daper
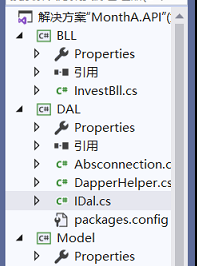

-------------------------------------------------------------数据访问层 using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using Dapper; using DataModel; using System.Data.SqlClient; using System.Data; namespace GoodsDAL { public class SpDal { string conStr = "Data Source=.;Initial Catalog=ExamDay;Integrated Security=True"; /// <summary> /// 获取购物车里的所有数据 /// </summary> /// <returns></returns> public List<SpGouWuCarModel> SpGouWus() { List<SpGouWuCarModel> spGous = new List<SpGouWuCarModel>(); using (IDbConnection conn = new SqlConnection(conStr)) { spGous = conn.Query<SpGouWuCarModel>("select g.GId,S.Sname,s.SMiao,s.Sprice from GouWuche g join ShangPins s on g.GSId = s.SId").ToList(); } return spGous; } /// <summary> /// 购物车的删除功能 /// </summary> /// <param name="Deid"></param> /// <returns></returns> public int DeleSp(string Deid) { using (IDbConnection conn = new SqlConnection(conStr)) { return conn.Execute($"delete from GouWuche where GId in({Deid})"); } } /// <summary> /// 获取收货人地址列表 /// </summary> /// <returns></returns> public List<DiZhiModel> DiZhis() { List<DiZhiModel> dis = new List<DiZhiModel>(); using (IDbConnection conn = new SqlConnection(conStr)) { dis = conn.Query<DiZhiModel>("select * from SpCity").ToList(); } return dis; } /// <summary> /// 收货人地址的添加 /// </summary> /// <param name="Dizhi"></param> /// <returns></returns> public int AddDizhi(DiZhiModel Dizhi) { try { using (IDbConnection conn = new SqlConnection(conStr)) { string sql = string.Format($"insert into SpCity values('{Dizhi.CChengShi}','{Dizhi.CXiangXi}','{Dizhi.Cname}','{Dizhi.Cnumber}')"); return conn.Execute(sql); } } catch (Exception) { throw; } } /// <summary> /// 订单的提交方法 /// </summary> /// <param name="dingDan"></param> /// <returns></returns> public int AddDingDan(DingDanInfo dingDan) { using (IDbConnection conn = new SqlConnection(conStr)) { string sql = string.Format($"insert into DingDans values('{dingDan.DSpId}','{dingDan.Dchengshi}','{dingDan.Dxiangxi}','{dingDan.Dname}','{dingDan.Dnumber}')"); return conn.Execute(sql); } } } } -----------------------------------------------------API控制器 using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Http; using System.Web.Http; using DataModel; using GoodsDAL; namespace WeekThreeExam_API.Controllers { public class XiaDanController : ApiController { SpDal dal = new SpDal(); /// <summary> /// 获取购物车里的所有数据 /// </summary> /// <returns></returns> [HttpGet] [Route("api/GetSpGwc")] public List<SpGouWuCarModel> SpGouWus() { return dal.SpGouWus(); } /// <summary> /// 购物车的删除功能 /// </summary> /// <param name="Deid"></param> /// <returns></returns> [HttpGet] [Route("api/Spdelete")] public int DeleSp(string Deid) { return dal.DeleSp(Deid); } /// <summary> /// 获取收货人地址列表 /// </summary> /// <returns></returns> [HttpGet] [Route("api/GetDizhi")] public List<DiZhiModel> DiZhis() { return dal.DiZhis(); } /// <summary> /// 收货人地址的添加 /// </summary> /// <param name="Dizhi"></param> /// <returns></returns> [HttpPost] [Route("api/AddSpGwc")] public int AddDizhi(DiZhiModel Dizhi) { return dal.AddDizhi(Dizhi); } /// <summary> /// 订单的提交方法 /// </summary> /// <param name="dingDan"></param> /// <returns></returns> [HttpPost] [Route("api/AddDingDan")] public int AddDingDan(DingDanInfo dingDan) { return dal.AddDingDan(dingDan); } } }

