tf的常见用法
BEGIN:
import tensorflow as tf
1、tf.constant:创建一个常数张量,传入list或者数值或字符串来填充
tf.constant([1,5,6,3,4,7,8,9])
###输出:
<tf.Tensor: id=7, shape=(8,), dtype=int32, numpy=array([1, 5, 6, 3, 4, 7, 8, 9])>
tensor = tf.constant(-1.0, shape=[2, 3])
print(tensor)
###输出:
tf.Tensor(
[[-1. -1. -1.]
[-1. -1. -1.]], shape=(2, 3), dtype=float32)
import tensorflow as tf mss = tf.constant("Welcom to tensorflow!\n") with tf.Session() as sess: print(sess.run(mss).decode())
###输出:
Welcom to tensorflow!
2、tf.nn.conv2d:创在TensorFlow中使用tf.nn.conv2d实现卷积操作
tf.nn.conv2d( input, filter, strides, padding, use_cudnn_on_gpu=True, data_format='NHWC', dilations=[1, 1, 1, 1], name=None )
input:输入图像,具有[batch,in_height,in_width,in_channels]这样的4维shape,分别是图片数量、图片高度、图片宽度、图片通道数(灰度图为1,彩图为3),数据
类型为float32或float64。
filter:CNN中的卷积核,shape是[filter_height,filter_width,in_channels,out_channels]:滤波器高度、宽度、图像通道数、滤波器个数,数据类型和input相同。
padding:定义元素边框和元素内容之间的空间,只能是‘SAME’(边缘填充)或者‘VALID’(边缘不填充)。
3、tf.zeros:创建一个全零矩阵
tf.zeros([2,3],'int32')
###输出:
<tf.Tensor: id=15, shape=(2, 3), dtype=int32, numpy=
array([[0, 0, 0],
[0, 0, 0]])>
4、tf.truncated_normal:从截断的正态分布中输出随机值。
生成的值服从具有指定平均值和标准偏差的正态分布,如果生成的值大于平均值2个标准偏差的值则丢弃重新选择。
在正态分布的曲线中:
横轴区间(μ-σ,μ+σ)内的面积为68.268949%。
横轴区间(μ-2σ,μ+2σ)内的面积为95.449974%。
横轴区间(μ-3σ,μ+3σ)内的面积为99.730020%。
X落在(μ-3σ,μ+3σ)以外的概率小于千分之三,在实际问题中常认为相应的事件是不会发生的,基本上可以把区间(μ-3σ,μ+3σ)看作是随机变量X实际可能的取值区间,这称
之为正态分布的“3σ”原则。
在tf.truncated_normal中如果x的取值在区间(μ-2σ,μ+2σ)之外则重新进行选择。这样保证了生成的值都在均值附近。
tf.truncated_normal( shape, mean=0.0, sttdev=1.0, dtype=tf.float32, seed=None, name=None )
shape:一维张量,输出张量
mean:正态分布的均值
stddev:正态分布的标准差
dtype:输出的类型
seed:一个整数,当设置之后,每次生成的随机数都一样
name:操作的名字
举个栗子:
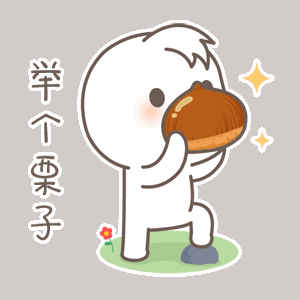
import tensorflow as tf c = tf.truncated_normal(shape=[3, 3], mean=0, stddev=1) with tf.Session() as sess: print(sess.run(c))
运行结果:
5、tf.random_normal:从正态分布中输出随机值
tf.random_normal( shape, mean=0.0, sttdev=1.0, dtype=tf.float32, seed=None, name=None )
shape:一维张量,输出张量
mean:正态分布的均值
stddev:正态分布的标准差
dtype:输出的类型
seed:一个整数,当设置之后,每次生成的随机数都一样
name:操作的名字
例如:
import tensorflow as tf a = tf.Variable(tf.random_normal([2,2],seed=1)) b = tf.Variable(tf.truncated_normal([2,2],seed=2)) init = tf.global_variables_initializer() with tf.Session() as sess: sess.run(init) print('\n','a:') print(sess.run(a)) print('\n','b:') print(sess.run(b))
输出
指定seed之后,a的值不变,b的值也不变。(即多次运行的结果是一样的)
全0矩阵:tf.zeros([2,3],int32)
全1矩阵:tf.ones([2,3],int32)
全为指定数字矩阵:tf.fill([2,3],9)
给定常量:tf.constant
矩阵对应位置相乘:*
矩阵变量:tf.Variable
矩阵乘法:tf.matmul
全1矩阵:tf.ones([2,3],int32)
全为指定数字矩阵:tf.fill([2,3],9)
给定常量:tf.constant
矩阵对应位置相乘:*
矩阵变量:tf.Variable
矩阵乘法:tf.matmul
均匀分布:tf.random_uniform
Gamma分布:tf.random_gamma
限制张量值得范围:tf.clip_by_value(y, 1e-10, 1.0)
Gamma分布:tf.random_gamma
限制张量值得范围:tf.clip_by_value(y, 1e-10, 1.0)
END.
勇气,不过就是人在无路可退时那最后的孤注一掷。