LeetCode第六天
第六天
30.(219) Contains Duplicate II
JAVA
class Solution {
public boolean containsNearbyDuplicate(int[] nums, int k) {
Map<Integer,Integer> map = new HashMap<Integer,Integer>();
for(int i =0;i<nums.length;i++){
if(map.containsKey(nums[i]))
if(i-map.get(nums[i])<=k)
return true;
else
map.put(nums[i],i);
else
map.put(nums[i],i);
}
return false;
}
}
31.(747) Largest Number At Least Twice of Others
JAVA
class Solution {
public int dominantIndex(int[] nums) {
int maxIndex = 0;
for(int i =0;i<nums.length;i++){
if(nums[i]>nums[maxIndex])
maxIndex = i;
}
for(int i = 0 ;i<nums.length;i++){
if(i!=maxIndex&&nums[maxIndex]<nums[i]*2)
return -1;
}
return maxIndex;
}
}
32.(665) Non-decreasing Array
有点难度
JAVA
class Solution {
public boolean checkPossibility(int[] nums) {
boolean isFirst = true;
for(int i = 0 ;i < nums.length-1;i++){
if(nums[i+1] < nums[i]){
if(isFirst){
if(i == 0){//首位置为较小值
nums[i] = nums[i+1];
}else if(i == nums.length -2){//末尾置为大值
nums[i+1] = nums[i];
}else{//中间位置通过二者左右两边数的值,判断把哪位置为何值。
if(nums[i]<nums[i+2]){
nums[i+1] = nums[i];
}else if(nums[i+1]>nums[i-1]){
nums[i] = nums[i+1];
}else{
return false;
}
}
isFirst = false;
}else{
return false;
}
}
}
return true;
}
}
33.(532) K-diff Pairs in an Array
JAVA
//注意,这里的Map中存放的是 (num,num+k)or(num,null)
//之后计算Map中value有多少个非Null即可;
class Solution {
public int findPairs(int[] nums, int k) {
int pairs = 0;
Map<Integer,Integer> map = new HashMap<Integer,Integer>();
if(nums==null||nums.length == 0||k<0) return 0;
for (int i = 0 ;i<nums.length;i++){
if(map.containsKey(nums[i]-k))
map.put(nums[i]-k,nums[i]);
if(map.containsKey(nums[i]+k))
map.put(nums[i],nums[i]+k);
if(!map.containsKey(nums[i]))
map.put(nums[i],null);
}
for(Integer key : map.keySet()) {
if(map.get(key)!=null)
pairs++;
}
return pairs;
}
}
34.(189) Rotate Array
JAVA
class Solution {
public void rotate(int[] nums, int k) {
k %=nums.length;
reverse(nums,0,nums.length-1);
reverse(nums,0,k-1);
reverse(nums,k,nums.length-1);
}
public void reverse(int[] nums,int start,int end){
while(start<end){
int temp = nums[start];
nums[start] = nums[end];
nums[end] = temp;
start++;
end--;
}
}
}
35.(169) Majority Element
JAVA
class Solution {
public int majorityElement(int[] nums) {
int count = 0;
Integer candidate = null;
for(int num :nums){
if(count == 0)
candidate = num;
count += (num == candidate)?1:-1;
}
return candidate;
}
}
36.(167) Two Sum II - Input array is sorted
JAVA
class Solution {
public int[] twoSum(int[] numbers, int target) {
int start = 0;
int end = numbers.length-1;
while(start<end && numbers[start]+numbers[end] != target){
if(numbers[start]+numbers[end]>target)
end--;
else
start++;
}
return new int[] {start+1,end+1};
}
}
37.(661) Image Smoother
JAVA
class Solution {
public int[][] imageSmoother(int[][] M) {
int R = M.length,C = M[0].length;
int[][] result = new int[R][C];
for(int r = 0 ;r<R;r++)
for(int c = 0;c<C;c++){
int count = 0;
result[r][c] = 0;
for(int nr=r-1;nr <= r+1;nr++)
for(int nc = c-1;nc <= c+1;nc++)
if(nr>=0&&nr<R&&nc>=0&&nc<C){
count++;
result[r][c] +=M[nr][nc];
}
result[r][c] /= count;
}
return result;
}
}
38.(53) Maximum Subarray
动态优化问题!重点,跟上楼梯那道题类似
解题思路
JAVA
class Solution {
public int maxSubArray(int[] nums) {
int n = nums.length;
int[] DP = new int[n];
DP[0] = nums[0];
int maxSum = DP[0];
for(int i = 1;i<nums.length;i++){
DP[i] = nums[i]+(DP[i-1]>0?DP[i-1]:0);
maxSum = Math.max(maxSum,DP[i]);
}
return maxSum;
}
}
39.(697) Degree of an Array
JAVA
class Solution {
public int findShortestSubArray(int[] nums) {
Map<Integer,Integer> left = new HashMap(),right = new HashMap(),count = new HashMap();
for(int i = 0;i<nums.length;i++){
if(left.get(nums[i]) == null) left.put(nums[i],i);
right.put(nums[i],i);
count.put(nums[i],count.getOrDefault(nums[i],0)+1);
}
int degree = Collections.max(count.values());//找出count中value的最大值
int minLength = nums.length;
for(int key : count.keySet()){
if(count.get(key)==degree){
minLength = Math.min(minLength,right.get(key)-left.get(key)+1);
}
}
return minLength;
}
}
作者:郭耀华
出处:http://www.guoyaohua.com
微信:guoyaohua167
邮箱:guo.yaohua@foxmail.com
本文版权归作者和博客园所有,欢迎转载,转载请标明出处。
【如果你觉得本文还不错,对你的学习带来了些许帮助,请帮忙点击右下角的推荐】
出处:http://www.guoyaohua.com
微信:guoyaohua167
邮箱:guo.yaohua@foxmail.com
本文版权归作者和博客园所有,欢迎转载,转载请标明出处。
【如果你觉得本文还不错,对你的学习带来了些许帮助,请帮忙点击右下角的推荐】
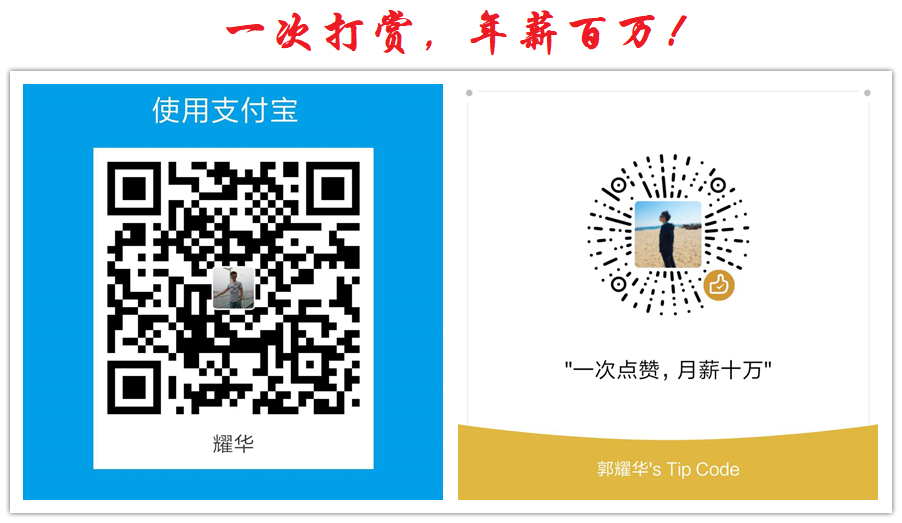