-------------------------显示购物车 <!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title></title> </head> <body> <link href="../Content/bootstrap.css" rel="stylesheet" /> <script src="../Scripts/jquery-3.3.1.js"></script> <table class="table table-bordered"> <thead> <tr> <th><input id="All_check" type="checkbox" />全选/<input id="Fan_Check" type="checkbox" />反选</th> <th>商品名称</th> <th>商品描述</th> <th>商品价格</th> <th>操作</th> </tr> </thead> <tbody id="Spbody"></tbody> </table> <div> 总计<b><span style="color:red" id="Money"></span>元</b><br /> <input id="Button1" type="button" class="btn btn-info" onclick="location.href = '/GoodsGouMai/Order.html'" value="去结算" /> </div> <script> $(function () { GetGuwc(); }) //全选 $("#All_check").click(function () { $(".Choose").prop("checked", this.checked); }) //反选 $("#Fan_Check").click(function () { $(".Choose").each(function () { this.checked = !this.checked; }) }) //选中价格 function SMoney(price) { var SumPric = Number($("#Money").html()); //if (this.checked = !this.checked) { // SumPric = SumPric - price; //} //if (this.checked = this.checked) { // SumPric = SumPric + price; //} //if ($("#Check").attr("checked", false)) { // SumPric = SumPric - price; //} if ($("#Check").attr("checked", "checked")) { SumPric = SumPric + price; } $("#Money").html(SumPric); sessionStorage["SumMoney"] = Number($("#Money").html()); sessionStorage["SpId"] = $("#Hidden1").val(); console.log(sessionStorage["SpId"]); } function GetGuwc() { $.ajax({ url: "http://localhost:51232/api/GetSpGwc", datatype: "json", type: "get", success: function (i) { $("#Spbody").empty(); $(i).each(function () { var line = `<tr> <td><input id="Check" onclick="SMoney(${this.Sprice})" class="Choose" type="checkbox" /><input id="Hidden1" value="${this.SId}" type="hidden" /></td> <td>${this.Sname}</td> <td>${this.SMiao}</td> <td>${this.Sprice}</td> <td><input id="Button1" type="button" onclick="DeleteSp(${this.GId})" value="删除" /></td> </tr>`; $("#Spbody").append(line); }) } }) } function DeleteSp(Did) { $.ajax({ url: "http://localhost:51232/api/Spdelete", type: "get", data: { Deid: Did }, datatype: "josn", success: function (i) { if (i > 0) { alert("删除成功"); } else { alert("删除失败!"); } } }) } </script> </body> </html> -------------------------------------提交订单 <!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title></title> </head> <body> <link href="../Content/bootstrap.css" rel="stylesheet" /> <script src="../Scripts/jquery-3.3.1.js"></script> <table class="table table-bordered"> <tr> <td>收获地址</td> <td> </td> <td> </td> <td><input id="Button1" type="button" onclick="AddDi()" value="添加地址" /></td> </tr> <tbody id="Dizhi"></tbody> </table> <table class="table table-bordered"> <tr> <td>支付方式</td> </tr> <tr> <td> <input id="Checkbox1" type="checkbox" />微信支付 </td> </tr> <tr> <td> <input id="Checkbox1" type="checkbox" />支付宝支付 </td> </tr> </table> <table> <tr> <td>订单结算</td> </tr> <tr> <td>总计<span style="color:red" id="ZongJia"></span></td> </tr> <tr> <td><input id="Button1" class="btn btn-info" type="button" onclick="AddDingDan()" value="提交订单" /></td> </tr> </table> <script> var Price = sessionStorage["SumMoney"]; $(function () { $("#ZongJia").html(Price); Dizhi(); }) function DefaultCheck(id) { $(".Choose").each(function () { if (this.value == id) { $(".Choose").prop("checked", "checked"); } }) } function Dizhi() { $.ajax({ url: "http://localhost:51232/api/GetDizhi", dataType: "json", type: "get", success: function (i) { $("#Dizhi").empty(); $(i).each(function () { var line = `<tr> <td><input id="CheckDizhi" class="Choose" value="${this.CId}" type="checkbox" /></td> <td>${this.CChengShi}</td> <td>${this.CXiangXi}</td> <td>${this.Cname}</td> <td>${this.Cnumber}</td> </tr>`; $("#Dizhi").append(line); }) } }) } function AddDi() { $("#Dizhi").empty(); var line = ` <tr> <td>选择地区:</td> <td> <select