Silverlight学习笔记三(鼠标点击动态画直线|动态设置Ellipse的Canvas.Top与Canvas.Left|动态设置Stroke属性的方法。)
我有可能需要把AutoCAD的部分功能搬到浏览器上,先练习一下画直线的方法。如图: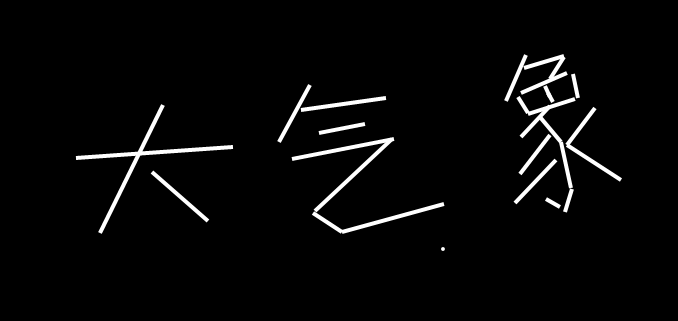
MainPage.xaml代码:
大气象
大气象
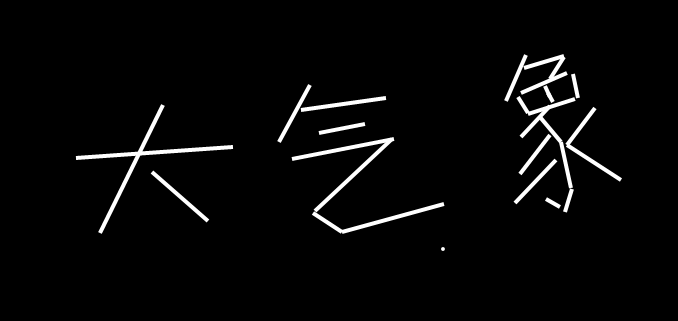
MainPage.xaml代码:

<UserControl x:Class="DrawLine.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d" d:DesignWidth="640" d:DesignHeight="480">
<Canvas x:Name="panel" Background="Black" MouseLeftButtonDown="panel_MouseLeftButtonDown">
</Canvas>
</UserControl>
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d" d:DesignWidth="640" d:DesignHeight="480">
<Canvas x:Name="panel" Background="Black" MouseLeftButtonDown="panel_MouseLeftButtonDown">
</Canvas>
</UserControl>
后台:

using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace DrawLine
{
public partial class MainPage : UserControl
{
public MainPage()
{
InitializeComponent();
}
private bool IsStart = true;//是否是起点。
private double pX1 = 0, pY1 = 0;//起点坐标
private double pX2 = 0, pY2 = 0;//终点坐标
Ellipse ellipse = new Ellipse();//画起点
private void panel_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
Point p = e.GetPosition(sender as FrameworkElement);//取得鼠标点下的位置
Canvas pnl = sender as Canvas;
if (IsStart)
{
pX1 = p.X;//设置起点坐标
pY1 = p.Y;
}
else
{
pX2 = p.X;//设置终点坐标
pY2 = p.Y;
}
if (IsStart)//如果是起点,则画起点。
{
DrawPoint(pnl);
}
else
{
DrawOneLine(pnl);
}
IsStart = !IsStart;
}
//画点
private void DrawPoint(Canvas pnl)
{
ellipse.Stroke = new SolidColorBrush(Color.FromArgb(255, 255, 255, 255));//动态设置Stroke属性的方法。
ellipse.StrokeThickness = 2;
ellipse.Width = 4;
ellipse.Height = 4;
Canvas.SetLeft(ellipse, pX1);//动态设置Ellipse的Canvas.Top与Canvas.Left
Canvas.SetTop(ellipse,pY1);
pnl.Children.Add(ellipse);
}
//画直线
private void DrawOneLine(Canvas pnl)
{
Line line = new Line();
line.X1 = pX1;
line.Y1 = pY1;
line.X2 = pX2;
line.Y2 = pY2;
line.Stroke = new SolidColorBrush(Color.FromArgb(255, 255, 255, 255));
line.StrokeThickness = 4;
pnl.Children.Add(line);
pnl.Children.Remove(ellipse);//删除起点。
}
/*参考代码,感谢小刚http://www.cnblogs.com/xiaogangqq123/
public MainPage()
{
InitializeComponent();
this.MouseLeftButtonDown += new MouseButtonEventHandler(MainPage_MouseLeftButtonDown);
this.MouseLeftButtonUp += new MouseButtonEventHandler(MainPage_MouseLeftButtonUp);
}
private Point pointStart;
private Path path;
private LineGeometry lg;
//private Point pointEnd;
private bool IsFist = true;//记录是否第一次按下鼠标
void MainPage_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
//第二次点下鼠标
if (!IsFist)
{
path = new Path();
path.Stroke = new SolidColorBrush(Colors.Red);
path.StrokeThickness = 5;
lg = new LineGeometry();
lg.StartPoint = pointStart;
