Vue2入门一:基础概念
vue2.0+入门学习笔记,vue.js下载
1、挂载点、模板、实例之间的关系
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width,initial-scale=1.0"> <title>Vue Learning</title> <script src="./vue.js"></script> </head> <body> <div id="app"> </div> <script> <!--实例--> new Vue({ el:"#app",<!--挂载点--> template:"<h1>xxx{{msg}}</h1>",<!--模板--> data:{ msg:"hello world" } }) </script> </body> </html>
2、数据、事件和方法
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width,initial-scale=1.0"> <title>Vue Learning</title> <script src="./vue.js"></script> </head> <body> <div id="app"> <h1>hello {{msg}}</h1><!--插值表达式{{}}--> <h1 v-text="text_fasle"></h1><!-- v-text 不转义--> <div v-html="html_true"></div><!-- v-html 转义--> <div v-on:click="handleClick">{{content}}</div><!-- 点击事件 v-on:click--> <div @click="handleClick">{{content}}</div><!-- 点击事件 @click --> </div> <script> new Vue({ el:"#app", data:{<!--数据--> msg:"world", text_fasle:"<h1>False</h1>", html_true:"<h1>True</h1>", content:"hello" }, methods:{<!--方法定义--> handleClick:function(){ this.content="world" } } }) </script> </body> </html>
3、属性绑定、双向数据绑定和样式绑定
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width,initial-scale=1.0"> <title>Vue Learning</title> <script src="./vue.js"></script> </head> <body> <div id="app"> <div v-bind:title="title">hello world</div><!-- 属性绑定 v-bind:title --> <div :title="title">hello world</div><!-- 属性绑定 :title --> <input :value="content"/><!-- 单向数据绑定 即上面的属性绑定 --> <br> <input v-model="content"/><!-- 双向数据绑定 v-model --> <div>{{content}}</div> </div> <script> new Vue({ el:"#app", data:{ title:"this is hello world", content:"this is content" } }) </script> </body> </html>
样式绑定:
<!--对象语法--> <div :class="{active:isActive}"></div> <!--数组语法--> <div :class="[activeClass,errorClass]"></div>
4、计算属性和侦听器
(1)为何需要计算属性?
表达式的计算逻辑可能会比较复杂,使用计算属性可以使模板内容更加简洁。
(2)计算属性与方法的区别
计算属性是基于它们的依赖进行缓存的;方法不存在缓存。
(3)侦听器
数据一旦发生变化就通知侦听器所绑定方法。
(4)侦听器的应用场景
数据变化时执行异步或开销较大的操作。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width,initial-scale=1.0"> <title>Vue Learning</title> <script src="./vue.js"></script> </head> <body> <div id="app"> 姓:<input v-model="firstName"/> 名:<input v-model="lastName"/> <div>{{fullName}}</div> <div>{{count}}</div> </div> <script> new Vue({ el:"#app", data:{ firstName:"", lastName:"", count:0 }, computed:{<!-- 计算属性 --> fullName:function(){ return this.firstName+this.lastName } }, watch:{<!-- 侦听器 --> fullName:function(){ this.count++ } } }) </script> </body> </html>
5、过滤器
(1)过滤器的作用是什么?
格式化数据,比如将字符串格式化为首字母大写,将日期格式化为指定的格式等。
(2)自定义过滤器
全局过滤器:
Vue.filter('过滤器名称',function (val) { //过滤器逻辑 })
局部过滤器:
filters:{ capitalize:function () { } }
(3)过滤器使用
<div>{{msg|upper}}</div> <div :id="id|formatId"></div>
例子:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width,initial-scale=1.0"> <title>Vue Learning</title> <script src="./vue.js"></script> </head> <body> <div id="app"> <input type="text" v-model="msg"> <div>{{msg|upper}}</div> <div :abc="msg|upper">测试数据</div> <div>{{msg|lower}}</div> </div> <script> Vue.filter('upper', function (val) { return val.charAt(0).toUpperCase() + val.slice(1) }); new Vue({ el: "#app", data: { msg: '' }, filters: { lower: function (val) { return val.charAt(0).toLowerCase() + val.slice(1) } } }) </script> </body> </html>
6、v-if,v-show与v-for指令
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width,initial-scale=1.0"> <title>Vue Learning</title> <script src="./vue.js"></script> </head> <body> <div id="app"> <div v-if="showd">hello world</div><!-- v-if 判断 值为false直接将元素从dom中删除 --> <div v-show="showd">hello world</div><!-- v-show 判断 值为false将元素从dom中隐藏 --> <button @click="handleClick">toggle</button> <ul> <li v-for="(item,index) of items" :key="index">{{item,index}}</li> <!-- v-for 循环显示数据 其中:key提高渲染效率,唯一 --> </ul> </div> <script> new Vue({ el:"#app", data:{ showd:true, items:[1,2,3] }, methods:{ handleClick:function(){ this.showd=!this.showd } } }) </script> </body> </html>
7、案例:to-do-list开发
输入的内容可以在下面实时显示:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width,initial-scale=1.0"> <title>Vue Learning</title> <script src="./vue.js"></script> </head> <body> <div id="app"> <div> <input v-model="inputValue"/> <button @click="handleClick">提交</button> </div> <ul> <li v-for="(item,index) of itemValues" :key="index">{{item}}</li> </ul> </div> <script> new Vue({ el:"#app", data:{ inputValue:"", itemValues:[] }, methods:{ handleClick:function(){ this.itemValues.push(this.inputValue) this.inputValue="" } } }) </script> </body> </html>
如果你真心觉得文章写得不错,而且对你有所帮助,那就不妨小小打赏一下吧,如果囊中羞涩,不妨帮忙“推荐"一下,您的“推荐”和”打赏“将是我最大的写作动力!
