可直接获取链表长度的单链表
SeqList.h文件
#pragma once
//存size的链表
#include<stdio.h>
#include<assert.h>
#include<stdbool.h>
#include<stdlib.h>
typedef int SeqDataType;
typedef struct SeqNode
{
struct SeqNode* next;
SeqDataType val;
}Node;
typedef struct SeqList
{
Node* head;
int sz;
}List;
Node* Buy_Node(SeqDataType x);
void Init(List* phead);
void Push_back(List* phead, SeqDataType x);
void Push_front(List* phead, SeqDataType x);
void Pop_back(List* phead);
void Pop_Front(List* phead);
void Print(List* phead);
void Destroy(List* phead);
void insert_pos(List* phead, Node* pos, SeqDataType x);
void erase_pos(List* phead, Node* pos);
int get_size(List* phead);
bool is_Empty(List* phead);
Node* find(List* phead,SeqDataType x);
SeqList.c文件
#include"SeqList.h"
void Init(List* phead) //初始化头结点和元素个数
{
phead->head = NULL; //头指针指向NULL
phead->sz = 0; //元素个数为0
}
Node* Buy_Node(SeqDataType x) //创建新节点
{
Node* newnode = (Node*)malloc(sizeof(Node));
if (newnode == NULL) //判断内存是否开辟成功
{
perror("malloc fail:"); //输出错误信息
exit(-1);
}
newnode->next = NULL; //新节点的next指向NULL
newnode->val = x; //新节点的值为x
return newnode; //返回新节点
}
void Push_back(List* phead, SeqDataType x) //尾插法
{
assert(phead);
Node* newnode = Buy_Node(x);
Node* cur = phead->head;
if (cur == NULL) //如果是第一个节点
{
phead->head = newnode;
}
else
{
while (cur->next != NULL) //找尾节点
{
cur = cur->next;
} cur->next = newnode; //尾节点的next指向新节点
} phead->sz++; //元素个数+1
}
void Print(List* phead) //打印链表
{
Node* cur = (phead->head);
while (cur != NULL)
{
printf("%d", cur->val);
cur = cur->next;
if (cur != NULL)
{
printf("->");
}
} printf("\n");
}
void Destroy(List* phead) //销毁链表
{
assert(phead);
Node* cur = phead->head;
while (cur != NULL)
{
Node* next = cur->next;
free(cur);
cur = next;
} phead->head = NULL;
phead->sz = 0;
}
int get_size(List* phead) //获取链表大小
{
return phead->sz;
}
bool is_Empty(List* phead) //判断链表是否为空
{
return phead->sz == 0;
}
void Push_front(List* phead, SeqDataType x) //头插法
{
Node* newnode = Buy_Node(x);
if (phead->head == NULL) //链表为空,新节点为头节点
{
phead->head = newnode;
}
else
{
Node** first = &phead->head; //保存头指针地址的指针first
newnode->next = *first; //新节点的next指向原头节点
*first = newnode; //first指向新节点,新节点成为头节点
}
}
void Pop_back(List* phead) //尾部删除
{
assert(phead);
assert(!is_Empty(phead)); //不能再删了
Node* cur = phead->head;
