组件介绍
组件(Component)是 Vue.js 最强大的功能之一。
组件可以扩展 HTML 元素,封装可重用的代码。
组件系统让我们可以用独立可复用的小组件来构建大型应用,几乎任意类型的应用的界面都可以抽象为一个组件树:
注册一个全局组件语法格式如下:
Vue.component(tagName, options)
tagName 为组件名,options 为配置选项。注册后,我们可以使用以下方式来调用组件:
<tagName></tagName>
组件使用
代码如下:
1 <!DOCTYPE html> 2 <html lang="en"> 3 4 <head> 5 <meta charset="UTF-8"> 6 <meta http-equiv="X-UA-Compatible" content="IE=edge"> 7 <meta name="viewport" content="width=device-width, initial-scale=1.0"> 8 <title>Document</title> 9 </head> 10 11 <body> 12 <div id="app"> 13 <button @click="count++">我被点击了 {{count}} 次</button> 14 <!--使用全局组件--> 15 <counter></counter> 16 <counter></counter> 17 <counter></counter> 18 <!--使用局面组件--> 19 <button-counter></button-counter> 20 <button-counter></button-counter> 21 </div> 22 <script src="../node_modules/vue/dist/vue.js"></script> 23 <script> 24 25 // 1、全局声明注册一个组件 26 Vue.component('counter', { 27 template: '<button @click="count++">我被点击了 {{count}} 次, 全局</button>', 28 data() { 29 return { 30 count: 1 31 } 32 } 33 }); 34 35 // 2、局部声明一个组件 36 const buttonCounter = { 37 template: '<button @click="count++">我被点击了 {{count}} 次, 局部</button>', 38 data() { 39 return { 40 count: 1 41 } 42 } 43 } 44 45 const vm = new Vue({ 46 el: '#app', 47 data: { 48 count: 1 49 }, 50 // 注入局面组件 51 components: { 52 'button-counter': buttonCounter 53 } 54 }); 55 </script> 56 </body> 57 58 </html>
效果图
Vue项目组件
1、项目首页index.html

1 <!DOCTYPE html> 2 <html> 3 <head> 4 <meta charset="utf-8"> 5 <meta name="viewport" content="width=device-width,initial-scale=1.0"> 6 <!-- <link rel="stylesheet" href="./static/css/bootstrap.css" > --> 7 <title>vue_demo</title> 8 </head> 9 <body> 10 <div id="app"></div> 11 <!-- built files will be auto injected --> 12 </body> 13 </html>
2、项目主要的js文件 main.js,可以看到js中引入了App组件,并进行了组件映射
1 /** 入口JS:创建Vue实例 */ 2 // 引入VUE 3 import Vue from 'vue' 4 // 引入App组件 5 import App from './App.vue' 6 7 // 固定写法 8 new Vue({ 9 el: '#app', 10 // 组件映射 11 components: { App: App }, 12 template: '<App/>' 13 });
3、App.vue组件内容如下,主要有三个模块(<template>,<script>,<style>)组成,其中引入了HelloWorld组件
<template> <div> <img class="logo" src="./assets/logo.png" alt="logo" /> <!-- 3、使用组件标签 --> <HelloWorld /> </div> </template> <script> // 1、引入组件 import HelloWorld from './components/HelloWorld.vue' // 导出组件 export default { // 2、映射组件标签 components: { HelloWorld: HelloWorld } } </script> <style> .logo { width: 200px; height: 200px; } </style>
4、HelloWorld.vue组件内容如下,主要有三个模块(<template>,<script>,<style>)组成,增加了p标签显示内容
1 <template> 2 <div> 3 <p class="msg">{{msg}}</p> 4 </div> 5 </template> 6 7 <script> 8 9 // 配置对象(与vue一致) 10 export default { 11 // data : {} 12 // 必须写函数,返回一个对象 13 data () { 14 return { 15 msg: 'Hello Vue Component' 16 } 17 } 18 } 19 </script> 20 21 <style> 22 .msg { 23 color: red; 24 font-size: 30px; 25 } 26 </style>
5、效果图如下:
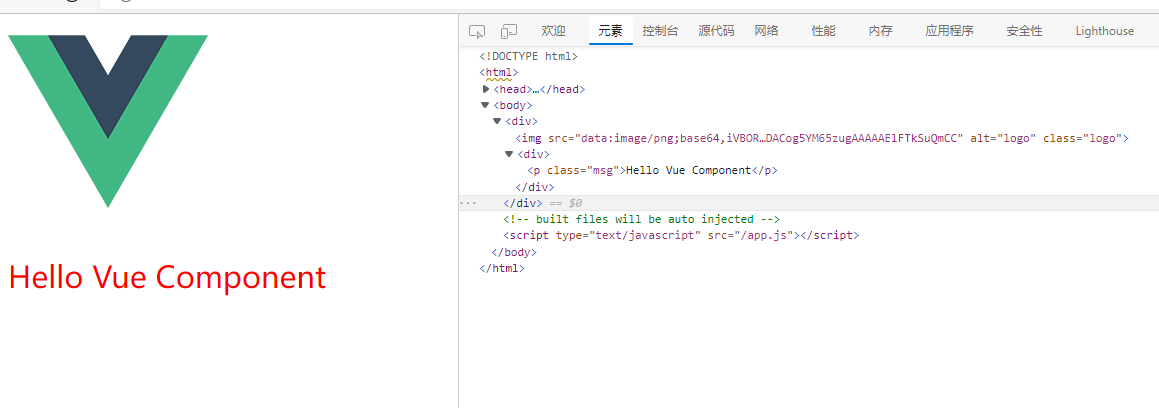
更多内容参考:
1、组件基础 — Vue.js 中文文档 (bootcss.com)
2、Vue.js 组件 | 菜鸟教程 (runoob.com)
3、(25条消息) vue组件_小菜龟儿的博客-CSDN博客_vue组件