本文版权归作者所有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接.
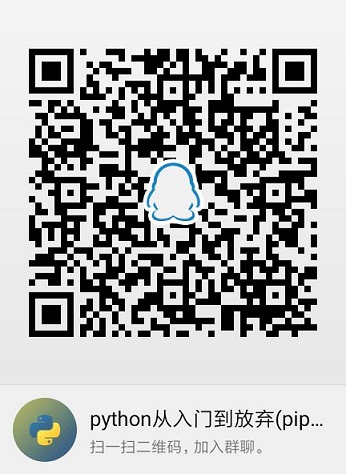
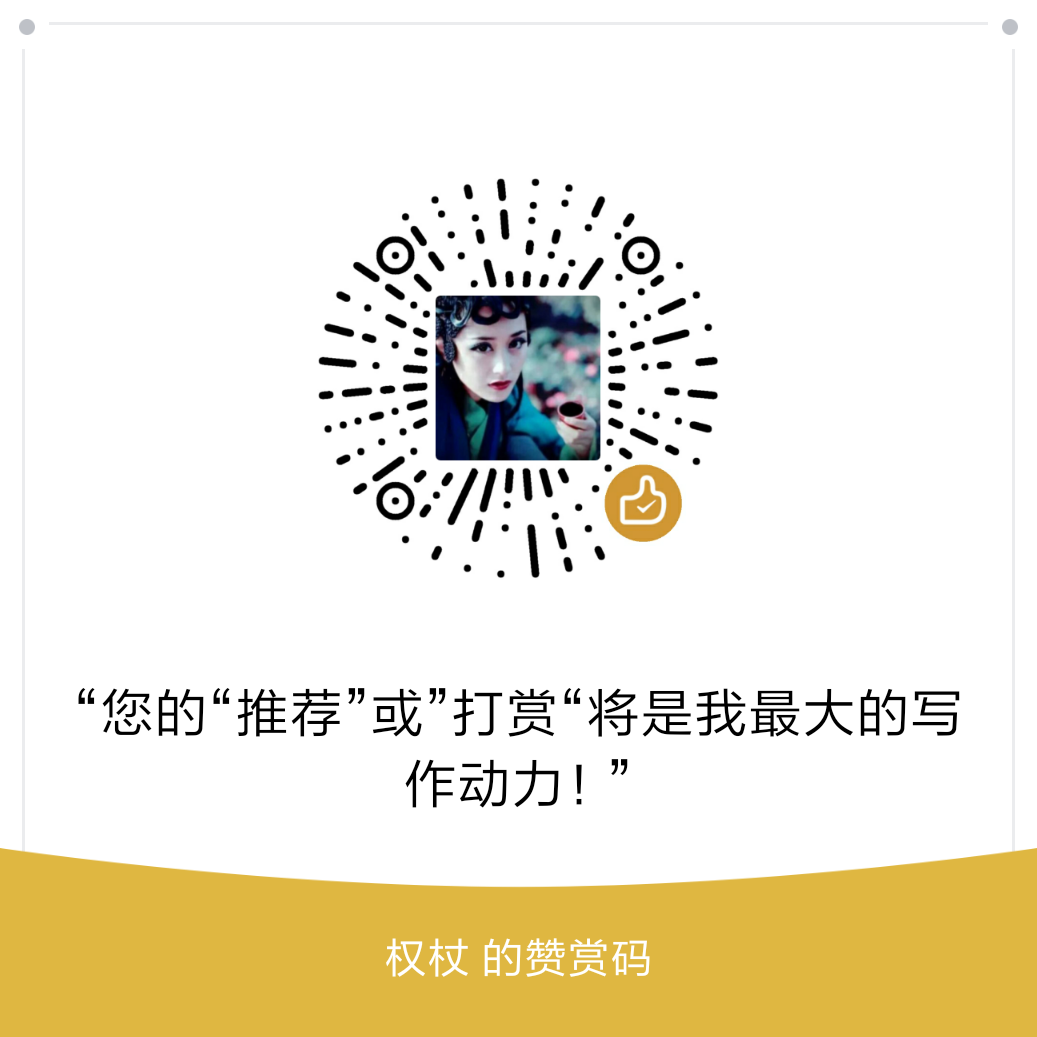