#include <stdio.h> #include <stdlib.h> #include <string.h> struct student //定义一个结构体,存放的是学生成绩档案 { int num; char name[12]; float score; struct student *next; }; typedef struct student STU; //声明STU代表struct student struct student *create(void) //创建学生成绩档案函数 { struct student *head, *tail, *p; //head头指针,tail尾指针。 int num; char name[12]; float score; head = tail = NULL; printf("please input num\n"); scanf("%d", &num); //输入学号 while(num != 0) { printf("please input: name score\n"); scanf("%s %f", name, &score); //输入姓名,成绩 p = (struct student *)malloc(sizeof(struct student)); //给结构体指针p分配空间 p->num = num; //把数据送进结构体 strcpy(p->name, name); p->score=score; p->next = NULL; if(head == NULL) { head = tail = p; } else { tail->next=p; //tail指针随着p后移 tail=p; } printf("please input a num\n"); scanf("%d", &num); } return head; } struct student *readfp(void) //从文件读入 { struct student *head, *tail, *p, new; int num; char name[12]; float score; head = tail = NULL; FILE *fp; fp = fopen("save.dat","r"); fread(&new, sizeof(STU),1, fp); while(!feof(fp)) //判断文件是否处于文件结束位置,如文件结束,则返回值为1,否则为0。一般当该函数返回值为1时,结束对文件的读取操作 { p = (struct student *)malloc(sizeof(struct student)); p->num = new.num; strcpy(p->name, new.name); p->score= new.score; p->next = NULL; if(head == NULL) { head = tail = p; } else { tail->next=p; tail=p; } fread(&new, sizeof(STU),1, fp); } return head; } show(struct student *head) //显示学生的档案 { struct student *p; p = head; while(p!=NULL) { printf("num=%d, name=%s, score=%f\n", p->num, p->name, p->score); p = p->next; } } struct student* insert(struct student *head) //插入学生的档案 { struct student *new1, *p, *q; int num; char name[12]; float score; p = head; new1 = (struct student *)malloc(sizeof(struct student)); printf("please input a num\n"); scanf("%d", &new1->num); printf("please input: name score\n"); scanf("%s %f", new1->name, &new1->score); //输入新学生的档案 if(p == NULL) { head = new1; new1->next=NULL; } else { while(p->num <new1->num && p->next!=NULL) //P指向的档案编号小于要插入的学生档案的编号时,p向后移动一位 { q=p; p=p->next; } if(p->num >= new1->num) { if(p == head) { head=new1; new1->next=p; } else //此时要插入的档案的位置在q和p之间 { q->next=new1; new1->next=p; } } else //要插入的学生档案编号比已有编号都大,因此放在最后面 { p->next = new1; new1->next = NULL; } } return head; } struct student *delete(struct student *head) //删除一条学生档案信息 { struct student *p, *q; int num; p=head; printf("please input data to delete\n"); scanf("%d", &num); //while(p->data!=x && p!=NULL ) while(p != NULL && p->num != num ) { q=p; p=p->next; } if(p==NULL) { printf("have not the data\n"); } else { if(p==head) { head=p->next; free(p); } else { q->next=p->next; free(p); } } return head; } void save(STU *head) //将学生档案保存到文件中 { FILE *fp; STU *p; fp=fopen("save.dat", "w"); p = head; while(p != NULL) { fwrite(p, sizeof(STU), 1, fp); p=p->next; } fclose(fp); } int main() { STU *head=NULL; int x; while(1) { printf("|-----Menu-------|\n"); printf("|--1: Create-----|\n"); printf("|--2: insert-----|\n"); printf("|--3: delete-----|\n"); printf("|--4: save -----|\n"); printf("|--5: show ------|\n"); printf("|--6: readfp-----|\n"); printf("|--0: exit -----|\n"); printf("please input choice\n"); scanf("%d", &x); switch(x) { case 1: head = create(); break; case 2: head = insert(head); break; case 3: head = delete(head); break; case 4: save(head); break; case 5: show(head); break; case 6: head = readfp(); break; case 0: printf("bye bye\n"); return 0; default : printf("invaild choice\n"); break; } getchar(); } }
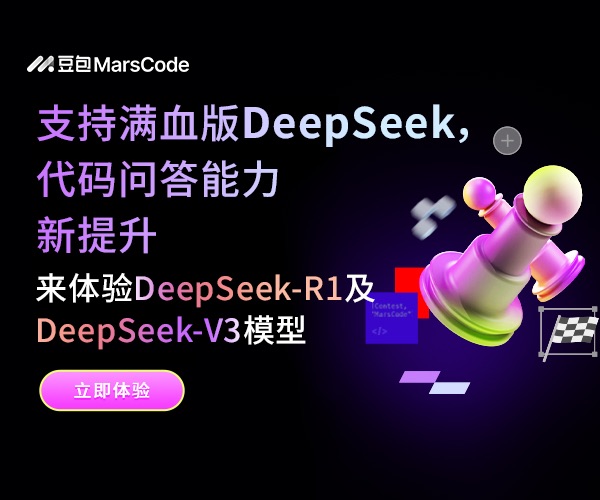