一 通过返回动态内存的指针
#include <iostream>
using namespace std;
int* test(int count)
{
int* p = (int*)malloc(sizeof(int) * count);
if (p)
{
*(p + 0) = 5;
}
return p;
}
int main()
{
int* p = test(3);
*(p + 1) = 6;
*(p + 2) = 7;
for (int i = 0; i < 3; i++)
{
cout << *(p + i) << endl;
}
free(p);
return 0;
}
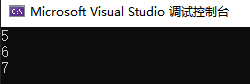
二 通过二级指针来保存
#include <iostream>
using namespace std;
void test(int** p, int count)
{
*p = new int[count];
if (*p)
{
*(*p + 0) = 5;
}
}
int main()
{
int* p = NULL;
test(&p, 3);
*(p + 1) = 6;
*(p + 2) = 7;
for (int i = 0; i < 3; i++)
{
cout << *(p + i) << endl;
}
delete[] p;
return 0;
}
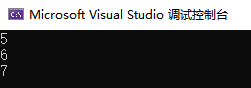