id="Select1"> <option>海淀区五环</option> <option>朝阳区三环</option> </select> </td> </tr> <tr> <td>详细地址</td> <td><input id="XiangXi" type="text" /></td> </tr> <tr> <td>收货人:</td> <td><input id="ShouHuoRen" type="text" /></td> </tr> <tr> <td>手机</td> <td><input id="Number" type="text" /></td> </tr> <tr> <td><input id="Button1" class="btn btn-info" type="button" onclick="QueRen(${this.CId})" value="确认收货地址" /></td> </tr>`; $("#Dizhi").append(line); } function QueRen(id) { var obj = {}; obj.CChengShi = $("#Select1").val(); obj.CXiangXi = $("#XiangXi").val(); obj.Cname = $("#ShouHuoRen").val(); obj.Cnumber = $("#Number").val(); $.ajax({ url: "http://localhost:51232/api/AddSpGwc", dataType: "json", data: obj, type: "post", success: function (i) { if (i > 0) { alert("保存成功"); Dizhi(); DefaultCheck(id); } else { alert("保存失败!") } } }) } function AddDingDan() { var obj = {}; obj.CId = sessionStorage["SpId"]; obj.CChengShi = "上海"; obj.CXiangXi = "奉贤区海湾镇"; obj.Cname = "王五"; obj.Cnumber = "18898567424"; $.ajax({ url: "http://localhost:51232/api/AddDingDan", dataType: "json", data: obj, type: "post", success: function (i) { if (i > 0) { alert("提交成功"); location.href = '/GoodsGouMai/Car.html'; } else { alert("保存失败") } } }) } </script> </body> </html>
---------------------------------------------------------dal
-----------------------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
namespace DAL
{
public abstract class Absconnection
{
private static string con = ConfigurationManager.ConnectionStrings["con"].ConnectionString;
/// <summary>
/// 数据库连接
/// </summary>
/// <returns></returns>
public static IDbConnection dbConnection()
{
IDbConnection connection = new SqlConnection(con);
if (connection.State == ConnectionState.Closed)
{
connection.Open();
}
return connection;
}
}
}
-----------------------------------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data;
using System.Data.SqlClient;
using Dapper;
namespace DAL
{
public class DapperHelper : Absconnection, IDal
{
IDbConnection conn = Absconnection.dbConnection();
/// <summary>
/// 增删改
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="sql"></param>
/// <returns></returns>
public int Excout<T>(string sql) where T : class, new()
{
return conn.Execute(sql);
}
/// <summary>
/// 查询
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="sql"></param>
/// <returns></returns>
public List<T> Query<T>(string sql) where T : class, new()
{
return conn.Query<T>(sql).ToList();
}
}
}
---------------------------------------------------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DAL
{
public interface IDal
{
/// <summary>
/// 查询显示
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="sql"></param>
/// <returns></returns>
List<T> Query<T>(string sql) where T : class, new();
/// <summary>
/// 增删改
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="sql"></param>
/// <returns></returns>
int Excout<T>(string sql) where T : class, new();
}
}
---------------------------------------------------------------------------------------------------------------
bll----------------------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Model;
using DAL;
namespace BLL
{
public class InvestBll
{
DapperHelper helper = new DapperHelper();
/// <summary>
/// 查询投资项目信息
/// </summary>
/// <returns></returns>
public List<Invest> InvestQuery()
{
string sql = "select * from Invest";
return helper.Query<Invest>(sql);
}
/// <summary>
/// 投资项目详情
/// </summary>
/// <returns></returns>
public List<AllDetial> InvestDetial()
{
string sql = "select b.DId,a.IId,a.IName,a.ISy,b.Ddate,b.DSMoney,b.DJd,a.IMoney,b.Dfs,b.DMs,b.DPj from invest a left join InvestDetial b on a.IId = b.IId";
