13th week blog
1.Json with Fetch API
1 html { 2 font-family: 'AlexBrush-Regular.ttf', helvetica, arial, sans-serif; 3 } 4 5 body { 6 width: 1000px; 7 margin: 0 auto; 8 background: pink 9 } 10 11 h1, 12 h2 { 13 font-family: 'Quicksand-Bold.otf', cursive; 14 } 15 16 17 /* header styles */ 18 19 h1 { 20 font-size: 4rem; 21 text-align: center; 22 color: red 23 } 24 25 header p { 26 font-size: 5rem; 27 font-weight: bold; 28 text-align: center; 29 } 30 31 32 /* section styles */ 33 34 section article { 35 width: 30%; 36 float: left; 37 } 38 39 section p { 40 margin: 5px 0; 41 } 42 43 h2 { 44 font-size: 2.5rem; 45 letter-spacing: -5px; 46 margin-bottom: 60px; 47 }
1 { 2 "squadName": "Photos Show", 3 "active": true, 4 "members": [{ 5 "name": "海浪绽放", 6 "author": "栖霞仙客", 7 "time": "Summer", 8 "explanation": [ 9 "I like the sea, and I love the wonderful moments when the waves hit the rocks." 10 ] 11 }, 12 { 13 "name": "河坊街夜市", 14 "author": "江南人家", 15 "time": "Autumn", 16 "explanation": [ 17 "The simplicity of the people fascinated me." 18 ] 19 }, 20 { 21 "name": "人间仙境", 22 "author": "时间流逝", 23 "time": "Spring", 24 "explanation": [ 25 "The beauty of the world is numerous." 26 ] 27 } 28 ] 29 }
var header = document.querySelector('header'); var section = document.querySelector('section'); var requestURL = 'https://raw.githubusercontent.com/Bayleee/js/master/photo.json'; //var request = new XMLHttpRequest(); //request.open('GET', requestURL); //request.responseType = 'json'; //request.send(); //request.onload = function() { // var photo = request.response; // populateHeader(photo); // showPhoto(photo); } fetch( reqUrl ).then( Photo => { return handleResponse( photo ) }).then( photo => { populateHeader(photo) showPhoto(photo); } ) function handleResponse( photo ){ let contentType = res.headers.get('content-type') populateHeader( contentType ) if( contentType.includes('application/json' ) ){ populateHeader('-------------数据是json---------------') return photo.json() }else if( contentType.includes('application/json' )){ populateHeader('-------------数据不是json---------------') return photo.text() } } function populateHeader(jsonObj) { var myH1 = document.createElement('h1'); myH1.textContent = jsonObj['squadName']; header.appendChild(myH1); } function showPhoto(jsonObj) { var photo = jsonObj['members']; for (i = 0; i < photo.length; i++) { var myArticle = document.createElement('article'); var myH2 = document.createElement('h2'); var myPara1 = document.createElement('p'); var myPara2 = document.createElement('p'); var myPara3 = document.createElement('p'); var myList = document.createElement('ul'); myH2.textContent = photo[i].name; myPara1.textContent = 'author: ' + photo[i].author; myPara2.textContent = 'time: ' + photo[i].time; myPara3.textContent = 'explanation:' + photo[i].explanation; myArticle.appendChild(myH2); myArticle.appendChild(myPara1); myArticle.appendChild(myPara2); myArticle.appendChild(myPara3); myArticle.appendChild(myList); section.appendChild(myArticle); } }
2.异步与闭包
(1)AsyncCallback:
意义:
异步操作完成时调用的方法
语法1:
构造异步回调对象
AsyncCallback 异步回调对象名asyncCallback = new AsyncCallback(异步操作完成时调用的方法MyAsyncCallback);
语法2:
定义委托,并进行异步调用,异步调用完成后自动触发
委托类型Action fn委托名 = Run委托定义;
委托名fn.BeginInvoke(异步回调对象名asyncCallback );
(来自:https://www.cnblogs.com/licin/p/8274405.html)
(2)Promise:
Promise
对象是一个代理对象(代理一个值),被代理的值在Promise对象创建时可能是未知的。它允许你为异步操作的成功和失败分别绑定相应的处理方法(handlers)。 这让异步方法可以像同步方法那样返回值,但并不是立即返回最终执行结果,而是一个能代表未来出现的结果的promise对象
一个 Promise
有以下几种状态:
- pending: 初始状态,既不是成功,也不是失败状态。
- fulfilled: 意味着操作成功完成。
- rejected: 意味着操作失败。
(3)Fetch:
Fetch API是基于Promise设计
a. fetch api返回的是一个promise对象
b.Options:
- method(String): HTTP请求方法,默认为
GET
- body(String): HTTP的请求参数
- headers(Object): HTTP的请求头,默认为{}
- credentials(String): 默认为
omit
,忽略的意思,也就是不带cookie;还有两个参数,same-origin
,意思就是同源请求带cookie;include
,表示无论跨域还是同源请求都会带cookie
c.第一个then函数里面处理的是response的格式,这里的response具体如下:
- status(number): HTTP返回的状态码,范围在100-599之间
- statusText(String): 服务器返回的状态文字描述,例如
Unauthorized
,上图中返回的是Ok
- ok(Boolean): 如果状态码是以2开头的,则为true
- headers: HTTP请求返回头
- body: 返回体,这里有处理返回体的一些方法
- text(): 将返回体处理成字符串类型
- json(): 返回结果和 JSON.parse(responseText)一样
- blob(): 返回一个Blob,Blob对象是一个不可更改的类文件的二进制数据
- arrayBuffer()
- formData()
(4)Closure:
JS中,在函数内部可以读取函数外部的变量,但在函数外部自然无法读取函数内的局部变量
使用闭包的好处:
-希望一个变量长期驻扎在内存当中;
-避免全局变量的污染;
-私有成员的存在
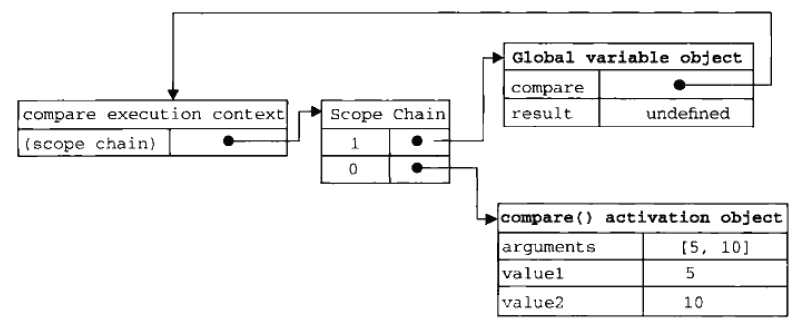
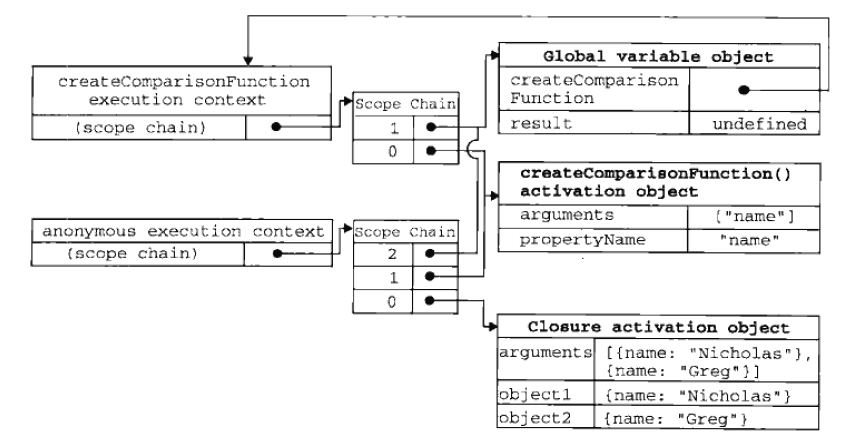
(来自:https://www.cnblogs.com/jingwhale/p/4574792.html)