SpringBoot入门二:与Mybatis整合
一、编程步骤
1、引入依赖
springboot相关依赖(略)、mybatis-spring-boot-starter、mysql、druid、lombook
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | < dependency > < groupId >org.mybatis.spring.boot</ groupId > < artifactId >mybatis-spring-boot-starter</ artifactId > < version >2.1.4</ version > </ dependency > < dependency > < groupId >mysql</ groupId > < artifactId >mysql-connector-java</ artifactId > </ dependency > < dependency > < groupId >com.alibaba</ groupId > < artifactId >druid</ artifactId > < version >1.2.5</ version > </ dependency > < dependency > < groupId >org.projectlombok</ groupId > < artifactId >lombok</ artifactId > </ dependency > |
2、整合mybatis
1)建表
2)实体类:使用lombook
1 2 3 4 5 6 7 8 9 10 | @Data @Accessors (chain = true ) @NoArgsConstructor @AllArgsConstructor public class User { private Integer id; private String name; private Integer age; private String email; } |
3)DAO接口:接口抽象:BaseDao
1 2 3 4 5 6 7 8 9 10 | //BaseDao public interface BaseDao<T> { List<T> getAll(); void insert(T t); } //UserDao @Repository public interface UserDao extends BaseDao<User> { } |
4)Mapper配置文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | <! DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> < mapper namespace="com.icucoder.learn.dao.UserDao"> < select id="getAll" resultType="user"> select id, name, age, email from user; </ select > < insert id="insert" parameterType="user"> insert into user values (#{id}, #{name}, #{age}, #{email}); </ insert > </ mapper > |
5)Service接口
1 2 3 4 | public interface UserService { List<User> getAll(); void insert(User t); } |
6)Service接口实现类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | @Service @Transactional public class UserServiceImpl implements UserService { @Autowired UserDao userDao; @Override public List<User> getAll() { return userDao.getAll(); } @Override public void insert(User user) { System.out.println( "insert success" ); userDao.insert(user); int i = 1 / 0 ; } } |
7)配置相关属性
(1)配置dao扫描位置
在入口类上加上注解:@MapperScan("com.icucoder.learn.dao")//指定dao接口扫描位置
1 2 3 4 5 6 7 8 9 | @SpringBootApplication @MapperScan ( "com.icucoder.learn.dao" ) //指定dao接口扫描位置 public class LearnApplication { public static void main(String[] args) { SpringApplication.run(LearnApplication. class , args); } } |
(2)在application.properties中配置数据源和Mapper文件位置
1 2 3 4 5 6 7 8 9 | #数据源 spring.datasource.type=com.alibaba.druid.pool.DruidDataSource spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver spring.datasource.url=jdbc:mysql://127.0.0.1:3306/springboot?characterEncoding=UTF-8&serverTimezone=UTC spring.datasource.username=root spring.datasource.password=xroot #Mapper文件位置 mybatis.mapper-locations=classpath:mapper/*.xml mybatis.type-aliases-package=com.icucoder.learn.entity |
8)编写Controller测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | @RestController @RequestMapping ( "/user" ) public class UserController { @Autowired UserService userService; @RequestMapping ( "/all" ) public List<User> all() { return userService.getAll(); } @RequestMapping ( "/insert" ) public String insertOne() { User user = new User( 7 , "mayun" , 29 , "admin@qq.com" ); userService.insert(user); return "insert success" ; } } |
9)服务器附属并访问测试
http://127.0.0.1:8080/user/all
如果你真心觉得文章写得不错,而且对你有所帮助,那就不妨小小打赏一下吧,如果囊中羞涩,不妨帮忙“推荐"一下,您的“推荐”和”打赏“将是我最大的写作动力!
本文版权归作者所有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接.