return helper.Query<AllDetial>(sql);
}
/// <summary>
/// 用户信息
/// </summary>
/// <returns></returns>
public List<UserInfo> GetUsers()
{
string sql = "select * from UserInfo";
return helper.Query<UserInfo>(sql);
}
/// <summary>
/// 投资记录表
/// </summary>
/// <returns></returns>
public List<InvestJLLS> JLShow()
{
string sql = "select b.UName,b.UMoney,a.Times,a.IId from InvestJL a left join UserInfo b on a.UId = b.UId";
return helper.Query<InvestJLLS>(sql);
}
/// <summary>
/// 添加到数据库
/// </summary>
/// <param name="model"></param>
/// <returns></returns>
public int Insert(TouZi model)
{
string sql = string.Format("insert into TouZi values('{0}','{1}','{2}','{3}','{4}')",model.IName,model.SyMoney,model.UMoney,model.Nsylv,model.DateTimes);
return helper.Excout<TouZi>(sql);
}
}
}
---------------------------------------------------------------------------------------------------------
------------------------------控制器
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
using BLL;
using Model;
using ServiceStack.Redis;
namespace MonthA.API.Controllers
{
public class InvestController : ApiController
{
InvestBll bll = new InvestBll();
RedisClient client = new RedisClient("127.0.0.1", 6379);
/// <summary>
/// 投资信息分页查询显示
/// </summary>
/// <param name="pageindex"></param>
/// <param name="pagesize"></param>
/// <param name="IName"></param>
/// <returns></returns>
[HttpGet]
[Route("api/InvestQuery")]
public InvestPage InvestQueryShow(int pageindex = 1, int pagesize = 2, string IName = "")
{
if (pageindex < 1)
{
pageindex = 1;
}
var list = bll.InvestQuery();
if (!string.IsNullOrEmpty(IName))
{
list = list.Where(s => s.IName.Contains(IName)).ToList();
}
int count = list.Count;
int pagecount;
if (count % pagesize != 0)
{
pagecount = count / pagesize + 1;
}
else
{
pagecount = count / pagesize;
}
list = list.Skip((pageindex - 1) * pagesize).Take(pagesize).ToList();
if (pagesize > pagecount)
{
pagesize = pagecount;
}
var slist = new InvestPage();
slist.pagelist = list;
slist.pageindex = pageindex;
slist.pagesize = pagesize;
slist.pagecount = pagecount;
slist.count = count;
return slist;
}
/// <summary>
/// 投资项目详情信息展示
/// </summary>
/// <param name="IId"></param>
/// <returns></returns>
[HttpGet]
[Route("api/DetialShow")]
public List<AllDetial> ShowDetial(int IId)
{
var list = bll.InvestDetial();
if (IId != 0)
{
list = list.Where(s => s.IId.Equals(IId)).ToList();
}
return list;
}
/// <summary>
/// 获取用户信息
/// </summary>
/// <param name="UId"></param>
/// <returns></returns>
[HttpGet]
[Route("api/UserShow")]
public List<UserInfo> UserShow(int UId)
{
var list = bll.GetUsers();
if (UId != 0)
{
list = list.Where(s => s.UId.Equals(UId)).ToList();
}
return list;
}
/// <summary>
/// 根据该投资项目Id查询出所有的投资记录
/// </summary>
/// <param name="IId"></param>
/// <returns></returns>
[HttpGet]
[Route("api/JLShow")]
public JLPage jlPageSHow(int IId,int pageindex = 1, int pagesize = 1)
{
if (pageindex < 1)
{
pageindex = 1;
}
var list = bll.JLShow();
if (IId != 0)
{
list = list.Where(s => s.IId.Equals(IId)).ToList();
}
int count = list.Count;
int pagecount;
if (count % pagesize != 0)
{
pagecount = count / pagesize + 1;
}
else
{
pagecount = count / pagesize;
}
list = list.Skip((pageindex - 1) * pagesize).Take(pagesize).ToList();
if (pagesize > pagecount)
{
pagesize = pagecount;
}
var slist = new JLPage();
slist.pagelist = list;
slist.pageindex = pageindex;
slist.pagesize = pagesize;
slist.pagecount = pagecount;
slist.count = count;
return slist;
}
/// <summary>
/// 加入redis
/// </summary>
/// <param name="model"></param>
