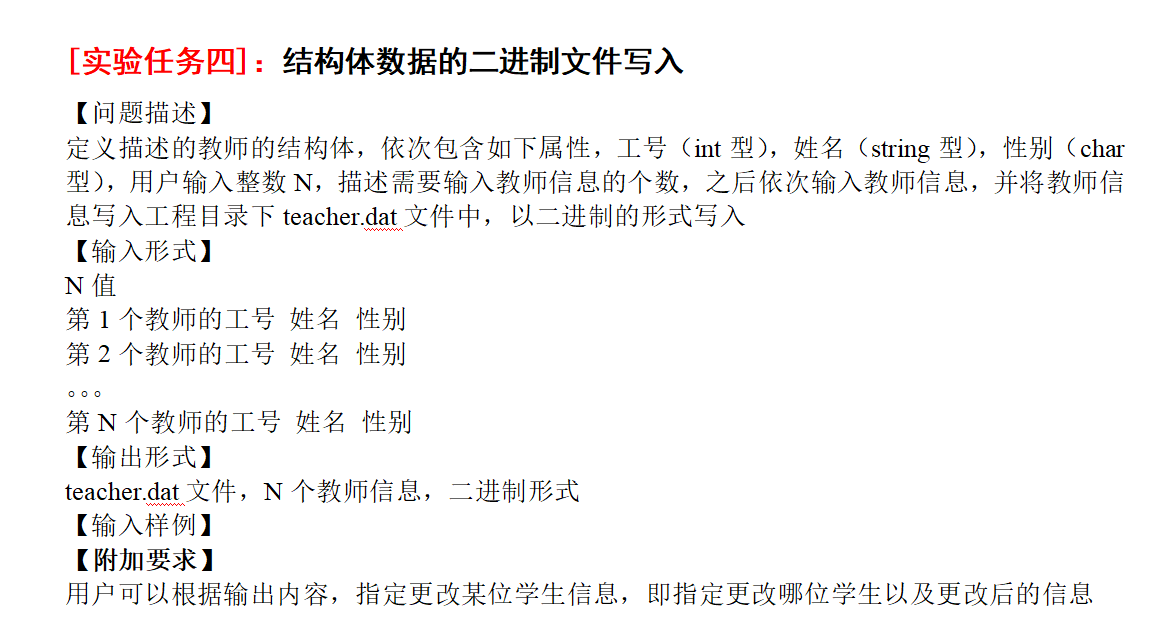
#include <iostream>
#include <fstream>
#include <cstring>
using namespace std;
// 定义教师结构体
struct Teacher {
int id; // 工号
string name; // 姓名
char gender; // 性别
};
int main() {
int N;
cout << "请输入教师信息的个数:";
cin >> N;
// 创建文件输出流
ofstream ofs("teacher.dat", ios::binary);
if (!ofs) {
cerr << "打开文件失败!" << endl;
return -1;
}
// 依次输入教师信息,并写入文件
for (int i = 0; i < N; i++) {
Teacher t;
cout << "请输入第" << i + 1 << "个教师的工号、姓名和性别:";
cin >> t.id >> t.name >> t.gender;
ofs.write((char*)&t, sizeof(Teacher));
}
ofs.close();
// 读取文件内容,验证是否写入成功
ifstream ifs("teacher.dat", ios::binary);
if (!ifs) {
cerr << "打开文件失败!" << endl;
return -1;
}
Teacher t;
while (ifs.read((char*)&t, sizeof(Teacher))) {
cout << "工号:" << t.id << "\t姓名:" << t.name << "\t性别:" << t.gender << endl;
}
ifs.close();
// 修改某位教师的信息
int modifyId;
cout << "请输入要修改的教师的工号:";
cin >> modifyId;
// 创建文件输入流和输出流
ifstream ifs2("teacher.dat", ios::binary);
if (!ifs2) {
cerr << "打开文件失败!" << endl;
return -1;
}
ofstream ofs2("teacher2.dat", ios::binary);
if (!ofs2) {
cerr << "打开文件失败!" << endl;
return -1;
}
while (ifs2.read((char*)&t, sizeof(Teacher))) {
if (t.id == modifyId) { // 如果是要修改的教师,则重新输入信息
cout << "请输入修改后的教师的工号、姓名和性别:";
cin >> t.id >> t.name >> t.gender;
}
ofs2.write((char*)&t, sizeof(Teacher)); // 将教师信息写入新文件
}
ifs2.close();
ofs2.close();
// 删除原文件,并将新文件名改为原文件名
remove("teacher.dat");
rename("teacher2.dat", "teacher.dat");
// 输出修改后的教师信息
ifstream ifs3("teacher.dat", ios::binary);
if (!ifs3) {
cerr << "打开文件失败!" << endl;
return -1;
}
while (ifs3.read((char*)&t, sizeof(Teacher))) {
