义,主要是来定义Options API
的选项。可以用defineOptionis定义任意的选项,props、emits、expose、slots
除外(因为这些可以使用defineXXX来做到)。
<script setup>
defineOptions({
name: 'Foo',
inheritAttrs:false,
//...更多自定义属性
})
</script>
2)defineModel
在Vue3中,自定义组件上使用v-model,相当于传递一个modelValue
属性,同时触发update:modelValue
事件。
<Child v-model="isVisible">
//相当于
<Child :modelValue="isVisible" @update:modelValue="isVisible=$event">
①不使用defineModel
我们需要先定义props,再定义emits。其中有许多重复的代码。如果需要修改此值,还需要手动调用emit函数。
示例代码:
点击查看代码
//父组件
<script setup>
import noDefineModelComponent from '@/components/defineModel/noDefineModelComponent.vue'
import {ref} from 'vue'
const txt=ref('1')
</script>
<template>
<div>
{{ txt }}
<noDefineModelComponent v-model="txt"></noDefineModelComponent>
</div>
</template>
<style scoped>
</style>
//子组件
<script setup>
defineProps({
modelValue: String
})
const emit = defineEmits(['update:modelValue'])
</script>
<template>
<div>
<input type="text" :value="modelValue" @input="e => emit('update:modelValue', e.target.value)">
</div>
</template>
<style scoped></style>
②使用defineModel
需要先在vite.config.js
中开启defineModel配置
plugins: [
vue({
script: {
defineModel: true,
},
}),
],
示例代码:
点击查看代码
//父组件
<script setup>
import defineModelComponent from '@/components/defineModel/defineModelComponent.vue'
import {ref} from 'vue'
const txt=ref('1')
</script>
<template>
<div>
{{ txt }}
<defineModelComponent v-model="txt"></defineModelComponent>
</div>
</template>
<style scoped>
</style>
//子组件
<script setup>
import { defineModel } from 'vue'
const modelValue = defineModel()
</script>
<template>
<div>
<input type="text" :value="modelValue" @input="e => modelValue= e.target.value">
</div>
</template >
<style scoped></style>
4、路由
1)路由初始化
创建路由实例由
createRouter
实现
路由模式:
①history模式使用createWebHistory()
②hash模式使用createWebHashHistory()
③参数是基础路径
,默认/
示例:
创建一个路由实例,路由模式是history模式,路由的基础地址是vite.config.js中base配置的值,默认是/。
2)路由对象和路由参数
(1)在Vue3 CompositionAPI中使用useRouter
获取路由对象
<script setup>
import {useRouter} from 'vue-router'
const router=useRouter()
</script>
(3)在Vue3 CompositionAPI中使用useRoute
获取路由参数
<script setup>
import {useRoute} from 'vue-router'
const route=useRoute()
</script>
5、Pinia
Pina是Vue的最新的状态管理工具
是Vuex的替代品。
提供更加坚定的API(去掉了mutation)
提供符合组合式风格的API(和Vu3新语法统一)
去掉了modules的概念,每一个store都是一个独立的模块
配合 TypeScript更加友好,提供可靠的类型推断
1)手动添加Pinia到Vue项目
在实际开发项目的时候,关于Pinia的配置,可以在项目创建时自动添加。
现在我们手动添加下:
①使用vite创建一个空的vue3项目
npm create vue@latest
②按照官方文档安装pinia到项目中
import { createApp } from 'vue'
import { createPinia } from 'pinia'
import App from './App.vue'
const pinia =createPinia()
createApp(App).use(pinia).mount('#app')
定义了一个store,该store在调用之前,store实例是不会被创建的。
store是一个用reactive包装的对象,这意味着不需要再getters后面写.value
,就像setup中的props一样,如果写了,也不能解构它。
2)Pinia基本语法-state、action、getters
(1)定义
新建store/couter.js
文件:
示例:
import { defineStore } from "pinia";
import { computed, ref } from "vue";
//定义store
export const counterStore = defineStore("counter", () => {
//声明数据 state
const count = ref(0);
//声明操作数据的方法 action
const addCount = () => count.value++;
const subCount = () => count.value--;
//声明基于数据派生的计算数学 getters
const double=computed(()=>count.value*2)
const msg = ref("hello pinia");
return {
count,addCount,subCount,double,
msg,
};
});
(2)使用:
导入couter.js后,可以在scritp或者template中直接使用。
示例:
<script setup>
import { counterStore } from '@/store/counter';
const userCounterStore=counterStore()
</script>
<template>
<div class="parent">
<h3>ParentApp-{{userCounterStore.count}}-{{ userCounterStore.msg }}-{{ userCounterStore.double }}</h3>
</div>
</template>
<style scoped>
.parent {
border: 1px solid #000;
padding: 30px;
}</style>
3)action异步写法
异步action函数的写法和组件中获取异步数据的写法完全一致。
4)storeToRefs写法
为了从store中提取属性(作为action的方法除外,可以直接解构)时保持其响应性,你需要使用
storeToRefs()
。它将为每一个响应式属性创建引用。
直接解构,不处理,数据会丢失响应式
<script setup>
//counter.js见上节
import { useChannelStore } from '@/store/counter';
const channelStore=useCounterStore()
const {count ,msg}=counterStore
</script>
使用storeToRefs
<script setup>
//counter.js见上节
import { useChannelStore } from '@/store/counter';
import {storeToRefs} from 'pinia'
const channelStore=useCounterStore()
const {count ,msg}=storeToRefs(counterStore)
</script>
5)持久化
Pinia持久化插件:
(1)使用步骤
①安装插件pinia-plugin-persistedstate
npm i pinia-plugin-persistedstate
②main.js使用
将插件添加到pinia实例上
import persist from 'pinia-plugin-persistedstate'
//...