/// <returns></returns>
[HttpPost]
[Route("api/SetRedis")]
public int SetRedisData(TouZi model)
{
if (client.Set<TouZi>("model", model))
{
return 1;
}
else
{
return 0;
}
}
/// <summary>
/// 取出Redis
/// </summary>
/// <returns></returns>
[HttpGet]
[Route("api/GetRedis")]
public TouZi GetRedis()
{
//异常处理
try
{
TouZi model = client.Get<TouZi>("model");
return model;
}
catch (Exception ex)
{
throw;
}
}
/// <summary>
/// 添加
/// </summary>
/// <param name="model"></param>
/// <returns></returns>
[HttpPost]
[Route("api/Insert")]
public int Insert(TouZi model)
{
return bll.Insert(model);
}
}
}
0-------------------------------------------------------------------
-----------------------------------------mvc控制器
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MonthA.MVC.Controllers
{
public class InvestController : Controller
{
// GET: Invest
public ActionResult Index()
{
return View();
}
public ActionResult InvestShow()
{
return View();
}
public ActionResult ShowDetials(int IId)
{
ViewBag.IId = IId;
return View();
}
public ActionResult RedisShow()
{
return View();
}
public ActionResult Shows()
{
return View();
}
}
}
-------------------------------------------------------
视图
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>InvestShow</title>
<link href="~/Content/bootstrap.css" rel="stylesheet" />
<script src="~/Scripts/jquery-3.3.1.js"></script>
<script>
//文档就绪
$(function () {
Show(1);
})
var pageindex = 1;
var pagesize = 2;
//投资页面显示
function Show(page) {
var obj = {
pageindex: page,
pagesize: pagesize,
IName: $("#IName").val()
};
$.ajax({
url: "http://localhost:53226/api/InvestQuery",
data: obj,
dataType: "json",
type: "get",
success: function (res) {
var str = "";
var strzt = "";
$("#pagecount").text(res.pagecount);
$("#count").text(res.count);
$("#pageindex").text(res.pageindex);
pageindex = res.pageindex;
$(res.pagelist).each(function (i, n) {
if (n.Izt == 1) {
strzt = "投资中";
}
else {
strzt = "已完成";
}
str += "<tr>" +
"<td>" + n.ITime + "</td>" +
"<td>" + n.IName + "</td>" +
"<td>" + n.IMoney + "</td>" +
"<td>" + n.ISy + "%</td>" +
"<td>" + strzt + "</td>" +
"<td><input onclick='ShowDetial(" + n.IId + ")' type='button' value='查看详情' /></td>" +
"</tr>";
})
$("#tb tr:gt(0)").remove();
$("#tb").append(str);
}
})
}
//跳转详情页面
function ShowDetial(IId) {
location.href = "/Invest/ShowDetials?IId=" + IId;
}
/**/</script>
</head>
<body>
<div>
<input id="IName" type="text" /><input id="Button1" type="button" value="查询" />
<table id="tb" class="table">
<tr>
<td>时间</td>
<td>标的名称</td>
<td>投资金额</td>
<td>年化收益率</td>
<td>当前状态</td>
<td>查看详情</td>
</tr>
</table>
<table>
<tr>
<td>
<input onclick="Show(pageindex-1)" type="button" value="上一页" />
共<span id="pagecount"></span>页
共<span id="count"></span>条数据
第<span id="pageindex"></span>页
<input onclick="Show(pageindex+1)" type="button" value="下一页" />
</td>
</tr>
</table>
</div>
</body>
</html>
-----------------------------
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>RedisShow</title>
<script src="~/Scripts/jquery-3.3.1.js"></script>
<script>
$(function () {
Show();
})
//从redeis取出信息
function Show() {
$.ajax({
url: "http://localhost:53226/api/GetRedis",
dataType: "json",
async: false,
type: "get",
success: function (res) {
$(res).each(function (i, n) {
$("#IName").text(n.IName);
$("#SyMoney").text(n.SyMoney);
$("#UMoney").text(n.UMoney);
$("#Nsylv").text(n.Nsylv);
$("#DateTimes").text(n.DateTimes);
})
}
})
}
//减redis信息添加到数据库
function Sure() {
var obj = {
IName: $("#IName").text(),
SyMoney: $("#SyMoney").text(),
UMoney: $("#UMoney").text(),
Nsylv: $("#Nsylv").text(),
DateTimes: $("#DateTimes").text()