cout << "工号:" << t.id << "\t姓名:" << t.name << "\t性别:" << t.gender << endl;
}
ifs3.close();
return 0;
}
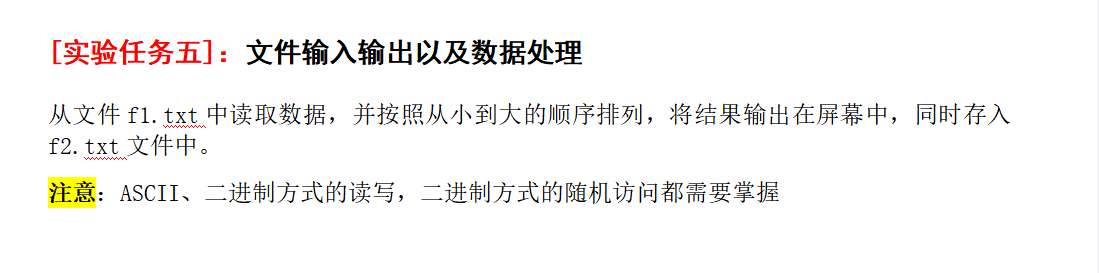
#include <iostream>
#include <fstream>
#include <string>
#include<iostream>
#include<algorithm>
using namespace std;
#include<vector>
//二进制
int main()
{
ifstream ifs("f1.txt", ios::binary);
ofstream ofs("f2.txt", ios::binary);
if (!ifs)
{
cout << "文件打开失败" << endl;
return -1;
}
vector<int> v;
int num;
while (ifs.read((char*)&num, sizeof(int)))
{
v.push_back(num);
}
ifs.close();
sort(v.begin(), v.end());
for (auto i : v)
{
cout << i << ' ';
ofs.write((char*)&i, sizeof(int));
}
ofs.close();
return 0;
}
#include <iostream>
#include <fstream>
#include <string>
#include<iostream>
#include<algorithm>
using namespace std;
#include<vector>
//文本文件
int main()
{
ifstream ifs("f1.txt", ios::in);
ofstream ofs("f2.txt", ios::out);
if (!ifs)
{
cout << "文件打开失败" << endl;
return -1;
}
vector<int> v;
int num;
while (ifs >> num)
{
v.push_back(num);
}
ifs.close();
sort(v.begin(), v.end());
for (int i = 0; i < v.size(); i++)
{
ofs << v[i] << " ";
}
ofs.close();
return 0;
}
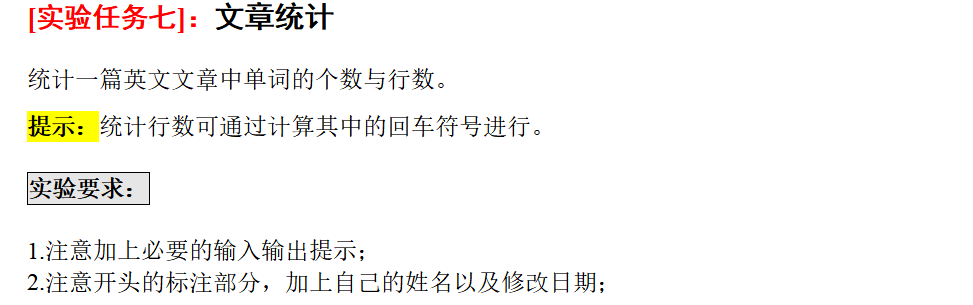
#include <fstream>
#include <string>
#include<iostream>
#include<algorithm>
using namespace std;
#include<vector>
int main()
{
ifstream ifs("mesage.txt", ios::in);
string line;
int linenum=0;
int wordnum = 0;
while (getline(ifs, line)) {
++linenum;
for (int i = 0; i < line.length(); i++)
{
if (line[i] == ' ')
{
++wordnum;
}
}
}
ifs.close();
cout << "单词的数目:" << wordnum << endl;
cout << "行数:" << linenum << endl;
return 0;
}