C#.NET中的定制异常(Exception)
参照MSDN的异常定制
/*
* Created by SharpDevelop.
* User: noo
* Date: 2009-8-17
* Time: 18:18
*
* 定制异常
*/
using System;
class NotEvenException : Exception//定制的异常要继承自System.Exception类
{
const string str1 = "函数所需的参数是偶数。";
public NotEvenException() :
base( str1 )//不带参数的构造函数,继承自基类的构造函数
{ }
public NotEvenException( string str2 ) :
base( String.Format( "{0} - {1}",str2, str1 ) )//带参数的构造函数,继承自基类的构造函数
{ }
}
class NewSExceptionDemo
{
public static void Main()
{
Console.WriteLine ("\n现在,一个异常通过基类的构造函数被抛出\n");
CalcHalf( 18 );
CalcHalf( 21 );
Console.WriteLine ("\n现在,一个异常通过派生类的构造函数被抛出\n");
CalcHalf2( 30 );
CalcHalf2( 33 );
}
//如果input参数不是偶数,Half函数就跑出一个基类异常
static int Half( int input )
{
if( input % 2 != 0 )
throw new Exception( String.Format("参数 {0} 不能被2整除。", input ) );
else return input / 2;
}
//如果input参数不是偶数,Half2函数就跑出一个派生类异常
static int Half2( int input )
{
if( input % 2 != 0 )
throw new NotEvenException(
String.Format( "无效参数:{0}", input ) );
else return input / 2;
}
// CalcHalf方法调用Half方法,并捕捉任何抛出的异常
static void CalcHalf(int input )
{
try
{
int halfInput = Half( input );
Console.WriteLine(
"{0}的一半为{1}.", input, halfInput );
}
catch( Exception ex )
{
Console.WriteLine( ex.ToString( ) );
}
}
// CalcHalf2方法调用Half2方法,并捕捉任何抛出的异常
static void CalcHalf2(int input )
{
try
{
int halfInput = Half2( input );
Console.WriteLine(
"{0}的一半是{1}.", input, halfInput );
}
catch( Exception ex )
{
Console.WriteLine( ex.ToString( ) );
}
}
}
* Created by SharpDevelop.
* User: noo
* Date: 2009-8-17
* Time: 18:18
*
* 定制异常
*/
using System;
class NotEvenException : Exception//定制的异常要继承自System.Exception类
{
const string str1 = "函数所需的参数是偶数。";
public NotEvenException() :
base( str1 )//不带参数的构造函数,继承自基类的构造函数
{ }
public NotEvenException( string str2 ) :
base( String.Format( "{0} - {1}",str2, str1 ) )//带参数的构造函数,继承自基类的构造函数
{ }
}
class NewSExceptionDemo
{
public static void Main()
{
Console.WriteLine ("\n现在,一个异常通过基类的构造函数被抛出\n");
CalcHalf( 18 );
CalcHalf( 21 );
Console.WriteLine ("\n现在,一个异常通过派生类的构造函数被抛出\n");
CalcHalf2( 30 );
CalcHalf2( 33 );
}
//如果input参数不是偶数,Half函数就跑出一个基类异常
static int Half( int input )
{
if( input % 2 != 0 )
throw new Exception( String.Format("参数 {0} 不能被2整除。", input ) );
else return input / 2;
}
//如果input参数不是偶数,Half2函数就跑出一个派生类异常
static int Half2( int input )
{
if( input % 2 != 0 )
throw new NotEvenException(
String.Format( "无效参数:{0}", input ) );
else return input / 2;
}
// CalcHalf方法调用Half方法,并捕捉任何抛出的异常
static void CalcHalf(int input )
{
try
{
int halfInput = Half( input );
Console.WriteLine(
"{0}的一半为{1}.", input, halfInput );
}
catch( Exception ex )
{
Console.WriteLine( ex.ToString( ) );
}
}
// CalcHalf2方法调用Half2方法,并捕捉任何抛出的异常
static void CalcHalf2(int input )
{
try
{
int halfInput = Half2( input );
Console.WriteLine(
"{0}的一半是{1}.", input, halfInput );
}
catch( Exception ex )
{
Console.WriteLine( ex.ToString( ) );
}
}
}
运行结果为:
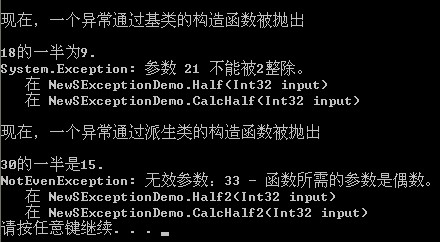