};
$.ajax({
url: "http://localhost:53226/api/Insert",
data: obj,
dataType: "json",
type: "post",
success: function (res) {
if (res > 0) {
location.href = "/Invest/Shows";
}
}
})
}
/**/</script>
</head>
<body>
<div>
<table>
<tr>
<td>
<table>
<tr>
<td>
<span id="IName"></span>
</td>
</tr>
<tr>
<td>
收益金额:
<span id="SyMoney" style="color:red">元</span>
</td>
</tr>
<tr>
<td>
投资金额:
<span id="UMoney" style="color:red">元</span>
</td>
</tr>
<tr>
<td>
年化利率:
<span id="Nsylv" style="color:red">%</span>
</td>
</tr>
<tr>
<td>
回款日期:
<span id="DateTimes" style="color:red"></span>
</td>
</tr>
<tr>
<td><input onclick="Sure()" type="button" value="确定" /></td>
</tr>
</table>
</td>
</tr>
</table>
</div>
</body>
</html>
------------------------------------------
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
.apply-record {
width: 400px;
}
.apply-record .tab {
height: 60px;
line-height: 60px;
}
.apply-record .tab .tab-item {
list-style: none;
display: inline-block;
width: 100px;
text-align: center;
font-size: 20px;
}
.apply-record .tab .tab-item.active {
color: #3F86FF;
border-bottom: 3px solid #0000FF;
}
.products .mainCont {
display: none;
width: 100%;
overflow: auto;
text-align: center;
}
.products .mainCont.selected {
display: block;
}
</style>
<title>ShowDetials</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<script type="text/javascript" charset="utf-8"></script>
<link href="~/Content/bootstrap.css" rel="stylesheet" />
<script src="~/Scripts/jquery-3.3.1.js"></script>
<script>
$(function () {
DetialShow();
JLShowQuery(1);
UserShow();
})
//详情展示
function DetialShow() {
$.ajax({
url: "http://localhost:53226/api/DetialShow",
data: {IId:@ViewBag.IId},
dataType: "json",
async: false,
type: "get",
success: function (res) {
$(res).each(function (i,n) {
$("#IName").text(n.IName);
$("#ISy").text(n.ISy);
$("#Ddate").text(n.Ddate);
$("#DSMoney").text(n.DSMoney);
$("#DJd").text(n.DJd);
$("#DPj").text(n.DPj);
$("#IMoney").text(n.IMoney);
$("#Dfs").text(n.Dfs);
$("#Ms").text(n.DMs);
})
}
})
}
///查询用户信息
function UserShow() {
$.ajax({
url: "http://localhost:53226/api/UserShow",
data: {UId:1},
dataType: "json",
async: false,
type: "get",
success: function (res) {
$(res).each(function (i, n) {
$("#UName").val(n.UName);
$("#UMoney").text(n.UMoney);
$("#UId").val(n.UId);
})
}
})
}
var pageindex = 1;
var pagesize = 2;
///分页记录
function JLShowQuery(page) {
var obj = {
IId:@ViewBag.IId,
pageindex: page,
pagesize: pagesize,
};
$.ajax({
url: "http://localhost:53226/api/JLShow",
data: obj,
dataType: "json",
async: false,
type: "get",
success: function (res) {
var str = "";
pageindex = res.pageindex;
$(res.pagelist).each(function (i, n) {
str += "<tr>" +
"<td>" + n.UName + "</td>" +
"<td>" + n.UMoney + "</td>" +
"<td>" + n.Times + "</td>" +
"</tr>";
})
$("#tb tr:gt(0)").remove();
$("#tb").append(str);
}
})
}
//投资加入redis
function TouZi() {
var Money = $("#Money").val();
var UMoney = $("#UMoney").text();
var ISy = $("#ISy").text();
if (parseInt(Money) > parseInt(UMoney)) {
alert("帐户余额不足!");
return;
}
$("#sy").text(parseInt(Money) * parseInt(ISy)/100);
var obj = {
IName: $("#IName").text(),
SyMoney: $("#sy").text(),
UMoney: $("#UMoney").text(),
Nsylv: $("#ISy").text(),
DateTimes: "2020-07-10"
};
$.ajax({
url: "http://localhost:53226/api/SetRedis",
data: obj,
dataType: "json",
type: "post",
success: function (res) {
if (res > 0) {
location.href = "/Invest/RedisShow";
}
}
})
}
</script>
</head>
<body>
<div>
<input id="UName" type="hidden" />
<input id="UId" type="hidden" />
<table>
<tr>
<td>项目名称:<span id="IName" style="color:red"></span></td>
</tr>
<tr>
