C++ STL(二)string与wstring互转
1.使用windows api方式转换
MultiByteToWideChar()
WideCharToMultiByte()
需要包含头文件<Windows.h>
1 void StringToWstring_WindowApi(const string &str, wstring &wstr) 2 { 3 int nLen = MultiByteToWideChar(CP_ACP, 0, str.c_str(), str.size(), NULL, 0); 4 wchar_t* buffer = new wchar_t[nLen + 1]; 5 6 MultiByteToWideChar(CP_ACP, 0, str.c_str(), str.size(), buffer, nLen); 7 8 buffer[nLen] = '\0'; //字符串断尾 9 wstr = buffer; //赋值 10 delete[] buffer; //删除缓冲区 11 } 12 void WstringToString_WindowsApi(const wstring &wstr, string &str) 13 { 14 int nLen = WideCharToMultiByte(CP_ACP, 0, wstr.c_str(), wstr.size(), NULL, 0, NULL, NULL); 15 char* buffer = new char[nLen + 1]; 16 WideCharToMultiByte(CP_ACP, 0, wstr.c_str(), wstr.size(), buffer, nLen, NULL, NULL); 17 buffer[nLen] = '\0'; //字符串断尾 18 str = buffer; //赋值 19 delete[] buffer; //删除缓冲区 20 }
2.使用ATL宏方式转换
A2W()
W2A()
需要包含头文件<atlconv.h>
使用API上一行加上USES_CONVERSION宏
注意:这个宏从堆栈上分配内存,直到调用它的函数返回,该内存不会被释放.千万别在循环中使用宏
1 void StringToWstring_ATL(const string &str, wstring &wstr) 2 { 3 USES_CONVERSION; 4 wstr = A2W(str.c_str()); 5 } 6 void WstringToString_ATL(const wstring &wstr, string &str) 7 { 8 USES_CONVERSION; 9 str = W2A(wstr.c_str()); 10 }
3.使用CRT库方式转换(这种方式可以跨平台)
mbstowwcs()
wcstombs()
需要包含头文件<locale.h> 设置locale
1 void StringToWstring_CRT(const string &str, wstring &wstr) 2 { 3 string curLocale = setlocale(LC_ALL,NULL); //curLocale = "C" 4 setlocale(LC_ALL, "chs"); 5 int nLen = str.size() + 1; 6 mbstowcs((wchar_t*)wstr.c_str(), str.c_str(), nLen); 7 setlocale(LC_ALL, curLocale.c_str()); 8 9 return ; 10 } 11 void WstringToString_CRT(const wstring &wstr, string &str) 12 { 13 string curLocale = setlocale(LC_ALL,NULL); //curLocale = "C" 14 setlocale(LC_ALL, "chs"); 15 int nLen = wstr.size() + 1; 16 wcstombs((char*)str.c_str(), wstr.c_str(), nLen); 17 setlocale(LC_ALL, curLocale.c_str()); 18 19 return ; 20 }
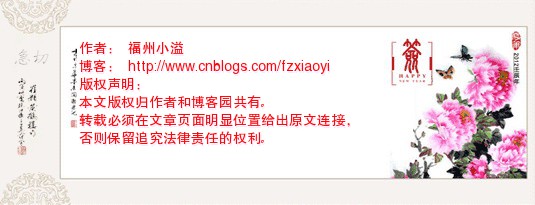