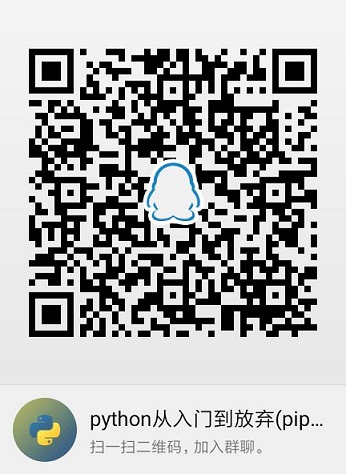
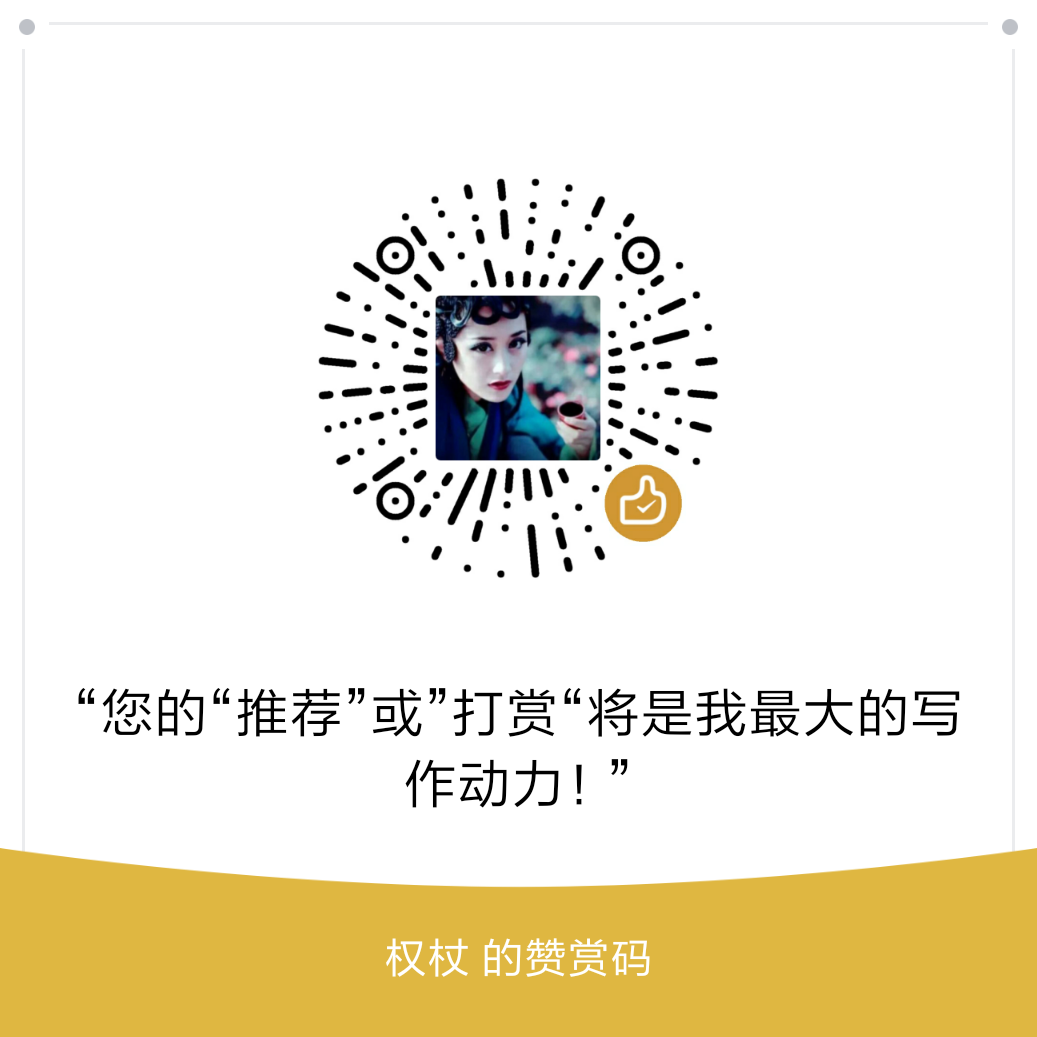
【推荐】编程新体验,更懂你的AI,立即体验豆包MarsCode编程助手
【推荐】凌霞软件回馈社区,博客园 & 1Panel & Halo 联合会员上线
【推荐】抖音旗下AI助手豆包,你的智能百科全书,全免费不限次数
【推荐】博客园社区专享云产品让利特惠,阿里云新客6.5折上折
【推荐】轻量又高性能的 SSH 工具 IShell:AI 加持,快人一步
· 开发中对象命名的一点思考
· .NET Core内存结构体系(Windows环境)底层原理浅谈
· C# 深度学习:对抗生成网络(GAN)训练头像生成模型
· .NET 适配 HarmonyOS 进展
· .NET 进程 stackoverflow异常后,还可以接收 TCP 连接请求吗?
· 本地部署 DeepSeek:小白也能轻松搞定!
· 基于DeepSeek R1 满血版大模型的个人知识库,回答都源自对你专属文件的深度学习。
· 在缓慢中沉淀,在挑战中重生!2024个人总结!
· Tinyfox 简易教程-1:Hello World!
· 大人,时代变了! 赶快把自有业务的本地AI“模型”训练起来!