<td>年化利率:<span id="ISy" style="color:red"></span></td>
<td>投资期限:<span id="Ddate" style="color:red"></span></td>
<td>剩余可投资金额:<span id="DSMoney" style="color:red"></span>元</td>
</tr>
<tr>
<td>当前进度:<span id="DJd"></span>%</td>
</tr>
<tr>
<td>安全评级:<span id="DPj"></span></td>
</tr>
<tr>
<td>项目总金额:<span id="IMoney"></span></td>
</tr>
<tr>
<td>收益方式:<span id="Dfs"></span></td>
</tr>
</table>
<br />
<table>
<tr>
<td>当前可用余额:<span id="UMoney"></span>元</td>
</tr>
<tr>
<td><input id="Money" type="text" />元</td>
</tr>
<tr>
<td>预计收益:<span id="sy"></span>元</td>
</tr>
<tr>
<td>
<input onclick="TouZi()" type="button" value="立即投资" />
</td>
</tr>
</table>
</div>
<div class="apply-record">
<ul class="tab">
<li class="tab-item active">项目人介绍</li>
<li class="tab-item">借款人信息</li>
<li class="tab-item">投资记录</li>
</ul>
<div class="content products">
<div class="mainCont selected">
<table>
<tr>
<td>
<span id="Ms"></span>
</td>
</tr>
</table>
</div>
<div class="mainCont">
借款人信息
</div>
<div class="mainCont">
<table id="tb">
<tr>
<td>投资人</td>
<td>投资金额</td>
<td>投资时间</td>
</tr>
</table>
<table>
<tr>
<td>
<input onclick="JLShowQuery(pageindex-1)" type="button" value="上一页" />
<input onclick="JLShowQuery(pageindex+1)" type="button" value="下一页" />
</td>
</tr>
</table>
</div>
<div class="mainCont">
</div>
</div>
</div>
<script type="text/javascript">
$(function () {
$(".apply-record .tab .tab-item").click(function () {
$(this).addClass("active").siblings().removeClass("active");
$(".products .mainCont").eq($(this).index()).show().siblings().hide();
})
})
</script>
</body>
</html>
--------------------------
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<div id="box">
恭喜您投资成功!
</div>
<script>
//5秒跳转页面
var b = document.getElementById("box");
b.innerHTML = "5秒跳转页面";
var time = 5;
//每隔一秒执行定时器
var timer = setInterval(function () {
if (time > 0) {
time--;
b.innerHTML = time + "秒钟跳转页面";
} else {//终止定时器
//alert("跳转页面");
//open("http://www.baidu.com");
window.location.href = "/Invest/InvestShow";
timer.clearInterval();
}
}, 1000);
</script>
</body>
</html>

DAL--------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Data.SqlClient; using System.Data; using DataModel; using Newtonsoft.Json; namespace EFDAL { public class XueYuanClass { WlWcontext ConText = new WlWcontext(); /// <summary> /// 数据显示连表联查 /// </summary> /// <returns></returns> public List<WuLianWangInfo> WlWInfos(string name = "") { var list = ConText.WlWtext.Include("JueseId").ToList(); if (!string.IsNullOrEmpty(name)) { list = list.Where(s => s.Name.Contains(name)).ToList(); } return list; } /// <summary> /// 删除 /// </summary> /// <param name="Did"></param> /// <returns></returns> public int DeleInfo(int Did) { var deid = ConText.WlWtext.Find(Did); ConText.WlWtext.Remove(deid); return ConText.SaveChanges(); } /// <summary> /// 修改数据 /// </summary> /// <param name="wuLianWang"></param> /// <returns></returns> public int UpdateInfo(WuLianWangInfo wuLianWang) { ConText.WlWtext.Attach(wuLianWang); ConText.Entry(wuLianWang).State = System.Data.Entity.EntityState.Modified; return ConText.SaveChanges(); } /// <summary> /// 根据Id获取数据 /// </summary> /// <param name="Id"></param> /// <returns></returns> public WuLianWangInfo FanTian(int Id) { return ConText.WlWtext.Find(Id); } /// <summary> /// 添加数据 /// </summary> /// <param name="WuLian"></param> /// <returns></returns> public int AddInfo(WuLianWangInfo WuLian) { ConText.WlWtext.Add(WuLian); return ConText.SaveChanges(); } /// <summary> /// 调用分页存储过程 /// </summary> /// <param name="Pageindex"></param> /// <param name="Pagesize"></param> /// <returns></returns> public