Android Instrumentation简介
APIs && Source code
Instrumentation 特点
- 该框架基于 JUnit,因此既可以直接使用 Junit 进行测试,也可以使用 Instrumentation 来测试 Android 组件
- 其为 Android 应用的每种组件提供了测试基类
- 可以在 Eclipse 中方便地创建 Android Test Project,并将 Test Case 直接以 Android JUnit 的方式运行
Instrumentation 简单例子
- 新建一个 Instrumentation 的 Android 项目
-
activity_main.xml 内容为:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.xuxu.unittest.MainActivity" >
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello_world" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:text="Button" />
</RelativeLayout>界面效果为:
-
配置 AndroidManifest.xml
-
完成 MainActivity,实现点击
Button
按钮之后TextView
的内容由 Hello world! 变为 Hello android!实现代码如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31package com.xuxu.unittest;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends Activity {
private TextView textView;
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = (TextView) findViewById(R.id.textView);
button = (Button) findViewById(R.id.button);
button.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
textView.setText("Hello android!");
}
});
}
} -
对MainActivity进行测试
- 建立测试类,可以单独建立一个对应的 Android Test Project,也可以就在原项目里面新建测试类,这里选择第二种:
- 右键点击待测项目 > New > Source Folder , 文件夹名 tests
- 右键新建好的 tests 文件夹 > New > Package , 包名取为待测包名 .test , 这里取为
com.xuxu.unittest.test
- 在包里面新建一个 Class:
Name:MainActivityTest
Superclass:android.test.ActivityInstrumentationTestCase2
-
完成 MainActivityTest , 运行测试。右键项目,选择 Run As Android JUnit
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48package com.xuxu.unittest.test;
import com.xuxu.unittest.MainActivity;
import android.test.ActivityInstrumentationTestCase2;
import android.widget.Button;
import android.widget.TextView;
public class MainActivityTest extends ActivityInstrumentationTestCase2<MainActivity> {
private MainActivity mActivity;
private TextView textView;
private Button button;
public MainActivityTest() {
super(MainActivity.class);
}
@Override
protected void setUp() throws Exception {
super.setUp();
mActivity = getActivity();
textView = (TextView) mActivity.findViewById(com.xuxu.unittest.R.id.textView);
button = (Button) mActivity.findViewById(com.xuxu.unittest.R.id.button);
}
//测试初始化条件
public void testInit() {
assertEquals("Hello world!", textView.getText().toString());
}
//测试点击Button之后TextView的值
public void testButton() throws Exception {
//在UI线程中操作
mActivity.runOnUiThread(new Runnable() {
@Override
public void run() {
button.performClick();
}
});
Thread.sleep(500); //加个延时,否则TextView内容还为更改,就已经做断言了
assertEquals("Hello android!", textView.getText().toString());
}
} -
右键项目,选择 Run As Android JUnit
- 建立测试类,可以单独建立一个对应的 Android Test Project,也可以就在原项目里面新建测试类,这里选择第二种:
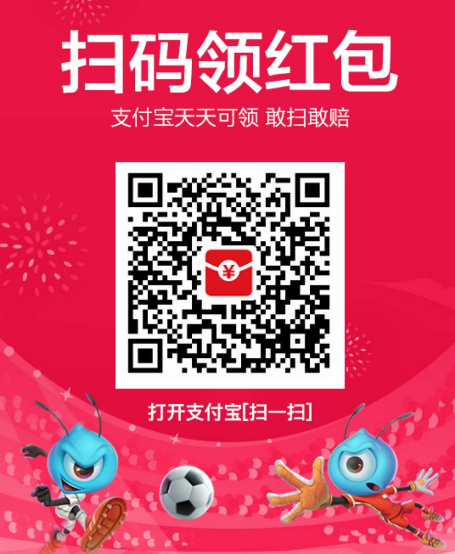
赠人玫瑰
手留余香
我们曾如此渴望命运的波澜,到最后才发现:人生最曼妙的风景,竟是内心的淡定与从容……我们曾如此期盼外界的认可,到最后才知道:世界是自己的,与他人毫无关系!-杨绛先生
如果,您希望更容易地发现我的新博客,不妨点击一下绿色通道的