lg.StartPoint = pointStart;
path.Data = lg;
lg.EndPoint = e.GetPosition(LayoutRoot);
LayoutRoot.Children.Add(path);
}
pointStart = e.GetPosition(LayoutRoot);
IsFist = true;
}
void MainPage_MouseLeftButtonUp(object sender, MouseButtonEventArgs e)
{
IsFist = false;
}
*/
}
}
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace DrawLine
{
public partial class MainPage : UserControl
{
public MainPage()
{
InitializeComponent();
}
private bool IsStart = true;//是否是起点。
private double pX1 = 0, pY1 = 0;//起点坐标
private double pX2 = 0, pY2 = 0;//终点坐标
Ellipse ellipse = new Ellipse();//画起点
private void panel_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
Point p = e.GetPosition(sender as FrameworkElement);//取得鼠标点下的位置
Canvas pnl = sender as Canvas;
if (IsStart)
{
pX1 = p.X;//设置起点坐标
pY1 = p.Y;
}
else
{
pX2 = p.X;//设置终点坐标
pY2 = p.Y;
}
if (IsStart)//如果是起点,则画起点。
{
DrawPoint(pnl);
}
else
{
DrawOneLine(pnl);
}
IsStart = !IsStart;
}
//画点
private void DrawPoint(Canvas pnl)
{
ellipse.Stroke = new SolidColorBrush(Color.FromArgb(255, 255, 255, 255));//动态设置Stroke属性的方法。
ellipse.StrokeThickness = 2;
ellipse.Width = 4;
ellipse.Height = 4;
Canvas.SetLeft(ellipse, pX1);//动态设置Ellipse的Canvas.Top与Canvas.Left
Canvas.SetTop(ellipse,pY1);
pnl.Children.Add(ellipse);
}
//画直线
private void DrawOneLine(Canvas pnl)
{
Line line = new Line();
line.X1 = pX1;
line.Y1 = pY1;
line.X2 = pX2;
line.Y2 = pY2;
line.Stroke = new SolidColorBrush(Color.FromArgb(255, 255, 255, 255));
line.StrokeThickness = 4;
pnl.Children.Add(line);
pnl.Children.Remove(ellipse);//删除起点。
}
/*参考代码,感谢小刚http://www.cnblogs.com/xiaogangqq123/
public MainPage()
{
InitializeComponent();
this.MouseLeftButtonDown += new MouseButtonEventHandler(MainPage_MouseLeftButtonDown);
this.MouseLeftButtonUp += new MouseButtonEventHandler(MainPage_MouseLeftButtonUp);
}
private Point pointStart;
private Path path;
private LineGeometry lg;
//private Point pointEnd;
private bool IsFist = true;//记录是否第一次按下鼠标
void MainPage_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
//第二次点下鼠标
if (!IsFist)
{
path = new Path();
path.Stroke = new SolidColorBrush(Colors.Red);
path.StrokeThickness = 5;
lg = new LineGeometry();
lg.StartPoint = pointStart;
lg.StartPoint = pointStart;
path.Data = lg;
lg.EndPoint = e.GetPosition(LayoutRoot);
LayoutRoot.Children.Add(path);
}
pointStart = e.GetPosition(LayoutRoot);
IsFist = true;
}
void MainPage_MouseLeftButtonUp(object sender, MouseButtonEventArgs e)
{
IsFist = false;
}
*/
}
}
实例源码:https://files.cnblogs.com/greatverve/DrawLine.rar
环境是Silverlight 3
我这个博客废弃不用了,今天想寻找外链的时候,突然想到这个博客权重很高。
有需要免费外链的,留言即可,我准备把这个博客变成免费的友情链接站点。