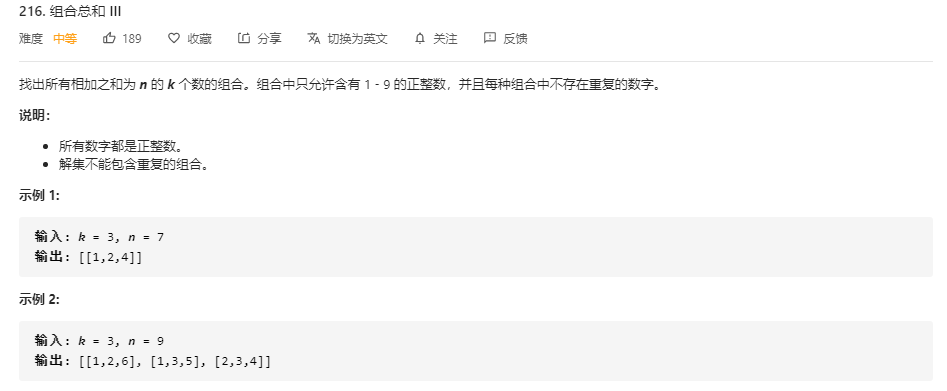
package com.example.lettcode.dailyexercises;
import java.util.ArrayList;
import java.util.List;
/**
* @Class CombinationSum3
* @Description 216 组合总和III
* 找出所有相加之和为 n 的 k 个数的组合。组合中只允许含有 1 - 9 的正整数,并且每种组合中不存在重复的数字。
* <p>
* 说明:
* 所有数字都是正整数。
* 解集不能包含重复的组合。
* <p>
* 示例 1:
* 输入: k = 3, n = 7
* 输出: [[1,2,4]]
* <p>
* 示例 2:
* 输入: k = 3, n = 9
* 输出: [[1,2,6], [1,3,5], [2,3,4]]
* @Author
* @Date 2020/9/11
**/
public class CombinationSum3 {
public static List<List<Integer>> combinationSum3(int k, int n) {
if (k <= 0 || n <= 0) {
return new ArrayList<>();
}
List<List<Integer>> res = new ArrayList<>();
List<Integer> integerList = new ArrayList<>();
int[] combines = new int[]{1, 2, 3, 4, 5, 6, 7, 8, 9};
recur(res, integerList, combines, k, n, 0);
return res;
}
// 回溯
public static void recur(List<List<Integer>> res, List<Integer> integers, int[] combines, int k, int n, int idx) {
// 判断找到的一组列表是否符合要求
if (n == 0 && integers.size() == k) {
res.add(new ArrayList<>(integers));
return;
}
if (idx >= combines.length || integers.size() > k) {
return;
}
// 包含当前位置的元素
if (n - combines[idx] >= 0) {
integers.add(combines[idx]);
recur(res, integers, combines, k, n - combines[idx], idx + 1);
integers.remove(integers.size() - 1);
}
// 跳过当前位置的元素
recur(res, integers, combines, k, n, idx + 1);
}
public static void main(String[] args) {
int k = 3;
int n = 7;
List<List<Integer>> res = combinationSum3(k, n);
System.out.println("combinationSum3 demo01 result:");
for (List<Integer> integerList : res) {
for (Integer integer : integerList) {
System.out.print("," + integer);
}
System.out.println();
}
k = 3;
n = 9;
res = combinationSum3(k, n);
System.out.println("CombinationSum3 demo02 result:");
for (List<Integer> integerList : res) {
for (Integer integer : integerList) {
System.out.print("," + integer);
}
System.out.println();
}
k = 3;
n = 15;
res = combinationSum3(k, n);
System.out.println("CombinationSum3 demo03 result:");
for (List<Integer> integerList : res) {
for (Integer integer : integerList) {
System.out.print("," + integer);
}
System.out.println();
}
}
}