app.use(CreatePinia().use(persist))
③store仓库中,persist:true(选项式)开启
以上步骤,整改store将使用默认持久化配置保持。
如果你真心觉得文章写得不错,而且对你有所帮助,那就不妨小小打赏一下吧,如果囊中羞涩,不妨帮忙“推荐"一下,您的“推荐”和”打赏“将是我最大的写作动力!
本文版权归作者所有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接.
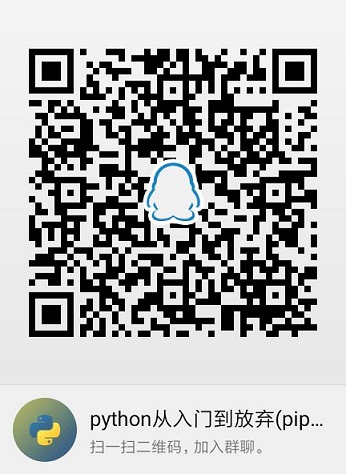
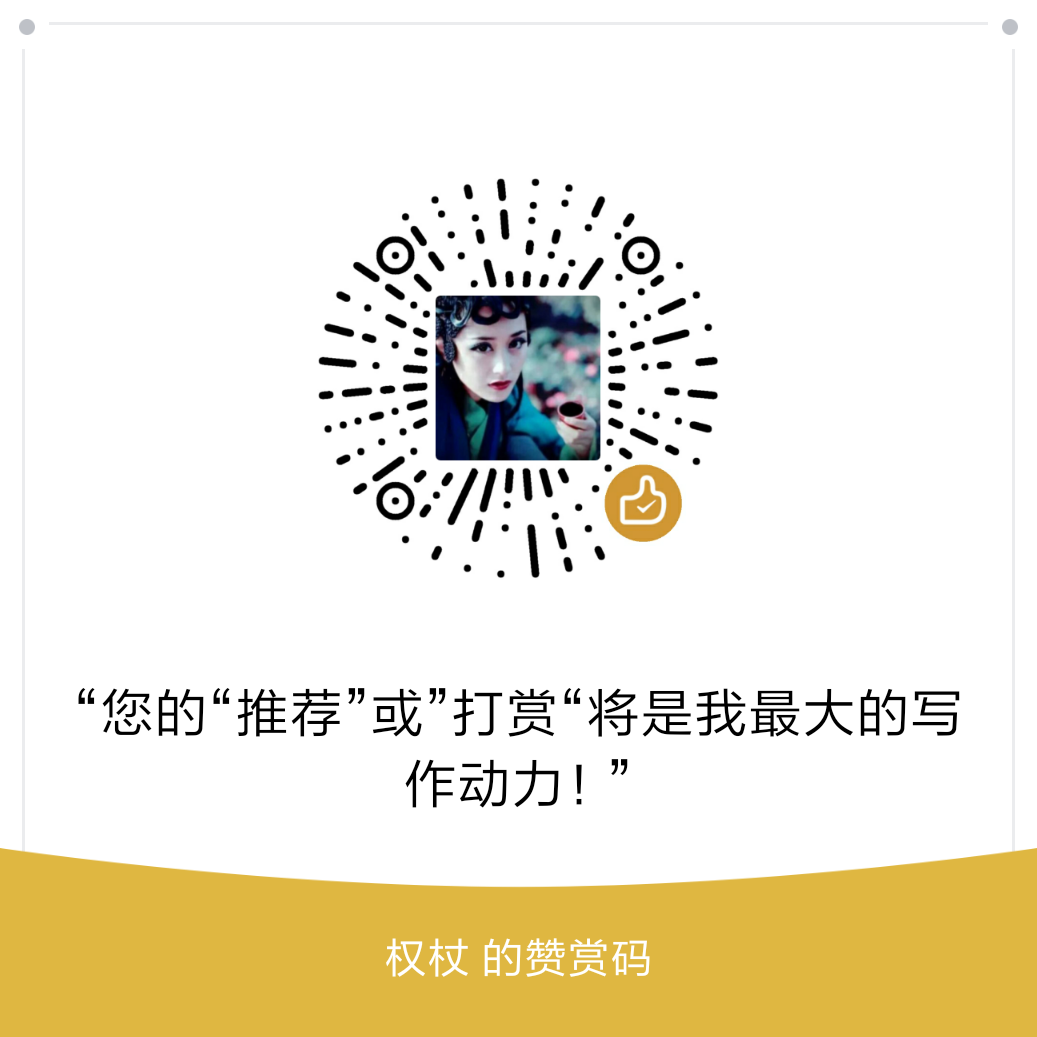