【剑指Offer】25、复杂链表的复制
题目描述:
输入一个复杂链表(每个节点中有节点值,以及两个指针,一个指向下一个节点,另一个特殊指针指向任意一个节点),返回结果为复制后复杂链表的head。(注意,输出结果中请不要返回参数中的节点引用,否则判题程序会直接返回空)。
解题思路:
本题有以下三种解法:
第一种:先按照next复制,然后依次添加random指针,添加时需要定位random的位置,定位一次需要一次遍历,需要O(n^2)的复杂度。
第二种:先按照next复制,然后用一个hashmap保存原节点和复制后节点的对应关系,则用O(n)的空间复杂度使时间复杂度降到了O(n)。
第三种(最优方法):同样先按next复制,但是把复制后的节点放到原节点后面,则可以很容易的添加random,最后按照奇偶位置拆成两个链表,时间复杂度O(n),不需要额外空间。
举例:
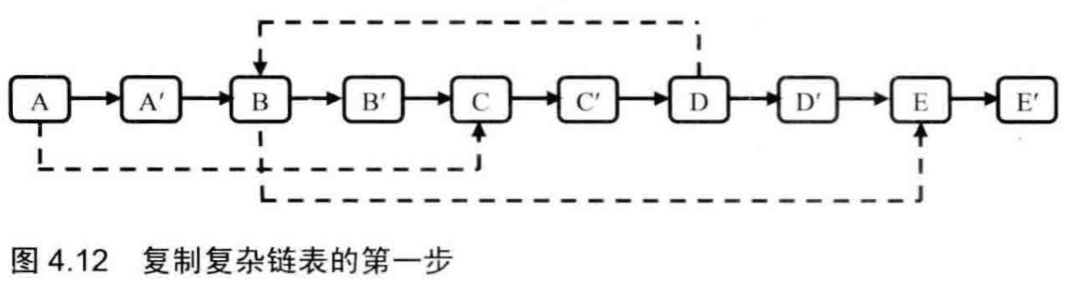
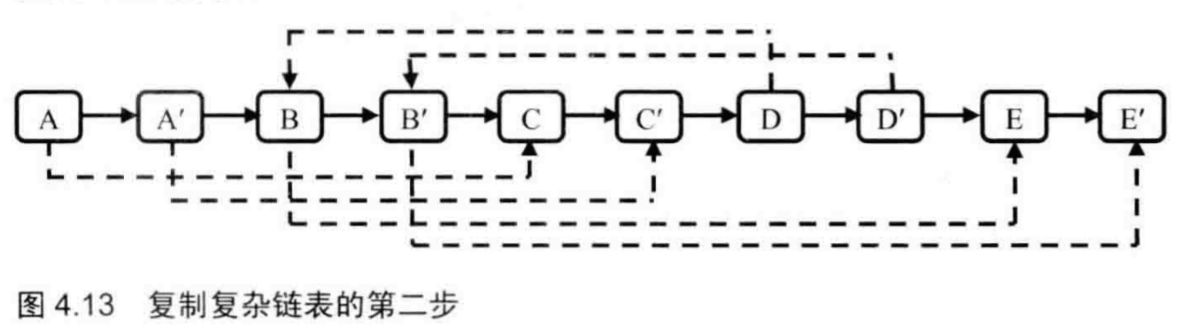
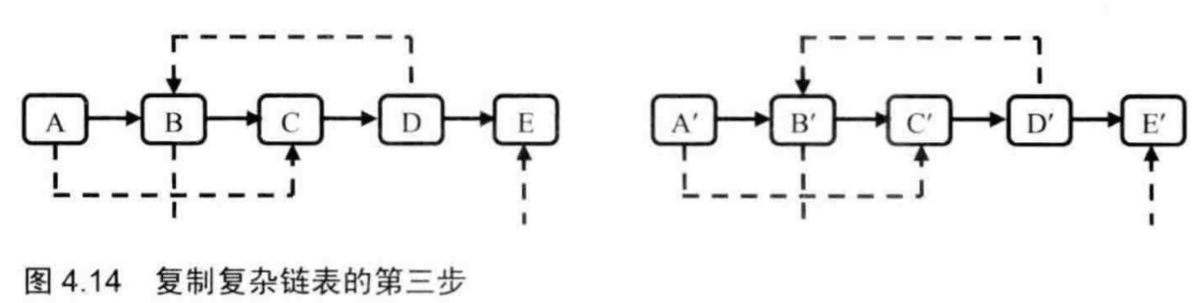
编程实现(Java):
public class Solution {
public RandomListNode Clone(RandomListNode pHead){
if(pHead==null)
return null;
//(1)先按next复制,但是把复制后的节点放到对应原节点后面
CopyNodes(pHead);
//(2)依次添加random指针
addRandom(pHead);
//(3)按照奇偶位置拆成两个链表
return ReconnectNodes(pHead);
}
//先按next复制,但是把复制后的节点放到对应原节点后面
public void CopyNodes(RandomListNode pHead){
RandomListNode head=pHead;
while(head!=null){
RandomListNode temp=new RandomListNode(head.label);
temp.next=head.next; //复制一个结点,插在对应的原节点的后面
temp.random=null;
head.next=temp;
head=temp.next;
}
}
//依次添加random指针
public void addRandom(RandomListNode pHead){
RandomListNode head=pHead;
while(head!=null){
RandomListNode head_new=head.next;
if(head.random!=null)
head_new.random=head.random.next;
head=head_new.next;
}
}
//按照奇偶位置拆成两个链表
public RandomListNode ReconnectNodes(RandomListNode pHead){
if(pHead==null)
return null;
RandomListNode head=pHead;
RandomListNode pHeadClone=head.next;
RandomListNode headClone=pHeadClone;
while(head!=null){
head.next=headClone.next;
head=head.next;
if(head!=null){
headClone.next=head.next;
headClone=headClone.next;
}
}
return pHeadClone;
}
}
博学 审问 慎思 明辨 笃行