if (cur->next == NULL) //只有一个节点,直接释放
{
free(phead->head);
phead->head = NULL;
}
else
{
Node* prev = phead->head;
while (cur->next != NULL) //找尾节点的前一个节点
{
prev = cur;
cur = cur->next;
}
free(cur); //释放尾节点
cur = NULL;
prev->next = NULL; //尾节点的前一个节点的next指向NULL
}
}
void Pop_Front(List* phead) //头部删除
{
assert(phead != NULL);
assert(!is_Empty(phead));
Node* next = phead->head->next; //保存头节点的next
if (next == NULL) //只有一个节点,直接释放
{
free(phead->head);
phead->head = NULL;
}
else
{
free(phead->head);
phead->head = next; //头指针指向原下一节点,实现删除头节点
}
}
Node* find(List* phead, SeqDataType x) //查找值为x的节点
{
Node* cur = phead->head;
while (cur != NULL)
{
if (cur->val == x) //找到节点
{
return cur;
}
cur = cur->next; //继续向后找
}
return NULL; //找不到
}
//在pos位置之后插入
void insert_pos(List* phead, Node* pos, SeqDataType x)
{
assert(phead);
assert(pos);
if (phead->head == pos) //在第一个节点之后插入
{
Push_front(phead, x);
}
else
{
Node* newnode = Buy_Node(x); //创建新节点
Node* next = pos->next;
pos->next = newnode; //新节点插入到pos节点之后
newnode->next = next;
} phead->sz++;
}
//在pos位置之后删除
void erase_pos(List* phead, Node* pos)
{
assert(phead);
assert(pos);
Node* next = pos->next;
if (pos == phead->head) //删除第一个节点
{
phead->head = next;
free(pos);
pos = NULL;
if (phead->head == NULL) //链表只有一个节点,释放
{
free(phead->head);
phead->head = NULL;
}
}
else
{
Node* prev = phead->head;
while (prev->next != pos) //找pos前一个节点
{
prev = prev->next;
}
prev->next = next; //前一个节点的next指向pos的next
free(pos);
pos = NULL;
}
phead->sz--;
}
test.c文件
#include"SeqList.h"
int main(void)
{
List phead;
Init(&phead);
Push_back(&phead, 1);
Push_back(&phead, 2);
Push_back(&phead, 3);
Push_back(&phead, 4);
Push_back(&phead, 5);
Push_back(&phead, 8);
Push_back(&phead, 10);
Print(&phead);
Push_front(&phead, 6);
Print(&phead);
puts("");
printf("sz= %d\n", get_size(&phead));
Node* find_node = find(&phead, 5);
insert_pos(&phead, find_node, 100);
Print(&phead);
Node* find_node1 = find(&phead, 6);
insert_pos(&phead, find_node1, 123);
Print(&phead);
erase_pos(&phead, find_node);
Print(&phead);
erase_pos(&phead, find_node1);
Print(&phead);
Node* find_node2 = find(&phead, 123);
Node* find_node3 = find(&phead, 10);
erase_pos(&phead, find_node2);
Print(&phead);
erase_pos(&phead, find_node3);
Print(&phead);
return 0;
}
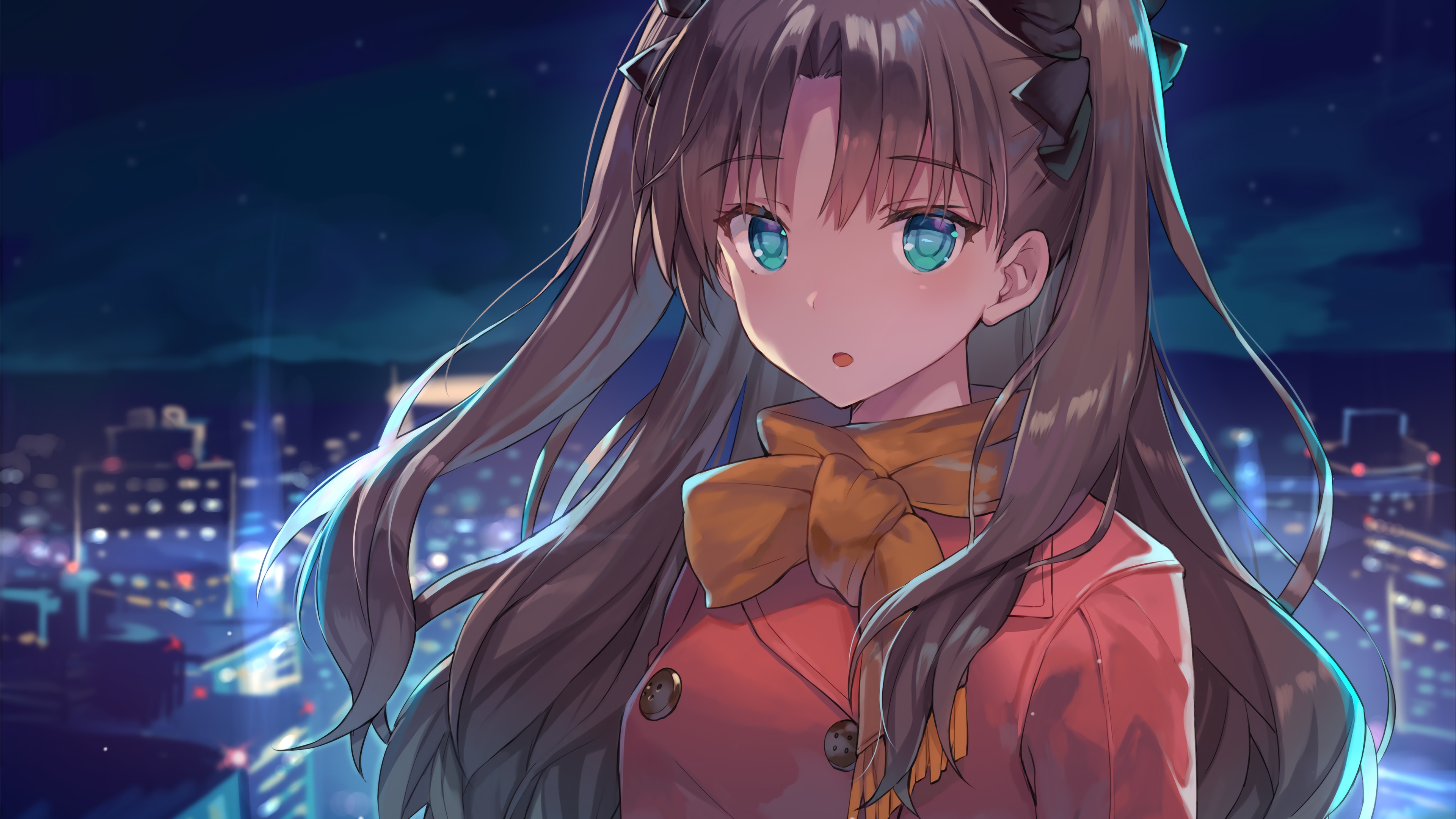