collections模块
# 该模块内部提供了一些高阶的数据类型
1.namedtuple(具名元组)
from collections import namedtuple
'''
namedtuple('名称',[名字1,名字2,....])
res = namedtuple('名称,'名字1 名字2 ...')
res1 = res('值1','值2')
print(res1) # 结果为: 名称(名字1='值1',名字2='值2')
print(res1.名字1) # 结果为 : 值1
'''
point = namedtuple('坐标',['x','y'])
res = point('11','22')
print(res) # 坐标(x=11, y=22)
print(res.x) # 11
print(res.y) # 22
#### 可以直接在字符串中空格隔开写
point = namedtuple('坐标', 'x y z')
res = point(11, 22, 33)
print(res) # 坐标(x=11, y=22, z=33)
### 扑克模拟
card = namedtuple('扑克','花色 点数')
card1 = card('♦','A')
print(card1) # 扑克(花色='♦', 点数='A')
print(card1.花色) # ♦
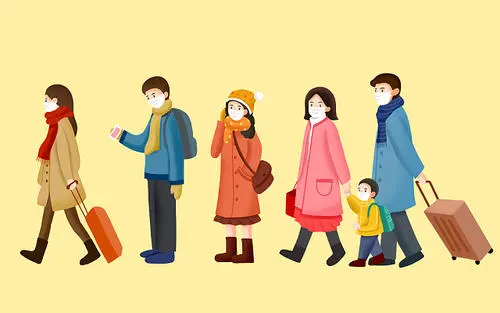
- 队列
队列模式
import queue # 内置队列模块FIFO
# 初始化队列
q = queue.Queue()
# 往队列中添加元素
q.put('first')
q.put('second')
q.put('third')
# 从队列中获取元素
print(q.get())
print(q.get())
print(q.get())
# print(q.get()) # 如果值取没了会原地等待 不会结束也不会报错
- 双端队列
from collections import deque
q=deque([11,22,33])
q.append(44) # 从右边末尾添加
q.appendleft(55) # 从左边添加
print(q.pop()) #从右边取值
print(q.popleft()) # 从左边取值
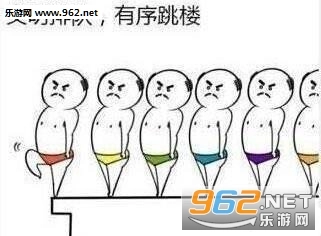
- 有序字典
# 如果直接用dict转为字典时 字典是无序的python3默认排序
order_dict = dict([('name', 'jason'), ('pwd', 123), ('hobby', 'study')])
print(order_dict)
{'hobby': 'study', 'pwd': 123, 'name': 'jason'}
# 如果在python2执行 字典是无序的
from collections import OrderedDict
normal_dict = OrderedDict([('name', 'jason'), ('pwd', 123), ('hobby', 'study')])
print(normal_dict)
## OrderedDict([('name', 'jason'), ('pwd', 123), ('hobby', 'study')])
normal_dict['xxx'] = 1111
print(normal_dict) ## 默认在后面添加
# OrderedDict([('name', 'jason'), ('pwd', 123), ('hobby', 'study'), ('xxx', 1111)])
order_dict = dict([('name', 'jason'), ('pwd', 123), ('hobby', 'study')])
print(order_dict)
order_dict['ttt'] = 2222
## 如果字典添加新的键值对 则是无序添加的
# {'hobby': 'study', 'pwd': 123, 'ttt': 2222, 'name': 'jason'}
- 默认值字典
from collections import defaultdict
values = [11, 22, 33,44,55,66,77,88,99,90]
my_dict = defaultdict(list)
for value in values:
if value>60:
my_dict['k1'].append(value)
else:
my_dict['k2'].append(value)
print(my_dict)
- 计数器
# 统计字符串中每个字符出现的次数
普通方法:
res = 'abcdeackjisdhvnvnvnvnbbvcccc'
new_dict = {}
for i in res:
if i not in new_dict:
new_dict[i] = 1
else:
new_dict[i] += 1
print(new_dict)
使用计数器方法:
from collections import Counter
ret = Counter(res)
print(ret)
Counter({'c': 6, 'v': 5, 'n': 4, 'b': 3, 'a': 2, 'd': 2, 'e': 1, 'k': 1, 'j': 1, 'i': 1, 's': 1, 'h': 1})
# 也可以统计列表中出现的次数
ret =Counter(['the', 'foo', 'the', 'foo', 'the', 'defenestration', 'the'])
print(ret)
Counter({'the': 4, 'foo': 2, 'defenestration': 1})
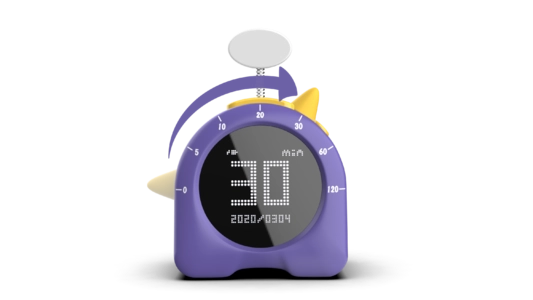