List<WuLianWangInfo> WPageProc(int Pageindex = 1,int Pagesize = 2) { var list = new List<WuLianWangInfo>(); int Pcount = 0; SqlParameter Index = new SqlParameter("@PageIndex", Pageindex); Index.Direction = ParameterDirection.Input; SqlParameter Size = new SqlParameter("@PageSize", Pagesize); Size.Direction = ParameterDirection.Input; SqlParameter Count = new SqlParameter("@PageCount", Pcount); Count.Direction = ParameterDirection.Output; ConText.WlWtext.SqlQuery("exec Proc_Page", new SqlParameter[] { Index, Size, Count }); return list; } } } --------------------------------------------------------- 控制器 using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Http; using System.Web.Http; using EFDAL; using DataModel; using ServiceStack.Redis; namespace EFExame_API.Controllers { public class WuLianWangController : ApiController { XueYuanClass dal = new XueYuanClass(); /// <summary> /// 获取数据并存到redis里面 /// </summary> /// <returns></returns> [HttpGet] [Route("api/GetWuLianWang")] public List<WuLianWangInfo> WlWinfos(string name = "") { //定义变量list接受返回的集合 var list = new List<WuLianWangInfo>(); //定义redis RedisClient redis = new RedisClient("127.0.0.1"); //将Redis赋值给list list = redis.Get<List<WuLianWangInfo>>("products"); //判断Redis是否为空或者他的个数是否为0 if (list == null || list.Count == 0) { list = dal.WlWInfos(name); redis.Set("products", list); } return list; } /// <summary> /// 删除数据 /// </summary> /// <param name="id"></param> /// <returns></returns> [HttpGet] [Route("api/DeleInfo")] public int DeletId(int id) { return dal.DeleInfo(id); } /// <summary> /// 修改数据 /// </summary> /// <param name="wuLianWang"></param> /// <returns></returns> [HttpPost] [Route("api/UpdateInfo")] public int UpdateInfo(WuLianWangInfo wuLianWang) { return dal.UpdateInfo(wuLianWang); } /// <summary> /// 根据Id获取数据 /// </summary> /// <param name="Id"></param> /// <returns></returns> [HttpGet] [Route("api/GetId")] public WuLianWangInfo FanTian(int Id) { return dal.FanTian(Id); } /// <summary> /// 添加数据 /// </summary> /// <param name="WuLian"></param> /// <returns></returns> [HttpPost] [Route("api/AddInfo")] public int AddInfo(WuLianWangInfo WuLian) { return dal.AddInfo(WuLian); } /// <summary> /// 调用分页存储过程 /// </summary> /// <param name="Pageindex"></param> /// <param name="Pagesize"></param> /// <returns></returns> [HttpPost] [Route("api/PageProc")] public List<WuLianWangInfo> WPageProc(int Pageindex = 1, int Pagesize = 2) { return dal.WPageProc(Pageindex, Pagesize); } } }-------------------------------------------- 数据库 insert into [dbo].[JueSe] values('学生'),('老师') select * from [JueSe] insert into [dbo].[WuLianWangInfo] values('3','小明','6417教室','1712A班级') insert into [dbo].[WuLianWangInfo] values('4','旺旺对','6417教室','1712A班级') insert into [dbo].[WuLianWangInfo] values('3','小强','6417教室','1712A班级') select * from [WuLianWangInfo] create proc Proc_Page @PageIndex int, @PageSize int, @Count int output as begin begin tran commit tran select @Count = COUNT(*) from [WuLianWangInfo] declare @PageCount int if(@PageIndex < 1) begin set @PageIndex = 1 end if(@PageCount % @PageSize = 0) begin select @PageCount = @PageCount / @PageSize end if(@PageCount % @PageSize = 1) begin select @PageCount = @PageCount / @PageSize + 1 end if(@PageIndex > @PageCount) begin set @PageIndex = @PageCount end select * from (select ROW_NUMBER() over(order by WId) as RowT,* from [WuLianWangInfo]) as Wpage join [JueSe] Jue on Jue.JId = Wpage.JsId where RowT between (@PageIndex - 1) * @PageSize and @PageSize end drop proc Proc_Page declare @a int declare @b int exec Proc_Page 1,2,@a out select @a 总页数